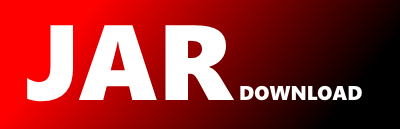
com.bazaarvoice.emodb.job.util.JobStatusUtil Maven / Gradle / Ivy
package com.bazaarvoice.emodb.job.util;
import com.bazaarvoice.emodb.common.json.JsonHelper;
import com.bazaarvoice.emodb.job.api.JobStatus;
import com.bazaarvoice.emodb.job.api.JobType;
import com.fasterxml.jackson.core.type.TypeReference;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import com.google.inject.util.Types;
import java.lang.reflect.Type;
/**
* Utility class for handling JobStatus object in a type-safe manner.
*/
abstract public class JobStatusUtil {
/**
* Optimization to perform narrowing of JobStatus objects by caching their TypeReferences based on the JobType.
*/
private static final LoadingCache, TypeReference>> _jobStatusTypeReferences =
CacheBuilder.newBuilder()
.build(new CacheLoader, TypeReference>>() {
@Override
public TypeReference> load(final JobType, ?> jobType)
throws Exception {
return new TypeReference>() {
private Type _type = Types.newParameterizedType(
JobStatus.class, jobType.getRequestType(), jobType.getResultType());
@Override
public Type getType() {
return _type;
}
};
}
});
public static JobStatus narrow(Object object, JobType jobType) {
return JsonHelper.convert(object, getTypeReferenceForJobType(jobType));
}
@SuppressWarnings("unchecked")
private static TypeReference> getTypeReferenceForJobType(JobType jobType) {
return (TypeReference) _jobStatusTypeReferences.getUnchecked(jobType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy