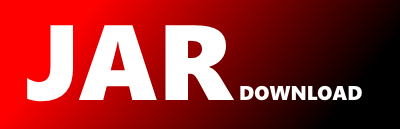
com.bazaarvoice.jolt.modifier.function.Strings Maven / Gradle / Ivy
/*
* Copyright 2013 Bazaarvoice, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.bazaarvoice.jolt.modifier.function;
import com.bazaarvoice.jolt.common.Optional;
import java.util.List;
@SuppressWarnings( "deprecated" )
public class Strings {
public static final class toLowerCase extends Function.SingleFunction {
@Override
protected Optional applySingle( final Object arg ) {
return arg == null ? Optional.of( null ) : Optional.of( arg.toString().toLowerCase() );
}
}
public static final class toUpperCase extends Function.SingleFunction {
@Override
protected Optional applySingle( final Object arg ) {
return arg == null ? Optional.of( null ): Optional.of( arg.toString().toUpperCase() );
}
}
public static final class concat extends Function.ListFunction {
@Override
protected Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy