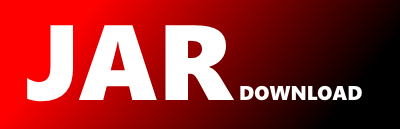
com.bazaarvoice.jolt.modifier.spec.ModifierCompositeSpec Maven / Gradle / Ivy
/*
* Copyright 2013 Bazaarvoice, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.bazaarvoice.jolt.modifier.spec;
import com.bazaarvoice.jolt.common.ComputedKeysComparator;
import com.bazaarvoice.jolt.common.ExecutionStrategy;
import com.bazaarvoice.jolt.common.Optional;
import com.bazaarvoice.jolt.common.pathelement.ArrayPathElement;
import com.bazaarvoice.jolt.common.pathelement.LiteralPathElement;
import com.bazaarvoice.jolt.common.pathelement.PathElement;
import com.bazaarvoice.jolt.common.pathelement.StarAllPathElement;
import com.bazaarvoice.jolt.common.pathelement.StarDoublePathElement;
import com.bazaarvoice.jolt.common.pathelement.StarRegexPathElement;
import com.bazaarvoice.jolt.common.pathelement.StarSinglePathElement;
import com.bazaarvoice.jolt.common.spec.BaseSpec;
import com.bazaarvoice.jolt.common.spec.OrderedCompositeSpec;
import com.bazaarvoice.jolt.common.tree.ArrayMatchedElement;
import com.bazaarvoice.jolt.common.tree.MatchedElement;
import com.bazaarvoice.jolt.common.tree.WalkedPath;
import com.bazaarvoice.jolt.exception.SpecException;
import com.bazaarvoice.jolt.modifier.DataType;
import com.bazaarvoice.jolt.modifier.OpMode;
import com.bazaarvoice.jolt.modifier.TemplatrSpecBuilder;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* Composite spec is non-leaf level spec that contains one or many child specs and processes
* them based on a pre-determined execution strategy
*/
public class ModifierCompositeSpec extends ModifierSpec implements OrderedCompositeSpec {
private static final HashMap orderMap;
private static final ComputedKeysComparator computedKeysComparator;
static {
orderMap = new HashMap<>();
orderMap.put( ArrayPathElement.class, 1 );
orderMap.put( StarRegexPathElement.class, 2 );
orderMap.put( StarDoublePathElement.class, 3 );
orderMap.put( StarSinglePathElement.class, 4 );
orderMap.put( StarAllPathElement.class, 5 );
computedKeysComparator = ComputedKeysComparator.fromOrder(orderMap);
}
private final Map literalChildren;
private final List computedChildren;
private final ExecutionStrategy executionStrategy;
private final DataType specDataType;
public ModifierCompositeSpec( final String key, final Map spec, final OpMode opMode, TemplatrSpecBuilder specBuilder ) {
super(key, opMode);
Map literals = new LinkedHashMap<>();
ArrayList computed = new ArrayList<>();
List children = specBuilder.createSpec( spec );
// remember max explicit index from spec to expand input array at runtime
// need to validate spec such that it does not specify both array and literal path element
int maxExplicitIndexFromSpec = -1, confirmedMapAtIndex = -1, confirmedArrayAtIndex = -1;
for(int i=0; i -1 && confirmedArrayAtIndex > -1) {
throw new SpecException( opMode.name() + " RHS cannot mix int array index and string map key, defined spec for " + key + " contains: " + children.get( confirmedMapAtIndex ).pathElement.getCanonicalForm() + " conflicting " + children.get( confirmedArrayAtIndex ).pathElement.getCanonicalForm() );
}
}
// set the dataType from calculated indexes
specDataType = DataType.determineDataType( confirmedArrayAtIndex, confirmedMapAtIndex, maxExplicitIndexFromSpec );
// Only the computed children need to be sorted
Collections.sort( computed, computedKeysComparator );
computed.trimToSize();
literalChildren = Collections.unmodifiableMap( literals );
computedChildren = Collections.unmodifiableList( computed );
// extract generic execution strategy
executionStrategy = determineExecutionStrategy();
}
@Override
@SuppressWarnings( "unchecked" )
public void applyElement( final String inputKey, Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy