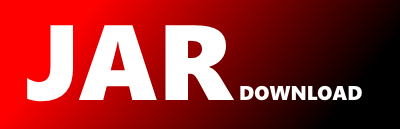
com.bazaarvoice.jolt.JsonUtil Maven / Gradle / Ivy
/*
* Copyright 2013 Bazaarvoice, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.bazaarvoice.jolt;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
/**
* Utility methods for getting JSON content loaded from
* the filesystem, the classpath, or in memory Strings.
*
* Also has methods to serialize Java object to JSON strings.
*
* Implementations of this interface can specify their own
* Jackson ObjectMapper so that Domain specific Java Objects
* can successfully be serialized and de-serialized.
*/
public interface JsonUtil {
// DE-SERIALIZATION
Object jsonToObject( String json );
Object jsonToObject( String json , String charset );
Object jsonToObject( InputStream in );
Map jsonToMap( String json );
Map jsonToMap( String json, String charset );
Map jsonToMap( InputStream in );
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy