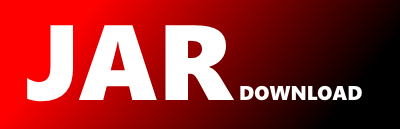
com.bazaarvoice.maven.plugin.s3repo.rebuild.RebuildContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3repo-maven-plugin Show documentation
Show all versions of s3repo-maven-plugin Show documentation
Create or update an S3 YUM repository.
package com.bazaarvoice.maven.plugin.s3repo.rebuild;
import com.amazonaws.services.s3.AmazonS3;
import com.bazaarvoice.maven.plugin.s3repo.S3RepositoryPath;
import com.bazaarvoice.maven.plugin.s3repo.support.LocalYumRepoFacade;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
final class RebuildContext {
private AmazonS3 s3Session;
private S3RepositoryPath s3RepositoryPath;
private S3RepositoryPath s3TargetRepositoryPath; // may be the same as s3RepositoryPath
private LocalYumRepoFacade localYumRepo;
/**
* Here we keep track of a Map of bucket key *prefixes* to full bucket keys that represent SNAPSHOTS of
* the same artifact. For example, we may discover these files in the repository:
*
* - path/to/awesome-artifact-1.4-SNAPSHOT1.noarch.rpm
* - path/to/awesome-artifact-1.4-SNAPSHOT-2.noarch.rpm
* - path/to/awesome-artifact-1.4-SNAPSHOT-blah.noarch.rpm
*
* This will result in the following map entry:
* path/to/awesome-artifact-1.4 => [ path/to/awesome-artifact-1.4-SNAPSHOT1.noarch.rpm, ...]
*
* The heuristic used here looks for any artifacts that contain the word "SNAPSHOT" and uses all characters
* before this string as the key.
*/
private final Map> bucketKeyPrefixToSnapshots = new HashMap>();
private final List snapshotsToDeleteRemotely = new ArrayList();
private final List snapshotsToRenameRemotely = new ArrayList();
/** Repo-relative file paths that are explicitly excluded. */
private final Set excludedFiles = new HashSet();
/** Repo-relative file paths that we will delete remotely. */
private final Set excludedFilesToDeleteFromSource = new HashSet();
private final Set excludedFilesToDeleteFromTarget = new HashSet();
/** Files that exist in target. */
private final Set filesFromTargetRepo = new HashSet();
public AmazonS3 getS3Session() {
return s3Session;
}
public void setS3Session(AmazonS3 s3Session) {
this.s3Session = s3Session;
}
public boolean sourceAndTargetRepositoryAreSame() {
return s3RepositoryPath.equals(s3TargetRepositoryPath);
}
public void setS3RepositoryPath(S3RepositoryPath s3RepositoryPath) {
this.s3RepositoryPath = s3RepositoryPath;
}
public S3RepositoryPath getS3RepositoryPath() {
return s3RepositoryPath;
}
public void setS3TargetRepositoryPath(S3RepositoryPath path) {
this.s3TargetRepositoryPath = path;
}
public S3RepositoryPath getS3TargetRepositoryPath() {
return s3TargetRepositoryPath;
}
public LocalYumRepoFacade getLocalYumRepo() {
return localYumRepo;
}
public void setLocalYumRepo(LocalYumRepoFacade localYumRepo) {
this.localYumRepo = localYumRepo;
}
public void addSnapshotDescription(SnapshotDescription snapshotDescription) {
List existing = bucketKeyPrefixToSnapshots.get(snapshotDescription.getBucketKeyPrefix());
if (existing == null) {
existing = new ArrayList();
bucketKeyPrefixToSnapshots.put(snapshotDescription.getBucketKeyPrefix(), existing);
}
existing.add(snapshotDescription);
}
public Map> getBucketKeyPrefixToSnapshots() {
return bucketKeyPrefixToSnapshots;
}
public void addSnapshotToDelete(SnapshotDescription toDelete) {
snapshotsToDeleteRemotely.add(toDelete);
}
public List getSnapshotsToDeleteRemotely() {
return snapshotsToDeleteRemotely;
}
public void addSnapshotToRename(RemoteSnapshotRename toRename) {
snapshotsToRenameRemotely.add(toRename);
}
public List getSnapshotsToRenameRemotely() {
return snapshotsToRenameRemotely;
}
public void setExcludedFiles(List repoRelativePaths) {
excludedFiles.clear();
excludedFiles.addAll(repoRelativePaths);
}
/** Repo-relative paths. */
public Set getExcludedFiles() {
return excludedFiles;
}
public void addExcludedFileToDelete(String repoRelativePath, S3RepositoryPath repo) {
if (!repo.equals(s3RepositoryPath) && !repo.equals(s3TargetRepositoryPath)) {
throw new IllegalStateException("repo not source or target: " + repo);
}
if (repo.equals(s3RepositoryPath)) {
excludedFilesToDeleteFromSource.add(repoRelativePath);
}
if (repo.equals(s3TargetRepositoryPath)) {
excludedFilesToDeleteFromTarget.add(repoRelativePath);
}
}
public Set getExcludedFilesToDeleteFromSource() {
return excludedFilesToDeleteFromSource;
}
public Set getExcludedFilesToDeleteFromTarget() {
return excludedFilesToDeleteFromTarget;
}
public void addFileFromTargetRepo(File targetFile) {
this.filesFromTargetRepo.add(targetFile);
}
public Set getFilesFromTargetRepo() {
return filesFromTargetRepo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy