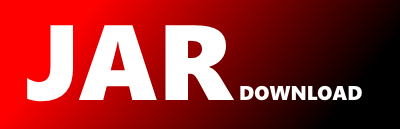
com.bbn.bue.common.evaluation.FMeasureCounts Maven / Gradle / Ivy
package com.bbn.bue.common.evaluation;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import com.google.common.primitives.Doubles;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link _FMeasureCounts}.
*
* Use the builder to create immutable instances:
* {@code FMeasureCounts.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "_FMeasureCounts"})
@Immutable
public final class FMeasureCounts extends com.bbn.bue.common.evaluation._FMeasureCounts {
private final double falsePositives;
private final double falseNegatives;
private final double numPredicted;
private final double numItemsInKey;
private FMeasureCounts(double falsePositives, double falseNegatives, double numPredicted, double numItemsInKey) {
this.falsePositives = falsePositives;
this.falseNegatives = falseNegatives;
this.numPredicted = numPredicted;
this.numItemsInKey = numItemsInKey;
}
/**
* @return The value of the {@code falsePositives} attribute
*/
@JsonProperty
@Override
public double falsePositives() {
return falsePositives;
}
/**
* @return The value of the {@code falseNegatives} attribute
*/
@JsonProperty
@Override
public double falseNegatives() {
return falseNegatives;
}
/**
* @return The value of the {@code numPredicted} attribute
*/
@JsonProperty
@Override
public double numPredicted() {
return numPredicted;
}
/**
* @return The value of the {@code numItemsInKey} attribute
*/
@JsonProperty
@Override
public double numItemsInKey() {
return numItemsInKey;
}
/**
* Copy the current immutable object by setting a value for the {@link _FMeasureCounts#falsePositives() falsePositives} attribute.
* @param value A new value for falsePositives
* @return A modified copy of the {@code this} object
*/
public final FMeasureCounts withFalsePositives(double value) {
double newValue = value;
return validate(new FMeasureCounts(newValue, this.falseNegatives, this.numPredicted, this.numItemsInKey));
}
/**
* Copy the current immutable object by setting a value for the {@link _FMeasureCounts#falseNegatives() falseNegatives} attribute.
* @param value A new value for falseNegatives
* @return A modified copy of the {@code this} object
*/
public final FMeasureCounts withFalseNegatives(double value) {
double newValue = value;
return validate(new FMeasureCounts(this.falsePositives, newValue, this.numPredicted, this.numItemsInKey));
}
/**
* Copy the current immutable object by setting a value for the {@link _FMeasureCounts#numPredicted() numPredicted} attribute.
* @param value A new value for numPredicted
* @return A modified copy of the {@code this} object
*/
public final FMeasureCounts withNumPredicted(double value) {
double newValue = value;
return validate(new FMeasureCounts(this.falsePositives, this.falseNegatives, newValue, this.numItemsInKey));
}
/**
* Copy the current immutable object by setting a value for the {@link _FMeasureCounts#numItemsInKey() numItemsInKey} attribute.
* @param value A new value for numItemsInKey
* @return A modified copy of the {@code this} object
*/
public final FMeasureCounts withNumItemsInKey(double value) {
double newValue = value;
return validate(new FMeasureCounts(this.falsePositives, this.falseNegatives, this.numPredicted, newValue));
}
/**
* This instance is equal to all instances of {@code FMeasureCounts} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof FMeasureCounts
&& equalTo((FMeasureCounts) another);
}
private boolean equalTo(FMeasureCounts another) {
return Double.doubleToLongBits(falsePositives) == Double.doubleToLongBits(another.falsePositives)
&& Double.doubleToLongBits(falseNegatives) == Double.doubleToLongBits(another.falseNegatives)
&& Double.doubleToLongBits(numPredicted) == Double.doubleToLongBits(another.numPredicted)
&& Double.doubleToLongBits(numItemsInKey) == Double.doubleToLongBits(another.numItemsInKey);
}
/**
* Computes a hash code from attributes: {@code falsePositives}, {@code falseNegatives}, {@code numPredicted}, {@code numItemsInKey}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + Doubles.hashCode(falsePositives);
h = h * 17 + Doubles.hashCode(falseNegatives);
h = h * 17 + Doubles.hashCode(numPredicted);
h = h * 17 + Doubles.hashCode(numItemsInKey);
return h;
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
static final class Json extends com.bbn.bue.common.evaluation._FMeasureCounts {
@Nullable java.lang.Double falsePositives;
@Nullable java.lang.Double falseNegatives;
@Nullable java.lang.Double numPredicted;
@Nullable java.lang.Double numItemsInKey;
public void setFalsePositives(double falsePositives) {
this.falsePositives = falsePositives;
}
public void setFalseNegatives(double falseNegatives) {
this.falseNegatives = falseNegatives;
}
public void setNumPredicted(double numPredicted) {
this.numPredicted = numPredicted;
}
public void setNumItemsInKey(double numItemsInKey) {
this.numItemsInKey = numItemsInKey;
}
@Override
public double falsePositives() { throw new UnsupportedOperationException(); }
@Override
public double falseNegatives() { throw new UnsupportedOperationException(); }
@Override
public double numPredicted() { throw new UnsupportedOperationException(); }
@Override
public double numItemsInKey() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator
static FMeasureCounts fromJson(Json json) {
FMeasureCounts.Builder builder = FMeasureCounts.builder();
if (json.falsePositives != null) {
builder.falsePositives(json.falsePositives);
}
if (json.falseNegatives != null) {
builder.falseNegatives(json.falseNegatives);
}
if (json.numPredicted != null) {
builder.numPredicted(json.numPredicted);
}
if (json.numItemsInKey != null) {
builder.numItemsInKey(json.numItemsInKey);
}
return builder.build();
}
private static FMeasureCounts validate(FMeasureCounts instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link _FMeasureCounts} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable FMeasureCounts instance
*/
public static FMeasureCounts copyOf(FMeasureCounts instance) {
if (instance instanceof FMeasureCounts) {
return (FMeasureCounts) instance;
}
return FMeasureCounts.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link com.bbn.bue.common.evaluation.FMeasureCounts FMeasureCounts}.
* @return A new FMeasureCounts builder
*/
public static FMeasureCounts.Builder builder() {
return new FMeasureCounts.Builder();
}
/**
* Builds instances of type {@link com.bbn.bue.common.evaluation.FMeasureCounts FMeasureCounts}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_FALSE_POSITIVES = 0x1L;
private static final long INIT_BIT_FALSE_NEGATIVES = 0x2L;
private static final long INIT_BIT_NUM_PREDICTED = 0x4L;
private static final long INIT_BIT_NUM_ITEMS_IN_KEY = 0x8L;
private long initBits = 0xf;
private double falsePositives;
private double falseNegatives;
private double numPredicted;
private double numItemsInKey;
private Builder() {}
/**
* Fill a builder with attribute values from the provided {@link _FMeasureCounts} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(FMeasureCounts instance) {
Preconditions.checkNotNull(instance, "instance");
falsePositives(instance.falsePositives());
falseNegatives(instance.falseNegatives());
numPredicted(instance.numPredicted());
numItemsInKey(instance.numItemsInKey());
return this;
}
/**
* Initializes the value for the {@link _FMeasureCounts#falsePositives() falsePositives} attribute.
* @param falsePositives The value for falsePositives
* @return {@code this} builder for use in a chained invocation
*/
public final Builder falsePositives(double falsePositives) {
this.falsePositives = falsePositives;
initBits &= ~INIT_BIT_FALSE_POSITIVES;
return this;
}
/**
* Initializes the value for the {@link _FMeasureCounts#falseNegatives() falseNegatives} attribute.
* @param falseNegatives The value for falseNegatives
* @return {@code this} builder for use in a chained invocation
*/
public final Builder falseNegatives(double falseNegatives) {
this.falseNegatives = falseNegatives;
initBits &= ~INIT_BIT_FALSE_NEGATIVES;
return this;
}
/**
* Initializes the value for the {@link _FMeasureCounts#numPredicted() numPredicted} attribute.
* @param numPredicted The value for numPredicted
* @return {@code this} builder for use in a chained invocation
*/
public final Builder numPredicted(double numPredicted) {
this.numPredicted = numPredicted;
initBits &= ~INIT_BIT_NUM_PREDICTED;
return this;
}
/**
* Initializes the value for the {@link _FMeasureCounts#numItemsInKey() numItemsInKey} attribute.
* @param numItemsInKey The value for numItemsInKey
* @return {@code this} builder for use in a chained invocation
*/
public final Builder numItemsInKey(double numItemsInKey) {
this.numItemsInKey = numItemsInKey;
initBits &= ~INIT_BIT_NUM_ITEMS_IN_KEY;
return this;
}
/**
* Builds a new {@link com.bbn.bue.common.evaluation.FMeasureCounts FMeasureCounts}.
* @return An immutable instance of FMeasureCounts
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public FMeasureCounts build() throws IllegalStateException {
checkRequiredAttributes();
return FMeasureCounts.validate(new FMeasureCounts(falsePositives, falseNegatives, numPredicted, numItemsInKey));
}
private boolean falsePositivesIsSet() {
return (initBits & INIT_BIT_FALSE_POSITIVES) == 0;
}
private boolean falseNegativesIsSet() {
return (initBits & INIT_BIT_FALSE_NEGATIVES) == 0;
}
private boolean numPredictedIsSet() {
return (initBits & INIT_BIT_NUM_PREDICTED) == 0;
}
private boolean numItemsInKeyIsSet() {
return (initBits & INIT_BIT_NUM_ITEMS_IN_KEY) == 0;
}
private void checkRequiredAttributes() throws IllegalStateException {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if (!falsePositivesIsSet()) attributes.add("falsePositives");
if (!falseNegativesIsSet()) attributes.add("falseNegatives");
if (!numPredictedIsSet()) attributes.add("numPredicted");
if (!numItemsInKeyIsSet()) attributes.add("numItemsInKey");
return "Cannot build FMeasureCounts, some of required attributes are not set " + attributes;
}
}
}