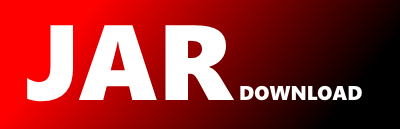
com.bbn.bue.common.math.MathUtils Maven / Gradle / Ivy
The newest version!
package com.bbn.bue.common.math;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Ordering;
import static com.google.common.base.Preconditions.checkArgument;
public final class MathUtils {
private MathUtils() {
throw new UnsupportedOperationException();
}
public static int max(int[] arr) {
checkArgument(arr.length > 0);
int mx = Integer.MIN_VALUE;
for (final int x : arr) {
if (x > mx) {
mx = x;
}
}
return mx;
}
public static int sum(int[] permutation) {
int ret = 0;
for (final int x : permutation) {
ret += x;
}
return ret;
}
public static Optional medianOfIntegers(Iterable sizes) {
final ImmutableList sorted = Ordering.natural().immutableSortedCopy(sizes);
if(sorted.size() == 0) {
return Optional.absent();
}
if (sorted.size() % 2 == 0) {
return Optional.of(0.5 * (sorted.get(sorted.size() / 2) + sorted.get(sorted.size() / 2 - 1)));
} else {
return Optional.of((double) sorted.get(sorted.size() / 2));
}
}
public static Optional medianOfDoubles(Iterable sizes) {
final ImmutableList sorted = Ordering.natural().immutableSortedCopy(sizes);
if(sorted.size() == 0) {
return Optional.absent();
}
if (sorted.size() % 2 == 0) {
return Optional.of(0.5 * (sorted.get(sorted.size() / 2) + sorted.get(sorted.size() / 2 - 1)));
} else {
return Optional.of(sorted.get(sorted.size() / 2));
}
}
public static Function, Optional> medianOfDoublesFunction() {
return new Function, Optional>() {
@Override
public Optional apply(final Iterable input) {
return medianOfDoubles(input);
}
};
}
public static double xLogX(double d) {
if (d == 0.0) {
return 0.0;
} else {
return d * Math.log(d);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy