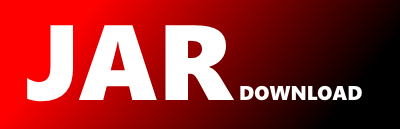
org.frameworkset.remote.EventRPCDispatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bboss-event Show documentation
Show all versions of bboss-event Show documentation
bboss is a j2ee framework include aop/ioc,mvc,persistent,taglib,rpc,event ,bean-xml serializable ,schedle and so on.http://www.bbossgroups.com
The newest version!
package org.frameworkset.remote;
import java.util.ArrayList;
import java.util.List;
import org.frameworkset.event.Event;
import org.jgroups.Address;
import org.jgroups.JChannel;
import org.jgroups.ReceiverAdapter;
import org.jgroups.blocks.RequestOptions;
import org.jgroups.blocks.ResponseMode;
import org.jgroups.blocks.RpcDispatcher;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class EventRPCDispatcher extends ReceiverAdapter implements
org.frameworkset.spi.InitializingBean,
org.frameworkset.spi.DisposableBean {
private static Logger log = LoggerFactory.getLogger(EventRPCDispatcher.class);
private JChannel channel;
private RpcDispatcher rpcDispatcher;
RpcDispatcherAnycastServerObject target;
EventRemoteService eventService;
final String GROUP = "EventRpcDispatcherMultipleCalls";
public EventRPCDispatcher() {
}
public Address getLocalAddress()
{
return this.channel.getAddress();
}
public boolean containSelf( List addresses)
{
Address address = this.channel.getAddress();
for(Address ad:addresses)
{
if(ad.equals(address) )
return true;
}
return false;
}
public List removeSelf( List addresses)
{
Address address = this.channel.getAddress();
List temp = new ArrayList();
temp.addAll(addresses);
temp.remove(address);
return temp;
}
public void callRemote(boolean excludeSelf, Event> event)
throws Exception {
// we need to copy the vector, otherwise the modification below will
// throw an exception because the underlying
// vector is unmodifiable
List v = new ArrayList();
v.addAll(channel.getView().getMembers());
if (excludeSelf) {
v.remove(channel.getAddress());
if(v.size() == 0)
return ;
boolean use_anycasting = true;
rpcDispatcher.callRemoteMethods(v, "remoteEventChange",
new Object[] {event}, new Class[] { Event.class },
new RequestOptions(ResponseMode.GET_NONE, 0,use_anycasting));
} else {
rpcDispatcher.callRemoteMethods(null, "remoteEventChange",
new Object[] {event}, new Class[] { Event.class },
new RequestOptions(ResponseMode.GET_NONE, 0));
}
}
public List getAddresses()
{
if(this.channel != null)
{
List v = new ArrayList();
v.addAll(channel.getView().getMembers());
return v;
}
return null;
}
public void callRemote( List addresses, Event> event)
throws Exception {
// we need to copy the vector, otherwise the modification below will
// throw an exception because the underlying
// vector is unmodifiable
boolean use_anycasting = true;
rpcDispatcher.callRemoteMethods(addresses, "remoteEventChange",
new Object[] {event}, new Class[] { Event.class },
new RequestOptions(ResponseMode.GET_NONE, 0,use_anycasting));
}
public void callRemote( Event> event) throws Exception {
callRemote(false, event);
}
private void shutdown() {
if(channel != null)
{
log.debug("Event RpcDispatcher Service shutdownding.");
channel.close();
log.debug("Event RpcDispatcher Service shutdownded.");
}
}
public void init() throws Exception {
log.debug("Event RpcDispatcher Service starting.");
channel = createChannel();
rpcDispatcher = new RpcDispatcher(channel, eventService);
rpcDispatcher.setMembershipListener(this);
rpcDispatcher.setStateListener(this);
channel.connect(GROUP);
log.debug("Event RpcDispatcher Service started.");
// BaseApplicationContext.addShutdownHook(new Runnable() {
//
// public void run() {
// shutdown();
// }
//
// });
}
private JChannel createChannel() throws Exception {
return new JChannel(EventUtils.getProtocolConfigFile());
}
public void destroy() throws Exception {
shutdown();
}
public void afterPropertiesSet() throws Exception {
// channel = createChannel();
// rpcDispatcher = new RpcDispatcher(channel, this, this, eventService);
//
// channel.connect(GROUP);
init() ;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy