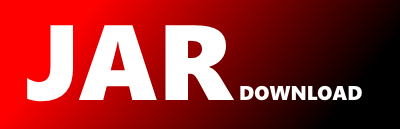
com.frameworkset.common.poolman.CallableParam Maven / Gradle / Ivy
Show all versions of bboss-persistent Show documentation
/*
* Copyright 2008 biaoping.yin
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.frameworkset.common.poolman;
import com.frameworkset.common.poolman.util.SQLUtil;
import org.frameworkset.persitent.type.BaseTypeMethod;
import org.frameworkset.persitent.type.procdule.*;
import java.sql.Types;
public class CallableParam extends Param
{
/**
* Registers the OUT parameter in ordinal position
* parameterIndex
to the JDBC type
* sqlType
. All OUT parameters must be registered
* before a stored procedure is executed.
*
* The JDBC type specified by sqlType
for an OUT
* parameter determines the Java type that must be used
* in the get
method to read the value of that parameter.
*
* If the JDBC type expected to be returned to this output parameter
* is specific to this particular database, sqlType
* should be java.sql.Types.OTHER
. The method
* {@link #getObject} retrieves the value.
*
* @param parameterIndex the first parameter is 1, the second is 2,
* and so on
* @param sqlType the JDBC type code defined by java.sql.Types
.
* If the parameter is of JDBC type NUMERIC
* or DECIMAL
, the version of
* registerOutParameter
that accepts a scale value
* should be used.
*
* @see Types
*/
static final BaseTypeMethod registerOutParameter_int_parameterIndex_int_sqlType = new Parameter_int_parameterIndex_int_sqlType();//"registerOutParameter(int parameterIndex, int sqlType)";
/**
* Registers the parameter in ordinal position
* parameterIndex
to be of JDBC type
* sqlType
. This method must be called
* before a stored procedure is executed.
*
* The JDBC type specified by sqlType
for an OUT
* parameter determines the Java type that must be used
* in the get
method to read the value of that parameter.
*
* This version of registerOutParameter
should be
* used when the parameter is of JDBC type NUMERIC
* or DECIMAL
.
*
* @param parameterIndex the first parameter is 1, the second is 2,
* and so on
* @param sqlType the SQL type code defined by java.sql.Types
.
* @param scale the desired number of digits to the right of the
* decimal point. It must be greater than or equal to zero.
* @see Types
*/
static final BaseTypeMethod registerOutParameter_int_parameterIndex_int_sqlType_int_scale = new Parameter_int_parameterIndex_int_sqlType_int_scale();//"registerOutParameter(int parameterIndex, int sqlType, int scale)";
/**
* Registers the designated output parameter. This version of
* the method registerOutParameter
* should be used for a user-defined or REF
output parameter. Examples
* of user-defined types include: STRUCT
, DISTINCT
,
* JAVA_OBJECT
, and named array types.
*
* Before executing a stored procedure call, you must explicitly
* call registerOutParameter
to register the type from
* java.sql.Types
for each
* OUT parameter. For a user-defined parameter, the fully-qualified SQL
* type name of the parameter should also be given, while a REF
* parameter requires that the fully-qualified type name of the
* referenced type be given. A JDBC driver that does not need the
* type code and type name information may ignore it. To be portable,
* however, applications should always provide these values for
* user-defined and REF
parameters.
*
* Although it is intended for user-defined and REF
parameters,
* this method may be used to register a parameter of any JDBC type.
* If the parameter does not have a user-defined or REF
type, the
* typeName parameter is ignored.
*
*
Note: When reading the value of an out parameter, you
* must use the getter method whose Java type corresponds to the
* parameter's registered SQL type.
*
* @param paramIndex the first parameter is 1, the second is 2,...
* @param sqlType a value from {@link java.sql.Types}
* @param typeName the fully-qualified name of an SQL structured type
* @see Types
* @since 1.2
*/
static final BaseTypeMethod registerOutParameter_int_paramIndex_int_sqlType_String_typeName = new Parameter_int_paramIndex_int_sqlType_String_typeName();//"registerOutParameter (int paramIndex, int sqlType, String typeName)";
//--------------------------JDBC 3.0-----------------------------
/**
* Registers the OUT parameter named
* parameterName
to the JDBC type
* sqlType
. All OUT parameters must be registered
* before a stored procedure is executed.
*
* The JDBC type specified by sqlType
for an OUT
* parameter determines the Java type that must be used
* in the get
method to read the value of that parameter.
*
* If the JDBC type expected to be returned to this output parameter
* is specific to this particular database, sqlType
* should be java.sql.Types.OTHER
. The method
* {@link #getObject} retrieves the value.
* @param parameterName the name of the parameter
* @param sqlType the JDBC type code defined by java.sql.Types
.
* If the parameter is of JDBC type NUMERIC
* or DECIMAL
, the version of
* registerOutParameter
that accepts a scale value
* should be used.
* @exception SQLException if a database access error occurs
* @since 1.4
* @see Types
*/
static final BaseTypeMethod registerOutParameter_String_parameterName_int_sqlType = new Parameter_String_parameterName_int_sqlType();//"registerOutParameter(String parameterName, int sqlType)";
/**
* Registers the parameter named
* parameterName
to be of JDBC type
* sqlType
. This method must be called
* before a stored procedure is executed.
*
* The JDBC type specified by sqlType
for an OUT
* parameter determines the Java type that must be used
* in the get
method to read the value of that parameter.
*
* This version of registerOutParameter
should be
* used when the parameter is of JDBC type NUMERIC
* or DECIMAL
.
* @param parameterName the name of the parameter
* @param sqlType SQL type code defined by java.sql.Types
.
* @param scale the desired number of digits to the right of the
* decimal point. It must be greater than or equal to zero.
* @since 1.4
* @see Types
*/
static final BaseTypeMethod registerOutParameter_String_parameterName_int_sqlType_int_scale = new Parameter_String_parameterName_int_sqlType_int_scale();//"registerOutParameter(String parameterName, int sqlType, int scale)";
/**
* Registers the designated output parameter. This version of
* the method registerOutParameter
* should be used for a user-named or REF output parameter. Examples
* of user-named types include: STRUCT, DISTINCT, JAVA_OBJECT, and
* named array types.
*
* Before executing a stored procedure call, you must explicitly
* call registerOutParameter
to register the type from
* java.sql.Types
for each
* OUT parameter. For a user-named parameter the fully-qualified SQL
* type name of the parameter should also be given, while a REF
* parameter requires that the fully-qualified type name of the
* referenced type be given. A JDBC driver that does not need the
* type code and type name information may ignore it. To be portable,
* however, applications should always provide these values for
* user-named and REF parameters.
*
* Although it is intended for user-named and REF parameters,
* this method may be used to register a parameter of any JDBC type.
* If the parameter does not have a user-named or REF type, the
* typeName parameter is ignored.
*
*
Note: When reading the value of an out parameter, you
* must use the getXXX
method whose Java type XXX corresponds to the
* parameter's registered SQL type.
*
* @param parameterName the name of the parameter
* @param sqlType a value from {@link java.sql.Types}
* @param typeName the fully-qualified name of an SQL structured type
* @exception SQLException if a database access error occurs
* @see Types
* @since 1.4
*/
static final BaseTypeMethod registerOutParameter_String_parameterName_int_sqlType_String_typeName = new Parameter_String_parameterName_int_sqlType_String_typeName();//"registerOutParameter (String parameterName, int sqlType, String typeName)";
/**
* Sets the designated parameter to the given input stream, which will have
* the specified number of bytes.
* When a very large ASCII value is input to a LONGVARCHAR
* parameter, it may be more practical to send it via a
* java.io.InputStream
. Data will be read from the stream
* as needed until end-of-file is reached. The JDBC driver will
* do any necessary conversion from ASCII to the database char format.
*
*
Note: This stream object can either be a standard
* Java stream object or your own subclass that implements the
* standard interface.
*
* @param parameterName the name of the parameter
* @param x the Java input stream that contains the ASCII parameter value
* @param length the number of bytes in the stream
* @exception SQLException if a database access error occurs
* @since 1.4
*/
static final BaseTypeMethod setAsciiStream_String_parameterName_InputStream_x_int_length = new AsciiStream_String_parameterName_InputStream_x_int_length();//"setAsciiStream(String parameterName, java.io.InputStream x, int length)";
/**
* Sets the designated parameter to the given
* java.math.BigDecimal
value.
* The driver converts this to an SQL NUMERIC
value when
* it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getBigDecimal
* @since 1.4
*/
static final BaseTypeMethod setBigDecimal_String_parameterName_BigDecimal_x = new BigDecimal_String_parameterName_BigDecimal_x();//"setBigDecimal(String parameterName, BigDecimal x)";
/**
* Sets the designated parameter to the given input stream, which will have
* the specified number of bytes.
* When a very large binary value is input to a LONGVARBINARY
* parameter, it may be more practical to send it via a
* java.io.InputStream
object. The data will be read from the stream
* as needed until end-of-file is reached.
*
*
Note: This stream object can either be a standard
* Java stream object or your own subclass that implements the
* standard interface.
*
* @param parameterName the name of the parameter
* @param x the java input stream which contains the binary parameter value
* @param length the number of bytes in the stream
* @since 1.4
*/
static final BaseTypeMethod setBinaryStream_String_parameterName_InputStream_x_int_length = new BinaryStream_String_parameterName_InputStream_x_int_length();//"setBinaryStream(String parameterName, java.io.InputStream x, int length)";
/**
* Sets the designated parameter to the given Java boolean
value.
* The driver converts this
* to an SQL BIT
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getBoolean
* @since 1.4
*/
static final BaseTypeMethod setBoolean_String_parameterName_boolean_x = new Boolean_String_parameterName_boolean_x();//"setBoolean(String parameterName, boolean x)";
/**
* Sets the designated parameter to the given Java byte
value.
* The driver converts this
* to an SQL TINYINT
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getByte
* @since 1.4
*/
static final BaseTypeMethod setByte_String_parameterName_byte_x = new Byte_String_parameterName_byte_x();//"setByte(String parameterName, byte x)";
/**
* Sets the designated parameter to the given Java array of bytes.
* The driver converts this to an SQL VARBINARY
or
* LONGVARBINARY
(depending on the argument's size relative
* to the driver's limits on VARBINARY
values) when it sends
* it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getBytes
* @since 1.4
*/
static final BaseTypeMethod setBytes_String_parameterName_byteArray_x = new Bytes_String_parameterName_byteArray_x();//"setBytes(String parameterName, byte x[])";
/**
* Sets the designated parameter to the given Reader
* object, which is the given number of characters long.
* When a very large UNICODE value is input to a LONGVARCHAR
* parameter, it may be more practical to send it via a
* java.io.Reader
object. The data will be read from the stream
* as needed until end-of-file is reached. The JDBC driver will
* do any necessary conversion from UNICODE to the database char format.
*
*
Note: This stream object can either be a standard
* Java stream object or your own subclass that implements the
* standard interface.
*
* @param parameterName the name of the parameter
* @param reader the java.io.Reader
object that
* contains the UNICODE data used as the designated parameter
* @param length the number of characters in the stream
* @since 1.4
*/
static final BaseTypeMethod setCharacterStream_String_parameterName_Reader_reader_int_length = new CharacterStream_String_parameterName_Reader_reader_int_length();//"setCharacterStream(String parameterName,java.io.Reader reader,int length)";
/**
* Sets the designated parameter to the given java.sql.Date
value.
* The driver converts this
* to an SQL DATE
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getDate
* @since 1.4
*/
static final BaseTypeMethod setDate_String_parameterName_Date_x = new Date_String_parameterName_Date_x();//"setDate(String parameterName, java.sql.Date x)";
/**
* Sets the designated parameter to the given java.sql.Date
value,
* using the given Calendar
object. The driver uses
* the Calendar
object to construct an SQL DATE
value,
* which the driver then sends to the database. With a
* a Calendar
object, the driver can calculate the date
* taking into account a custom timezone. If no
* Calendar
object is specified, the driver uses the default
* timezone, which is that of the virtual machine running the application.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @param cal the Calendar
object the driver will use
* to construct the date
* @exception SQLException if a database access error occurs
* @see #getDate
* @since 1.4
*/
static final BaseTypeMethod setDate_String_parameterName_Date_x_Calendar_cal = new Date_String_parameterName_Date_x_Calendar_cal();//"setDate(String parameterName, java.sql.Date x, Calendar cal)";
/**
* Sets the designated parameter to the given Java double
value.
* The driver converts this
* to an SQL DOUBLE
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getDouble
* @since 1.4
*/
static final BaseTypeMethod setDouble_String_parameterName_double_x = new Double_String_parameterName_double_x();//"setDouble(String parameterName, double x)";
/**
* Sets the designated parameter to the given Java float
value.
* The driver converts this
* to an SQL FLOAT
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getFloat
* @since 1.4
*/
static final BaseTypeMethod setFloat_String_parameterName_float_x = new Float_String_parameterName_float_x();//"setFloat(String parameterName, float x)";
/**
* Sets the designated parameter to the given Java int
value.
* The driver converts this
* to an SQL INTEGER
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getInt
* @since 1.4
*/
static final BaseTypeMethod setInt_String_parameterName_int_x = new Int_String_parameterName_int_x();//"setInt(String parameterName, int x)";
/**
* Sets the designated parameter to the given Java long
value.
* The driver converts this
* to an SQL BIGINT
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getLong
* @since 1.4
*/
static final BaseTypeMethod setLong_String_parameterName_long_x = new Long_String_parameterName_long_x();//"setLong(String parameterName, long x)";
/**
* Sets the designated parameter to SQL NULL
.
*
*
Note: You must specify the parameter's SQL type.
*
* @param parameterName the name of the parameter
* @param sqlType the SQL type code defined in java.sql.Types
* @exception SQLException if a database access error occurs
* @since 1.4
*/
static final BaseTypeMethod setNull_String_parameterName_int_sqlType = new Null_String_parameterName_int_sqlType();//"setNull(String parameterName, int sqlType)";
/**
* Sets the designated parameter to SQL NULL
.
* This version of the method setNull
should
* be used for user-defined types and REF type parameters. Examples
* of user-defined types include: STRUCT, DISTINCT, JAVA_OBJECT, and
* named array types.
*
*
Note: To be portable, applications must give the
* SQL type code and the fully-qualified SQL type name when specifying
* a NULL user-defined or REF parameter. In the case of a user-defined type
* the name is the type name of the parameter itself. For a REF
* parameter, the name is the type name of the referenced type. If
* a JDBC driver does not need the type code or type name information,
* it may ignore it.
*
* Although it is intended for user-defined and Ref parameters,
* this method may be used to set a null parameter of any JDBC type.
* If the parameter does not have a user-defined or REF type, the given
* typeName is ignored.
*
*
* @param parameterName the name of the parameter
* @param sqlType a value from java.sql.Types
* @param typeName the fully-qualified name of an SQL user-defined type;
* ignored if the parameter is not a user-defined type or
* SQL REF
value
* @exception SQLException if a database access error occurs
* @since 1.4
*/
static final BaseTypeMethod setNull_String_parameterName_int_sqlType_String_typeName = new Null_String_parameterName_int_sqlType_String_typeName();//"setNull(String parameterName, int sqlType, String typeName)";
/**
* Sets the value of the designated parameter with the given object.
* The second parameter must be of type Object
; therefore, the
* java.lang
equivalent objects should be used for built-in types.
*
*
The JDBC specification specifies a standard mapping from
* Java Object
types to SQL types. The given argument
* will be converted to the corresponding SQL type before being
* sent to the database.
*
*
Note that this method may be used to pass datatabase-
* specific abstract data types, by using a driver-specific Java
* type.
*
* If the object is of a class implementing the interface SQLData
,
* the JDBC driver should call the method SQLData.writeSQL
* to write it to the SQL data stream.
* If, on the other hand, the object is of a class implementing
* Ref
, Blob
, Clob
, Struct
,
* or Array
, the driver should pass it to the database as a
* value of the corresponding SQL type.
*
* This method throws an exception if there is an ambiguity, for example, if the
* object is of a class implementing more than one of the interfaces named above.
*
* @param parameterName the name of the parameter
* @param x the object containing the input parameter value
* @exception SQLException if a database access error occurs or if the given
* Object
parameter is ambiguous
* @see #getObject
* @since 1.4
*/
static final BaseTypeMethod setObject_String_parameterName_Object_x = new Object_String_parameterName_Object_x();//"setObject(String parameterName, Object x)";
/**
* Sets the value of the designated parameter with the given object.
* This method is like the method setObject
* above, except that it assumes a scale of zero.
*
* @param parameterName the name of the parameter
* @param x the object containing the input parameter value
* @param targetSqlType the SQL type (as defined in java.sql.Types) to be
* sent to the database
* @exception SQLException if a database access error occurs
* @see #getObject
* @since 1.4
*/
static final BaseTypeMethod setObject_String_parameterName_Object_x_int_targetSqlType = new Object_String_parameterName_Object_x_int_targetSqlType();//"setObject(String parameterName, Object x, int targetSqlType)";
/**
* Sets the value of the designated parameter with the given object. The second
* argument must be an object type; for integral values, the
* java.lang
equivalent objects should be used.
*
*
The given Java object will be converted to the given targetSqlType
* before being sent to the database.
*
* If the object has a custom mapping (is of a class implementing the
* interface SQLData
),
* the JDBC driver should call the method SQLData.writeSQL
to write it
* to the SQL data stream.
* If, on the other hand, the object is of a class implementing
* Ref
, Blob
, Clob
, Struct
,
* or Array
, the driver should pass it to the database as a
* value of the corresponding SQL type.
*
* Note that this method may be used to pass datatabase-
* specific abstract data types.
*
* @param parameterName the name of the parameter
* @param x the object containing the input parameter value
* @param targetSqlType the SQL type (as defined in java.sql.Types) to be
* sent to the database. The scale argument may further qualify this type.
* @param scale for java.sql.Types.DECIMAL or java.sql.Types.NUMERIC types,
* this is the number of digits after the decimal point. For all other
* types, this value will be ignored.
* @exception SQLException if a database access error occurs
* @see Types
* @see #getObject
* @since 1.4
*/
static final BaseTypeMethod setObject_String_parameterName_Object_x_int_targetSqlType_int_scale = new Object_String_parameterName_Object_x_int_targetSqlType_int_scale();//"setObject(String parameterName, Object x, int targetSqlType, int scale)";
/**
* Sets the designated parameter to the given Java short
value.
* The driver converts this
* to an SQL SMALLINT
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getShort
* @since 1.4
*/
static final BaseTypeMethod setShort_String_parameterName_short_x = new Short_String_parameterName_short_x();//"setShort(String parameterName, short x)";
/**
* Sets the designated parameter to the given Java String
value.
* The driver converts this
* to an SQL VARCHAR
or LONGVARCHAR
value
* (depending on the argument's
* size relative to the driver's limits on VARCHAR
values)
* when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getString
* @since 1.4
*/
static final BaseTypeMethod setString_String_parameterName_String_x = new String_String_parameterName_String_x();//"setString(String parameterName, String x)";
/**
* Sets the designated parameter to the given java.sql.Time
value.
* The driver converts this
* to an SQL TIME
value when it sends it to the database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @since 1.4
*/
static final BaseTypeMethod setTime_String_parameterName_Time_x = new Time_String_parameterName_Time_x();//"setTime(String parameterName, java.sql.Time x)";
/**
* Sets the designated parameter to the given java.sql.Time
value,
* using the given Calendar
object. The driver uses
* the Calendar
object to construct an SQL TIME
value,
* which the driver then sends to the database. With a
* a Calendar
object, the driver can calculate the time
* taking into account a custom timezone. If no
* Calendar
object is specified, the driver uses the default
* timezone, which is that of the virtual machine running the application.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @param cal the Calendar
object the driver will use
* to construct the time
* @exception SQLException if a database access error occurs
* @see #getTime
* @since 1.4
*/
static final BaseTypeMethod setTime_String_parameterName_Time_x_Calendar_cal = new Time_String_parameterName_Time_x_Calendar_cal();//"setTime(String parameterName, java.sql.Time x, Calendar cal)";
/**
* Sets the designated parameter to the given java.sql.Timestamp
value.
* The driver
* converts this to an SQL TIMESTAMP
value when it sends it to the
* database.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @exception SQLException if a database access error occurs
* @see #getTimestamp
* @since 1.4
*/
static final BaseTypeMethod setTimestamp_String_parameterName_Timestamp_x = new Timestamp_String_parameterName_Timestamp_x();//"setTimestamp(String parameterName, java.sql.Timestamp x)";
/**
* Sets the designated parameter to the given java.sql.Timestamp
value,
* using the given Calendar
object. The driver uses
* the Calendar
object to construct an SQL TIMESTAMP
value,
* which the driver then sends to the database. With a
* a Calendar
object, the driver can calculate the timestamp
* taking into account a custom timezone. If no
* Calendar
object is specified, the driver uses the default
* timezone, which is that of the virtual machine running the application.
*
* @param parameterName the name of the parameter
* @param x the parameter value
* @param cal the Calendar
object the driver will use
* to construct the timestamp
* @exception SQLException if a database access error occurs
* @see #getTimestamp
* @since 1.4
*/
static final BaseTypeMethod setTimestamp_String_parameterName_Timestamp_x_Calendar_cal = new Timestamp_String_parameterName_Timestamp_x_Calendar_cal();//"setTimestamp(String parameterName, java.sql.Timestamp x, Calendar cal)";
/**
* Sets the designated parameter to the given java.net.URL
object.
* The driver converts this to an SQL DATALINK
value when
* it sends it to the database.
*
* @param parameterName the name of the parameter
* @param val the parameter value
* @exception SQLException if a database access error occurs,
* or if a URL is malformed
* @see #getURL
* @since 1.4
*/
static final BaseTypeMethod setURL_String_parameterName_URL_val = new URL_String_parameterName_URL_val();//"setURL(String parameterName, java.net.URL val)";
String parameterName;
String typeName;
int sqlType;
int scale;
boolean isOut = false;
public String getParameterName() {
return parameterName;
}
public String getTypeName() {
return typeName;
}
public int getScale() {
return scale;
}
public String toString()
{
return new StringBuilder("method[")
.append(method)
.append("]index[")
.append(index)
.append("]value[")
.append(data)
.append("]parameterName[")
.append(parameterName)
.append("]sqlType[").append(SQLUtil.getSchemaType(null,sqlType,null))
.append("]scale[")
.append(scale)
.append("]isOut[")
.append(isOut)
.append("]")
.toString();
}
public String toString(String dbName)
{
return new StringBuilder("method[")
.append(method)
.append("]index[")
.append(index)
.append("]value[")
.append(data)
.append("]parameterName[")
.append(parameterName)
.append("]sqlType[").append(SQLUtil.getSchemaType(dbName,sqlType,null))
.append("]scale[")
.append(scale)
.append("]isOut[")
.append(isOut)
.append("]")
.toString();
}
public int getSqlType() {
return sqlType;
}
RegisterOutMethod registerOutMethod;
public void setRegisterOutMethod(RegisterOutMethod registerOutMethod) {
this.registerOutMethod = registerOutMethod;
}
public RegisterOutMethod getRegisterOutMethod() {
return registerOutMethod;
}
}