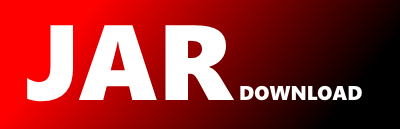
com.frameworkset.common.poolman.Record Maven / Gradle / Ivy
Show all versions of bboss-persistent Show documentation
/*
* Copyright 2008 biaoping.yin
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.frameworkset.common.poolman;
import com.frameworkset.common.poolman.handle.ValueExchange;
import com.frameworkset.common.poolman.sql.PoolManResultSetMetaData.WrapInteger;
import com.frameworkset.common.poolman.util.SQLUtil.DBHashtable;
import com.frameworkset.util.ValueObjectUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.*;
import java.math.BigDecimal;
import java.sql.*;
import java.util.Calendar;
import java.util.Map;
/**
*
*
* Title: Record.java
*
* Description: 封装每条记录的详细信息
*
* Copyright: Copyright (c) 2007
*
* @Date Oct 28, 2008 10:30:27 AM
* @author biaoping.yin
* @version 1.0
*/
public class Record extends DBHashtable{
private String[] fields;
private Map sameCols = null;
public Record(int i) {
super(i,false);
}
public Record(boolean columnLableUpperCase,int i,String[] fields,Map sameCols) {
super(i,columnLableUpperCase);
this.fields = fields;
if(sameCols != null && sameCols.size() > 0)
this.sameCols = sameCols;
}
public Record(String[] fields,Map sameCols)
{
super();
this.fields = fields;
if(sameCols != null && sameCols.size() > 0)
this.sameCols = sameCols;
}
public Record()
{
super();
}
/**
* 设置记录对应的数据库原始记录行号
*/
private int rowid;
public void setRowid(int rowid)
{
this.rowid = rowid;
}
public int getRowid()
{
return rowid;
}
public Record(int initialCapacity, float loadFactor,String[] fields,Map sameCols)
{
super(initialCapacity,loadFactor);
this.fields = fields;
if(sameCols != null && sameCols.size() > 0)
this.sameCols = sameCols;
}
public Record(int initialCapacity, float loadFactor)
{
super(initialCapacity,loadFactor);
}
private boolean isnull = true;
public Record(Map t)
{
super(t);
if(t != null)
isnull = false;
}
public Record(Map t,String[] fields)
{
super(t);
if(t != null)
isnull = false;
this.fields = fields;
}
// private Map data = new HashMap();
private static Logger log = LoggerFactory.getLogger(Record.class);
/**
* Retrieves whether the last OUT parameter read had the value of
* SQL NULL
. Note that this method should be called only after
* calling a getter method; otherwise, there is no value to use in
* determining whether it is null
or not.
*
* @return true
if the last parameter read was SQL
* NULL
; false
otherwise
* @exception SQLException if a database access error occurs
*/
public boolean wasNull() throws SQLException
{
return isnull;
}
/**
* Retrieves the value of the designated JDBC CHAR
,
* VARCHAR
, or LONGVARCHAR
parameter as a
* String
in the Java programming language.
*
* For the fixed-length type JDBC CHAR
,
* the String
object
* returned has exactly the same value the JDBC
* CHAR
value had in the
* database, including any padding added by the database.
*
* @param parameterIndex
* 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
,
* the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setString
*/
public String getString(int parameterIndex) throws SQLException
{
Object object = this.getObject(parameterIndex);
return ValueExchange.getStringFromObject(object);
}
/**
* Retrieves the value of the designated JDBC BIT
parameter as a
* boolean
in the Java programming language.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
,
* the result is false
.
* @exception SQLException if a database access error occurs
* @see #setBoolean
*/
public boolean getBoolean(int parameterIndex) throws SQLException
{
Boolean value = (Boolean)this.getObject(parameterIndex);
if(value != null)
{
return value.booleanValue();
}
throw new SQLException("getBoolean(" + parameterIndex + ") failed:value=null.");
}
/**
* Retrieves the value of the designated JDBC TINYINT
parameter
* as a byte
in the Java programming language.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setByte
*/
public byte getByte(int parameterIndex) throws SQLException
{
Byte byte_ = (Byte)this.getObject(parameterIndex);
if(byte_ != null)
return byte_.byteValue();
throw new SQLException("getByte(" + parameterIndex + ") failed:value=null.");
}
/**
* Retrieves the value of the designated JDBC SMALLINT
parameter
* as a short
in the Java programming language.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setShort
*/
public short getShort(int parameterIndex) throws SQLException
{
Object value = this.getObject(parameterIndex);
return getShortFromObject(value);
// Short byte_ = (Short)this.getObject(parameterIndex);
// if(byte_ != null)
// return byte_.byteValue();
// throw new SQLException("getShort(" + parameterIndex + ") failed:value=null.");
}
public short getShortFromObject(Object value) throws SQLException
{
if(value == null)
return 0;
else if(value instanceof Short)
{
Short s = (Short)value;
return s.shortValue();
}
if(value instanceof Integer)
{
Integer data = (Integer)value;
return data.shortValue();
}
else if(value instanceof BigDecimal)
{
BigDecimal b = (BigDecimal)value;
return b.shortValue();
}
else if(value instanceof Double)
{
Double b = (Double)value;
return b.shortValue();
}
else if(value instanceof Float)
{
Float f = (Float)value;
return f.shortValue();
}
else if(value instanceof Long)
{
Long l = (Long)value;
return l.shortValue();
}
else
{
String i = String.valueOf(value);
try
{
return Short.parseShort(i);
}
catch(Exception e)
{
throw new NestedSQLException(e);
}
}
}
public int getIntFromObject(Object value) throws SQLException
{
if(value == null)
return 0;
else if(value instanceof Integer)
{
Integer data = (Integer)value;
return data.intValue();
}
else if(value instanceof Short)
{
Short s = (Short)value;
return s.intValue();
}
else if(value instanceof BigDecimal)
{
BigDecimal b = (BigDecimal)value;
return b.intValue();
}
else if(value instanceof Double)
{
Double b = (Double)value;
return b.intValue();
}
else if(value instanceof Float)
{
Float f = (Float)value;
return f.intValue();
}
else if(value instanceof Long)
{
Long l = (Long)value;
return l.intValue();
}
else
{
String i = String.valueOf(value);
try
{
return Integer.parseInt(i);
}
catch(Exception e)
{
throw new NestedSQLException(e);
}
}
}
public float getFloatFromObject(Object value) throws SQLException
{
if(value == null)
return 0;
else if(value instanceof Float)
{
Float f = (Float)value;
return f.floatValue();
}
if(value instanceof Integer)
{
Integer data = (Integer)value;
return data.floatValue();
}
else if(value instanceof BigDecimal)
{
BigDecimal b = (BigDecimal)value;
return b.floatValue();
}
else if(value instanceof Short)
{
Short s = (Short)value;
return s.floatValue();
}
else if(value instanceof Long)
{
Long l = (Long)value;
return l.floatValue();
}
else if(value instanceof Double)
{
Double l = (Double)value;
return l.floatValue();
}
else
{
String i = String.valueOf(value);
try
{
return Float.parseFloat(i);
}
catch(Exception e)
{
throw new NestedSQLException(e);
}
}
}
public double getDoubleFromObject(Object value) throws SQLException
{
if(value == null)
return 0;
else if(value instanceof Double)
{
Double l = (Double)value;
return l.doubleValue();
}
else if(value instanceof Float)
{
Float f = (Float)value;
return f.doubleValue();
}
if(value instanceof Integer)
{
Integer data = (Integer)value;
return data.doubleValue();
}
else if(value instanceof BigDecimal)
{
BigDecimal b = (BigDecimal)value;
return b.doubleValue();
}
else if(value instanceof Short)
{
Short s = (Short)value;
return s.doubleValue();
}
else if(value instanceof Long)
{
Long l = (Long)value;
return l.doubleValue();
}
else
{
String i = String.valueOf(value);
try
{
return Double.parseDouble(i);
}
catch(Exception e)
{
throw new NestedSQLException(e);
}
}
}
/**
* Retrieves the value of the designated JDBC INTEGER
parameter
* as an int
in the Java programming language.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setInt
*/
public int getInt(int parameterIndex) throws SQLException
{
// if(Integer )
Object value = this.getObject(parameterIndex);
return getIntFromObject(value);
// throw new SQLException("getInt(" + parameterIndex + ") failed:value=null.");
}
/**
* Retrieves the value of the designated JDBC BIGINT
parameter
* as a long
in the Java programming language.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setLong
*/
public long getLong(int parameterIndex) throws SQLException
{
Object value = this.getObject(parameterIndex);
return getLongFromObject(value);
// Long data = (Long)this.getObject(parameterIndex);
// if(data != null)
// {
// return data.longValue();
// }
// throw new SQLException("getInt(" + parameterIndex + ") failed:value=null.");
}
public long getLongFromObject(Object value) throws SQLException
{
if(value == null)
return 0;
if(value instanceof Long)
{
Long l = (Long)value;
return l.longValue();
}
else if(value instanceof Double)
{
Double l = (Double)value;
return l.longValue();
}
else if(value instanceof Integer)
{
Integer data = (Integer)value;
return data.longValue();
}
else if(value instanceof BigDecimal)
{
BigDecimal b = (BigDecimal)value;
return b.longValue();
}
else if(value instanceof Short)
{
Short s = (Short)value;
return s.longValue();
}
else if(value instanceof Float)
{
Float f = (Float)value;
return f.longValue();
}
else
{
String i = String.valueOf(value);
try
{
return Long.parseLong(i);
}
catch(Exception e)
{
throw new NestedSQLException(e);
}
}
}
/**
* Retrieves the value of the designated JDBC FLOAT
parameter
* as a float
in the Java programming language.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setFloat
*/
public float getFloat(int parameterIndex) throws SQLException
{
Object value = this.getObject(parameterIndex);
return getFloatFromObject( value);
}
/**
* Retrieves the value of the designated JDBC DOUBLE
parameter as a double
* in the Java programming language.
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setDouble
*/
public double getDouble(int parameterIndex) throws SQLException
{
Object value = this.getObject(parameterIndex);
return getDoubleFromObject(value);
// if(data != null)
// {
// return data.doubleValue();
// }
// throw new SQLException("getDouble(" + parameterIndex + ") failed:value=null.");
}
/**
* Retrieves the value of the designated JDBC NUMERIC
parameter as a
* java.math.BigDecimal
object with scale digits to
* the right of the decimal point.
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @param scale the number of digits to the right of the decimal point
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @deprecated use getBigDecimal(int parameterIndex)
* or getBigDecimal(String parameterName)
* @see #setBigDecimal
*/
public BigDecimal getBigDecimal(int parameterIndex, int scale)
throws SQLException
{
BigDecimal big = this.getBigDecimal(parameterIndex);
if(big != null)
return big.setScale(scale);
return null;
}
/**
* Retrieves the value of the designated JDBC BINARY
or
* VARBINARY
parameter as an array of byte
* values in the Java programming language.
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setBytes
*/
public byte[] getBytes(int parameterIndex) throws SQLException
{
Object bytes = this.getObject(parameterIndex);
if(bytes instanceof byte[])
return (byte[])bytes;
else
{
return ValueExchange.convertObjectToBytes(bytes);
}
// return (byte[]) this.getObject(parameterIndex);
}
/**
* Retrieves the value of the designated JDBC DATE
parameter as a
* java.sql.Date
object.
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setDate
*/
public java.sql.Date getDate(int parameterIndex) throws SQLException
{
Object date = this.getObject(parameterIndex);
if(date == null)
return null;
if(date instanceof java.sql.Date)
{
return (java.sql.Date)date;
}
else if(date instanceof java.util.Date)
{
return new java.sql.Date(((java.util.Date)date).getTime());
}
throw new SQLException("Record.getDate(" + parameterIndex +") failed: error data type["+date.getClass().getName()+","+ date +"]");
// return (java.sql.Date)this.getObject(parameterIndex);
}
/**
* Retrieves the value of the designated JDBC TIME
parameter as a
* java.sql.Time
object.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setTime
*/
public java.sql.Time getTime(int parameterIndex) throws SQLException
{
Object object = this.getObject(parameterIndex);
if(object == null)
return null;
if(object instanceof java.sql.Time)
return (java.sql.Time)object;
if(object instanceof java.util.Date)
{
return new java.sql.Time(((java.util.Date)object).getTime());
}
// return (java.sql.Time)this.getObject(parameterIndex);
throw new SQLException("Record.getTime(" + parameterIndex +") failed: error data type["+object.getClass().getName()+","+ object +"]");
}
/**
* Retrieves the value of the designated JDBC TIMESTAMP
parameter as a
* java.sql.Timestamp
object.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setTimestamp
*/
public java.sql.Timestamp getTimestamp(int parameterIndex)
throws SQLException
{
// return (java.sql.Timestamp)this.getObject(parameterIndex);
Object object = this.getObject(parameterIndex);
if(object == null)
return null;
if(object instanceof java.sql.Timestamp)
return (java.sql.Timestamp)object;
if(object instanceof java.util.Date)
{
return new java.sql.Timestamp(((java.util.Date)object).getTime());
}
// return (java.sql.Time)this.getObject(parameterIndex);
throw new SQLException("Record.getTimestamp(" + parameterIndex +") failed: error data type["+object.getClass().getName()+","+ object +"]");
}
//----------------------------------------------------------------------
// Advanced features:
/**
*
* @param column
* start from zero
* @return 列索引对应的属性名称
* @throws SQLException
*/
private String seekField(int column) throws SQLException {
// return f_temps[column];
if(column > fields.length || column < 0)
throw new SQLException(new StringBuilder("column out of range:column=").append(column)
.append(",fields.length=").append(fields.length ).toString());
if(this.sameCols == null || column == 0)
return this.fields[column];
String temp = fields[column];
WrapInteger wi = (WrapInteger)sameCols.get(temp );
if(wi == null)
return temp ;
else
return wi.getColumnName(column);
}
/**
* Retrieves the value of the designated parameter as an Object
* in the Java programming language. If the value is an SQL NULL
,
* the driver returns a Java null
.
*
* This method returns a Java object whose type corresponds to the JDBC
* type that was registered for this parameter using the method
* registerOutParameter
. By registering the target JDBC
* type as java.sql.Types.OTHER
, this method can be used
* to read database-specific abstract data types.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return A java.lang.Object
holding the OUT parameter value
* @exception SQLException if a database access error occurs
* @see Types
* @see #setObject
*/
public Object getObject(int parameterIndex) throws SQLException
{
if(this.fields != null)
{
return get(seekField(parameterIndex));
// return get(new Integer(parameterIndex));
}
else
{
return get(new Integer(parameterIndex));
}
}
//--------------------------JDBC 2.0-----------------------------
/**
* Retrieves the value of the designated JDBC NUMERIC
parameter as a
* java.math.BigDecimal
object with as many digits to the
* right of the decimal point as the value contains.
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value in full precision. If the value is
* SQL NULL
, the result is null
.
* @exception SQLException if a database access error occurs
* @see #setBigDecimal
* @since 1.2
*/
public BigDecimal getBigDecimal(int parameterIndex) throws SQLException
{
return (BigDecimal)getObject(parameterIndex);
}
/**
* Returns an object representing the value of OUT parameter
* i
and uses map
for the custom
* mapping of the parameter value.
*
* This method returns a Java object whose type corresponds to the
* JDBC type that was registered for this parameter using the method
* registerOutParameter
. By registering the target
* JDBC type as java.sql.Types.OTHER
, this method can
* be used to read database-specific abstract data types.
* @param i 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1, and so on
* @param map the mapping from SQL type names to Java classes
* @return a java.lang.Object
holding the OUT parameter value
* @exception SQLException if a database access error occurs
* @see #setObject
* @since 1.2
*/
public Object getObject (int i, java.util.Map map) throws SQLException
{
return this.getObject(i);
}
public java.io.Reader getCharacterStream( int columnIndex) throws SQLException
{
Object object = this.getObject(columnIndex);
if(object == null)
return null;
if(object instanceof java.io.Reader)
return (java.io.Reader)object;
else if(object instanceof java.sql.Clob)
{
Clob clob = (java.sql.Clob)object;
return clob.getCharacterStream();
}
// else if(object instanceof java.sql.Blob)
// {
// Blob clob = (java.sql.Blob)object;
// return clob.getCharacterStream();
// }
throw new SQLException("Error type cast column index["+columnIndex+"]:From [" + object.getClass().getName() + "] to [" + java.io.Reader.class.getName() + "]");
}
/**
* Retrieves the value of the designated column in the current row of this
* ResultSet
object as a stream of ASCII characters. The
* value can then be read in chunks from the stream. This method is
* particularly suitable for retrieving large LONGVARCHAR
* values. The JDBC driver will do any necessary conversion from the
* database format into ASCII.
*
*
* Note: All the data in the returned stream must be read prior to
* getting the value of any other column. The next call to a getter method
* implicitly closes the stream. Also, a stream may return 0
* when the method InputStream.available
is called whether
* there is data available or not.
*
* @param rowid
* the first row is 0, the second is 1
* @param columnIndex
* the first column is 0, the second is 1, ...
* @return a Java input stream that delivers the database column value as a
* stream of one-byte ASCII characters; if the value is SQL
* NULL
, the value returned is null
* @exception SQLException
* if a database access error occurs
*/
public java.io.InputStream getAsciiStream(int columnIndex)
throws SQLException {
// inrange(rowid, colName);
Object value = this.getObject(columnIndex);
if(value == null)
{
return null;
}
if(value instanceof java.io.InputStream)
return (java.io.InputStream)value;
if(value instanceof Clob)
{
Clob clob = (Clob)value;
return clob.getAsciiStream();
// java.io.InputStream
}
else if(value instanceof Blob)
{
Blob clob = (Blob)value;
return clob.getBinaryStream();
// java.io.InputStream
}
throw new SQLException("Error type cast column index["+columnIndex+"]:From [" + value.getClass().getName() + "] to [" + java.io.InputStream.class.getName() + "]");
// return allResults[getTrueRowid(rowid)].getBoolean(colName);
// return (java.io.InputStream) getObject(rowid, columnIndex);
}
public java.io.InputStream getAsciiStream(String colName)
throws SQLException {
//inrange(rowid, colName);
Object value = this.getObject(colName);
if(value == null)
{
return null;
}
if(value instanceof java.io.InputStream)
return (java.io.InputStream)value;
if(value instanceof Clob)
{
Clob clob = (Clob)value;
return clob.getAsciiStream();
// java.io.InputStream
}
else if(value instanceof Blob)
{
Blob clob = (Blob)value;
return clob.getBinaryStream();
// java.io.InputStream
}
throw new SQLException("Error type cast column["+colName+"]:From [" + value.getClass().getName() + "] to [" + java.io.InputStream.class.getName() + "]");
//return allResults[getTrueRowid(rowid)].getBoolean(colName);
//return (java.io.InputStream) getObject(rowid, columnIndex);
}
public java.io.Reader getCharacterStream(String columnName) throws SQLException
{
Object object = this.getObject(columnName);
if(object == null)
return null;
if(object instanceof java.io.Reader)
return (java.io.Reader)object;
else if(object instanceof java.sql.Clob)
{
Clob clob = (java.sql.Clob)object;
return clob.getCharacterStream();
}
// else if(object instanceof java.sql.Blob)
// {
// Blob clob = (java.sql.Blob)object;
// return clob.getCharacterStream();
// }
throw new SQLException("Error type cast column["+columnName+"]:From [" + object.getClass().getName() + "] to [" + java.io.Reader.class.getName() + "]");
}
/**
* Retrieves the value of the designated column in the current row of this
* ResultSet
object as as a stream of two-byte Unicode
* characters. The first byte is the high byte; the second byte is the low
* byte.
*
* The value can then be read in chunks from the stream. This method is
* particularly suitable for retrieving large LONGVARCHAR
values.
* The JDBC driver will do any necessary conversion from the database format
* into Unicode.
*
*
* Note: All the data in the returned stream must be read prior to
* getting the value of any other column. The next call to a getter method
* implicitly closes the stream. Also, a stream may return 0
* when the method InputStream.available
is called, whether
* there is data available or not.
*
* @param rowid
* the first row is 0, the second is 1
* @param columnIndex
* the first column is 0, the second is 1, ...
* @return a Java input stream that delivers the database column value as a
* stream of two-byte Unicode characters; if the value is SQL
* NULL
, the value returned is null
*
* @exception SQLException
* if a database access error occurs
* @deprecated use getCharacterStream
in place of
* getUnicodeStream
*/
public java.io.InputStream getUnicodeStream( int columnIndex)
throws SQLException {
Object value = this.getObject( columnIndex);
if(value == null)
return null;
if(value instanceof Blob)
{
Blob blob = (Blob)value;
return blob.getBinaryStream();
}
else if(value instanceof Clob)
{
Clob blob = (Clob)value;
return blob.getAsciiStream();
}
throw new SQLException("Error type cast column index["+columnIndex+"]:From [" + value.getClass().getName() + "] to [" + java.io.InputStream.class.getName() + "]");
// return (java.io.InputStream) this.getObject(rowid, columnIndex);
}
/**
* Retrieves the value of the designated column in the current row of this
* ResultSet
object as a binary stream of uninterpreted
* bytes. The value can then be read in chunks from the stream. This method
* is particularly suitable for retrieving large LONGVARBINARY
* values.
*
*
* Note: All the data in the returned stream must be read prior to
* getting the value of any other column. The next call to a getter method
* implicitly closes the stream. Also, a stream may return 0
* when the method InputStream.available
is called whether
* there is data available or not.
*
* @param rowid
* the first row is 0, the second is 1
* @param columnIndex
* the first column is 0, the second is 1, ...
* @return a Java input stream that delivers the database column value as a
* stream of uninterpreted bytes; if the value is SQL
* NULL
, the value returned is null
* @exception SQLException
* if a database access error occurs
*/
public java.io.InputStream getBinaryStream(int columnIndex)
throws SQLException {
Object value = this.getObject( columnIndex);
if(value == null)
return null;
if(value instanceof Blob)
{
Blob blob = (Blob)value;
return blob.getBinaryStream();
}
else if(value instanceof Clob)
{
Clob blob = (Clob)value;
return blob.getAsciiStream();
}
throw new SQLException("Error type cast column index["+columnIndex+"]:From [" + value.getClass().getName() + "] to [" + java.io.InputStream.class.getName() + "]");
// return (java.io.InputStream) this.getObject(rowid, columnIndex);
}
public java.io.InputStream getBinaryStream(String columnName)
throws SQLException {
Object value = this.getObject( columnName);
if(value == null)
return null;
if(value instanceof Blob)
{
Blob blob = (Blob)value;
return blob.getBinaryStream();
}
else if(value instanceof Clob)
{
Clob blob = (Clob)value;
return blob.getAsciiStream();
}
throw new SQLException("Error type cast column["+columnName+"]:From [" + value.getClass().getName() + "] to [" + java.io.InputStream.class.getName() + "]");
//return (java.io.InputStream) this.getObject(rowid, columnIndex);
}
/**
* Retrieves the value of the designated column in the current row of this
* ResultSet
object as as a stream of two-byte Unicode
* characters. The first byte is the high byte; the second byte is the low
* byte.
*
* The value can then be read in chunks from the stream. This method is
* particularly suitable for retrieving large LONGVARCHAR
values.
* The JDBC driver will do any necessary conversion from the database format
* into Unicode.
*
*
* Note: All the data in the returned stream must be read prior to
* getting the value of any other column. The next call to a getter method
* implicitly closes the stream. Also, a stream may return 0
* when the method InputStream.available
is called, whether
* there is data available or not.
*
* @param rowid
* the first row is 0, the second is 1
* @param columnIndex
* the first column is 0, the second is 1, ...
* @return a Java input stream that delivers the database column value as a
* stream of two-byte Unicode characters; if the value is SQL
* NULL
, the value returned is null
*
* @exception SQLException
* if a database access error occurs
* @deprecated use getCharacterStream
in place of
* getUnicodeStream
*/
public java.io.InputStream getUnicodeStream( String columnName)
throws SQLException {
Object value = this.getObject( columnName);
if(value == null)
return null;
if(value instanceof Blob)
{
Blob blob = (Blob)value;
return blob.getBinaryStream();
}
else if(value instanceof Clob)
{
Clob blob = (Clob)value;
return blob.getAsciiStream();
}
throw new SQLException("Error type cast column["+columnName+"]:From [" + value.getClass().getName() + "] to [" + java.io.InputStream.class.getName() + "]");
// return (java.io.InputStream) this.getObject(rowid, columnIndex);
}
/**
* Retrieves the value of the designated JDBC REF(<structured-type>)
* parameter as a {@link Ref} object in the Java programming language.
* @param i 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @return the parameter value as a Ref
object in the
* Java programming language. If the value was SQL NULL
, the value
* null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.2
*/
public Ref getRef (int i) throws SQLException
{
return (Ref)this.getObject(i);
}
/**
* Retrieves the value of the designated JDBC BLOB
parameter as a
* {@link Blob} object in the Java programming language.
* @param i 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1, and so on
* @return the parameter value as a Blob
object in the
* Java programming language. If the value was SQL NULL
, the value
* null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.2
*/
public Blob getBlob (int i) throws SQLException
{
return (Blob)this.getObject(i);
}
/**
* Retrieves the value of the designated JDBC CLOB
parameter as a
* Clob
object in the Java programming language.
* @param i 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1, and
* so on
* @return the parameter value as a Clob
object in the
* Java programming language. If the value was SQL NULL
, the
* value null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.2
*/
public Clob getClob (int i) throws SQLException
{
return (Clob)this.getObject(i);
}
/**
*
* Retrieves the value of the designated JDBC ARRAY
parameter as an
* {@link Array} object in the Java programming language.
* @param i 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1, and
* so on
* @return the parameter value as an Array
object in
* the Java programming language. If the value was SQL NULL
, the
* value null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.2
*/
public Array getArray (int i) throws SQLException
{
return (Array)this.getObject(i);
}
/**
* Retrieves the value of the designated JDBC DATE
parameter as a
* java.sql.Date
object, using
* the given Calendar
object
* to construct the date.
* With a Calendar
object, the driver
* can calculate the date taking into account a custom timezone and locale.
* If no Calendar
object is specified, the driver uses the
* default timezone and locale.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @param cal the Calendar
object the driver will use
* to construct the date
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setDate
* @since 1.2
*/
public java.sql.Date getDate(int parameterIndex, Calendar cal)
throws SQLException
{
java.sql.Date date = this.getDate(parameterIndex);
if(date != null)
{
cal.setTime(date);
return new java.sql.Date(cal.getTimeInMillis());
// java.util.Date date_ = cal.getTime();
// return new java.sql.Date(date,cal);
}
return null;
}
/**
* Retrieves the value of the designated JDBC TIME
parameter as a
* java.sql.Time
object, using
* the given Calendar
object
* to construct the time.
* With a Calendar
object, the driver
* can calculate the time taking into account a custom timezone and locale.
* If no Calendar
object is specified, the driver uses the
* default timezone and locale.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @param cal the Calendar
object the driver will use
* to construct the time
* @return the parameter value; if the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setTime
* @since 1.2
*/
public java.sql.Time getTime(int parameterIndex, Calendar cal)
throws SQLException
{
java.sql.Time time = this.getTime(parameterIndex);
if(time != null)
{
cal.setTimeInMillis(time.getTime());
return new java.sql.Time(cal.getTimeInMillis());
}
return null;
}
/**
* Retrieves the value of the designated JDBC TIMESTAMP
parameter as a
* java.sql.Timestamp
object, using
* the given Calendar
object to construct
* the Timestamp
object.
* With a Calendar
object, the driver
* can calculate the timestamp taking into account a custom timezone and locale.
* If no Calendar
object is specified, the driver uses the
* default timezone and locale.
*
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,
* and so on
* @param cal the Calendar
object the driver will use
* to construct the timestamp
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setTimestamp
* @since 1.2
*/
public java.sql.Timestamp getTimestamp(int parameterIndex, Calendar cal)
throws SQLException
{
java.sql.Timestamp date = this.getTimestamp(parameterIndex);
if(date != null)
{
cal.setTimeInMillis(date.getTime());
return new java.sql.Timestamp(cal.getTimeInMillis());
}
return null;
}
/**
* Retrieves the value of the designated JDBC DATALINK
parameter as a
* java.net.URL
object.
*
* @param parameterIndex 存储过程返回的数据:the first parameter is 1, the second is 2 一般查询的结果集: the first parameter is 0, the second is 1,...
* @return a java.net.URL
object that represents the
* JDBC DATALINK
value used as the designated
* parameter
* @exception SQLException if a database access error occurs,
* or if the URL being returned is
* not a valid URL on the Java platform
* @see #setURL
* @since 1.4
*/
public java.net.URL getURL(int parameterIndex) throws SQLException
{
return (java.net.URL)this.getObject(parameterIndex);
}
/**
* Retrieves the value of a JDBC CHAR
, VARCHAR
,
* or LONGVARCHAR
parameter as a String
in
* the Java programming language.
*
* For the fixed-length type JDBC CHAR
,
* the String
object
* returned has exactly the same value the JDBC
* CHAR
value had in the
* database, including any padding added by the database.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setString
* @since 1.4
*/
public String getString(String parameterName) throws SQLException
{
Object object = this.getObject(parameterName);
return ValueExchange.getStringFromObject(object);
}
/**
* Retrieves the value of a JDBC BIT
parameter as a
* boolean
in the Java programming language.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result
* is false
.
* @exception SQLException if a database access error occurs
* @see #setBoolean
* @since 1.4
*/
public boolean getBoolean(String parameterName) throws SQLException
{
Boolean value = (Boolean)this.getObject(parameterName);
if(value != null)
{
return value.booleanValue();
}
throw new SQLException("getBoolean(" + parameterName + ") failed:value=null.");
}
/**
* Retrieves the value of a JDBC TINYINT
parameter as a byte
* in the Java programming language.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setByte
* @since 1.4
*/
public byte getByte(String parameterName) throws SQLException
{
Byte byte_ = (Byte)this.getObject(parameterName);
if(byte_ != null)
return byte_.byteValue();
throw new SQLException("getByte(" + parameterName + ") failed:value=null.");
}
/**
* Retrieves the value of a JDBC SMALLINT
parameter as a short
* in the Java programming language.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result
* is 0
.
* @exception SQLException if a database access error occurs
* @see #setShort
* @since 1.4
*/
public short getShort(String parameterName) throws SQLException
{
Object value = this.getObject(parameterName);
return getShortFromObject(value);
}
/**
* Retrieves the value of a JDBC INTEGER
parameter as an int
* in the Java programming language.
*
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
,
* the result is 0
.
* @exception SQLException if a database access error occurs
* @see #setInt
* @since 1.4
*/
public int getInt(String parameterName) throws SQLException
{
// Integer data = (Integer)this.getObject(parameterName);
// if(data != null)
// {
// return data.intValue();
// }
// throw new SQLException("getInt(" + parameterName + ") failed:value=null.");
Object value = this.getObject(parameterName);
return getIntFromObject(value);
}
/**
* Retrieves the value of a JDBC BIGINT
parameter as a long
* in the Java programming language.
*
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
,
* the result is 0
.
* @exception SQLException if a database access error occurs
* @see #setLong
* @since 1.4
*/
public long getLong(String parameterName) throws SQLException
{
// Long data = (Long)this.getObject(parameterName);
// if(data != null)
// {
// return data.longValue();
// }
// throw new SQLException("getInt(" + parameterName + ") failed:value=null.");
Object value = this.getObject(parameterName);
return getLongFromObject(value);
}
/**
* Retrieves the value of a JDBC FLOAT
parameter as a float
* in the Java programming language.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
,
* the result is 0
.
* @exception SQLException if a database access error occurs
* @see #setFloat
* @since 1.4
*/
public float getFloat(String parameterName) throws SQLException
{
Object value = this.getObject(parameterName);
return getFloatFromObject( value);
}
/**
* Retrieves the value of a JDBC DOUBLE
parameter as a double
* in the Java programming language.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
,
* the result is 0
.
* @exception SQLException if a database access error occurs
* @see #setDouble
* @since 1.4
*/
public double getDouble(String parameterName) throws SQLException
{
Object value = this.getObject(parameterName);
return getDoubleFromObject(value);
}
/**
* Retrieves the value of a JDBC BINARY
or VARBINARY
* parameter as an array of byte
values in the Java
* programming language.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result is
* null
.
* @exception SQLException if a database access error occurs
* @see #setBytes
* @since 1.4
*/
public byte[] getBytes(String parameterName) throws SQLException
{
Object bytes = this.getObject(parameterName);
if(bytes instanceof byte[])
return (byte[])bytes;
else
{
return ValueExchange.convertObjectToBytes(bytes);
}
}
/**
* Retrieves the value of a JDBC DATE
parameter as a
* java.sql.Date
object.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setDate
* @since 1.4
*/
public java.sql.Date getDate(String parameterName) throws SQLException
{
// return (java.sql.Date)this.getObject(parameterName);
Object date = this.getObject(parameterName);
if(date == null)
return null;
if(date instanceof java.sql.Date)
{
return (java.sql.Date)date;
}
else if(date instanceof java.util.Date)
{
return new java.sql.Date(((java.util.Date)date).getTime());
}
throw new SQLException("Record.getDate(" + parameterName +") failed: error data type["+date.getClass().getName()+","+ date +"]");
}
/**
* Retrieves the value of a JDBC TIME
parameter as a
* java.sql.Time
object.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setTime
* @since 1.4
*/
public java.sql.Time getTime(String parameterName) throws SQLException
{
// return (java.sql.Time)this.getObject(parameterName);
Object object = this.getObject(parameterName);
if(object == null)
return null;
if(object instanceof java.sql.Time)
return (java.sql.Time)object;
if(object instanceof java.util.Date)
{
return new java.sql.Time(((java.util.Date)object).getTime());
}
// return (java.sql.Time)this.getObject(parameterIndex);
throw new SQLException("Record.getTime(" + parameterName +") failed: error data type["+object.getClass().getName()+","+ object +"]");
}
/**
* Retrieves the value of a JDBC TIMESTAMP
parameter as a
* java.sql.Timestamp
object.
* @param parameterName the name of the parameter
* @return the parameter value. If the value is SQL NULL
, the result
* is null
.
* @exception SQLException if a database access error occurs
* @see #setTimestamp
* @since 1.4
*/
public java.sql.Timestamp getTimestamp(String parameterName) throws SQLException
{
// return (java.sql.Timestamp)this.getObject(parameterName);
Object object = this.getObject(parameterName);
if(object == null)
return null;
if(object instanceof java.sql.Timestamp)
return (java.sql.Timestamp)object;
if(object instanceof java.util.Date)
{
return new java.sql.Timestamp(((java.util.Date)object).getTime());
}
// return (java.sql.Time)this.getObject(parameterIndex);
throw new SQLException("Record.getTimestamp(" + parameterName +") failed: error data type["+object.getClass().getName()+","+ object +"]");
}
/**
* Retrieves the value of a parameter as an Object
in the Java
* programming language. If the value is an SQL NULL
, the
* driver returns a Java null
.
*
* This method returns a Java object whose type corresponds to the JDBC
* type that was registered for this parameter using the method
* registerOutParameter
. By registering the target JDBC
* type as java.sql.Types.OTHER
, this method can be used
* to read database-specific abstract data types.
* @param parameterName the name of the parameter
* @return A java.lang.Object
holding the OUT parameter value.
* @exception SQLException if a database access error occurs
* @see Types
* @since 1.4
*/
public Object getObject(String parameterName) throws SQLException
{
if(columnLableUpperCase) {
return get(parameterName.toUpperCase());
}
else
{
return get(parameterName);
}
}
/**
* Retrieves the value of a JDBC NUMERIC
parameter as a
* java.math.BigDecimal
object with as many digits to the
* right of the decimal point as the value contains.
* @param parameterName the name of the parameter
* @return the parameter value in full precision. If the value is
* SQL NULL
, the result is null
.
* @exception SQLException if a database access error occurs
* @since 1.4
*/
public BigDecimal getBigDecimal(String parameterName) throws SQLException
{
return (BigDecimal)this.getObject(parameterName);
}
/**
* Returns an object representing the value of OUT parameter
* i
and uses map
for the custom
* mapping of the parameter value.
*
* This method returns a Java object whose type corresponds to the
* JDBC type that was registered for this parameter using the method
* registerOutParameter
. By registering the target
* JDBC type as java.sql.Types.OTHER
, this method can
* be used to read database-specific abstract data types.
* @param parameterName the name of the parameter
* @param map the mapping from SQL type names to Java classes
* @return a java.lang.Object
holding the OUT parameter value
* @exception SQLException if a database access error occurs
* @see #setObject
* @since 1.4
*/
public Object getObject (String parameterName, java.util.Map map) throws SQLException
{
// if(data != null)
// {
// return data.get(parameterName.toLowerCase());
//
// }
// return null;
return get(parameterName.toLowerCase());
}
/**
* Retrieves the value of a JDBC REF(<structured-type>)
* parameter as a {@link Ref} object in the Java programming language.
*
* @param parameterName the name of the parameter
* @return the parameter value as a Ref
object in the
* Java programming language. If the value was SQL NULL
,
* the value null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.4
*/
public Ref getRef (String parameterName) throws SQLException
{
return (Ref)this.getObject(parameterName);
}
/**
* Retrieves the value of a JDBC BLOB
parameter as a
* {@link Blob} object in the Java programming language.
*
* @param parameterName the name of the parameter
* @return the parameter value as a Blob
object in the
* Java programming language. If the value was SQL NULL
,
* the value null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.4
*/
public Blob getBlob (String parameterName) throws SQLException
{
return (Blob)this.getObject(parameterName);
}
/**
* Retrieves the value of a JDBC CLOB
parameter as a
* Clob
object in the Java programming language.
* @param parameterName the name of the parameter
* @return the parameter value as a Clob
object in the
* Java programming language. If the value was SQL NULL
,
* the value null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.4
*/
public Clob getClob (String parameterName) throws SQLException
{
return (Clob)this.getObject(parameterName);
}
/**
* Retrieves the value of a JDBC ARRAY
parameter as an
* {@link Array} object in the Java programming language.
*
* @param parameterName the name of the parameter
* @return the parameter value as an Array
object in
* Java programming language. If the value was SQL NULL
,
* the value null
is returned.
* @exception SQLException if a database access error occurs
* @since 1.4
*/
public Array getArray (String parameterName) throws SQLException
{
return (Array)this.getObject(parameterName);
}
/**
* Retrieves the value of a JDBC DATE
parameter as a
* java.sql.Date
object, using
* the given Calendar
object
* to construct the date.
* With a Calendar
object, the driver
* can calculate the date taking into account a custom timezone and locale.
* If no Calendar
object is specified, the driver uses the
* default timezone and locale.
*
* @param parameterName the name of the parameter
* @param cal the Calendar
object the driver will use
* to construct the date
* @return the parameter value. If the value is SQL NULL
,
* the result is null
.
* @exception SQLException if a database access error occurs
* @see #setDate
* @since 1.4
*/
public java.sql.Date getDate(String parameterName, Calendar cal)
throws SQLException
{
java.sql.Date date = this.getDate(parameterName);
if(date != null)
{
cal.setTime(date);
return new java.sql.Date(cal.getTimeInMillis());
// java.util.Date date_ = cal.getTime();
// return new java.sql.Date(date,cal);
}
return null;
}
/**
* Retrieves the value of a JDBC TIME
parameter as a
* java.sql.Time
object, using
* the given Calendar
object
* to construct the time.
* With a Calendar
object, the driver
* can calculate the time taking into account a custom timezone and locale.
* If no Calendar
object is specified, the driver uses the
* default timezone and locale.
*
* @param parameterName the name of the parameter
* @param cal the Calendar
object the driver will use
* to construct the time
* @return the parameter value; if the value is SQL NULL
, the result is
* null
.
* @exception SQLException if a database access error occurs
* @see #setTime
* @since 1.4
*/
public java.sql.Time getTime(String parameterName, Calendar cal)
throws SQLException
{
java.sql.Time time = this.getTime(parameterName);
if(time != null)
{
cal.setTimeInMillis(time.getTime());
return new java.sql.Time(cal.getTimeInMillis());
}
return null;
}
/**
* Retrieves the value of a JDBC TIMESTAMP
parameter as a
* java.sql.Timestamp
object, using
* the given Calendar
object to construct
* the Timestamp
object.
* With a Calendar
object, the driver
* can calculate the timestamp taking into account a custom timezone and locale.
* If no Calendar
object is specified, the driver uses the
* default timezone and locale.
*
*
* @param parameterName the name of the parameter
* @param cal the Calendar
object the driver will use
* to construct the timestamp
* @return the parameter value. If the value is SQL NULL
, the result is
* null
.
* @exception SQLException if a database access error occurs
* @see #setTimestamp
* @since 1.4
*/
public java.sql.Timestamp getTimestamp(String parameterName, Calendar cal)
throws SQLException
{
java.sql.Timestamp date = this.getTimestamp(parameterName);
if(date != null)
{
cal.setTimeInMillis(date.getTime());
return new java.sql.Timestamp(cal.getTimeInMillis());
}
return null;
}
/**
* Retrieves the value of a JDBC DATALINK
parameter as a
* java.net.URL
object.
*
* @param parameterName the name of the parameter
* @return the parameter value as a java.net.URL
object in the
* Java programming language. If the value was SQL NULL
, the
* value null
is returned.
* @exception SQLException if a database access error occurs,
* or if there is a problem with the URL
* @see #setURL
* @since 1.4
*/
public java.net.URL getURL(String parameterName) throws SQLException
{
return (java.net.URL)this.getObject(parameterName);
}
public void getFile(int index,File file) throws SQLException, IOException
{
Object value = this.getObject(index);
this.getFile(value, file);
}
public void getFile(Object value,File file) throws SQLException
{
if (value == null)
{
return;
}
else
{
if (value instanceof Blob) {
ValueObjectUtil.getFileFromBlob((Blob)value, file);
}
else if(value instanceof Clob)
{
ValueObjectUtil.getFileFromClob((Clob)value, file);
}
else if (value instanceof byte[])
{
ValueObjectUtil.getFileFromBytes((byte[])value, file);
}
else if (value instanceof String)
{
ValueObjectUtil.getFileFromString((String)value, file);
}
else
{
ValueObjectUtil.getFileFromString(value.toString(), file);
}
}
}
public void getFile(String parameterName,File file) throws SQLException, IOException
{
Object value = this.getObject(parameterName);
this.getFile(value, file);
}
/*
* 获取序列化对象
*/
public Serializable getSerializable(String field) throws SQLException
{
Blob blog = this.getBlob(field);
if(blog == null)
return null;
Serializable object = null;
InputStream in = null;
java.io.ObjectInputStream objectin = null;
try
{
in = blog.getBinaryStream();
objectin = new ObjectInputStream(in);
object = (Serializable)objectin.readObject();
}
catch(SQLException e)
{
throw e;
}
catch(Exception e)
{
throw new NestedSQLException(e);
}
finally
{
if(in != null)
try
{
in.close();
}
catch (IOException e)
{
// // TODO Auto-generated catch block
// e.printStackTrace();
}
if(objectin != null)
try
{
objectin.close();
}
catch (IOException e)
{
// // TODO Auto-generated catch block
// e.printStackTrace();
}
}
return object;
}
public Serializable getSerializable(int parameterIndex) throws SQLException
{
Blob blog = this.getBlob(parameterIndex);
if(blog == null)
return null;
Serializable object = null;
InputStream in = null;
java.io.ObjectInputStream objectin = null;
try
{
in = blog.getBinaryStream();
objectin = new ObjectInputStream(in);
object = (Serializable)objectin.readObject();
}
catch(SQLException e)
{
throw e;
}
catch(Exception e)
{
throw new NestedSQLException(e);
}
finally
{
if(in != null)
try
{
in.close();
}
catch (IOException e)
{
// // TODO Auto-generated catch block
// e.printStackTrace();
}
if(objectin != null)
try
{
objectin.close();
}
catch (IOException e)
{
// // TODO Auto-generated catch block
// e.printStackTrace();
}
}
return object;
}
}