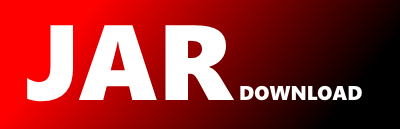
com.frameworkset.common.poolman.util.SQLResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bboss-persistent Show documentation
Show all versions of bboss-persistent Show documentation
bboss is a j2ee framework include aop/ioc,mvc,persistent,taglib,rpc,event ,bean-xml serializable and so on.http://www.bbossgroups.com
The newest version!
/*
* Copyright 2008 biaoping.yin
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.frameworkset.common.poolman.util;
import java.io.Serializable;
import java.util.HashMap;
/**
* SQLResult is a simplified means of keeping track of
* results returned from SQL queries. It encapsulates
* the HashMap array returned from a query made by SQLUtil.
*/
public class SQLResult implements Serializable{
protected HashMap[] records;
private int index;
/**
* Build a SQLResult object from an array of HashMaps
* returned from a SQLUtil query.
*/
public SQLResult(HashMap[] h) {
this.records = h;
this.index = 0;
}
public boolean hasNext() {
if (null == this.records)
return false;
if (this.index < this.records.length)
return true;
return false;
}
public HashMap next() {
this.index++;
return records[(this.index - 1)];
}
public int size() {
if (null == this.records)
return 0;
return records.length;
}
public Object getObject(String name) {
HashMap h = records[index];
// if (h.containsKey(name)) {
// return h.get(name);
// }
// else {
// for (Enumeration enum_ = h.keys(); enum_.hasMoreElements();) {
// String colname = enum_.nextElement().toString();
// if (colname.toLowerCase().equals(name.toLowerCase()))
// return h.get(colname);
// }
// }
return h.get(name.toUpperCase());
}
public String getString(String name) {
Object o = getObject(name);
if (o != null) {
if (o instanceof java.lang.String)
return (String) o;
else
throw new ClassCastException();
}
return null;
}
// public String toString() {
//
// if (records == null)
// return "SQLResult [0]";
//
// StringBuilder out = new StringBuilder();
// out.append("SQLResult [" + records.length + "]\n");
// if (records.length > 0) {
// out.append("[");
// HashMap h = records[0];
// Enumeration keys = h.keys();
// while (keys.hasMoreElements()) {
// String key = (String) keys.nextElement();
// out.append(key);
// if (keys.hasMoreElements())
// out.append(", ");
// }
// out.append("]\n");
// out.append("[");
// keys = h.keys();
// while (keys.hasMoreElements()) {
// String key = (String) keys.nextElement();
// Object o = h.get(key);
// String ClassName = o.getClass().getName();
//
// out.append(ClassName);
// if (keys.hasMoreElements())
// out.append(", ");
// }
// out.append("]\n\n");
//
// for (int n = 0; n < records.length; n++) {
// h = records[n];
// out.append("[");
// keys = h.keys();
// while (keys.hasMoreElements()) {
// String key = (String) keys.nextElement();
// Object o = h.get(key);
//
// out.append(o.toString());
// if (keys.hasMoreElements())
// out.append(", ");
// }
// out.append("]\n");
// }
//
// }
// return out.toString();
// }
// public String toHtml() {
//
// if (records == null)
// return "SQLResult [0]
";
//
// StringBuilder out = new StringBuilder();
// //out.append("SQLResult ["+records.length+"]
");
// out.append("");
// if (records.length > 0) {
// out.append("");
// HashMap h = records[0];
// Enumeration keys = h.keys();
// while (keys.hasMoreElements()) {
// String key = (String) keys.nextElement();
// out.append("");
// out.append(key);
// out.append(" ");
// }
// out.append(" ");
// out.append("");
// keys = h.keys();
// while (keys.hasMoreElements()) {
// String key = (String) keys.nextElement();
// Object o = h.get(key);
// String ClassName = o.getClass().getName();
//
// out.append("");
// out.append("Java Type:
");
// out.append(ClassName);
// out.append(" ");
// }
// out.append(" ");
//
// for (int n = 0; n < records.length; n++) {
// h = records[n];
// out.append("");
// keys = h.keys();
// while (keys.hasMoreElements()) {
// String key = (String) keys.nextElement();
// Object o = h.get(key);
//
// out.append("");
// out.append(o.toString());
// out.append(" ");
// }
// out.append(" ");
// }
// out.append("
");
// }
// return out.toString();
// }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy