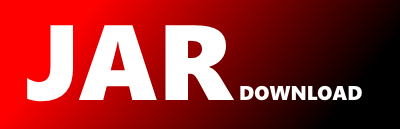
com.frameworkset.orm.engine.model.Database Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bboss-persistent Show documentation
Show all versions of bboss-persistent Show documentation
bboss is a j2ee framework include aop/ioc,mvc,persistent,taglib,rpc,event ,bean-xml serializable and so on.http://www.bbossgroups.com
The newest version!
/* * Copyright 2008 biaoping.yin * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package com.frameworkset.orm.engine.model; /* * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ import com.frameworkset.orm.engine.EngineException; import com.frameworkset.orm.platform.Platform; import com.frameworkset.orm.platform.PlatformFactory; import org.xml.sax.Attributes; import java.io.Serializable; import java.util.*; /** * A class for holding application data structures. * * @author John McNally * @author Daniel Rall * @author IDMethod.ID_BROKER
to create ids for torque OM * objects. * @return true if there is at least one table in this database that * uses theIDMethod.ID_BROKER
method of generating * ids. returns false otherwise. */ public boolean requiresIdTable() { Iterator iter = getTables().iterator(); while (iter.hasNext()) { Table table = (Table) iter.next(); if (table.getIdMethod().equals(IDMethod.ID_BROKER)) { return true; } } return false; } /** * Initializes the model. * * @throws EngineException */ public void doFinalInitialization() throws EngineException { Iterator iter = getTables().iterator(); while (iter.hasNext()) { Table currTable = (Table) iter.next(); // check schema integrity // if idMethod="autoincrement", make sure a column is // specified as autoIncrement="true" // FIXME: Handle idMethod="native" via DB adapter. // TODO autoincrement is no longer supported!!! if (currTable.getIdMethod().equals("autoincrement")) { boolean foundOne = false; Iterator colIter = currTable.getColumns().iterator(); while (colIter.hasNext() && !foundOne) { foundOne = ((Column) colIter.next()).isAutoIncrement(); } if (!foundOne) { String errorMessage = "Table '" + currTable.getName() + "' is marked as autoincrement, but it does not " + "have a column which declared as the one to " + "auto increment (i.e. autoIncrement=\"true\")\n"; throw new EngineException("Error in XML schema: " + errorMessage); } } currTable.doFinalInitialization(); // setup reverse fk relations Iterator fks = currTable.getForeignKeys().iterator(); while (fks.hasNext()) { ForeignKey currFK = (ForeignKey) fks.next(); Table foreignTable = getTable(currFK.getForeignTableName()); if (foreignTable == null) { throw new EngineException("Attempt to set foreign" + " key to nonexistent table, " + currFK.getForeignTableName()); } else { // TODO check type and size List referrers = foreignTable.getReferrers(); if ((referrers == null || !referrers.contains(currFK))) { foreignTable.addReferrer(currFK); } // local column references Iterator localColumnNames = currFK.getLocalColumns().iterator(); while (localColumnNames.hasNext()) { Column local = currTable .getColumn((String) localColumnNames.next()); // give notice of a schema inconsistency. // note we do not prevent the npe as there is nothing // that we can do, if it is to occur. if (local == null) { throw new EngineException("Attempt to define foreign" + " key with nonexistent column in table, " + currTable.getName()); } else { //check for foreign pk's if (local.isPrimaryKey()) { currTable.setContainsForeignPK(true); } } } // foreign column references Iterator foreignColumnNames = currFK.getForeignColumns().iterator(); while (foreignColumnNames.hasNext()) { String foreignColumnName = (String) foreignColumnNames.next(); Column foreign = foreignTable.getColumn(foreignColumnName); // if the foreign column does not exist, we may have an // external reference or a misspelling if (foreign == null) { throw new EngineException("Attempt to set foreign" + " key to nonexistent column: table=" + currTable.getName() + ", foreign column=" + foreignColumnName); } else { foreign.addReferrer(currFK); } } } } } } /** * Creats a string representation of this Database. * The representation is given in xml format. * * @return string representation in xml */ public String toString() { StringBuilder result = new StringBuilder(); result.append ("\n"); //noted by biaoping.yin on 2005.9.7 // result.append ("\n"); result.append("\n"); result.append("\n"); for (Iterator i = tableList.iterator(); i.hasNext();) { result.append(i.next()); } result.append(" "); return result.toString(); } /** * 将一个数据库中的所有表复制到你一个数据库中 * * @param db Database */ public void addDataBase(Database db) { this.setFileNames(db.getFileNames()); this.addTables(db.getTables()); } private void addTables(List tables) { //this.tableList.addAll(tables); Iterator itr = tables.iterator(); for(;itr.hasNext();) { Table table = (Table)itr.next(); if(!contain(table)) this.addTable(table); } } /** * 判断是否包含同一个表 * @param table * @return */ private boolean contain(Table table) { for(Iterator itr = tableList.iterator();itr.hasNext();) { Table tb = (Table)itr.next(); if(tb.equals(table)) { return true; } } return false; } }
© 2015 - 2024 Weber Informatics LLC | Privacy Policy