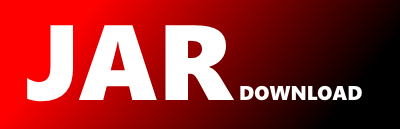
com.frameworkset.orm.platform.PlatformDerbyImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bboss-persistent Show documentation
Show all versions of bboss-persistent Show documentation
bboss is a j2ee framework include aop/ioc,mvc,persistent,taglib,rpc,event ,bean-xml serializable and so on.http://www.bbossgroups.com
package com.frameworkset.orm.platform;
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import com.frameworkset.orm.engine.model.Domain;
import com.frameworkset.orm.engine.model.SchemaType;
import com.frameworkset.orm.engine.model.SizedForBitDataDomain;
/**
* Derby Platform implementation.
*
* @author Johnny Macchione
* @author Greg Monroe
* @version $Id: PlatformDerbyImpl.java 482880 2006-12-06 03:55:24Z gmonroe $
*/
public class PlatformDerbyImpl extends PlatformDefaultImpl
{
/**
* Default constructor.
*/
public PlatformDerbyImpl()
{
super();
initialize();
}
/**
* Initializes db specific domain mapping.
*/
private void initialize()
{
setSchemaDomainMapping(
new Domain(SchemaType.LONGVARCHAR, "LONG VARCHAR"));
setSchemaDomainMapping( new SizedForBitDataDomain(
SchemaType.VARBINARY, "VARCHAR", "32672"));
setSchemaDomainMapping(
new SizedForBitDataDomain(SchemaType.BINARY, "CHAR", "1"));
setSchemaDomainMapping( new Domain(SchemaType.LONGVARBINARY,
"LONG VARCHAR FOR BIT DATA"));
setSchemaDomainMapping(
new Domain(SchemaType.LONGVARCHAR, "LONG VARCHAR"));
setSchemaDomainMapping(
new Domain(SchemaType.BIT,"CHAR(1)"));
setSchemaDomainMapping(
new Domain(SchemaType.TINYINT, "SMALLINT"));
}
/**
* @see Platform#getMaxColumnNameLength()
*/
public int getMaxColumnNameLength()
{
return 128;
}
/**
* @see Platform#getAutoIncrement()
*/
public String getAutoIncrement()
{
return "GENERATED BY DEFAULT AS IDENTITY";
}
/**
* @see Platform#getNativeIdMethod()
*/
public String getNativeIdMethod()
{
return Platform.IDENTITY;
}
/**
* @see Platform#hasScale(String)
*/
public boolean hasScale(String sqlType)
{
return "NUMERIC".equals(sqlType) || "DECIMAL".equals(sqlType);
}
/**
* @see Platform#hasSize(String)
*/
public boolean hasSize(String sqlType)
{
return "NUMERIC".equals(sqlType) || "DECIMAL".equals(sqlType)
|| "VARCHAR".equals(sqlType) || "CHAR".equals(sqlType)
|| "BINARY".equals(sqlType) || "VARBINARY".equals(sqlType)
|| "BLOB".equals(sqlType) || "CLOB".equals(sqlType);
}
/**
* @return Only produces a SQL fragment if null values are
* disallowed.
* @see Platform#getNullString(boolean)
*/
public String getNullString(boolean notNull)
{
// TODO: Check whether this is true for all DBs. Also verify
// the old Sybase templates.
return (notNull ? " NOT NULL" : "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy