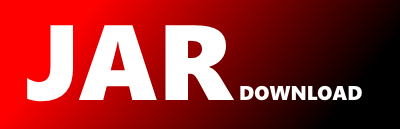
com.frameworkset.util.RegexUtilOro Maven / Gradle / Ivy
//package com.frameworkset.util;
//
//import org.slf4j.Logger;
//import org.slf4j.LoggerFactory;
//
//import java.util.ArrayList;
//import java.util.List;
//
///**
// * Title:
// *
// * Description:
// *
// * Copyright: Copyright (c) 2005
// *
// * Company:
// *
// * @author biaoping.yin
// * @version 1.0
// */
//public class RegexUtilOro {
// private static Logger log = LoggerFactory.getLogger(RegexUtilOro.class);
// private static final char
// __CASE_INSENSITIVE = 0x0001,
// __GLOBAL = 0x0002,
// __KEEP = 0x0004,
// __MULTILINE = 0x0008,
// __SINGLELINE = 0x0010,
// __EXTENDED = 0x0020,
// __READ_ONLY = 0x8000;
// public static final int SINGLELINE_MASK = __SINGLELINE;
// public static final int DEFAULT_MASK = 0;
// public static final int default_mask = SINGLELINE_MASK | DEFAULT_MASK;
// public static String[] parser(String src, String regex) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
// boolean match = matcher.matches(src, pattern);
//
// if (match) {
// result = matcher.getMatch();
// int size = result.groups() - 1;
// tokens = new String[size];
// for (int i = 0; i < size; i++) {
// tokens[i] = result.group(i + 1);
// }
// }
// return tokens;
// }
//
// /**
// * 从匹配regex的src字符串中提取部分子字符串
// * @param src
// * @param regex
// * @return
// */
// public static String[] matchWithPatternMatcherInput(String src, String regex) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
// List sets = new ArrayList();
// PatternMatcherInput input = new PatternMatcherInput(src);
//
//
// while (matcher.matches(input, pattern)) {
// result = matcher.getMatch();
// int size = result.groups();
//
// for (int i = 1; i < size; i++) {
// sets.add(result.group(i));
// }
// }
// tokens = new String[sets.size()];
// sets.toArray(tokens);
// return tokens;
// }
//
// public static String[] match(String src, String regex) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
//
// if(matcher.matches(src, pattern)) {
// result = matcher.getMatch();
// int size = result.groups() - 1;
// tokens = new String[size];
// for (int i = 0; i < size; i++) {
// tokens[i] = result.group(i + 1);
// }
// }
// return tokens;
// }
//
// /**
// * 从src中提取包含模式regex的子字符串
// * @param src
// * @param regex
// * @return
// */
// public static String[] containWithPatternMatcherInput(String src, String regex) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
// List sets = new ArrayList();
// PatternMatcherInput input = new PatternMatcherInput(src);
//
//
// while (matcher.contains(input, pattern)) {
// result = matcher.getMatch();
//
//
// int size = result.groups();
//
// for (int i = 1; i < size; i++) {
// sets.add(result.group(i));
// }
// }
// tokens = new String[sets.size()];
// sets.toArray(tokens);
// return tokens;
// }
//
// /**
// * 从src中提取包含模式regex的子字符串
// * @param src
// * @param regex
// * @return
// */
// public static String[] containWithPatternMatcherInput(String src, String regex,int mask) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
// List sets = new ArrayList();
// PatternMatcherInput input = new PatternMatcherInput(src);
//
//
// while (matcher.contains(input, pattern)) {
// result = matcher.getMatch();
//
//
// int size = result.groups();
//
// for (int i = 1; i < size; i++) {
// sets.add(result.group(i));
// }
// }
// tokens = new String[sets.size()];
// sets.toArray(tokens);
// return tokens;
// }
//
// /**
// * 从串src中析取匹配regex模式的所有字符串,并且用substitution替换匹配上模式的子符串
// * @param src
// * @param regex
// * @param substitution
// * @return String[][]二维数组,第一维表示替换后的src,第二维表示匹配regex的所有的子串数组
// */
// public static String[][] contain2ndReplaceWithPatternMatcherInput(String src, String regex,String substitution) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
// List sets = new ArrayList();
// PatternMatcherInput input = new PatternMatcherInput(src);
//
//
// while (matcher.contains(input, pattern)) {
// result = matcher.getMatch();
//
//
// int size = result.groups();
//
// for (int i = 1; i < size; i++) {
// sets.add(result.group(i));
// }
// }
// String[][] retvalue = null;
// String newsrc = src;
// if(sets.size() > 0)
// {
// newsrc = Util.substitute(matcher, pattern, new Perl5Substitution(substitution),
// src, Util.SUBSTITUTE_ALL);
// }
//
// tokens = new String[sets.size()];
// sets.toArray(tokens);
// retvalue = new String[][] {{newsrc},tokens};
// return retvalue;
// }
//
// /**
// * 从串src中析取匹配regex模式的所有字符串,并且用substitution替换匹配上模式的子符串
// * @param src
// * @param regex
// * @param substitution
// * @return String[][]二维数组,第一维表示替换后的src,第二维表示匹配regex的所有的子串数组
// */
// public static String[][] contain2ndReplaceWithPatternMatcherInput(String src, String regex,String substitution,int MASK) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// MASK);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
// List sets = new ArrayList();
// PatternMatcherInput input = new PatternMatcherInput(src);
//
//
// while (matcher.contains(input, pattern)) {
// result = matcher.getMatch();
//
//
// int size = result.groups();
//
// for (int i = 1; i < size; i++) {
// sets.add(result.group(i));
// }
// }
// String[][] retvalue = null;
// String newsrc = src;
// if(sets.size() > 0)
// {
// newsrc = Util.substitute(matcher, pattern, new Perl5Substitution(substitution),
// src, Util.SUBSTITUTE_ALL);
// }
//
// tokens = new String[sets.size()];
// sets.toArray(tokens);
// retvalue = new String[][] {{newsrc},tokens};
// return retvalue;
// }
//
// /**
// * 从包含
// * @param src
// * @param regex
// * @return
// */
// public static String[] contain(String src, String regex) {
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return null;
// }
// PatternMatcher matcher = new Perl5Matcher();
// MatchResult result = null;
// String[] tokens = null;
//
//
//
// if (matcher.contains(src, pattern)) {
// result = matcher.getMatch();
// int size = result.groups() - 1;
// tokens = new String[size];
// for (int i = 0; i < size; i++) {
// tokens[i] = result.group(i + 1);
// }
// }
// return tokens;
// }
//
//
// public static boolean isContain(String src, String regex) {
// if(src == null )
// return false;
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
//
// return false;
// }
// PatternMatcher matcher = new Perl5Matcher();
// return matcher.contains(src, pattern);
//
// }
//
//
//
// public RegexUtilOro() {
// }
//
// /**
// * 检查src与正则表达式regex匹配结果
// * @param src String
// * @param regex String
// * @return boolean
// */
// public static boolean isMatch(String src, String regex) {
// if(src == null )
// return false;
// boolean flag = false;
// String patternStr = regex;
//
// /**
// * 编译正则表达式patternStr,并用该表达式与传入的src字符串进行模式匹配,
// * 如果匹配正确,则从匹配对象中提取出以上定义好的6部分,存放到数组中并返回
// * 该数组
// */
//
// PatternCompiler compiler = new Perl5Compiler();
// Pattern pattern = null;
// try {
// pattern = compiler.compile(patternStr,
// default_mask);
// } catch (MalformedPatternException e) {
// e.printStackTrace();
// return false;
// }
// PatternMatcher matcher = new Perl5Matcher();
//// log.debug("src:" + src);;
//// log.debug("pattern:" + pattern);
//// log.debug("regex:" + regex);
// flag = matcher.matches(src, pattern);
// return flag;
// }
//
// /**
// * 将content中符合模式pattern的字符串替换为newstr字符串
// * 暂未实现
// * @param content
// * @param pattern
// * @param newstr
// */
// public static void replace(String content,String pattern,String newstr)
// {
//
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy