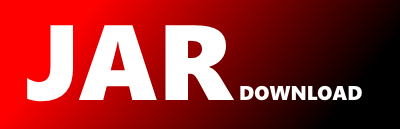
com.bebound.spring.controller.BeBoundController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-utils Show documentation
Show all versions of spring-utils Show documentation
Handle Be-Bound requests with a spring server
The newest version!
package com.bebound.spring.controller;
import com.bebound.spring.annotation.OperationName;
import com.bebound.spring.api.Operation;
import com.bebound.spring.exception.OperationNotFoundException;
import com.bebound.spring.model.Request;
import com.bebound.spring.model.Response;
import com.bebound.spring.utils.C;
import com.google.gson.*;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.context.annotation.ClassPathScanningCandidateComponentProvider;
import org.springframework.core.type.filter.AssignableTypeFilter;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.RequestBody;
import java.lang.annotation.Annotation;
import java.lang.reflect.InvocationTargetException;
import java.util.logging.Logger;
/**
* Created by mbiamont on 16/06/2016.
*/
public abstract class BeBoundController {
@Value("${bebound.operations}")
private String operationPackageName;
public ResponseEntity performBeBoundRequest(@RequestBody String body) {
JsonParser parser = new JsonParser();
JsonElement jsonRequest = parser.parse(body);
JsonObject jsonRequestObject = jsonRequest.getAsJsonObject();
if (!jsonRequestObject.has(C.Parameter.PARAM_APP_NAME)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_APP_NAME + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_APP_NAME + " missing", HttpStatus.BAD_REQUEST);
}
if (!jsonRequestObject.has(C.Parameter.PARAM_APP_ID)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_APP_ID + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_APP_ID + " missing", HttpStatus.BAD_REQUEST);
}
if (!jsonRequestObject.has(C.Parameter.PARAM_APP_VERSION)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_APP_VERSION + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_APP_VERSION + " missing", HttpStatus.BAD_REQUEST);
}
if (!jsonRequestObject.has(C.Parameter.PARAM_OPERATION_NAME)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_OPERATION_NAME + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_OPERATION_NAME + " missing", HttpStatus.BAD_REQUEST);
}
if (!jsonRequestObject.has(C.Parameter.PARAM_PARAMETER_LIST)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_PARAMETER_LIST + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_PARAMETER_LIST + " missing", HttpStatus.BAD_REQUEST);
}
if (!jsonRequestObject.has(C.Parameter.PARAM_PHONE_NUMBER)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_PHONE_NUMBER + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_PHONE_NUMBER + " missing", HttpStatus.BAD_REQUEST);
}
if (!jsonRequestObject.has(C.Parameter.PARAM_TRANSPORT)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_TRANSPORT + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_TRANSPORT + " missing", HttpStatus.BAD_REQUEST);
}
if (!jsonRequestObject.has(C.Parameter.PARAM_USER_ID)) {
Logger.getLogger("Be-Bound").severe("Impossible to handle incoming request: " + C.Parameter.PARAM_USER_ID + " missing");
return new ResponseEntity<>(C.Parameter.PARAM_USER_ID + " missing", HttpStatus.BAD_REQUEST);
}
try {
Gson gson = new GsonBuilder().create();
Request.MapRequest request = gson.fromJson(body, Request.MapRequest.class);
request.setJsonParams(gson.toJson(request.getParameters()));
Response response;
try {
response = performRequest(request);
} catch (OperationNotFoundException e) {
Logger.getLogger("Be-Bound").severe("Cannot found a class Operation in the package '" + getOperationsPackageName() + "' which can handle the operation '" + e.getOperationName() + "'..");
return new ResponseEntity<>("Operation '" + e.getOperationName() + "' cannot be found on the server.", HttpStatus.INTERNAL_SERVER_ERROR);
}
String stringResponse = new GsonBuilder().create().toJson(response);
return new ResponseEntity<>(stringResponse, HttpStatus.OK);
} catch (ClassNotFoundException | NoSuchMethodException | InvocationTargetException | IllegalAccessException | InstantiationException e) {
e.printStackTrace();
}
return new ResponseEntity<>("", HttpStatus.INTERNAL_SERVER_ERROR);
}
private Response performRequest(Request request) throws ClassNotFoundException, NoSuchMethodException, InvocationTargetException, IllegalAccessException, InstantiationException, OperationNotFoundException {
ClassPathScanningCandidateComponentProvider scanner = new ClassPathScanningCandidateComponentProvider(false);
scanner.addIncludeFilter(new AssignableTypeFilter(Operation.class));
for (BeanDefinition bd : scanner.findCandidateComponents(getOperationsPackageName())) {
Class clazz = Class.forName(bd.getBeanClassName());
for (Annotation annotation : clazz.getAnnotations())
if (annotation instanceof OperationName && ((OperationName) annotation).value().equals(request.getOperationName()))
return callMethod(request, clazz, clazz);
}
throw new OperationNotFoundException(request.getOperationName());
}
private Response callMethod(Request request, Class clazz, Class originalClass) throws IllegalAccessException, InstantiationException, InvocationTargetException {
try {
return (Response) clazz.getDeclaredMethod("performRequest", Request.class).invoke(originalClass.newInstance(), request);
} catch (NoSuchMethodException e) {
return callMethod(request, clazz.getSuperclass(), originalClass);
}
}
private String getOperationsPackageName() {
return operationPackageName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy