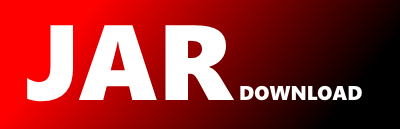
com.diboot.core.mapper.BaseCrudMapper Maven / Gradle / Ivy
Show all versions of diboot-core Show documentation
/*
* Copyright (c) 2015-2020, www.dibo.ltd ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.diboot.core.mapper;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.core.metadata.TableInfo;
import com.baomidou.mybatisplus.core.metadata.TableInfoHelper;
import com.baomidou.mybatisplus.core.toolkit.Assert;
import com.baomidou.mybatisplus.core.toolkit.Constants;
import com.baomidou.mybatisplus.core.toolkit.ReflectionKit;
import com.baomidou.mybatisplus.core.toolkit.StringUtils;
import org.apache.ibatis.annotations.*;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
/**
* 基础CRUD的父类Mapper
* @author [email protected]
* @version v2.0
* @date 2018/12/22
*/
public interface BaseCrudMapper extends BaseMapper {
default List findAll() {
return this.selectList(null);
}
default void deleteAll() {
this.delete(null);
}
int deleteInRecycleBin(@Param(Constants.WRAPPER) Wrapper queryWrapper);
T selectByIdInRecycleBin(Serializable id);
int deleteBatchIdsInRecycleBin(@Param(Constants.COLL) Collection> idList);
int updateInRecycleBin(@Param(Constants.ENTITY) T entity, @Param(Constants.WRAPPER) Wrapper updateWrapper);
> P selectPageInRecycleBin(P page, @Param("ew") Wrapper queryWrapper);
default boolean save(T entity) {
if (null == entity) {
return false;
} else {
TableInfo tableInfo = TableInfoHelper.getTableInfo(entity.getClass());
Assert.notNull(tableInfo, "error: can not execute. because can not find cache of TableInfo for entity!", new Object[0]);
String keyProperty = tableInfo.getKeyProperty();
Assert.notEmpty(keyProperty, "error: can not execute. because can not find column for id from entity!", new Object[0]);
Object idVal = ReflectionKit.getFieldValue(entity, tableInfo.getKeyProperty());
return !StringUtils.checkValNull(idVal) && !Objects.isNull(this.selectById((Serializable)idVal)) ? this.updateById(entity) > 0 : this.insert(entity) > 0;
}
}
/***
* 通过id撤回当前记录的删除状态
* @param tableName
* @param id
* @return
*/
@Update("UPDATE `${tableName}` SET is_deleted=0 WHERE id=#{id}")
int cancelDeletedById(@Param("tableName") String tableName, @Param("id") Serializable id);
@Delete("DELETE FROM `${tableName}` WHERE `${fieldName}`=#{id}")
int deleteByTableNameAndFieldNameValue(@Param("tableName") String tableName, @Param("fieldName") String fieldName, @Param("id") Serializable id);
@Insert("INSERT INTO `${tableName}` (${fieldNames}) VALUES (${values})")
int insertByTableNameAndFieldNameValues(@Param("tableName") String tableName, @Param("fieldNames") String fieldNames, @Param("values") String values);
@Select("")
List