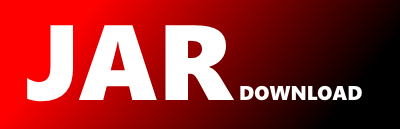
com.diboot.core.service.BaseService Maven / Gradle / Ivy
Show all versions of diboot-core Show documentation
/*
* Copyright (c) 2015-2020, www.dibo.ltd ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.diboot.core.service;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.core.toolkit.CollectionUtils;
import com.baomidou.mybatisplus.core.toolkit.support.SFunction;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.IService;
import com.baomidou.mybatisplus.extension.toolkit.SqlHelper;
import com.diboot.core.binding.binder.EntityBinder;
import com.diboot.core.binding.binder.EntityListBinder;
import com.diboot.core.binding.binder.FieldBinder;
import com.diboot.core.binding.binder.FieldListBinder;
import com.baomidou.mybatisplus.extension.conditions.query.LambdaQueryChainWrapper;
import com.baomidou.mybatisplus.extension.conditions.query.QueryChainWrapper;
import com.baomidou.mybatisplus.extension.conditions.update.LambdaUpdateChainWrapper;
import com.baomidou.mybatisplus.extension.conditions.update.UpdateChainWrapper;
import com.diboot.core.dto.SortParamDTO;
import com.diboot.core.mapper.BaseCrudMapper;
import com.diboot.core.util.IGetter;
import com.diboot.core.util.ISetter;
import com.diboot.core.vo.LabelValue;
import com.diboot.core.vo.Pagination;
import org.springframework.lang.Nullable;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
/**
* 基础服务Service
* @author [email protected]
* @version 2.0
* @date 2019/01/01
*/
public interface BaseService extends IService {
/**
* 获取对应 entity 的 BaseMapper
*
* @return BaseMapper
*/
BaseMapper getMapper();
/**
* 获取Entity实体
* @param id 主键
* @return entity
*/
/**
* comment by wangxin
*
T getEntity(Serializable id);
*/
/**
* comment by wangxin
* 创建Entity实体
* @param entity
* @return true:成功, false:失败
boolean createEntity(T entity);
*/
/***
* comment by wangxin
* 批量创建Entity
* @param entityList 实体对象列表
* @return true:成功, false: 失败
boolean createEntities(Collection entityList);
*/
/**
* 添加entity 及 其关联子项entities
* @param entity 主表entity
* @param relatedEntities 关联表entities
* @param relatedEntitySetter 关联Entity类的setter
* @return
*/
boolean createEntityAndRelatedEntities(T entity, List relatedEntities, ISetter relatedEntitySetter);
/**
* 添加entity 及 其关联子项entities
* @param entity 主表entity
* @param relatedEntities 关联表entities
* @param relatedEntitySetter 关联Entity类的setter
* @return
*/
boolean createEntityAndRelatedEntities(T entity, List relatedEntities, ISetter relatedEntitySetter, ISetter otherEntitySetter, Object value);
/**
* 创建或更新n-n关联
* (在主对象的service中调用,不依赖中间表service实现中间表操作)
*
* @param driverIdGetter 驱动对象getter
* @param driverId 驱动对象ID
* @param followerIdGetter 从动对象getter
* @param followerIdList 从动对象id集合
* @return
*/
boolean createOrUpdateN2NRelations(SFunction driverIdGetter, Object driverId,
SFunction followerIdGetter, Collection extends Serializable> followerIdList);
/**
* 创建或更新n-n关联
* (在主对象的service中调用,不依赖中间表service实现中间表操作)
*
* 可自定附加条件、参数
*
* @param driverIdGetter 驱动对象getter
* @param driverId 驱动对象ID
* @param followerIdGetter 从动对象getter
* @param followerIdList 从动对象id集合
* @param queryConsumer 附加查询条件
* @param setConsumer 附加插入参数
* @param 关联对象类型
* @return
*/
boolean createOrUpdateN2NRelations(SFunction driverIdGetter, Object driverId,
SFunction followerIdGetter, Collection extends Serializable> followerIdList,
Consumer> queryConsumer, Consumer setConsumer);
/**
* 创建或更新n-n关联
* 只依赖注解
*
* @param entity 保存数据的实体
* @param updateRelationFields 要更新的关联字段名
* @return boolean 是否成功
*/
boolean createOrUpdateN2NRelations(T entity, List updateRelationFields);
/**
* 创建或更新n-n关联
* 只依赖注解
*
* @param entity 保存数据的实体
* @param updateRelationFields 要更新的关联字段名
* @return boolean 是否成功
*/
boolean createOrUpdateAndRelatedRelations(T entity, List updateRelationFields);
/**
* comment by wangxin
* 更新Entity实体
* @param entity
* @return
boolean updateEntity(T entity);
*/
/**
* comment by wangxin
* 更新Entity实体(更新符合条件的所有非空字段)
* @param entity
* @param updateCriteria
* @return
boolean updateEntity(T entity, Wrapper updateCriteria);
*/
/**
* comment by wangxin
* 更新Entity实体(仅更新updateWrapper.set指定的字段)
* @param updateWrapper
* @return
boolean updateEntity(Wrapper updateWrapper);
*/
/**
* comment by wangxin
* 批量更新entity
* @param entityList
* @return
boolean updateEntities(Collection entityList);
*/
/**
* comment by wangxin
* 创建或更新entity(entity.id存在则新建,否则更新)
* @param entity
* @return
boolean createOrUpdateEntity(T entity);
*/
/**
* comment by wangxin
* 批量创建或更新entity(entity.id存在则新建,否则更新)
* @param entityList
* @return
boolean createOrUpdateEntities(Collection entityList);
*/
/**
* 更新entity 及 其关联子项entities
* @param entity 主表entity
* @param relatedEntities 关联表entities
* @param relatedEntitySetter 关联Entity类的setter
* @return
*/
boolean updateEntityAndRelatedEntities(T entity, List relatedEntities, ISetter relatedEntitySetter);
/**
* 添加entity 及 其关联子项entities
* @param entity 主表entity
* @param relatedEntities 关联表entities
* @param relatedEntitySetter 关联Entity类的setter
* @return
*/
boolean updateEntityAndRelatedEntities(T entity, List relatedEntities, ISetter relatedEntitySetter, ISetter otherEntitySetter, Object value);
/**
* 删除entity 及 其关联子项entities
* @param id 待删除entity的主键
* @param relatedEntityClass 待删除关联Entity类
* @param relatedEntitySetter 待删除类的setter方法
* @return
*/
boolean deleteEntityAndRelatedEntities(Serializable id, Class relatedEntityClass, ISetter relatedEntitySetter);
/**
* 删除entity 及 其关联子项entities
* @param id 待删除entity的主键
* @param relatedEntityClass 待删除关联Entity类
* @param relatedEntitySetter 待删除类的setter方法
* @return
*/
boolean deleteEntityAndRelatedEntities(Serializable id, Class relatedEntityClass, ISetter relatedEntitySetter, ISetter otherEntitySetter, Object value);
/**
* comment by wangxin
* 根据主键删除实体
* @param id 主键
* @return true:成功, false:失败
boolean deleteEntity(Serializable id);
*/
/**
* 根据主键撤销删除
* @param id
* @return
*/
boolean cancelDeletedById(Serializable id);
/**
* comment by wangxin
* 按条件删除实体
* @param queryWrapper
* @return
* @throws Exception
boolean deleteEntities(Wrapper queryWrapper);
*/
/**
* 根据指定字段及匹配值删除实体
* @param fieldKey 字段名
* @param fieldVal 字段值
* @return true:成功, false:失败
*/
boolean deleteEntity(String fieldKey, Object fieldVal);
/**
* comment by wangxin
* 批量删除指定id的实体
* @param entityIds
* @return
* @throws Exception
boolean deleteEntities(Collection extends Serializable> entityIds);
*/
/**
* 根据指定字段及值的条件删除匹配记录
* @param fldGetterFn
* @param fieldValue
* @return
* @param
* @param
*/
boolean deleteEntities(SFunction fldGetterFn, Object fieldValue);
/**
* comment by wangxin
* 获取符合条件的entity记录总数
* @return
long getEntityListCount(Wrapper queryWrapper);
*/
/**
* comment by wangxin
* 获取指定条件的Entity集合
* @param queryWrapper
* @return
* @throws Exception
List getEntityList(Wrapper queryWrapper);
*/
/**
* 获取指定条件的Entity集合
* @param queryWrapper
* @param pagination
* @return
* @throws Exception
*/
List getEntityList(Wrapper queryWrapper, Pagination pagination);
/**
* 获取指定条件的Entity集合
* @param queryWrapper
* @param page
* @return
* @throws Exception
*/
IPage getEntityPage(Wrapper queryWrapper, Page page);
/**
* 获取entity某个属性值
* @param idVal id值
* @param getterFn 返回属性getter
* @return
*/
FT getValueOfField(Serializable idVal, SFunction getterFn);
/**
* 获取entity某个属性值
* @param idFieldFn 查询字段
* @param idVal 查询字段值
* @param getterFn 返回属性getter
* @return
*/
FT getValueOfField(SFunction idFieldFn, Serializable idVal, SFunction getterFn);
/**
* 获取entity某个属性值
*
* @param queryWrapper
* @param getterFn
* @return
* @param
*/
FT getValueOfField(LambdaQueryWrapper queryWrapper, SFunction getterFn);
/**
* 根据指定的字段和值,获取匹配的结果字段值
* @param fieldKey
* @param fieldVal
* @param getterFn
* @return
* @param
*/
List getValuesOfField(String fieldKey, Object fieldVal, SFunction getterFn);
/**
* 获取指定条件的Entity ID集合
* @param queryWrapper
* @param getterFn
* @return
* @throws Exception
*/
List getValuesOfField(Wrapper queryWrapper, SFunction getterFn);
/**
* comment by wangxin
* 获取指定条件的Entity集合
* @param ids
* @return
List getEntityListByIds(List ids);
*/
/**
* 获取指定ID集合,获取映射map
*
* @param idFieldFn
* @param idValList
* @param getterFn
* @return
* @param
* @param
*/
Map getValueMapOfField(SFunction idFieldFn, List idValList, SFunction getterFn);
/**
* 获取指定数量的entity记录
* @param queryWrapper
* @param limitCount
* @return
* @throws Exception
*/
List getEntityListLimit(Wrapper queryWrapper, int limitCount);
/**
* 获取符合条件的一个Entity实体
* @param queryWrapper
* @return entity
*/
T getSingleEntity(Wrapper queryWrapper);
/**
* 是否存在符合条件的记录
* @param getterFn entity的getter方法
* @param value 需要检查的值
* @return
*/
boolean exists(SFunction getterFn, Object value);
/**
* 是否存在符合条件的记录
* @param queryWrapper
* @return
*/
boolean exists(Wrapper queryWrapper);
/**
* 检查值是否唯一
* @param getterFn
* @param value
* @param id
* @return
*/
boolean isValueUnique(SFunction getterFn, Object value, Serializable id);
/**
* 检查值是否唯一
* @param field
* @param value
* @param id
* @return
*/
boolean isValueUnique(String field, Object value, Serializable id);
/**
* comment by wangxin
* 获取指定属性的Map列表
* @param queryWrapper
* @return
*/
List