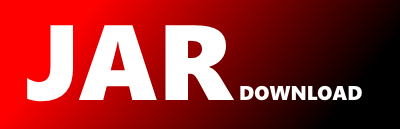
com.bekioui.jaxrs.security.util.JWTUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxrs-security Show documentation
Show all versions of jaxrs-security Show documentation
Provide an authorization filter that uses Authorization HTTP header to set JAX-RS API security context.
The newest version!
/**
* Copyright (C) 2016 Mehdi Bekioui ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.bekioui.jaxrs.security.util;
import static com.bekioui.jaxrs.security.token.ApplicationToken.IDENTIFIER;
import static com.bekioui.jaxrs.security.token.ApplicationToken.ROLES;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import com.auth0.jwt.JWTSigner;
import com.auth0.jwt.JWTVerifier;
import com.bekioui.jaxrs.security.token.ApplicationToken;
import com.bekioui.jaxrs.security.token.MultipleApplicationToken;
public final class JWTUtils {
public static String create(ApplicationToken token, String secret) {
Map claims = new HashMap<>();
claims.put(IDENTIFIER, token.identifier);
claims.put(ROLES, token.roles);
return create(claims, secret);
}
public static String create(MultipleApplicationToken token, String secret) {
Map claims = new HashMap<>();
claims.put(IDENTIFIER, token.identifier);
claims.put(ROLES, token.roles);
return create(claims, secret);
}
public static ApplicationToken verifyApplicationToken(String jwt, String secret) throws Exception {
return toApplicationToken(verify(jwt, secret));
}
public static MultipleApplicationToken verifyMultipleApplicationToken(String jwt, String secret) throws Exception {
return toMultiApplicationToken(verify(jwt, secret));
}
private static String create(Map claims, String secret) {
return new JWTSigner(secret).sign(claims);
}
private static Map verify(String jwt, String secret) throws Exception {
return new JWTVerifier(secret, "audience").verify(jwt);
}
@SuppressWarnings("unchecked")
private static ApplicationToken toApplicationToken(Map payload) {
return new ApplicationToken((String) payload.get(IDENTIFIER), (Set) payload.get(ROLES));
}
@SuppressWarnings("unchecked")
private static MultipleApplicationToken toMultiApplicationToken(Map payload) {
return new MultipleApplicationToken((String) payload.get(IDENTIFIER), (Map>) payload.get(ROLES));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy