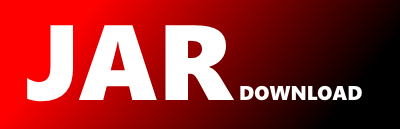
com.bekioui.netty.jaxrs.core.NettyServer Maven / Gradle / Ivy
/**
* Copyright 2016 Mehdi Bekioui
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.bekioui.netty.jaxrs.core;
import io.swagger.jaxrs.listing.SwaggerSerializers;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import javax.ws.rs.Path;
import javax.ws.rs.ext.Provider;
import org.jboss.resteasy.plugins.server.netty.NettyJaxrsServer;
import org.jboss.resteasy.spi.ResteasyDeployment;
import org.slf4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.ApplicationContext;
import org.springframework.stereotype.Component;
import com.bekioui.netty.jaxrs.filter.NettyCorsFilter;
import com.excilys.ebi.utils.spring.log.slf4j.InjectLogger;
@Component
public class NettyServer {
@Value("${netty.jaxrs.rootResourcePath:}")
private String rootResourcePath;
@Value("${netty.jaxrs.port:8080}")
private int port;
// Default: 1024 * 1024 * 10
@Value("${netty.jaxrs.maxRequestSize:10485760}")
private int maxRequestSize;
@Value("${netty.jaxrs.cors.enabled:false}")
private boolean corsEnabled;
@Value("${netty.jaxrs.swagger.enabled:false}")
private boolean swaggerEnabled;
@InjectLogger
private Logger logger;
@Autowired
private ApplicationContext applicationContext;
@Autowired
private SwaggerResource swaggerResource;
private NettyJaxrsServer server;
@PostConstruct
private void postConstruct() {
ResteasyDeployment deployment = new ResteasyDeployment();
resources(deployment);
providers(deployment);
server = new NettyJaxrsServer();
server.setDeployment(deployment);
server.setRootResourcePath(rootResourcePath);
server.setPort(port);
server.setMaxRequestSize(maxRequestSize);
server.setSecurityDomain(null);
server.start();
logger.info("Netty Jaxrs server started.");
}
@PreDestroy
private void preDestroy() {
server.stop();
}
private void resources(ResteasyDeployment deployment) {
Map resources = applicationContext.getBeansWithAnnotation(Path.class);
if (!swaggerEnabled) {
remove(resources, SwaggerResource.class);
} else {
Set> classes = new HashSet<>();
for (Object resource : resources.values()) {
classes.add(resource.getClass());
}
swaggerResource.addClasses(classes);
deployment.getProviders().add(new SwaggerSerializers());
}
deployment.getResources().addAll(resources.values());
}
private void providers(ResteasyDeployment deployment) {
Map providers = applicationContext.getBeansWithAnnotation(Provider.class);
if (!corsEnabled) {
remove(providers, NettyCorsFilter.class);
}
deployment.getProviders().addAll(providers.values());
}
private void remove(Map map, Class> clazz) {
for (Map.Entry entry : map.entrySet()) {
if (entry.getValue().getClass().equals(clazz)) {
map.remove(entry.getKey());
break;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy