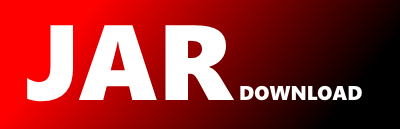
org.jetbrains.android.anko.annotations.annotationProviders.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anko-generator Show documentation
Show all versions of anko-generator Show documentation
The generator module from Anko.
The newest version!
/*
* Copyright 2016 JetBrains s.r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jetbrains.android.anko.annotations
import java.io.File
import java.util.zip.ZipFile
enum class ExternalAnnotation {
NotNull,
GenerateLayout,
GenerateView
}
interface AnnotationProvider {
fun getExternalAnnotations(packageName: String): Map>
}
class ZipFileAnnotationProvider(val zipFile: File) : AnnotationProvider {
private val archive by lazy { ZipFile(zipFile) }
override fun getExternalAnnotations(packageName: String): Map> {
val entryName = packageName.replace('.', '/') + "/annotations.xml"
val entry = archive.getEntry(entryName) ?: return emptyMap()
return archive.getInputStream(entry).reader().use {
parseAnnotations(parseXml(it.readText()))
}
}
}
class DirectoryAnnotationProvider(val directory: File) : AnnotationProvider {
override fun getExternalAnnotations(packageName: String): Map> {
val annotationFile = File(directory, packageName.replace('.', '/') + "/annotations.xml")
if (!annotationFile.exists()) return emptyMap()
return parseAnnotations(parseXml(annotationFile.readText()))
}
}
class CachingAnnotationProvider(val underlyingProvider: AnnotationProvider) : AnnotationProvider {
private val cache = hashMapOf>>()
override fun getExternalAnnotations(packageName: String) = cache.getOrPut(packageName) {
underlyingProvider.getExternalAnnotations(packageName)
}
}
class CompoundAnnotationProvider(vararg private val providers: AnnotationProvider) : AnnotationProvider {
override fun getExternalAnnotations(packageName: String): Map> {
val providerAnnotations = providers.map { it.getExternalAnnotations(packageName) }
val map = hashMapOf>()
for (providerAnnotationMap in providerAnnotations) {
for ((key, value) in providerAnnotationMap) {
val existingAnnotations = map[key]
if (existingAnnotations == null) {
map.put(key, value)
} else {
map.put(key, (value + existingAnnotations).toSet())
}
}
}
return map
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy