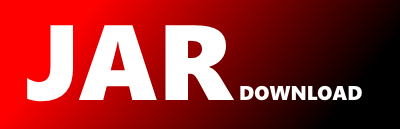
com.bertramlabs.plugins.karman.azure.AzureBlobStorageProvider.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of karman-azure Show documentation
Show all versions of karman-azure Show documentation
Karman Storage Provider interface for Azure Cloud Storage api
package com.bertramlabs.plugins.karman.azure
import com.bertramlabs.plugins.karman.Directory
import com.bertramlabs.plugins.karman.StorageProvider
import groovy.util.logging.Commons
import org.apache.http.HttpEntity
import org.apache.http.client.HttpClient
import org.apache.http.HttpResponse
import org.apache.http.client.methods.HttpGet
import org.apache.http.util.EntityUtils
import java.text.*;
/**
* Storage provider implementation for the Azure Storage PageBlob and Container API
* This is the starting point from which all calls to Azure originate for storing blobs within the Cloud File Containers
*
* Below is an example of how this might be initialized.
*
*
* {@code
* import com.bertramlabs.plugins.karman.StorageProvider
* def provider = StorageProvider(
* provider: 'azure-pageblob',
* storageAccount: 'storage account name',
* storageKey: 'storage key'
* )
*
* def blob = provider['container']['example.txt']
* blob.setBytes(byteArray)
* blob.save()
* }
*
*
* @author Bob Whiton
*/
@Commons
public class AzureBlobStorageProvider extends AzureStorageProvider {
static String providerName = "azure-pageblob"
public String getProviderName() {
return providerName
}
@Override
public String getEndpointUrl() {
def base = baseEndpointDomain ?: 'core.windows.net'
return "${protocol}://${storageAccount}.blob.${base}"
}
Directory getDirectory(String name) {
new AzureContainer(name: name, provider: this)
}
List getDirectories() {
def opts = [
verb: 'GET',
queryParams: [comp: 'list'],
path: '',
uri: "${getEndpointUrl()}".toString()
]
def (HttpClient client, HttpGet request) = prepareRequest(opts)
HttpResponse response = client.execute(request)
if(response.statusLine.statusCode != 200) {
HttpEntity responseEntity = response.getEntity()
log.error("Error fetching Directory List ${response.statusLine.statusCode}, content: ${responseEntity.content}")
EntityUtils.consume(response.entity)
return null
}
HttpEntity responseEntity = response.getEntity()
def xmlDoc = new XmlSlurper().parse(responseEntity.content)
EntityUtils.consume(response.entity)
def provider = this
def directories = []
xmlDoc.Containers?.Container?.each { container ->
directories << new AzureContainer(name: container.Name, provider: provider)
}
return directories
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy