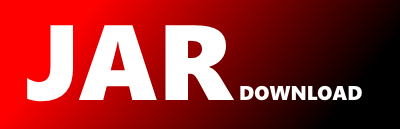
com.bimface.sdk.BimfaceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bimface-java-sdk Show documentation
Show all versions of bimface-java-sdk Show documentation
Bimface provide the required call java sdk.
The newest version!
package com.bimface.sdk;
import com.bimface.api.bean.compatible.response.BatchDeleteResultBean;
import com.bimface.api.bean.compatible.response.ShareLinkBean;
import com.bimface.api.bean.request.clashDetective.ClashDetectiveRequest;
import com.bimface.api.bean.request.extraction.ExtractionQueryRequest;
import com.bimface.api.bean.request.extraction.ExtractionRequest;
import com.bimface.api.bean.request.integrate.FileIntegrateRequest;
import com.bimface.api.bean.request.integrate.IntegrateQueryRequest;
import com.bimface.api.bean.request.modelCompare.CompareRequest;
import com.bimface.api.bean.request.modelCompare.ModelCompareQueryRequest;
import com.bimface.api.bean.request.modelCompare.ModelCompareRequest;
import com.bimface.api.bean.request.scene.AutoUpdateSceneLayerRequest;
import com.bimface.api.bean.request.translate.FileTranslateRequest;
import com.bimface.api.bean.request.translate.TranslateQueryRequest;
import com.bimface.api.bean.request.translate.TranslateSource;
import com.bimface.api.bean.request.v1.FileTranslateRequestV1;
import com.bimface.api.bean.response.*;
import com.bimface.api.bean.response.clashDetective.ClashDetectiveList;
import com.bimface.api.bean.response.clashDetective.ClashDetectiveResponse;
import com.bimface.api.bean.response.databagDerivative.DatabagDerivativeBean;
import com.bimface.api.bean.response.databagDerivative.IntegrateDatabagDerivativeBean;
import com.bimface.api.bean.response.databagDerivative.TranslateDatabagDerivativeBean;
import com.bimface.api.bean.response.extraction.ExtractionInfoBean;
import com.bimface.api.bean.response.extraction.ExtractionResponse;
import com.bimface.api.bean.response.scene.AutoUpdateSceneLayerResponse;
import com.bimface.api.bean.response.v1.FileTranslateResponseV1;
import com.bimface.bdfs.bean.*;
import com.bimface.bdfs.bean.dto.*;
import com.bimface.bdfs.bean.dto.v1.FileItemDTOV1;
import com.bimface.bdfs.bean.dto.v1.multipartupload.*;
import com.bimface.data.bean.*;
import com.bimface.data.bean.response.EssentialPipingElementResponseBean;
import com.bimface.data.bean.response.EssentialSystemResponseBean;
import com.bimface.data.enums.ToleranceType;
import com.bimface.exception.BimfaceException;
import com.bimface.file.bean.*;
import com.bimface.page.PagedList;
import com.bimface.piping.bean.EssentialPipingPathBean;
import com.bimface.piping.bean.request.EssentialPipingElementTypeAndFilterRequest;
import com.bimface.piping.bean.request.EssentialPipingElementTypeAndFiltersRequest;
import com.bimface.scene.bean.CreateSceneRequest;
import com.bimface.scene.bean.CreateSceneResp;
import com.bimface.scene.bean.response.SceneInfoResponse;
import com.bimface.sdk.bean.request.CreateFolderRequest;
import com.bimface.sdk.bean.request.FileItemQueryRequest;
import com.bimface.sdk.bean.request.*;
import com.bimface.sdk.bean.request.compare.CompareElementRequest;
import com.bimface.sdk.bean.response.FileItemUploadStatusBean;
import com.bimface.sdk.config.Config;
import com.bimface.sdk.config.Endpoint;
import com.bimface.sdk.config.authorization.AccessTokenStorage;
import com.bimface.sdk.config.authorization.Credential;
import com.bimface.sdk.config.authorization.DefaultAccessTokenStorage;
import com.bimface.sdk.constants.BimfaceConstants;
import com.bimface.sdk.service.*;
import com.bimface.sdk.utils.AssertUtils;
import com.google.gson.internal.LinkedTreeMap;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
/**
* 访问bimface服务的入口
*
* @author bimface, 2016-06-01.
*/
public class BimfaceClient {
private Credential credential; // APP证书
private Endpoint endpoint; // API调用地址入口
private Config config = null;
private AccessTokenService accessTokenService;
private FileService fileService;
private BdfsService bdfsService;
private TranslateService translateService;
private ViewTokenService viewTokenService;
private ShareLinkService shareLinkService;
private PropertyService propertyService;
private DownloadService downloadService;
private ElementService elementService;
private CategoryTreeService categoryTreeService;
private IntegrateService integrateService;
private CompareService compareService;
private OfflineDatabagService offlineDatabagService;
private ExportDatabagService exportDatabagService;
private BakeService bakeService;
private FloorService floorService;
private SignatureService signatureService;
private DataService dataService;
private DatabagService databagService;
private DrawingSplitService drawingSplitService;
private SceneService sceneService;
private ClashDetectiveService clashDetectiveService;
private ExtractionService extractionService;
/**
* 构造BimfaceClient对象
*
* @param appKey AppKey
* @param appSecret AppSecret
*/
public BimfaceClient(String appKey, String appSecret) {
this(appKey, appSecret, null, null, null);
}
/**
* 构造BimfaceClient对象
*
* @param appKey AppKey
* @param appSecret AppSecret
* @param config 参数配置
*/
public BimfaceClient(String appKey, String appSecret, Config config) {
this(appKey, appSecret, null, config, null);
}
/**
* 构造BimfaceClient对象
*
* @param appKey AppKey
* @param appSecret AppSecret
* @param endpoint 参数配置
*/
public BimfaceClient(String appKey, String appSecret, Endpoint endpoint) {
this(appKey, appSecret, endpoint, null, null);
}
/**
* 构造BimfaceClient对象
*
* @param appKey AppKey
* @param appSecret AppSecret
* @param endpoint API调用地址入口
* @param config 参数配置
*/
public BimfaceClient(String appKey, String appSecret, Endpoint endpoint, Config config) {
this(appKey, appSecret, endpoint, config, null);
}
/**
* 构造BimfaceClient对象
*
* @param appKey AppKey
* @param appSecret AppSecret
* @param endpoint API调用地址入口
* @param config 参数配置
* @param accessTokenStorage AccessToken的缓存方式
*/
public BimfaceClient(String appKey, String appSecret, Endpoint endpoint, Config config,
AccessTokenStorage accessTokenStorage) {
// 初始化APP证书
this.credential = new Credential(appKey, appSecret);
// 初始化API调用地址入口
if (endpoint == null) {
this.endpoint = new Endpoint();
} else {
this.endpoint = endpoint;
}
if (config != null) {
this.config = config;
}
// 初始化缓存AccessToken的方式
AccessTokenStorage usedAccessTokenStorage;
if (accessTokenStorage == null) {
usedAccessTokenStorage = new DefaultAccessTokenStorage();
} else {
usedAccessTokenStorage = accessTokenStorage;
}
// 初始化Service
accessTokenService = new AccessTokenService(this.endpoint, credential, this.config, usedAccessTokenStorage);
fileService = new FileService(this.endpoint, this.config, accessTokenService);
bdfsService = new BdfsService(this.endpoint, this.config, accessTokenService);
translateService = new TranslateService(this.endpoint, this.config, accessTokenService);
viewTokenService = new ViewTokenService(this.endpoint, this.config, accessTokenService);
shareLinkService = new ShareLinkService(this.endpoint, this.config, accessTokenService);
propertyService = new PropertyService(this.endpoint, this.config, accessTokenService);
downloadService = new DownloadService(this.endpoint, this.config, accessTokenService);
elementService = new ElementService(this.endpoint, this.config, accessTokenService);
categoryTreeService = new CategoryTreeService(this.endpoint, this.config, accessTokenService);
integrateService = new IntegrateService(this.endpoint, this.config, accessTokenService);
compareService = new CompareService(this.endpoint, this.config, accessTokenService);
offlineDatabagService = new OfflineDatabagService(this.endpoint, this.config, accessTokenService);
exportDatabagService = new ExportDatabagService(this.endpoint, this.config, accessTokenService);
floorService = new FloorService(this.endpoint, this.config, accessTokenService);
signatureService = new SignatureService(credential);
dataService = new DataService(this.endpoint, this.config, accessTokenService);
databagService = new DatabagService(this.endpoint, this.config, accessTokenService);
bakeService = new BakeService(this.endpoint, this.config, accessTokenService);
drawingSplitService = new DrawingSplitService(this.endpoint, this.config, accessTokenService);
sceneService = new SceneService(this.endpoint, this.config, accessTokenService);
clashDetectiveService = new ClashDetectiveService(this.endpoint, this.config, this.accessTokenService);
extractionService = new ExtractionService(this.endpoint, this.config, this.accessTokenService);
}
/**
* 获取支持的文件格式及文件大小
*
* @return 文件后缀名数组 {@link SupportFileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public SupportFileBean getSupport() throws BimfaceException {
return fileService.getSupportedFileTypes();
}
/**
* 上传文件
*
* @param fileUploadRequest {@link FileUploadRequest}
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean upload(FileUploadRequest fileUploadRequest) throws BimfaceException {
return fileService.upload(fileUploadRequest);
}
/**
* 上传文件,文件流方式
*
* @param name 文件名
* @param contentLength 文件长度
* @param inputStream 文件流
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean upload(String name, Long contentLength, InputStream inputStream) throws BimfaceException {
return upload(name, null, contentLength, inputStream);
}
/**
* 上传文件,文件流方式
*
* @param name 文件名
* @param sourceId 上传源文件Id
* @param contentLength 文件长度
* @param inputStream 文件流
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean upload(String name, String sourceId, Long contentLength,
InputStream inputStream) throws BimfaceException {
return fileService.upload(new FileUploadRequest(name, sourceId, contentLength, inputStream));
}
/**
* 上传文件,URL方式
*
* @param name 文件名
* @param url 文件下载地址
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean upload(String name, String url) throws BimfaceException {
return upload(name, null, url);
}
/**
* 上传文件,URL方式
*
* @param name 文件名
* @param sourceId 上传源文件Id
* @param url 文件下载地址
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean upload(String name, String sourceId, String url) throws BimfaceException {
return fileService.upload(new FileUploadRequest(name, sourceId, url));
}
/**
* 根据文件id获取文件元信息
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getFile(java.lang.Long) getFile(java.lang.Long)}
*
* @param fileId 文件Id
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public FileBean getFileMetadata(Long fileId) throws BimfaceException {
return fileService.getFileMetadata(fileId);
}
/**
* 根据文件id获取文件元信息
*
* @param fileId 文件Id
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean getFile(Long fileId) throws BimfaceException {
return fileService.getFile(fileId);
}
/**
* 批量查询文件元信息
*
* @param request {@link FileBatchQueryRequest}
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public List getFiles(FileBatchQueryRequest request) throws BimfaceException {
return fileService.getFiles(request);
}
/**
* 根据文件id获取文件上传状态信息
*
* @param fileId 文件Id
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileUploadStatusBean getFileUploadStatus(Long fileId) throws BimfaceException {
return fileService.getFileUploadStatus(fileId);
}
/**
* 删除文件
*
* @param fileId 文件id
* @throws BimfaceException {@link BimfaceException}
*/
public void deleteFile(Long fileId) throws BimfaceException {
fileService.deleteFile(fileId);
}
/**
* 获取上传凭证,用于直传到OSS
*
* @param name 文件名
* @return {@link UploadPolicyBean}
* @throws BimfaceException {@link BimfaceException}
*/
public UploadPolicyBean getPolicy(String name) throws BimfaceException {
return getPolicy(name, null);
}
/**
* 获取上传凭证,用于直传到OSS
*
* @param name 文件名
* @param sourceId 上传源文件Id
* @return {@link UploadPolicyBean}
* @throws BimfaceException {@link BimfaceException}
*/
public UploadPolicyBean getPolicy(String name, String sourceId) throws BimfaceException {
return fileService.getPolicy(name, sourceId);
}
/**
* 基于阿里云的policy机制,将文件直接上传阿里云 分两步:1. 调用bimface接口取得policy;2. 用policy将文件直接上传阿里云
*
* @param name 文件名
* @param contentLength 文件内容长度
* @param inputStream 文件流
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean uploadByPolicy(String name, Long contentLength, InputStream inputStream) throws BimfaceException {
return uploadByPolicy(name, null, contentLength, inputStream);
}
/**
* 基于阿里云的policy机制,将文件直接上传阿里云 分两步:1. 调用bimface接口取得policy;2. 用policy将文件直接上传阿里云
*
* @param name 文件名
* @param sourceId 上传源文件Id
* @param contentLength 文件内容长度
* @param inputStream 文件流
* @return {@link FileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileBean uploadByPolicy(String name, String sourceId, Long contentLength,
InputStream inputStream) throws BimfaceException {
return fileService.uploadByPolicy(name, sourceId, contentLength, inputStream);
}
/**
* 获取文件下载链接,直接从OSS下载
*
* @param fileId 文件ID
* @return {@link String} 文件下载链接
* @throws BimfaceException {@link BimfaceException}
*/
public String getDownloadUrl(Long fileId) throws BimfaceException {
return downloadService.getDownloadUrl(fileId);
}
/**
* 获取文件下载链接,直接从OSS下载
*
* @param fileId 文件ID
* @param fileName 文件名
* @return {@link String} 文件下载链接
* @throws BimfaceException {@link BimfaceException}
*/
public String getDownloadUrl(Long fileId, String fileName) throws BimfaceException {
return downloadService.getDownloadUrl(fileId, fileName);
}
/**
* 获取文件流
*
* @param fileId 文件id
* @param fileName 文件名
* @return {@link InputStream} 文件流
* @throws BimfaceException {@link BimfaceException}
*/
public InputStream download(Long fileId, String fileName) throws BimfaceException {
return downloadService.getFileContent(fileId, fileName);
}
/**
* 获取hub列表
*
* @param name hub名称
* @param tenantCode 租户标识
* @param info 描述信息
* @param dateTimeFrom 开始时间
* @param dateTimeTo 结束时间
* @return {@link HubDTO} 项目hub列表
* @throws BimfaceException {@link BimfaceException}
*/
public List getHubList(String name, String tenantCode, String info, String dateTimeFrom, String dateTimeTo) throws BimfaceException {
return bdfsService.getHubList(name, tenantCode, info, dateTimeFrom, dateTimeTo);
}
/**
* 获取Hub Meta信息
*
* @param hubId hubId
* @return 单个Hub
*/
public HubDTO getHubMeta(Long hubId) throws BimfaceException {
return bdfsService.getHubMeta(hubId);
}
/**
* 创建项目
*
* @param hubId hubId
* @param request 项目目创建请求
* @return {@link ProjectDTO} 项目
* @throws BimfaceException {@link BimfaceException}
*/
public ProjectDTO createProject(String hubId, ProjectCreateRequest request) throws BimfaceException {
return bdfsService.createProject(hubId, request);
}
/**
* 获取项目列表
*
* @param hubId hubId
* @param name 项目名称
* @param useFuzzySearch 是否使用模糊查询
* @return {@link ProjectDTO} 项目列表
* @throws BimfaceException {@link BimfaceException}
*/
public List getProjectList(String hubId, String name, Boolean useFuzzySearch) throws BimfaceException {
return bdfsService.getProjectList(hubId, name, useFuzzySearch);
}
/**
* 获取项目信息
*
* @param hubId hubId
* @param projectId 项目Id
* @return
*/
public ProjectDTO getProjectMeta(String hubId, String projectId) throws BimfaceException {
return bdfsService.getProjectMeta(hubId, projectId);
}
/**
* 删除项目
*
* @param hubId hubId
* @param projectId 项目Id
* @return
*/
public ProjectDTO deleteProject(String hubId, String projectId) throws BimfaceException {
return bdfsService.deleteProject(hubId, projectId);
}
/**
* 更新项目信息
*
* @param hubId hubId
* @param projectId 项目Id
* @param updateProjectReq 更新项目信息请求体
* @return
*/
public ProjectDTO updateProject(String hubId, String projectId, UpdateProjectRequest updateProjectReq) throws BimfaceException {
return bdfsService.updateProject(hubId, projectId, updateProjectReq);
}
/**
* 获取项目根文件夹信息
*
* @param hubId hubId
* @param projectId 项目Id
* @return
*/
public FileItemWithPathDTO getProjectRootFolderInfo(String hubId, String projectId) throws BimfaceException {
return bdfsService.getProjectRootFolderInfo(hubId, projectId);
}
/**
* 指定目录创建文件夹
*
* @param projectId 项目id
* @param createFolderRequest 文件夹创建请求
* @return {@link FileItemDTO} 文件项
* @throws BimfaceException {@link BimfaceException}
*/
public FileItemDTO createFolder(String projectId, CreateFolderRequest createFolderRequest) throws BimfaceException {
return bdfsService.createFolder(projectId, createFolderRequest);
}
/**
* 获取文件夹信息
*/
public FileItemDTO getFolder(String projectId, String folderId, String path) throws BimfaceException {
return bdfsService.getFolder(projectId, folderId, path);
}
/**
* 获取children列表(不包含itemSource)
*
* @param projectId 项目ID
* @param fileItemQueryRequest 请求body
* @return {@link FileItemDTO} 文件项
* @throws BimfaceException {@link BimfaceException}
*/
public PagedList getChildrenFileItems(String projectId, FileItemQueryRequest fileItemQueryRequest) throws BimfaceException {
return bdfsService.getChildrenFileItems(projectId, fileItemQueryRequest);
}
/**
* 根据folderId 获取path
*
* @param projectId 项目ID
* @param folderId 文件夹ID
* @return {@link String} 文件路径
* @throws BimfaceException {@link BimfaceException}
*/
public String getFolderPathById(String projectId, String folderId) throws BimfaceException {
return bdfsService.getFolderPathById(projectId, folderId);
}
/**
* 获取父文件夹
*
* @param projectId
* @param folderId
* @return
*/
public FileItemDTO getParent(String projectId, String folderId) throws BimfaceException {
return bdfsService.getParent(projectId, folderId);
}
/**
* 文件夹重命名
* 根据folderId(path)更新文件夹
*
* @param projectId 项目id
* @param updateFolderReq 更新文件夹请求体
* @return
*/
public FileItemDTO updateFolder(String projectId, UpdateFolderRequest updateFolderReq) throws BimfaceException {
return bdfsService.updateFolder(projectId, updateFolderReq);
}
/**
* 删除文件夹
* 根据folderId(path)删除文件夹
*
* @param projectId 项目id
* @param folderId 文件夹id(folderId和path,必须二选一填入)
* @param path 文件夹路径,使用URL编码(UTF-8),最多256个字符(folderId和path,必须二选一填入)
* @return
*/
public String deleteFolder(String projectId, String folderId, String path) throws BimfaceException {
return bdfsService.deleteFolder(projectId, folderId, path);
}
/**
* 上传文件流
*
* @param fileIn 文件流
* @param projectId 项目id
* @param parentId 父文件夹id
* @param parentPath 父文件夹路径
* @param name 文件名称
* @param length 文件大小
* @param sourceId 源文件id
* @return {@link FileItemDTO} 文件项
* @throws BimfaceException {@link BimfaceException}
*/
public FileItemDTO uploadFileItem(InputStream fileIn, String projectId, String parentId, String parentPath, String name, Long length, String sourceId) throws BimfaceException {
return bdfsService.uploadFileItem(fileIn, projectId, parentId, parentPath, name, length, sourceId);
}
/**
* 指定外部文件url方式上传
*
* @param projectId 项目ID
* @param parentId 父文件夹Id
* @param parentPath 父文件夹路径
* @param name 文件名称
* @param sourceId 调用方的文件源ID,不能重复
* @param url sourceUrl
* @param etag 文件etag
* @param maxLength maxLength
* @return
*/
public FileItemDTO uploadByUrl(String projectId, String parentId, String parentPath, String name, String sourceId, String url, String etag, Long maxLength) throws BimfaceException {
return bdfsService.uploadByUrl(projectId, parentId, parentPath, name, sourceId, url, etag, maxLength);
}
/**
* 创建追加文件
*
* @param projectId 项目ID
* @param parentId 父文件夹Id
* @param parentPath 父文件夹路径
* @param name 文件名称
* @param length 文件流的长度
* @param sourceId 调用方的文件源ID,不能重复
* @return
*/
public FileItemAppendFileDTO createAppendFile(String projectId, String parentId, String parentPath, String name, Long length, String sourceId) throws BimfaceException {
return bdfsService.createAppendFile(projectId, parentId, parentPath, name, length, sourceId);
}
/**
* 获取追加文件信息
* 获取FileItemAppendFile
*
* @param projectId 项目ID
* @param appendFileId 追加上传ID
* @return
*/
public FileItemAppendFileDTO getAppendFile(String projectId, String appendFileId) throws BimfaceException {
return bdfsService.getAppendFile(projectId, appendFileId);
}
/**
* 追加上传文件
*
* @param projectId 项目ID
* @param appendFileId 父文件夹Id
* @param position 父文件夹路径
* @return
*/
public FileItemAppendFileDTO appendUpload(InputStream fileIn, String projectId, String appendFileId, String position) throws BimfaceException {
return bdfsService.appendUpload(fileIn, projectId, appendFileId, position);
}
/**
* 复制文件
*
* @param projectId 项目ID
* @param copyFileRequest 复制文件请求体
* @return
*/
public List copyFile(String projectId, CopyFileRequest copyFileRequest) throws BimfaceException {
return bdfsService.copyFile(projectId, copyFileRequest);
}
/**
* 获取文件直传的policy凭证
* 获取上传file的policy
*
* @param projectId 项目ID
* @param parentId 父文件ID
* @param parentPath 父文件路径
* @param name 文件名称
* @param sourceId 调用方的文件源ID
* @param maxLength 文件流的长度
* @return
*/
public UploadPolicyResponse getFileItemPolicy(String projectId, String parentId, String parentPath, String name, String sourceId, Long maxLength) throws BimfaceException {
return bdfsService.getFileItemPolicy(projectId, parentId, parentPath, name, sourceId, maxLength);
}
/**
* 获取文件信息
*
* @param projectId 项目id
* @param fileItemId 文件id
* @param path 文件路径
* @param withItemSource 是否携带fileItemSource信息
* @return {@link FileItemDTO} 文件项
* @throws BimfaceException {@link BimfaceException}
*/
public FileItemDTO getFileItemMeta(String projectId, String fileItemId, String path, Boolean withItemSource) throws BimfaceException {
return bdfsService.getFileItemMeta(projectId, fileItemId, path, withItemSource);
}
/**
* 获取文件状态
*
* @param projectId 项目id
* @param fileItemId 文件id
* @param path 文件路径
* @return {@link FileItemUploadStatusBean} 文件上传状态
* @throws BimfaceException {@link BimfaceException}
*/
public FileItemUploadStatusBean getFileItemStatus(String projectId, String fileItemId, String path) throws BimfaceException {
return bdfsService.getFileItemStatus(projectId, fileItemId, path);
}
/**
* 获取文件下载签名URL
*
* @param projectId 项目id
* @param fileItemId 文件id
* @param path 文件路径
* @param expireTime 过期时间
* @return {@link String} 文件下载地址
* @throws BimfaceException {@link BimfaceException}
*/
public String getDownloadURl(String projectId, String fileItemId, String path, Integer expireTime) throws BimfaceException {
return bdfsService.getDownloadURl(projectId, fileItemId, path, expireTime);
}
/**
* 获取文件路径
* 根据fileItemId获取fileItemPath
*
* @param projectId 项目ID
* @param fileItemId 文件ID
* @return
*/
public String getFileItemPathById(String projectId, String fileItemId) throws BimfaceException {
return bdfsService.getFileItemPathById(projectId, fileItemId);
}
/**
* 打包下载压缩文件
*
* @param projectId 项目ID
* @param fileItemIds 文件ID
* @return
*/
public String downloadFiles(String projectId, List fileItemIds) throws BimfaceException {
return bdfsService.downloadFiles(projectId, fileItemIds);
}
/**
* 移动文件位置
*
* @param projectId 项目ID
* @param moveFileRequest 移动文件请求体
* @return
*/
public List moveFile(String projectId, MoveFileRequest moveFileRequest) throws BimfaceException {
return bdfsService.moveFile(projectId, moveFileRequest);
}
/**
* 文件重命名
*
* @param projectId 项目ID
* @param updateFileRequest 更新文件请求体
* @return
*/
public FileItemDTO fileRename(String projectId, UpdateFileRequest updateFileRequest) throws BimfaceException {
return bdfsService.fileRename(projectId, updateFileRequest);
}
/**
* 批量删除文件
*
* @param projectId 项目id
* @param fileItemIds 文件id集合
* @throws BimfaceException {@link BimfaceException}
*/
public void batchDeleteFileItems(String projectId, List fileItemIds) throws BimfaceException {
bdfsService.batchDeleteFileItems(projectId, fileItemIds);
}
/**
* 创建分片上传任务
*
* @param projectId 项目id
* @param initMultipartUploadRequest 初始化分片上传的请求体
* @throws BimfaceException {@link BimfaceException}
*/
public InitMultipartUploadDTO initMultipartUpload(String projectId, InitMultipartUploadRequest initMultipartUploadRequest) throws BimfaceException {
return bdfsService.initMultipartUpload(projectId, initMultipartUploadRequest);
}
/**
* 获取分片上传url
*
* @param projectId 项目 id
* @param multipartSignedUrlRequest 获取分片的 signedUrl的请求体
* @return 生成的 signed url
*/
public String getMultipartSignedUrl(String projectId, MultipartSignedUrlRequest multipartSignedUrlRequest) throws BimfaceException {
return bdfsService.getMultipartSignedUrl(projectId, multipartSignedUrlRequest);
}
/**
* 合并分片生成文件
*
* @param projectId 项目 id
* @param completeMultipartUploadRequest 完成分片上传的请求体
* @return 返回生成的 FileItem
*/
public FileItemDTOV1 completeMultiPartUpload(String projectId,
CompleteMultipartUploadRequest completeMultipartUploadRequest) throws BimfaceException {
return bdfsService.completeMultiPartUpload(projectId, completeMultipartUploadRequest);
}
/**
* 终止分片上传任务
*
* @param projectId 项目 id
* @param abortMultipartUploadRequest 终止分片上传的请求体
* @return 提示信息
*/
public String abortMultiPartUpload(String projectId,
AbortMultipartUploadRequest abortMultipartUploadRequest) throws BimfaceException {
return bdfsService.abortMultiPartUpload(projectId, abortMultipartUploadRequest);
}
public MultipartUploadSuccessPartListDTO getSuccessUploadParts(String projectId,
String id) throws BimfaceException {
return bdfsService.getSuccessUploadParts(projectId, id);
}
/**
* 获取所有版本文件信息
*
* @param projectId 项目ID
* @param fileItemId 文件Id
* @param path 文件路径
* @param pageNo 开始页码
* @param pageSize 每页大小
* @return PagedList
*/
public PagedList getAllVersions(String projectId,
String fileItemId,
String path,
Integer pageNo,
Integer pageSize) throws BimfaceException {
return bdfsService.getAllVersions(projectId, fileItemId, path, pageNo, pageSize);
}
/**
* 上传版本文件
*
* @param fileIn 文件流
* @param projectId 项目ID
* @param fileItemId 文件Id
* @param path 文件路径
* @param name 文件名称
* @param length 文件长度
* @param sourceId 调用方的文件源ID,不能重复
* @return FileItemDTO
*/
public FileItemDTO createVersion(InputStream fileIn,
String projectId,
String fileItemId,
String path,
String name,
Long length,
String sourceId) throws BimfaceException {
return bdfsService.createVersion(fileIn, projectId, fileItemId, path, name, length, sourceId);
}
/**
* 获取新版本直传的policy凭证
*
* @param projectId 项目ID
* @param fileItemId 文件Id
* @param path 文件路径
* @param name 文件名称
* @param sourceId 调用方的文件源ID,不能重复
* @param maxLength 文件流的长度
* @return UploadPolicyResponse
*/
public UploadPolicyResponse getFilePolicy(String projectId,
String fileItemId,
String path,
String name,
String sourceId,
Long maxLength) throws BimfaceException {
return bdfsService.getFilePolicy(projectId, fileItemId, path, name, sourceId, maxLength);
}
/**
* 获取指定版本文件信息
*
* @param projectId 项目ID
* @param fileId 文件版本Id
* @param withFileSource 是否携带itemSource
* @return FileItemDTO
*/
public FileItemDTO getVersion(String projectId,
String fileId,
Boolean withFileSource) throws BimfaceException {
return bdfsService.getVersion(projectId, fileId, withFileSource);
}
/**
* 下载指定版本文件
*
* @param projectId 项目ID
* @param fileId 文件版本Id
* @param expireTime 有限期,默认3600s
* @return String
*/
public String getVersionSignedUrl(String projectId,
String fileId,
Integer expireTime) throws BimfaceException {
return bdfsService.getVersionSignedUrl(projectId, fileId, expireTime);
}
/**
* 删除指定版本文件
*
* @param projectId 项目ID
* @param fileIds 文件版本Id
* @return String
*/
public String deleteVersion(String projectId,
List fileIds) throws BimfaceException {
return bdfsService.deleteVersion(projectId, fileIds);
}
/**
* 通过条件查询构件ID组
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getElementIdsV2(java.lang.Long, java.lang.String, java.lang.String,
* java.lang.String, java.lang.String, java.lang.String)
* getElementIdsV2(java.lang.Long, java.lang.String, java.lang.String,
* java.lang.String, java.lang.String, java.lang.String)}
*
* @param fileId 文件ID
* @param categoryId 构件类型ID
* @param family 族名称
* @param familyType 族类型
* @return List<{@link String}> 文件列表
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public List getElements(Long fileId, String categoryId, String family,
String familyType) throws BimfaceException {
return elementService.getElements(fileId, null, null, categoryId, family, familyType);
}
/**
* 通过条件查询构件ID组
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getElementIdsV2(java.lang.Long, java.lang.String, java.lang.String,
* java.lang.String, java.lang.String, java.lang.String)
* getElementIdsV2(java.lang.Long, java.lang.String, java.lang.String,
* java.lang.String, java.lang.String, java.lang.String)}
*
* @param fileId 文件ID
* @param floor 楼层
* @param specialty 专业
* @param categoryId 构件类型ID
* @param family 族名称
* @param familyType 族类型
* @return List<{@link String}>
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public List getElements(Long fileId, String floor, String specialty, String categoryId, String family,
String familyType) throws BimfaceException {
return elementService.getElements(fileId, floor, specialty, categoryId, family, familyType);
}
/**
* 获取集成模型的构件列表
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getIntegrateModelElementIds(java.lang.Long, java.lang.String, java.lang.String,
* java.lang.String, java.lang.String, java.lang.String, java.lang.String, java.lang.String)
* getIntegrateModelElementIds(java.lang.Long, java.lang.String, java.lang.String,
* java.lang.String, java.lang.String, java.lang.String, java.lang.String, java.lang.String)}
*
* @param integrateId 集成id
* @param floor 楼层
* @param specialty 专业
* @param categoryId 分类id
* @param family 族
* @param familyType 族类型
* @return {@link ElementsWithBoundingBox}
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public ElementsWithBoundingBox getIntegrateElements(Long integrateId, String floor, String specialty, String categoryId,
String family, String familyType) throws BimfaceException {
return elementService.getIntegrateElements(integrateId, floor, specialty, categoryId, family, familyType);
}
/**
* 发起文件转换
*
* @param fileTranslateRequest {@link FileTranslateRequest}
* @return {@link FileTranslateBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileTranslateBean translate(FileTranslateRequest fileTranslateRequest) throws BimfaceException {
return translateService.translate(fileTranslateRequest);
}
/**
* 发起文件转换
*
* @param fileId 文件id
* @return {@link FileTranslateBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileTranslateBean translate(Long fileId) throws BimfaceException {
FileTranslateRequest fileTranslateRequest = new FileTranslateRequest();
TranslateSource source = new TranslateSource();
source.setFileId(fileId);
fileTranslateRequest.setSource(source);
return translate(fileTranslateRequest);
}
/**
* 发起文件转换
*
* @param fileId 文件id
* @param callback 回调地址以http或者https开头
* @return {@link FileTranslateBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileTranslateBean translate(Long fileId, String callback) throws BimfaceException {
FileTranslateRequest fileTranslateRequest = new FileTranslateRequest();
fileTranslateRequest.setCallback(callback);
TranslateSource source = new TranslateSource();
source.setFileId(fileId);
fileTranslateRequest.setSource(source);
return translateService.translate(fileTranslateRequest);
}
/**
* 获取文件转换状态
*
* @param fileId 文件id
* @return {@link FileTranslateBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileTranslateBean getTranslate(Long fileId) throws BimfaceException {
return translateService.getTranslate(fileId);
}
/**
* 批量获取文件转换状态
*
* @param translateQueryRequest {@link TranslateQueryRequest}
* @return PagedList<{@link FileTranslateDetailBean}>
* @throws BimfaceException {@link BimfaceException}
*/
public PagedList getTranslates(TranslateQueryRequest translateQueryRequest) throws BimfaceException {
return translateService.getTranslates(translateQueryRequest);
}
/**
* 发起模型转换
*
* @param request FileTranslateRequest请求体
* @return
* @throws BimfaceException {@link BimfaceException}
*/
public FileTranslateBean translate2Bimtiles(FileTranslateRequest request) throws BimfaceException {
return translateService.translate2Bimtiles(request);
}
/**
* 获取转换资源列表
*
* @param projectId 项目ID
* @param request FileTranslateRequestV1请求体
* @return
* @throws BimfaceException {@link BimfaceException}
*/
public com.bimface.page.es.PagedList fileTranslateList(Long projectId, FileTranslateRequestV1 request) throws BimfaceException {
return translateService.fileTranslateList(projectId, request);
}
/**
* 发起模型对比
* 已过期,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#compareV2(com.bimface.api.bean.request.modelCompare.CompareRequest)
* compareV2(com.bimface.api.bean.request.modelCompare.CompareRequest)}
*
* @param modelCompareRequest {@link ModelCompareBean}
* @return {@link FileTranslateBean}
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public ModelCompareBean compare(ModelCompareRequest modelCompareRequest) throws BimfaceException {
return compareService.compare(modelCompareRequest);
}
/**
* 发起模型对比,V2
*
* @param compareRequest {@link ModelCompareBean}
* @return {@link FileTranslateBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ModelCompareBean compareV2(CompareRequest compareRequest) throws BimfaceException {
return compareService.compareV2(compareRequest);
}
/**
* 获取模型对比状态
*
* @param compareId 模型对比id
* @return {@link ModelCompareBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ModelCompareBean getCompareInfo(Long compareId) throws BimfaceException {
return compareService.getCompareInfo(compareId);
}
/**
* 批量获取模型对比状态
*
* @param modelCompareQueryRequest {@link ModelCompareQueryRequest}
* @return PagedList <{@link ModelCompareBean }>
* @throws BimfaceException {@link BimfaceException}
*/
public PagedList getCompares(ModelCompareQueryRequest modelCompareQueryRequest) throws BimfaceException {
return compareService.getCompares(modelCompareQueryRequest);
}
/**
* 删除模型对比
*
* @param compareId 模型对比id
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
public void deleteCompare(Long compareId) throws BimfaceException {
compareService.deleteCompare(compareId);
}
/**
* 获取模型对比结果
*
* @param compareId 模型对比id
* @return {@link ModelCompareTree.SpecialtyNode}
* @throws BimfaceException {@link BimfaceException}
*/
public List getCompareResult(Long compareId) throws BimfaceException {
return compareService.getCompareResult(compareId);
}
/**
* 获取模型对比构件差异
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getModelCompareElementChange(java.lang.Long, java.lang.Long, java.lang.String,
* java.lang.Long, java.lang.String)
* getModelCompareElementChange(java.lang.Long, java.lang.Long, java.lang.String, java.lang.Long, java.lang.String)}
*
* @param compareElementRequest {@link CompareElementRequest}
* @return {@link ModelCompareChange}
* @throws BimfaceException {@link BimfaceException}
*/
public ModelCompareChange getCompareElementDiff(CompareElementRequest compareElementRequest) throws BimfaceException {
return compareService.getCompareElementDiff(compareElementRequest);
}
/**
* 获取集成模型viewToken, 用于模型预览的凭证
*
* @param integrateId 集成id
* @return {@link String} viewToken 预览凭证
* @throws BimfaceException {@link BimfaceException}
*/
public String getViewTokenByIntegrateId(Long integrateId) throws BimfaceException {
return viewTokenService.getViewTokenByIntegrateId(integrateId);
}
/**
* 获取模型对比viewToken, 用于模型预览的凭证
*
* @param compareId 模型对比id
* @return {@link String} viewToken 预览凭证
* @throws BimfaceException {@link BimfaceException}
*/
public String getViewTokenByCompareId(Long compareId) throws BimfaceException {
return viewTokenService.getViewTokenByCompareId(compareId);
}
/**
* 获取viewToken, 用于模型预览的凭证
*
* @param fileId 文件id
* @return {@link String} viewToken 预览凭证
* @throws BimfaceException {@link BimfaceException}
*/
public String getViewTokenByFileId(Long fileId) throws BimfaceException {
return viewTokenService.getViewTokenByFileId(fileId);
}
/**
* 创建单个文件浏览分享链接
*
* @param fileId 文件id
* @param activeHours 从当前算起,分享链接的有效时间,单位:小时; 如果为空,表示该分享链接永久有效
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean createTranslateShare(Long fileId, Integer activeHours) throws BimfaceException {
return shareLinkService.createShare(fileId, activeHours);
}
/**
* 创建单个文件浏览分享链接,带分享密码
*
* @param fileId 文件id
* @param expireDate 过期日期
* @param needPassword 是否需要密码
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean createTranslateShare(Long fileId, String expireDate, Boolean needPassword) throws BimfaceException {
return shareLinkService.createShare(fileId, expireDate, needPassword);
}
/**
* 创建单个文件浏览分享链接,永久有效
*
* @param fileId 文件id
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean createTranslateShare(Long fileId) throws BimfaceException {
return shareLinkService.createShare(fileId);
}
/**
* 取消单个文件浏览分享链接
*
* @param fileId 文件id
* @throws BimfaceException {@link BimfaceException}
*/
public void deleteTranslateShare(Long fileId) throws BimfaceException {
shareLinkService.deleteShare(fileId);
}
/**
* 创建集成文件浏览分享链接
*
* @param integrateId 集成id
* @param activeHours 从当前算起,分享链接的有效时间,单位:小时; 如果为空,表示该分享链接永久有效
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean createIntegrateShare(Long integrateId, Integer activeHours) throws BimfaceException {
return shareLinkService.createIntegrateShare(integrateId, activeHours);
}
/**
* 创建集成文件浏览分享链接,带分享密码
*
* @param integrateId 集成id
* @param expireDate 过期日期
* @param needPassword 是否需要密码
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean createIntegrateShare(Long integrateId, String expireDate, Boolean needPassword) throws BimfaceException {
return shareLinkService.createIntegrateShare(integrateId, expireDate, needPassword);
}
/**
* 创建集成文件浏览分享链接,永久有效
*
* @param integrateId 集成id
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean createIntegrateShare(Long integrateId) throws BimfaceException {
return shareLinkService.createIntegrateShare(integrateId);
}
/**
* 取消集成文件浏览分享链接
*
* @param integrateId 集成id
* @throws BimfaceException {@link BimfaceException}
*/
public void deleteIntegrateShare(Long integrateId) throws BimfaceException {
shareLinkService.deleteIntegrateShare(integrateId);
}
/**
* 批量删除分享链接
*
* @param sourceIds 源id
* @return {@link BatchDeleteResultBean}
* @throws BimfaceException {@link BimfaceException}
*/
public BatchDeleteResultBean batchDeteleShare(List sourceIds) throws BimfaceException {
return shareLinkService.batchDeteleShare(sourceIds);
}
/**
* 获取分享链接信息
*
* @param token token
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean getShareLink(String token) throws BimfaceException {
return shareLinkService.getShareLink(token);
}
/**
* 获取分享链接信息
*
* @param fileId 文件id
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean getTranslateShare(Long fileId) throws BimfaceException {
return shareLinkService.getTranslateShare(fileId);
}
/**
* 获取分享链接信息
*
* @param integrateId 集成id
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean getIntegrateShare(Long integrateId) throws BimfaceException {
return shareLinkService.getIntegrateShare(integrateId);
}
/**
* 获取分享信息链接
*
* @param sceneId 场景id
* @return {@link ShareLinkBean}
* @throws BimfaceException {@link BimfaceException}
*/
public ShareLinkBean getSceneShare(Long sceneId) throws BimfaceException {
return shareLinkService.getSceneShare(sceneId);
}
/**
* 获取分享列表
*
* @param pageNo 分页查询的页数
* @param pageSize 分页查询的每页大小
* @return PagedList <{@link ShareLinkBean }>
* @throws BimfaceException {@link BimfaceException}
*/
public PagedList shares(Integer pageNo, Integer pageSize) throws BimfaceException {
return shareLinkService.shareList(pageNo, pageSize);
}
/**
* 获取单文件模型对应构件下的属性
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getSingleModelElementV2(java.lang.Long, java.lang.String)
* getSingleModelElementV2(java.lang.Long, java.lang.String)}
*
* @param fileId 文件id
* @param elementId 构件id
* @return PropertyBean {@link Property}
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public Property getProperty(Long fileId, String elementId) throws BimfaceException {
return propertyService.getElementProperty(fileId, elementId);
}
/**
* 获取集成模型对应构件下的属性
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getIntegrateModelElementMaterials(java.lang.Long, java.lang.String, java.lang.String)
* getIntegrateModelElementMaterials(java.lang.Long, java.lang.String, java.lang.String)}
*
* @param integrateId 集成id
* @param fileId 文件id
* @param elementId 构件id
* @return {@link Property}
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public Property getIntegrationProperty(Long integrateId, Long fileId,
String elementId) throws BimfaceException {
return propertyService.getIntegrationElementProperty(integrateId, fileId, elementId);
}
/**
* 获取单文件构件分类树
* 已过时,推荐使用V2版本文件分类数
* {@linkplain com.bimface.sdk.BimfaceClient#getSingleModelTreeV2(java.lang.Long, com.bimface.data.bean.FileTreeRequestBody)
* getSingleModelTreeV2(java.lang.Long, com.bimface.data.bean.FileTreeRequestBody)}
*
* @param fileId 文件id
* @return List<{@link Category}>
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public List getCategory(Long fileId) throws BimfaceException {
return categoryTreeService.getCategoryTree(fileId);
}
/**
* 获取单文件构件分类树V2
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getSingleModelTreeV2(java.lang.Long, com.bimface.data.bean.FileTreeRequestBody)
* getSingleModelTreeV2(java.lang.Long, com.bimface.data.bean.FileTreeRequestBody)}
*
* @param fileId 文件id
* @return List <{@link Tree.TreeNode }>
* @throws BimfaceException {@link BimfaceException} {@link com.bimface.exception.BimfaceException}
* @deprecated
*/
@Deprecated
public List getCategoryV2(Long fileId) throws BimfaceException {
return categoryTreeService.getCategoryTreeV2(fileId);
}
/**
* 获取集成模型专业层次结构
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getIntegrateModelTree(java.lang.Long, java.lang.String,
* java.util.List, com.bimface.data.bean.IntegrationTreeOptionalRequestBody)
* getIntegrateModelTree(java.lang.Long, java.lang.String, java.util.List, com.bimface.data.bean.IntegrationTreeOptionalRequestBody)}
*
* @param integrateId 集成id
* @return {@link SpecialtyTree}
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public SpecialtyTree getSpecialtyTree(Long integrateId) throws BimfaceException {
return categoryTreeService.getSpecialtyTree(integrateId);
}
/**
* 获取集成模型楼层层次结构
* 已过时,推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getIntegrateModelTree(java.lang.Long, java.lang.String,
* java.util.List, com.bimface.data.bean.IntegrationTreeOptionalRequestBody)
* getIntegrateModelTree(java.lang.Long, java.lang.String, java.util.List, com.bimface.data.bean.IntegrationTreeOptionalRequestBody)}
*
* @param integrateId 集成id
* @return {@link FloorTree}
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public FloorTree getFloorTree(Long integrateId) throws BimfaceException {
return categoryTreeService.getFloorTree(integrateId);
}
/**
* 发起文件集成
*
* @param request {@link FileIntegrateRequest}
* @return {@link FileIntegrateBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileIntegrateBean integrate(FileIntegrateRequest request) throws BimfaceException {
return integrateService.integrate(request);
}
/**
* 获取文件集成状态
*
* @param integrateId 集成Id
* @return {@link FileIntegrateBean}
* @throws BimfaceException {@link BimfaceException}
*/
public FileIntegrateBean getIntegrate(Long integrateId) throws BimfaceException {
return integrateService.getIntegrate(integrateId);
}
/**
* 批量获取文件集成状态
*
* @param integrateQueryRequest 集成文件查询条件
* @return PagedList <{@link FileIntegrateDetailBean }>
* @throws BimfaceException {@link BimfaceException}
*/
public PagedList getIntegrates(IntegrateQueryRequest integrateQueryRequest) throws BimfaceException {
return integrateService.getIntegrates(integrateQueryRequest);
}
/**
* 发起模型集成
*
* @param request 模型集成请求体
* @return
* @throws BimfaceException {@link BimfaceException}
*/
public FileIntegrateBean integrate2Bimtiles(FileIntegrateRequest request) throws BimfaceException {
return integrateService.integrate2Bimtiles(request);
}
/**
* 删除集成模型
*
* @param integrateId 集成模型id
* @throws BimfaceException {@link BimfaceException}
*/
public void deleteIntegrate(Long integrateId) throws BimfaceException {
integrateService.deleteIntegrate(integrateId);
}
/**
* 更新集成信息
*
* @param integrateId 集成模型id
* @param name 集成新名称
* @throws BimfaceException {@link BimfaceException}
*/
public FileIntegrateBean modify(Long integrateId, String name) throws BimfaceException {
return integrateService.modify(integrateId, name);
}
/**
* 验证签名, 接收回调时使用
*
* @param signature 签名字符
* @param id 可以是fileId或者integrateId
* @param status 转换状态(success || failed)
* @param nonce 随机数
* @return true: 验证成功, false: 校验失败
* @throws BimfaceException {@link BimfaceException}
*/
public boolean validateSignature(String signature, String id, String status, String nonce) throws BimfaceException {
return signatureService.validate(signature, id, status, nonce);
}
/**
* 构建离线数据包
*
* @param offlineDatabagRequest {@link OfflineDatabagRequest}
* @return {@link DatabagDerivativeBean}
* @throws BimfaceException {@link BimfaceException}
*/
public DatabagDerivativeBean generateOfflineDatabag(OfflineDatabagRequest offlineDatabagRequest) throws BimfaceException {
return offlineDatabagService.generateOfflineDatabag(offlineDatabagRequest);
}
/**
* 查询离线数据包
* 已过时:推荐使用:
* 查询转换的离线数据包
* {@linkplain com.bimface.sdk.BimfaceClient#queryTranslateOfflineDatabag(java.lang.Long) queryTranslateOfflineDatabag(java.lang.Long)}
* 查询集成的离线数据包
* {@linkplain com.bimface.sdk.BimfaceClient#queryIntegrateOfflineDatabag(java.lang.Long) queryIntegrateOfflineDatabag(java.lang.Long)}
* 查询比较的离线数据包
* {@linkplain com.bimface.sdk.BimfaceClient#queryCompareOfflineDatabag(java.lang.Long) queryCompareOfflineDatabag(java.lang.Long)}
*
* @param offlineDatabagRequest {@link OfflineDatabagRequest}
* @return List <{@link DatabagDerivativeBean }>
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public List extends DatabagDerivativeBean> queryOfflineDatabag(OfflineDatabagRequest offlineDatabagRequest) throws BimfaceException {
return offlineDatabagService.queryOfflineDatabag(offlineDatabagRequest);
}
/**
* 查询离线数据包
*
* @param fileId 文件id
* @return List <{@link DatabagDerivativeBean }>
* @throws BimfaceException {@link BimfaceException}
*/
public List extends DatabagDerivativeBean> queryTranslateOfflineDatabag(Long fileId) throws BimfaceException {
return offlineDatabagService.queryOfflineDatabag(fileId, null, null);
}
/**
* 查询离线数据包
*
* @param integrateId 集成id
* @return List <{@link DatabagDerivativeBean }>
* @throws BimfaceException {@link BimfaceException}
*/
public List extends DatabagDerivativeBean> queryIntegrateOfflineDatabag(Long integrateId) throws BimfaceException {
return offlineDatabagService.queryOfflineDatabag(null, integrateId, null);
}
/**
* 查询离线数据包
*
* @param compareId 比较id
* @return List <{@link DatabagDerivativeBean }>
* @throws BimfaceException {@link BimfaceException}
*/
public List extends DatabagDerivativeBean> queryCompareOfflineDatabag(Long compareId) throws BimfaceException {
return offlineDatabagService.queryOfflineDatabag(null, null, compareId);
}
/**
* 通过文件ID导出gltf数据包
*
* @param fileId 文件id
* @param callback 回调url地址,BIMFACE支持在创建离线数据包完成以后,通过Callback机制通知调用方
* @return TranslateDatabagDerivativeBean {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public TranslateDatabagDerivativeBean exportTranslateGltfDatabag(Long fileId, String callback) throws BimfaceException {
return exportDatabagService.exportTranslateGltfDatabag(fileId, callback);
}
/**
* 查询gltf数据包信息
*
* @param fileId 文件id
* @return List<TranslateDatabagDerivativeBean> {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public List getTranslateGltfDatabag(Long fileId) throws BimfaceException {
return exportDatabagService.getTranslateGltfDatabag(fileId);
}
/**
* 通过集成模型ID创建gltf数据包
*
* @param integrateId 集成id
* @param callback 回调url地址,BIMFACE支持在创建离线数据包完成以后,通过Callback机制通知调用方
* @return IntegrateDatabagDerivativeBean {@link IntegrateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public IntegrateDatabagDerivativeBean exportIntegrateGltfDatabag(Long integrateId, String callback) throws BimfaceException {
return exportDatabagService.exportIntegrateGltfDatabag(integrateId, callback);
}
/**
* 查询集成模型gltf数据包
*
* @param integrateId 集成id
* @return List<IntegrateDatabagDerivativeBean> {@link IntegrateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public List getIntegrateGltfDatabags(Long integrateId) throws BimfaceException {
return exportDatabagService.getIntegrateGltfDatabags(integrateId);
}
/**
* 指定文件ID,获取gltf数据包的下载链接
* 如果只有一个数据包版本,则下载唯一的数据包,如果多个,则必须指定数据包版本
* 在浏览器中输入gltf数据包的下载地址,即可下载得到对应模型的gltf数据包。
*
* @param fileId 文件id
* @param databagVersion 数据包版本
* @return gltf数据包的下载地址
* @throws BimfaceException {@link BimfaceException}
*/
public String getTranslateGltfDatabagUrl(Long fileId, String databagVersion) throws BimfaceException {
return exportDatabagService.getTranslateGltfDatabagUrl(fileId, databagVersion);
}
/**
* 指定集成ID,获取gltf数据包的下载链接
* 如果只有一个数据包版本,则下载唯一的数据包,如果多个,则必须指定数据包版本
* 在浏览器中输入gltf数据包的下载地址,即可下载得到对应模型的gltf数据包。
*
* @param integrateId 集成id
* @param databagVersion 数据包版本
* @return gltf数据包的下载地址
* @throws BimfaceException {@link BimfaceException}
*/
public String getIntegrateGltfDatabagUrl(Long integrateId, String databagVersion) throws BimfaceException {
return exportDatabagService.getIntegrateGltfDatabagUrl(integrateId, databagVersion);
}
/**
* 通过文件ID创建3DTiles数据包
* 需要文件转换成功后调用
*
* @param fileId 文件id
* @param callback 回调url,BIMFACE支持在创建离线数据包完成以后,通过Callback机制通知调用方
* @param config 参数设置
* @return TranslateDatabagDerivativeBean {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public TranslateDatabagDerivativeBean exportTranslate3DTilesDatabag(Long fileId, String callback, Map config) throws BimfaceException {
return exportDatabagService.exportTranslate3DTilesDatabag(fileId, callback, config);
}
/**
* 查询文件的3DTiles数据包
*
* @param fileId 文件id
* @return List<TranslateDatabagDerivativeBean> {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public List getTranslate3DTilesDatabags(Long fileId) throws BimfaceException {
return exportDatabagService.getTranslate3DTilesDatabags(fileId);
}
/**
* 通过集成模型ID创建3DTiles数据包
* 需要模型集成成功后调用
*
* @param integrateId 集成id
* @param callback 回调url,BIMFACE支持在创建离线数据包完成以后,通过Callback机制通知调用方
* @param config 参数设置
* @return IntegrateDatabagDerivativeBean {@link IntegrateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public IntegrateDatabagDerivativeBean exportIntegrate3DTilesDatabag(Long integrateId, String callback, Map config) throws BimfaceException {
return exportDatabagService.exportIntegrate3DTilesDatabag(integrateId, callback, config);
}
/**
* 查询集成模型3DTiles数据包
*
* @param integrateId 集成id
* @return List<IntegrateDatabagDerivativeBean> {@link IntegrateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public List getIntegrate3DTilesDatabags(Long integrateId) throws BimfaceException {
return exportDatabagService.getIntegrate3DTilesDatabags(integrateId);
}
/**
* 指定文件ID,获取3DTiles数据包下载地址
* 数据包版本;如果只有一个,则下载唯一的数据包,如果多个,则必须指定数据包版本
* 在浏览器中输入3DTiles数据包的下载地址,即可下载得到对应模型的3DTiles数据包。
*
* @param fileId 文件Id
* @param databagVersion 数据包的版本
* @return 3DTiles数据包的下载地址
* @throws BimfaceException {@link BimfaceException}
*/
public String getTranslate3dTilesDatabagUrl(Long fileId, String databagVersion) throws BimfaceException {
return exportDatabagService.getTranslate3dTilesDatabagUrl(fileId, databagVersion);
}
/**
* 指定集成ID,获取3DTiles数据包下载地址
* 数据包版本;如果只有一个,则下载唯一的数据包,如果多个,则必须指定数据包版本
* 在浏览器中输入3DTiles数据包的下载地址,即可下载得到对应模型的3DTiles数据包。
*
* @param integrateId 集成Id
* @param databagVersion 数据包版本
* @return 3DTiles数据包的下载地址
* @throws BimfaceException {@link BimfaceException}
*/
public String getIntegrate3dTilesDatabagUrl(Long integrateId, String databagVersion) throws BimfaceException {
return exportDatabagService.getIntegrate3dTilesDatabagUrl(integrateId, databagVersion);
}
/**
* 为文件创建bake数据包
* 需要文件转换成功后调用
*
* @param fileId 文件id
* @param callback 回调url,BIMFACE支持在创建离线数据包完成以后,通过Callback机制通知调用方
* @param config 参数设置
* @return TranslateDatabagDerivativeBean {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public TranslateDatabagDerivativeBean createTranslateBakeDatabag(Long fileId, String callback, Map config) throws BimfaceException {
return bakeService.createTranslateBakeDatabag(fileId, callback, config);
}
/**
* 查询文件bake数据包
*
* @param fileId 文件id
* @return List<TranslateDatabagDerivativeBean> {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public List getTranslateBakeDatabags(Long fileId) throws BimfaceException {
return bakeService.getTranslateBakeDatabags(fileId);
}
/**
* 为集成模型创建bake数据包
* 需要模型集成成功后调用
*
* @param integrateId 集成id
* @param callback 回调url,BIMFACE支持在创建离线数据包完成以后,通过Callback机制通知调用方
* @param config 参数设置
* @return IntegrateDatabagDerivativeBean {@link IntegrateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public IntegrateDatabagDerivativeBean createIntegrateBakeDatabag(Long integrateId, String callback, Map config) throws BimfaceException {
return bakeService.createIntegrateBakeDatabag(integrateId, callback, config);
}
/**
* 查询集成模型bake数据包
*
* @param integrateId 集成id
* @return List<IntegrateDatabagDerivativeBean> {@link IntegrateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public List getIntegrateBakeDatabags(Long integrateId) throws BimfaceException {
return bakeService.getIntegrateBakeDatabags(integrateId);
}
/**
* 发起图纸拆分
*
* @param fileId 文件id
* @param callback 回调url,BIMFACE支持在创建离线数据包完成以后,通过Callback机制通知调用方
* @param config 参数设置
* @return TranslateDatabagDerivativeBean {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public TranslateDatabagDerivativeBean createDrawingSplit(Long fileId, String callback, Map config) throws BimfaceException {
return drawingSplitService.createDrawingSplit(fileId, callback, config);
}
/**
* 获取拆图状态
* 图纸发起拆分后,可以通过该接口查询拆图状态
*
* @param fileId 文件id
* @return TranslateDatabagDerivativeBean {@link TranslateDatabagDerivativeBean} 数据包元信息
* @throws BimfaceException {@link BimfaceException}
*/
public TranslateDatabagDerivativeBean getDrawingSplit(Long fileId) throws BimfaceException {
return drawingSplitService.getDrawingSplit(fileId);
}
/**
* 获取图纸拆分结果
*
* @param fileId 文件id
* @return List<DrawingSplitLayout> {@link DrawingSplitLayout}
* @throws BimfaceException {@link BimfaceException}
*/
public List getDrawingFrames(Long fileId) throws BimfaceException {
List drawingFrames = drawingSplitService.getDrawingFrames(fileId);
drawingFrames.stream().forEach(drawing -> _processDrawingSplitLayout((LinkedTreeMap) drawing));
return drawingFrames;
}
private LinkedTreeMap _processDrawingSplitLayout(LinkedTreeMap map) {
Double o = (Double) map.get(BimfaceConstants.ID);
long l = o.longValue();
map.remove(BimfaceConstants.ID);
map.put(BimfaceConstants.ID, l);
List frames = (List) map.get(BimfaceConstants.FIELD_FRAMES);
if (frames != null) {
frames.stream().forEach(frame -> _processDrawingSplitLayout(frame));
}
return map;
}
/**
* 分页获取图纸对比结果
*
* @param comparisonId 图纸对比ID
* @param layer 图层名称
* @param page 页码
* @param pageSize 每页记录数
* @return Pagination <{@link DrawingCompareDiff }>
* @throws BimfaceException {@link BimfaceException}
*/
public Pagination pageGetDrawingCompareResult(Long comparisonId, String layer, Integer page, Integer pageSize) throws BimfaceException {
return dataService.pageGetDrawingCompareResult(comparisonId, layer, page, pageSize);
}
/**
* 获取MEP系统信息
*
* @param fileId 需要获取系统信息的文件Id
* @param systemCategory 希望获取的系统的systemCategory
* @param systemType 希望获取的系统的systemType
* @return List<MEPSystem> {@link MEPSystem}
* @throws BimfaceException {@link BimfaceException}
*/
public List getMEPSystem(Long fileId, String systemCategory, String systemType) throws BimfaceException {
return dataService.getMEPSystem(fileId, systemCategory, systemType);
}
/**
* 计算指定构件列表的包围盒列表
* 获取每个构件对应的包围盒
*
* @param integrateId 模型集成ID
* @param fileIdWithEleIdList 构件ID列表,由','分隔.每个构件ID由fileID和elementID组成,由'.'分隔;也可以由单个elementId组成
* @return List<ElementIdWithBoundingBox> {@link ElementIdWithBoundingBox}
* @throws BimfaceException {@link BimfaceException}
*/
public List getBoundingBoxesByEleIds(Long integrateId, List fileIdWithEleIdList) throws BimfaceException {
return dataService.getBoundingBoxes(integrateId, fileIdWithEleIdList);
}
/**
* 获取离线数据包信息
* 已过时:
* 请使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getOfflineDatabagUrl(java.lang.Long, java.lang.Long, java.lang.Long,
* java.lang.String) getOfflineDatabagUrl(java.lang.Long, java.lang.Long, java.lang.Long, java.lang.String)}
*
* @param offlineDatabagRequest {@link OfflineDatabagRequest}
* @return 离线数据包下载地址
* @throws BimfaceException {@link BimfaceException}
* @deprecated
*/
@Deprecated
public String getOfflineDatabagUrl(OfflineDatabagRequest offlineDatabagRequest) throws BimfaceException {
return offlineDatabagService.getOfflineDatabagUrl(offlineDatabagRequest);
}
/**
* 获取离线数据包下载链接
*
* @param fileId 文件id
* @param integrateId 集成id
* @param compareId 比较id
* @param databagVersion 离线数据包版本
* @return 离线数据包下载链接
* @throws BimfaceException {@link BimfaceException}
*/
public String getOfflineDatabagUrl(Long fileId, Long integrateId, Long compareId, String databagVersion) throws BimfaceException {
AssertUtils.assertTrue(fileId != null || integrateId != null || compareId != null,
"fileId, integrateId, compareId can not be null for all !");
return offlineDatabagService.getOfflineDatabagUrl(fileId, integrateId, compareId, databagVersion);
}
/**
* 创建追加文件
*
* @param name 文件的全名,使用URL编码(UTF-8),最多256个字符
* @param sourceId 调用方的文件源ID,不能重复
* @param length 上传文件长度
* @return AppendFileBean {@link AppendFileBean}
* @throws BimfaceException {@link BimfaceException}
*/
public AppendFileBean createAppendFile(String name, String sourceId, Long length) throws BimfaceException {
return fileService.createAppendFile(name, sourceId, length);
}
/**
* 查询追加文件信息
*
* @param appendFileId 追加文件ID
* @return AppendFileBean {@link AppendFileBean} 追加文件的元信息
* @throws BimfaceException {@link BimfaceException}
*/
public AppendFileBean queryAppendFile(Long appendFileId) throws BimfaceException {
return fileService.queryAppendFile(appendFileId);
}
/**
* 追加上传
*
* @param inputStream 文件的整个完整流
* @param appendFileId 追加文件ID
* @return AppendFileBean {@link AppendFileBean} 追加文件的元信息
* @throws BimfaceException {@link BimfaceException}
*/
public AppendFileBean uploadAppendFile(InputStream inputStream, Long appendFileId) throws BimfaceException {
return fileService.uploadAppendFile(inputStream, appendFileId);
}
/**
* 追加上传
*
* @param inputStream 文件的整个完整流
* @param appendFileId 追加文件ID
* @param length 上传文件长度
* @param position 追加上传开始位置
* @return AppendFileBean {@link AppendFileBean} 追加文件的元信息
* @throws BimfaceException
*/
public AppendFileBean uploadAppendFile(InputStream inputStream, Long appendFileId, Long length, Long position) throws BimfaceException {
return fileService.uploadAppendFile(inputStream, appendFileId, length, position);
}
/**
* 获取楼层信息
* 已过时:推荐使用:
* {@linkplain com.bimface.sdk.BimfaceClient#getFileFloors(java.lang.Long, Boolean, Boolean)
* getFileFloors(java.lang.Long, Boolean, Boolean)
* }
*
* @param fileId 文件Id
* @return 楼层列表
* @throws BimfaceException {@link BimfaceException} {@link BimfaceClient}
* @deprecated
*/
@Deprecated
public List getFileFloors(Long fileId) throws BimfaceException {
return getFileFloors(fileId, false, false);
}
/**
* 获取楼层信息
*
* @param fileId fileId 文件Id
* @param includeArea 是否将楼层中的面积分区ID、名称一起返回
* @param includeRoom 是否将楼层中的房间ID、名称一起返回
* @return 楼层列表
* @throws BimfaceException {@link BimfaceException}
*/
public List getFileFloors(Long fileId, Boolean includeArea, Boolean includeRoom) throws BimfaceException {
return floorService.getFileFloors(fileId, includeArea, includeRoom);
}
/**
* 获取集成模型楼层信息
* 已过时:推荐使用
* {@link com.bimface.sdk.BimfaceClient#getIntegrateFloors(java.lang.Long, java.lang.Boolean, java.lang.Boolean)
* getIntegrateFloors(java.lang.Long, java.lang.Boolean, java.lang.Boolean)
* }
*
* @param integrateId 模型集成ID
* @return 楼层列表
* @throws BimfaceException {@link BimfaceException} {@link BimfaceClient}
* @deprecated
*/
@Deprecated
public List getIntegrateFloors(Long integrateId) throws BimfaceException {
return getIntegrateFloors(integrateId, false, false);
}
/**
* 获取集成模型楼层信息
*
* @param integrateId 模型集成ID
* @param includeArea 是否将楼层中的空间ID、名称一起返回
* @param includeRoom 是否将楼层中的房间ID、名称一起返回
* @return 楼层列表
* @throws BimfaceException {@link BimfaceException}
*/
public List getIntegrateFloors(Long integrateId, Boolean includeArea, Boolean includeRoom) throws BimfaceException {
return floorService.getIntegrateFloors(integrateId, includeArea, includeRoom);
}
/**
* 按查询条件查询单模型构件ID 组,v2
* 已过时:推荐使用:
* {@link com.bimface.sdk.BimfaceClient#getElementIdsV2(java.lang.Long,
* com.bimface.sdk.bean.request.QueryElementIdsRequest)
* getElementIdsV2(java.lang.Long, com.bimface.sdk.bean.request.QueryElementIdsRequest)
* }
*
* @param fileId 文件ID
* @param specialty 专业
* @param floor 楼层
* @param categoryId 类别
* @param family 族
* @param familyType 族类型
* @return 构件 ID 列表
* @throws BimfaceException {@link BimfaceException} {@link BimfaceClient}
*/
@Deprecated
public List getElementIdsV2(Long fileId, String specialty, String floor, String categoryId,
String family, String familyType) throws BimfaceException {
QueryElementIdsRequest queryElementIdsRequest = new QueryElementIdsRequest(specialty, floor, categoryId, family,
familyType);
return getElementIdsV2(fileId, queryElementIdsRequest);
}
/**
* 按查询条件查询单模型构件ID 组,v2
*
* @param fileId 文件id
* @param queryElementIdsRequest 元素查询条件
* @return 构建id
* @throws BimfaceException {@link BimfaceException}
*/
public List getElementIdsV2(Long fileId, QueryElementIdsRequest queryElementIdsRequest) throws BimfaceException {
return elementService.getElementIdsV2(fileId, queryElementIdsRequest);
}
/**
* 获取单模型文件 id 与楼层的映射关系,v2
*
* @param fileIds 文件 IDs
* @param includeArea includeArea
* @param includeRoom includeRoom
* @return 楼层的映射关系
* @throws BimfaceException {@link BimfaceException} {@link BimfaceClient}
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy