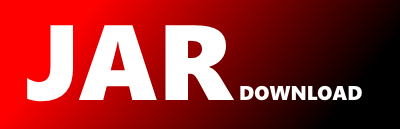
com.binance4j.market.client.MarketMapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binance4j-market Show documentation
Show all versions of binance4j-market Show documentation
Connector for the Market endpoints of the Binance API
package com.binance4j.market.client;
import java.util.List;
import java.util.Map;
import com.binance4j.core.client.RestMapping;
import com.binance4j.core.dto.AggTrade;
import com.binance4j.core.dto.Candle;
import com.binance4j.core.security.AuthenticationInterceptor;
import com.binance4j.market.dto.AveragePrice;
import com.binance4j.market.dto.BookTicker;
import com.binance4j.market.dto.ExchangeInfo;
import com.binance4j.market.dto.OrderBook;
import com.binance4j.market.dto.PriceTicker;
import com.binance4j.market.dto.ServerTimeResponse;
import com.binance4j.market.dto.TickerStatistics;
import com.binance4j.market.dto.Trade;
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Headers;
import retrofit2.http.QueryMap;
/** {@link MarketClient} mapping. */
public interface MarketMapping extends RestMapping {
/** Base uri */
String BASE = "/api/v3/";
/**
* @return The generated Retrofit call.
*/
@GET(BASE + "ping")
Call ping();
/**
* @return The generated Retrofit call.
*/
@GET(BASE + "time")
Call getServerTime();
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "exchangeInfo")
Call getExchangeInfo(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "depth")
Call getOrderBook(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "trades")
Call> getTrades(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@Headers(AuthenticationInterceptor.ENDPOINT_SECURITY_TYPE_APIKEY_HEADER)
@GET(BASE + "historicalTrades")
Call> getHistoricalTrades(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "aggTrades")
Call> getAggTrades(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "klines")
Call> getKlines(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "avgPrice")
Call getAveragePrice(@QueryMap Map map);
/**
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/24hr")
Call> get24hTickerStatistics();
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/24hr")
Call get24hTickerStatistics(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/24hr")
Call> get24hTickersStatistics(@QueryMap Map map);
/**
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/price")
Call> getTicker();
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/price")
Call> getTickers(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/price")
Call getTicker(@QueryMap Map map);
/**
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/bookTicker")
Call> getBookTicker();
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/bookTicker")
Call getBookTicker(@QueryMap Map map);
/**
* @param map The query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "ticker/bookTicker")
Call> getBookTickers(@QueryMap Map map);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy