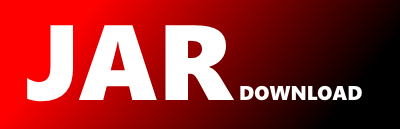
com.binance4j.savings.client.SavingsMapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binance4j-savings Show documentation
Show all versions of binance4j-savings Show documentation
Connector for the savings endpoints of the Binance API
package com.binance4j.savings.client;
import java.util.List;
import java.util.Map;
import com.binance4j.core.client.RestMapping;
import com.binance4j.savings.dto.FixedProject;
import com.binance4j.savings.dto.FixedProjectPosition;
import com.binance4j.savings.dto.FlexibleProduct;
import com.binance4j.savings.dto.FlexibleProductPosition;
import com.binance4j.savings.dto.Interest;
import com.binance4j.savings.dto.LendingAccount;
import com.binance4j.savings.dto.PositionChangedResponse;
import com.binance4j.savings.dto.Purchase;
import com.binance4j.savings.dto.PurchaseQuota;
import com.binance4j.savings.dto.PurchaseResponse;
import com.binance4j.savings.dto.Redemption;
import com.binance4j.savings.dto.RedemptionQuota;
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Headers;
import retrofit2.http.POST;
import retrofit2.http.QueryMap;
/** {@link SavingsClient} mapping. */
public interface SavingsMapping extends RestMapping {
/** The base uri. */
String BASE = "/sapi/v1/lending/";
// FLEXIBLE //
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "daily/product/list")
@Headers(SIGNED_H)
Call> getFlexibleProducts(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "daily/userLeftQuota")
@Headers(SIGNED_H)
Call getLeftDailyFlexiblePurchaseQuota(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@POST(BASE + "daily/purchase")
@Headers(SIGNED_H)
Call purchaseFlexible(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "daily/userRedemptionQuota")
@Headers(SIGNED_H)
Call getLeftDailyRedemptionQuota(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@POST(BASE + "daily/redeem")
@Headers(SIGNED_H)
Call redeemFlexible(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "daily/token/position")
@Headers(SIGNED_H)
Call> getFlexibleProductPosition(@QueryMap Map map);
// FIXED //
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "project/list")
@Headers(SIGNED_H)
Call> getFixedProjects(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@POST(BASE + "customizedFixed/purchase")
@Headers(SIGNED_H)
Call purchaseFixed(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "project/position/list")
@Headers(SIGNED_H)
Call> getFixedProjectPosition(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "union/account")
@Headers(SIGNED_H)
Call getAccount(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "union/purchaseRecord")
@Headers(SIGNED_H)
Call> getPurchases(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "union/redemptionRecord")
@Headers(SIGNED_H)
Call> getRedemptions(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@GET(BASE + "union/interestHistory")
@Headers(SIGNED_H)
Call> getInterests(@QueryMap Map map);
/**
* @param map Query map.
* @return The generated Retrofit call.
*/
@POST(BASE + "positionChanged")
@Headers(SIGNED_H)
Call fixedToDailyPosition(@QueryMap Map map);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy