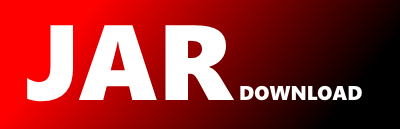
cb.parser.MyPrintVisitor Maven / Gradle / Ivy
/**
* Copyright (c) 2001 Markus Dahm
* Copyright (C) 2015-2018 BITPlan GmbH http://www.bitplan.com
*
* This source is part of
* https://github.com/BITPlan/CrazyBeans
* and the license as outlined there applies
*/
package cb.parser;
import java.io.PrintStream;
import java.util.Iterator;
import cb.petal.Association;
import cb.petal.HasQuidu;
import cb.petal.Named;
import cb.petal.PetalFile;
import cb.petal.PetalNode;
import cb.petal.PetalObject;
import cb.petal.QuidObject;
import cb.petal.Role;
/**
* (Experimental) Just prints some information about the traversed class.
*
* @version $Id: MyPrintVisitor.java,v 1.7 2001/11/01 15:56:49 dahm Exp $
* @author M. Dahm
*/
public class MyPrintVisitor extends PrintVisitor {
public MyPrintVisitor(PrintStream out) {
super(out);
}
public void visitObject(PetalObject obj) {
PetalNode node = obj.getProperty("quidu");
if(node != null) {
if(!(obj instanceof HasQuidu))
System.err.println(obj.getName() + " has quidu " + node);
} else if(obj instanceof HasQuidu) {
System.err.println(obj.getName() + " has NO quidu ");
}
super.visitObject(obj);
}
public void visit(cb.petal.Class obj) {
cb.petal.Class super_ = obj.getSuperclass();
java.util.List list = obj.getAssociations();
System.err.println(obj.getQualifiedName());
if(super_ != null) {
System.err.println("Super class of " + obj.getNameParameter() + ":" +
super_.getNameParameter());
} else if(list != null) {
System.err.print("Associations of " + obj.getNameParameter() + ":");
for(Iterator i = list.iterator(); i.hasNext(); ) {
Association a = (Association)i.next();
System.err.println(((Named)a.getFirstClient()).getNameParameter() + " <-> " +
((Named)a.getSecondClient()).getNameParameter());
cb.petal.Class clazz = a.getAssociationClass();
if(clazz != null)
System.err.println("ASSOCIATIONCLASS:" + clazz.getNameParameter());
}
}
visitObject(obj);
}
public void visit(Association obj) {
java.util.List list = obj.getRoles().getElements();
Role role1 = (Role)list.get(0);
Role role2 = (Role)list.get(1);
QuidObject class1 = obj.getFirstClient();
QuidObject class2 = obj.getSecondClient();
String name1 = role1.getNameParameter();
String name2 = role2.getNameParameter();
String card1 = role1.getCardinality();
String card2 = role2.getCardinality();
System.err.println("Association named " + obj.getNameParameter() + " between:\n" +
((Named)class1).getNameParameter() +
"(" + name1 + ":" + card1 +
":" + role1.isNavigable() + ":" + role1.isAggregate() +
")" +
"\n" +
((Named)class2).getNameParameter() +
"(" + name2 + ":" + card2 +
":" + role2.isNavigable() + ":" + role2.isAggregate() +
")"
);
visitObject(obj);
}
public static void main(String[] args) {
PetalFile tree = PetalParser.parse(args);
tree.accept(new MyPrintVisitor(System.out));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy