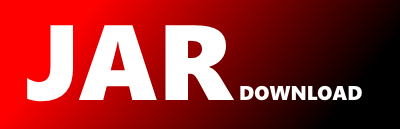
jvmMain.com.bkahlert.kommons.test.UnicodeFont.kt Maven / Gradle / Ivy
package com.bkahlert.kommons.test
import kotlin.streams.asSequence
internal enum class UnicodeFont(
private val capitalLetters: IntArray,
private val smallLetters: IntArray,
private val digits: IntArray? = null,
) {
Bold("๐".."๐", "๐".."๐ณ", "๐".."๐"),
Italic(("๐ด".."๐").toIntArray(), "๐๐๐๐๐๐๐โ๐๐๐๐๐๐๐๐๐๐๐ ๐ก๐ข๐ฃ๐ค๐ฅ๐ฆ๐ง".codePoints),
BoldItalic("๐จ".."๐", "๐".."๐"),
Script("๐โฌ๐๐โฐโฑ๐ขโโ๐ฅ๐ฆโโณ๐ฉ๐ช๐ซ๐ฌโ๐ฎ๐ฏ๐ฐ๐ฑ๐ฒ๐ณ๐ด๐ต".codePoints, "๐ถ๐ท๐ธ๐นโฏ๐ปโ๐ฝ๐พ๐ฟ๐๐๐๐โด๐
๐๐๐๐๐๐๐๐๐๐".codePoints),
BoldScript("๐".."๐ฉ", "๐ช".."๐"),
Fraktur("๐๐
โญ๐๐๐๐โโ๐๐๐๐๐๐๐๐โ๐๐๐๐๐๐๐โจ".codePoints, ("๐".."๐ท").toIntArray()),
BoldFraktur("๐ฌ".."๐
", "๐".."๐"),
DoubleStruck("๐ธ๐นโ๐ป๐ผ๐ฝ๐พโ๐๐๐๐๐โ๐โโโ๐๐๐๐๐๐๐โค".codePoints, ("๐".."๐ซ").toIntArray(), ("๐".."๐ก").toIntArray()),
SansSerif("๐ ".."๐น", "๐บ".."๐", "๐ข".."๐ซ"),
SansSerifBold("๐".."๐ญ", "๐ฎ".."๐", "๐ฌ".."๐ต"),
SansSerifItalic("๐".."๐ก", "๐ข".."๐ป"),
SansSerifBoldItalic("๐ผ".."๐", "๐".."๐ฏ"),
Monospace("๐ฐ".."๐", "๐".."๐ฃ", "๐ถ".."๐ฟ"),
;
init {
check(capitalLetters.size == 26) { "26 capital letters needed" }
check(smallLetters.size == 26) { "26 small letters needed" }
digits?.also { check(it.size == 10) { "either no or 10 digits needed" } }
}
constructor(capitalLetters: IntRange, smallLetters: IntRange, digits: IntRange? = null) : this(
capitalLetters.toIntArray(), smallLetters.toIntArray(), digits?.toIntArray(),
)
fun convertCodePointOrNull(codePoint: Int): Int? =
capitalLetters.getOrNull(codePoint - capitalLetterACodePoint)
?: smallLetters.getOrNull(codePoint - smallLetterACodePoint)
?: digits?.getOrNull(codePoint - digitZeroCodePoint)
fun format(text: String, onFailure: (codePoint: Int) -> Int = { it }): String {
return buildString {
text.codePoints().forEach {
val codePoint = convertCodePointOrNull(it) ?: onFailure(it)
appendCodePoint(codePoint)
}
}
}
companion object {
const val capitalLetterACodePoint = 'A'.code
const val smallLetterACodePoint = 'a'.code
const val digitZeroCodePoint = '0'.code
}
}
private val String.codePoints: IntArray get() = codePoints().asSequence().toList().toIntArray()
private val String.codePoint: Int get() = codePoints.single()
private operator fun String.rangeTo(other: String): IntRange = codePoint..other.codePoint
private fun IntRange.toIntArray(): IntArray = toList().toIntArray()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy