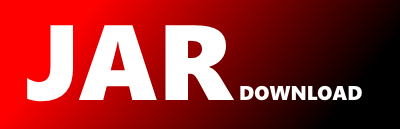
com.blackbirdai.client.model.Condition Maven / Gradle / Ivy
package com.blackbirdai.client.model;
import com.blackbirdai.client.InvalidRequestException;
import com.blackbirdai.client.util.Patterns;
import javax.annotation.Nullable;
/**
* Boolean condition for filter parameters.
*/
public abstract class Condition extends Param {
/**
* Returns Equals condition: $field == 'value'.
* @param field Name of the field.
* @param value Value being compared to in string format.
*/
public static Condition eq(String field, Value value) {
return new EqualsCondition(field, value);
}
/**
* Returns Not-Equals condition: $field != 'value'.
* @param field Name of the field.
* @param value Value being compared to in string format.
*/
public static Condition ne(String field, Value value) {
return new NotEqualsCondition(field, value);
}
/**
* Returns Greater-Than condition: 'lower-bound' < $field
* @param field Name of the field.
* @param lowerBound Lower-bound in string format.
*/
public static Condition gt(String field, Value lowerBound) {
return new RangeCondition(field, lowerBound, null, false, true);
}
/**
* Returns Greater-than-or-Equals-to condition: 'lower-bound' <= $field
* @param field Name of the field.
* @param lowerBound Lower-bound in string format.
*/
public static Condition ge(String field, Value lowerBound) {
return new RangeCondition(field, lowerBound, null, true, true);
}
/**
* Returns Less-Than condition: $field < 'upper-bound'
* @param field Name of the field.
* @param upperBound Upper-bound in string format.
*/
public static Condition lt(String field, Value upperBound) {
return new RangeCondition(field, null, upperBound, true, false);
}
/**
* Returns Less-than-or-Equals-to condition: $field <= 'upper-bound'
* @param field Name of the field.
* @param upperBound Upper-bound in string format.
*/
public static Condition le(String field, Value upperBound) {
return new RangeCondition(field, null, upperBound, true, true);
}
/**
* Returns Range condition: 'lower-bound' < $field < 'upper-bound'
* @param field Name of the field.
* @param lowerBound Lower-bound in string format. If null, there is no lower-bound condition.
* @param upperBound Upper-bound in string format. If null, there is no upper-bound condition.
* @param lowerBoundInclusive If true, lower-bound condition is inclusive.
* @param upperBoundInclusive If true, upper-bound condition is inclusive.
*/
public static Condition range(
String field, @Nullable Value lowerBound, @Nullable Value upperBound,
boolean lowerBoundInclusive, boolean upperBoundInclusive) {
return new RangeCondition(field, lowerBound, upperBound, lowerBoundInclusive, upperBoundInclusive);
}
}
/**
* Equals condition on a field.
*/
class EqualsCondition extends Condition {
private final String field;
private final Value value;
/**
* Internal use only. Construct instances using {@link Condition}'s static methods.
*/
EqualsCondition(String field, Value value) {
this.field = field;
this.value = value;
}
@Override
StringBuilder appendQueryStr(StringBuilder builder) throws InvalidRequestException {
if (!Patterns.FIELD_P.matcher(field).matches()) {
throw new InvalidRequestException("Invalid field-name found: " + field);
}
builder.append(field);
builder.append(":");
builder.append(value.toQueryStr());
return builder;
}
}
/**
* Not-equals condition on a field.
*/
class NotEqualsCondition extends Condition {
private final String field;
private final Value value;
/**
* Internal use only. Construct instances using {@link Condition}'s static methods.
*/
NotEqualsCondition(String field, Value value) {
this.field = field;
this.value = value;
}
@Override
StringBuilder appendQueryStr(StringBuilder builder) throws InvalidRequestException {
if (!Patterns.FIELD_P.matcher(field).matches()) {
throw new InvalidRequestException("Invalid field-name found: " + field);
}
builder.append(field);
builder.append(":!");
builder.append(value.toQueryStr());
return builder;
}
}
/**
* Range condition on a comparable field.
*/
class RangeCondition extends Condition {
private final String field;
private final Value lowerBound;
private final Value upperBound;
private final boolean lowerBoundInclusive;
private final boolean upperBoundInclusive;
/**
* Internal use only. Construct instances using {@link Condition}'s static methods.
*/
RangeCondition(String field, @Nullable Value lowerBound, @Nullable Value upperBound,
boolean lowerBoundInclusive, boolean upperBoundInclusive) {
this.field = field;
this.lowerBound = lowerBound;
this.upperBound = upperBound;
this.lowerBoundInclusive = lowerBoundInclusive;
this.upperBoundInclusive = upperBoundInclusive;
}
@Override
StringBuilder appendQueryStr(StringBuilder builder) throws InvalidRequestException {
if (!Patterns.FIELD_P.matcher(field).matches()) {
throw new InvalidRequestException("Invalid field-name found: " + field);
}
builder.append(field);
builder.append(':');
builder.append(lowerBoundInclusive ? '[' : '(');
if (lowerBound != null) {
builder.append(lowerBound.toQueryStr());
}
builder.append(':');
if (upperBound != null) {
builder.append(upperBound.toQueryStr());
}
builder.append(upperBoundInclusive ? ']' : ')');
return builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy