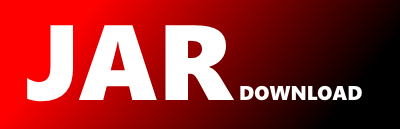
com.blackbirdai.client.model.DeleteRequest Maven / Gradle / Ivy
package com.blackbirdai.client.model;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.collect.Lists;
import java.util.List;
/**
* Container for the parameters of a delete request.
*/
public class DeleteRequest extends UploadRequest {
/**
* Constructor for DeleteRequest.
*/
public DeleteRequest(String subject) {
super(subject);
}
/**
* Default empty constructor for DeleteRequest.
*/
public DeleteRequest() {
this("products");
}
/**
* Update ids to be deleted.
*
* @return Updated request object.
*/
public DeleteRequest withIds(List ids) {
this.docs = Lists.newArrayListWithCapacity(ids.size());
for (String id : ids) {
ObjectNode json = new ObjectNode(JsonNodeFactory.instance);
json.put("id", id);
super.docs.add(json);
}
return this;
}
/**
* Append ids to be deleted.
*
* @return Updated request object.
*/
public DeleteRequest addIds(List ids) {
if (this.docs == null) {
this.docs = Lists.newArrayListWithCapacity(ids.size());
}
for (String id : ids) {
ObjectNode json = new ObjectNode(JsonNodeFactory.instance);
json.put("id", id);
this.docs.add(json);
}
return this;
}
/**
* Append an id to be deleted.
*
* @return Updated request object.
*/
public DeleteRequest addId(String id) {
if (this.docs == null) {
this.docs = Lists.newArrayList();
}
ObjectNode json = new ObjectNode(JsonNodeFactory.instance);
json.put("id", id);
this.docs.add(json);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy