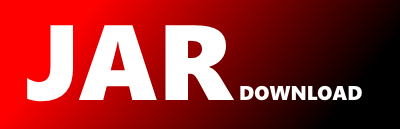
com.blackbirdai.client.model.Document Maven / Gradle / Ivy
package com.blackbirdai.client.model;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectReader;
import com.fasterxml.jackson.databind.ObjectWriter;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.base.Function;
import com.google.common.collect.Maps;
import javax.annotation.Nullable;
import java.util.Map;
/**
* Container for a document.
*/
public class Document {
static final ObjectMapper Mapper = new ObjectMapper();
static final ObjectReader Reader = Mapper.reader(JsonNode.class);
static final ObjectWriter Writer = Mapper.writer();
final JsonNode underlying;
Document(JsonNode json) {
this.underlying = json;
}
public Document(Map values) {
Map jsonMap = Maps.transformValues(values, new Function() {
public JsonNode apply(Value v) {
return v.underlying;
}
});
this.underlying = new ObjectNode(JsonNodeFactory.instance, jsonMap);
}
/**
* Returns {@link Value} of a field. If field is missing, returns null.
*/
@Nullable
public Value get(String field) {
if (underlying.get(field) == null) {
return null;
}
return new Value(underlying.get(field));
}
/**
* Returns document in raw JSON format.
*/
public String asRawJsonString() {
try {
return Writer.writeValueAsString(underlying);
} catch (JsonProcessingException e) {
return ""; // This should never happen though.
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy