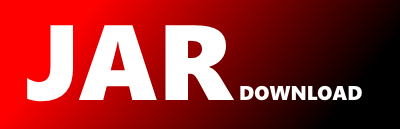
com.blackbirdai.client.model.Facets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of search-client Show documentation
Show all versions of search-client Show documentation
Java client for Blackbird search API
The newest version!
package com.blackbirdai.client.model;
import com.fasterxml.jackson.databind.JsonNode;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.base.Predicates;
import com.google.common.collect.Iterators;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* Container for facet response.
*/
public class Facets {
private final Map> enumFacets;
private final Map> histogramFacets;
private final Map rangeFacets;
/**
* Internal use only.
*/
Facets(JsonNode jsonNode) {
JsonNode enums = jsonNode.get("enums");
if (enums != null && enums.isObject() && enums.size() > 0) {
this.enumFacets = Maps.newHashMap();
Iterator> it = enums.fields();
while (it.hasNext()) {
Map.Entry entry = it.next();
JsonNode facetsJson = entry.getValue().get("enums");
if (facetsJson != null && facetsJson.isArray()) {
Iterator transformed = Iterators.transform(
facetsJson.elements(),
new Function() {
public EnumFacet apply(JsonNode node) {
return parseEnumJson(node).orNull();
}
}
);
Iterator filtered = Iterators.filter(transformed, Predicates.notNull());
this.enumFacets.put(entry.getKey(), Lists.newArrayList(filtered));
}
}
} else {
this.enumFacets = Collections.EMPTY_MAP;
}
JsonNode histograms = jsonNode.get("histograms");
if (histograms != null && histograms.isObject() && histograms.size() > 0) {
this.histogramFacets = Maps.newHashMap();
Iterator> it = histograms.fields();
while (it.hasNext()) {
Map.Entry entry = it.next();
JsonNode facetsJson = entry.getValue().get("histograms");
if (facetsJson != null && facetsJson.isArray()) {
Iterator transformed = Iterators.transform(
facetsJson.elements(),
new Function() {
public HistogramFacet apply(JsonNode node) {
return parseHistogramJson(node).orNull();
}
}
);
Iterator filtered = Iterators.filter(transformed, Predicates.notNull());
this.histogramFacets.put(entry.getKey(), Lists.newArrayList(filtered));
}
}
} else {
this.histogramFacets = Collections.EMPTY_MAP;
}
JsonNode ranges = jsonNode.get("ranges");
if (ranges != null && ranges.isObject() && ranges.size() > 0) {
this.rangeFacets = Maps.newHashMap();
Iterator> it = ranges.fields();
while (it.hasNext()) {
Map.Entry entry = it.next();
Optional parsed = parseRangeJson(entry.getValue());
if (parsed.isPresent()) {
this.rangeFacets.put(entry.getKey(), parsed.get());
}
}
} else {
this.rangeFacets = Collections.EMPTY_MAP;
}
}
/**
* Returns enum-facets for 'field'. Returns null if 'field' is missing.
*/
public List getEnumFacets(String field) {
return enumFacets.get(field);
}
/**
* Returns histogram-facets for 'field'. Returns null if 'field' is missing.
*/
public List getHistogramFacets(String field) {
return histogramFacets.get(field);
}
/**
* Returns a range-facet for 'field'. Returns null if 'field' is missing.
*/
public RangeFacet getRangeFacet(String field) {
return rangeFacets.get(field);
}
private Optional parseEnumJson(JsonNode json) {
if (!json.isObject() || json.get("term") == null || json.get("count") == null
|| !json.get("count").isIntegralNumber()) {
return Optional.absent();
}
return Optional.of(new EnumFacet(json.get("term").asText(), json.get("count").asLong()));
}
private Optional parseHistogramJson(JsonNode json) {
if (!json.isObject() || json.get("from") == null || json.get("to") == null || json.get("count") == null
|| !json.get("count").isIntegralNumber()) {
return Optional.absent();
}
Number from = (json.get("from").isNumber() ? json.get("from").numberValue() : json.get("from").asDouble());
Number to = (json.get("to").isNumber() ? json.get("to").numberValue() : json.get("to").asDouble());
return Optional.of(new HistogramFacet(from, to, json.get("count").asLong()));
}
private Optional parseRangeJson(JsonNode json) {
if (!json.isObject() || json.get("min") == null || json.get("max") == null
|| !json.get("min").isNumber() || !json.get("max").isNumber()) {
return Optional.absent();
}
return Optional.of(new RangeFacet(json.get("min").numberValue(), json.get("max").numberValue()));
}
public class EnumFacet {
private final String term;
private final long count;
public EnumFacet(String term, long count) {
this.term = term;
this.count = count;
}
public String getTerm() {
return term;
}
public long getCount() {
return count;
}
}
public class HistogramFacet {
private final Number from;
private final Number to;
private final long count;
public HistogramFacet(Number from, Number to, long count) {
this.from = from;
this.to = to;
this.count = count;
}
public Number getFrom() {
return from;
}
public Number getTo() {
return to;
}
public long getCount() {
return count;
}
}
public class RangeFacet {
private final Number min;
private final Number max;
public RangeFacet(Number min, Number max) {
this.min = min;
this.max = max;
}
public Number getMax() {
return max;
}
public Number getMin() {
return min;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy