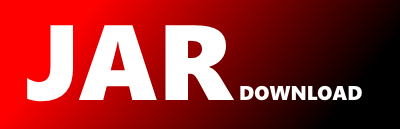
com.blackbirdai.client.model.MSearchRequest Maven / Gradle / Ivy
package com.blackbirdai.client.model;
import com.blackbirdai.client.InvalidRequestException;
import com.blackbirdai.client.util.Encoders;
import com.blackbirdai.client.util.Joiners;
import com.blackbirdai.client.util.Patterns;
import com.google.common.collect.Lists;
import java.util.List;
public class MSearchRequest {
private List qcs = Lists.newArrayList();
private Integer start = null;
private Integer num = null;
private List fields = null;
private List sortParams = null;
private List facetParams = null;
private String sId = null;
private String uId = null;
/**
* Default empty constructor for MSearchRequest.
*/
public MSearchRequest() {}
/**
* Update 'qc' parameters.
*
* @return A reference to this updated object.
*/
public MSearchRequest withQCs(List qcs) {
this.qcs = qcs;
return this;
}
/**
* Append a 'qc' parameter.
*
* @return A reference to this updated object.
*/
public MSearchRequest addQC(QueryComponent qc) {
if (this.qcs == null) {
this.qcs = Lists.newArrayList();
}
this.qcs.add(qc);
return this;
}
/**
* Update 'start' parameter.
*
* @return A reference to this updated object.
*/
public MSearchRequest withStart(int start) {
this.start = start;
return this;
}
/**
* Update 'num' parameter.
*
* @return A reference to this updated object.
*/
public MSearchRequest withNum(int num) {
this.num = num;
return this;
}
/**
* Update 'fields' parameters.
*
* @return A reference to this updated object.
*/
public MSearchRequest withFields(List fields) {
this.fields = fields;
return this;
}
/**
* Append a 'fields' parameter.
*
* @return A reference to this updated object.
*/
public MSearchRequest addField(String field) {
if (this.fields == null) {
this.fields = Lists.newArrayList();
}
this.fields.add(field);
return this;
}
/**
* Update 'sort' parameters.
*
* @param sortParams List of sort parameters. Results are sorted by first parameter and then second parameter and so on.
* @return A reference to this updated object.
*/
public MSearchRequest withSort(List sortParams) {
this.sortParams = sortParams;
return this;
}
/**
* Append a 'sort' parameter.
*
* @return A reference to this updated object.
*/
public MSearchRequest addSort(SortParam sortParam) {
if (this.sortParams == null) {
this.sortParams = Lists.newArrayList();
}
this.sortParams.add(sortParam);
return this;
}
/**
* Update 'facet' parameterss.
*
* @return A reference to this updated object.
*/
public MSearchRequest withFacets(List facetParams) {
this.facetParams = facetParams;
return this;
}
/**
* Append a 'facet' parameter.
*
* @return A reference to this updated object.
*/
public MSearchRequest addFacet(FacetParam facetParam) {
if (this.facetParams == null) {
this.facetParams = Lists.newArrayList();
}
this.facetParams.add(facetParam);
return this;
}
/**
* Update 'sid' parameter (session-id).
*
* @return Updated object.
*/
public MSearchRequest withSId(String sId) {
this.sId = sId;
return this;
}
/**
* Update 'uid' parameter (user-id).
*
* @return Updated object.
*/
public MSearchRequest withUId(String uId) {
this.uId = uId;
return this;
}
/**
* For internal use. Returns query-string for http request.
*/
public String toQueryStr(String instanceId, String subject) throws InvalidRequestException {
if (qcs.isEmpty()) {
throw new InvalidRequestException("");
}
StringBuilder builder = new StringBuilder("/v1/" + instanceId + "/" + subject + "/msearch?");
boolean ampersand = false;
for (QueryComponent qc: qcs) {
if (ampersand) builder.append('&');
ampersand = true;
qc.appendQueryStr(builder);
}
if (start != null) {
builder.append("&start=");
builder.append(start);
}
if (num != null) {
builder.append("&num=");
builder.append(num);
}
if (fields != null) {
for (String field: fields) {
if (!Patterns.FIELD_P.matcher(field).matches()) {
throw new InvalidRequestException("Invalid field-name found: " + field);
}
}
builder.append("&fields=");
Joiners.COMMA_JOINER.appendTo(builder, fields);
}
if (sortParams != null) {
builder.append("&sort=");
boolean comma = false;
for (SortParam sortParam: sortParams) {
if (comma) builder.append(',');
sortParam.appendQueryStr(builder);
comma = true;
}
}
if (facetParams != null) {
for (FacetParam facetParam : facetParams) {
builder.append('&');
facetParam.appendQueryStr(builder);
}
}
if (sId != null) {
builder.append("&sid=");
builder.append(Encoders.urlEncode(sId));
}
if (uId != null) {
builder.append("&uid=");
builder.append(Encoders.urlEncode(uId));
}
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy