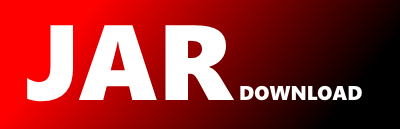
com.blackbirdai.client.model.Response Maven / Gradle / Ivy
package com.blackbirdai.client.model;
import com.fasterxml.jackson.databind.JsonNode;
import com.google.common.base.Charsets;
import org.jboss.netty.handler.codec.http.HttpResponse;
/**
* Container for responses.
*/
public abstract class Response {
private final boolean success;
private final int statusCode;
private final String message;
private final String rawJson;
protected Response(boolean success, int statusCode, String message, String rawJson) {
this.success = success;
this.statusCode = statusCode;
this.message = message;
this.rawJson = rawJson;
}
protected Response(boolean success, int statusCode, String message) {
this(success, statusCode, message, "");
}
protected Response(HttpResponse httpResponse) {
int code = httpResponse.getStatus().getCode();
Boolean success = (code == 0 || code == 200);
String message = "";
String rawJson = "";
try {
if (success) {
rawJson = httpResponse.getContent().toString(Charsets.UTF_8);
JsonNode jsonNode = Document.Reader.readValue(rawJson);
message = jsonNode.get("msg").toString();
success = jsonNode.get("success").asBoolean(success);
} else {
message = "Request failed with HTTP status " + httpResponse.getStatus();
rawJson = httpResponse.getContent().toString(Charsets.UTF_8);
JsonNode jsonNode = Document.Reader.readValue(rawJson);
message = message + ": " + jsonNode.get("msg").toString();
}
} catch (Exception e) { /* invalid response body */ }
this.success = success;
this.statusCode = code;
this.message = message;
this.rawJson = rawJson;
}
/**
* Returns true, if request was successful.
*/
public boolean getSuccess() {
return success;
}
/**
* Returns HTTP status-code for request. Returns -1, if not available.
*/
public int getStatusCode() {
return statusCode;
}
/**
* Returns additional information about the response.
*/
public String getMessage() {
return message;
}
/**
* Returns result in raw json format. Same as the original HTTP response.
*/
public String getRawJson() { return rawJson; }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy