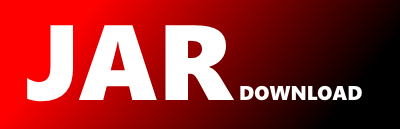
com.blackbirdai.client.model.SearchResponse Maven / Gradle / Ivy
package com.blackbirdai.client.model;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.base.Charsets;
import org.jboss.netty.handler.codec.http.HttpResponse;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
/**
* Container for the output of a search request.
*/
public class SearchResponse extends Response {
private static final Facets EMPTY_FACETS = new Facets(new ObjectNode(JsonNodeFactory.instance));
private final int numFound;
private final List hits;
private final Facets facets;
private final Correction correction;
private final String queryId;
public static SearchResponse build(HttpResponse httpResponse) {
int statusCode = httpResponse.getStatus().getCode();
if (statusCode != 0 && statusCode != 200) {
// Generate error response
return new SearchResponse(httpResponse);
}
final String rawJson;
final JsonNode jsonNode;
try {
rawJson = httpResponse.getContent().toString(Charsets.UTF_8);
jsonNode = Document.Reader.readValue(rawJson);
} catch (Exception e) {
return new SearchResponse(false, statusCode, "Error in response: invalid JSON format");
}
JsonNode results = jsonNode.get("results");
if (results == null || results.get("numfound") == null || results.get("hits") == null) {
return new SearchResponse(false, statusCode, "Error in response: invalid format", rawJson);
}
int numFound = results.get("numfound").asInt();
List hits = new ArrayList(results.get("hits").size());
Iterator it = results.get("hits").elements();
while (it.hasNext()) {
hits.add(new Document(it.next()));
}
final Facets facets;
if (results.get("facets") != null) {
facets = new Facets(results.get("facets"));
} else {
facets = EMPTY_FACETS;
}
final Correction correction;
if (results.get("cq") != null) {
correction = Correction.parseJson(results.get("cq"));
} else {
correction = null;
}
final String queryId;
if (jsonNode.get("qId") != null) {
queryId = jsonNode.get("qId").asText();
} else if (jsonNode.get("qid") != null) {
queryId = jsonNode.get("qid").asText();
} else {
queryId = null;
}
return new SearchResponse(true, statusCode, "", rawJson, numFound, hits, facets, correction, queryId);
}
public SearchResponse(boolean success, int statusCode, String message, String rawJson, int numFound,
List hits, Facets facets, Correction correction, String queryId) {
super(success, statusCode, message, rawJson);
this.numFound = numFound;
this.hits = hits;
this.facets = facets;
this.correction = correction;
this.queryId = queryId;
}
public SearchResponse(boolean success, int statusCode, String message, String rawJson) {
this(success, statusCode, message, rawJson, 0, Collections.EMPTY_LIST, EMPTY_FACETS, null, null);
}
public SearchResponse(boolean success, int statusCode, String message) {
this(success, statusCode, message, "");
}
public SearchResponse(boolean success, String message) {
this(success, -1, message);
}
// Private constructor for error responses
private SearchResponse(HttpResponse httpResponse) {
super(httpResponse);
this.numFound = 0;
this.hits = Collections.EMPTY_LIST;
this.facets = EMPTY_FACETS;
this.correction = null;
this.queryId = null;
}
/**
* Returns the exact number of matching indexed documents.
*/
public int getNumFound() { return numFound; }
/**
* Returns a list of matching documents. Number of documents may be smaller than 'numfound'.
*/
public List getHits() { return hits; }
/**
* Returns requested facets from this search query.
*/
public Facets getFacets() {
return facets;
}
/**
* Returns query-correction and level of confidence for the correction. If there is no correction, returns null.
*/
public Correction getCorrection() {
return correction;
}
/**
* Returns an true, if query was corrected.
*/
public boolean hasCorrection() {
return correction != null;
}
/**
* Returns an ID to feedback user activities following this search query. In case of errors, returns null.
*/
public String getQueryId() { return queryId; }
/**
* Returns an true, if query-id was provided by the search query.
*/
public boolean hasQueryId() {
return queryId != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy