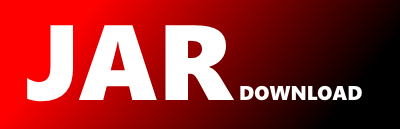
com.blackbirdai.client.model.Value Maven / Gradle / Ivy
package com.blackbirdai.client.model;
import com.blackbirdai.client.InvalidRequestException;
import com.blackbirdai.client.util.Encoders;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
/**
* Container for {@link Document} values.
*/
public class Value {
final JsonNode underlying;
/**
* Constructor for string value.
*/
public Value(String value) {
this.underlying = new TextNode(value);
}
/**
* Constructor for int value.
*/
public Value(int value) {
this.underlying = new IntNode(value);
}
/**
* Constructor for long value.
*/
public Value(long value) {
this.underlying = new LongNode(value);
}
/**
* Constructor for float value.
*/
public Value(float value) {
this.underlying = new FloatNode(value);
}
/**
* Constructor for double value.
*/
public Value(double value) {
this.underlying = new DoubleNode(value);
}
/**
* Constructor for boolean value.
*/
public Value(boolean value) {
this.underlying = BooleanNode.valueOf(value);
}
/**
* Constructor for string-list value.
*/
public Value(List values) {
ArrayNode jsonNode = new ArrayNode(JsonNodeFactory.instance);
for (String v : values) {
jsonNode.add(v);
}
this.underlying = jsonNode;
}
Value (JsonNode jsonNode) {
this.underlying = jsonNode;
}
/**
* Returns value as a Java int. Floating-point numbers are truncated. Booleans are converted to 0 and 1.
* Strings are parsed to integers, if possible. Otherwise, returns 0.
*/
public int asInt() {
return underlying.asInt();
}
/**
* Returns value as a Java long. Floating-point numbers are truncated. Booleans are converted to 0 and 1.
* Strings are parsed, if possible. Otherwise, returns 0.
*/
public long asLong() {
return underlying.asLong();
}
/**
* Returns value as a Java float. Booleans are converted to 0.0 and 1.0. Strings are parsed, if possible.
* Otherwise, returns 0.
*/
public float asFloat() {
return (float) underlying.asDouble();
}
/**
* Returns value as a Java double. Booleans are converted to 0.0 and 1.0. Strings are parsed, if possible.
* Otherwise, returns 0.
*/
public double asDouble() {
return underlying.asDouble();
}
/**
* Returns value as {@link Number}. If not numeric, returns null.
*/
public Number asNumber() {
return underlying.numberValue();
}
/**
* Returns value as a Java boolean. 0 is converted to false and other numeric values are converted to true.
* Strings are parsed, if possible. Otherwise, returns false.
*/
public boolean asBoolean() {
return underlying.asBoolean();
}
/**
* Returns value as {@link String}. Numeric an boolean values are converted to string values.
* For arrays and objects, returns empty string.
*/
public String asString() {
return underlying.asText();
}
/**
* Returns value as {@link List} of {@link String}. For non-array values, returns an empty list.
* Each value of the array is converted to a string value using the same rule as {@link Value#asString()}.
*/
public List asStringList(String field) {
if (!underlying.isArray() || underlying.size() <= 0) {
return Collections.EMPTY_LIST;
}
List rv = new ArrayList(underlying.size());
Iterator it = underlying.elements();
while (it.hasNext()) {
rv.add(it.next().asText());
}
return rv;
}
/**
* Returns value as raw Json string.
*/
public String asRawJsonString() {
try {
return Document.Writer.writeValueAsString(underlying);
} catch (JsonProcessingException e) {
return ""; // This should never happen though.
}
}
public String toQueryStr() throws InvalidRequestException {
String queryStr = this.underlying.asText();
if (this.underlying != null && this.underlying.isTextual()) {
return Encoders.urlEncode(queryStr);
} else {
return queryStr;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy