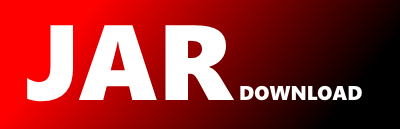
com.blacksquircle.ui.language.smali.lexer.SmaliLexer Maven / Gradle / Ivy
/*
* Copyright 2023 Squircle CE contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.blacksquircle.ui.language.smali.lexer;
import org.jetbrains.annotations.NotNull;
@SuppressWarnings("all")
/**
* This class is a scanner generated by
* JFlex 1.8.2
* from the specification file smali.flex
*/
public class SmaliLexer {
/** This character denotes the end of file. */
public static final int YYEOF = -1;
/** Initial size of the lookahead buffer. */
private static final int ZZ_BUFFERSIZE = 16384;
// Lexical states.
public static final int YYINITIAL = 0;
/**
* ZZ_LEXSTATE[l] is the state in the DFA for the lexical state l
* ZZ_LEXSTATE[l+1] is the state in the DFA for the lexical state l
* at the beginning of a line
* l is of the form l = 2*k, k a non negative integer
*/
private static final int ZZ_LEXSTATE[] = {
0, 0
};
/**
* Top-level table for translating characters to character classes
*/
private static final int [] ZZ_CMAP_TOP = zzUnpackcmap_top();
private static final String ZZ_CMAP_TOP_PACKED_0 =
"\1\0\1\u0100\1\u0200\1\u0300\1\u0400\1\u0500\1\u0600\1\u0700"+
"\1\u0800\1\u0900\1\u0a00\1\u0b00\1\u0c00\1\u0d00\1\u0e00\1\u0f00"+
"\1\u1000\1\u0100\1\u1100\1\u1200\1\u1300\1\u0100\1\u1400\1\u1500"+
"\1\u1600\1\u1700\1\u1800\1\u1900\1\u1a00\1\u1b00\1\u0100\1\u1c00"+
"\1\u1d00\1\u1e00\12\u1f00\1\u2000\1\u2100\1\u2200\1\u1f00\1\u2300"+
"\1\u2400\2\u1f00\31\u0100\1\u2500\121\u0100\1\u2600\4\u0100\1\u2700"+
"\1\u0100\1\u2800\1\u2900\1\u2a00\1\u2b00\1\u2c00\1\u2d00\53\u0100"+
"\1\u2e00\10\u2f00\31\u1f00\1\u0100\1\u3000\1\u3100\1\u0100\1\u3200"+
"\1\u3300\1\u3400\1\u3500\1\u3600\1\u3700\1\u3800\1\u3900\1\u3a00"+
"\1\u0100\1\u3b00\1\u3c00\1\u3d00\1\u3e00\1\u3f00\1\u4000\3\u1f00"+
"\1\u4100\1\u4200\1\u4300\1\u4400\1\u4500\1\u4600\1\u4700\1\u4800"+
"\1\u4900\1\u1f00\1\u4a00\1\u1f00\1\u4b00\1\u4c00\2\u1f00\3\u0100"+
"\1\u4d00\1\u4e00\1\u4f00\12\u1f00\4\u0100\1\u5000\17\u1f00\2\u0100"+
"\1\u5100\41\u1f00\2\u0100\1\u5200\1\u5300\3\u1f00\1\u5400\27\u0100"+
"\1\u5500\2\u0100\1\u5600\45\u1f00\1\u0100\1\u5700\1\u5800\11\u1f00"+
"\1\u5900\24\u1f00\1\u5a00\1\u5b00\1\u1f00\1\u5c00\1\u5d00\1\u5e00"+
"\1\u5f00\2\u1f00\1\u6000\5\u1f00\1\u6100\7\u1f00\1\u6200\1\u6300"+
"\4\u1f00\1\u6400\21\u1f00\246\u0100\1\u6500\20\u0100\1\u6600\1\u6700"+
"\25\u0100\1\u6800\34\u0100\1\u6900\14\u1f00\2\u0100\1\u6a00\u0b05\u1f00"+
"\1\u6b00\1\u6c00\u02fe\u1f00";
private static int [] zzUnpackcmap_top() {
int [] result = new int[4352];
int offset = 0;
offset = zzUnpackcmap_top(ZZ_CMAP_TOP_PACKED_0, offset, result);
return result;
}
private static int zzUnpackcmap_top(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/**
* Second-level tables for translating characters to character classes
*/
private static final int [] ZZ_CMAP_BLOCKS = zzUnpackcmap_blocks();
private static final String ZZ_CMAP_BLOCKS_PACKED_0 =
"\11\0\1\1\1\2\1\3\1\4\1\5\16\0\4\6"+
"\1\7\1\6\1\10\1\11\1\12\2\6\1\13\1\14"+
"\1\15\1\6\1\16\1\17\1\20\1\21\1\22\1\23"+
"\1\24\1\25\1\26\1\27\1\30\1\31\1\30\1\32"+
"\1\30\1\33\1\34\1\6\1\35\1\36\1\6\1\37"+
"\1\40\1\41\1\42\1\43\1\44\1\45\2\12\2\46"+
"\1\12\1\47\3\12\1\50\2\12\1\46\2\12\1\51"+
"\1\12\1\52\1\12\1\46\1\6\1\53\2\6\1\54"+
"\1\6\1\55\1\56\1\57\1\60\1\61\1\62\1\63"+
"\1\64\1\65\1\66\1\67\1\70\1\71\1\72\1\73"+
"\1\74\1\75\1\76\1\77\1\100\1\101\1\102\1\103"+
"\1\104\1\105\1\106\1\107\1\6\1\110\1\6\6\0"+
"\1\111\32\0\2\6\4\12\4\6\1\12\2\6\1\0"+
"\7\6\1\12\4\6\1\12\5\6\27\12\1\6\37\12"+
"\1\6\u01ca\12\4\6\14\12\16\6\5\12\7\6\1\12"+
"\1\6\1\12\21\6\160\0\5\12\1\6\2\12\2\6"+
"\4\12\1\6\1\12\6\6\1\12\1\6\3\12\1\6"+
"\1\12\1\6\24\12\1\6\123\12\1\6\213\12\1\6"+
"\5\0\2\6\246\12\1\6\46\12\2\6\1\12\7\6"+
"\47\12\7\6\1\12\1\6\55\0\1\6\1\0\1\6"+
"\2\0\1\6\2\0\1\6\1\0\10\6\33\12\5\6"+
"\3\12\15\6\6\0\5\6\1\12\4\6\13\0\1\6"+
"\1\0\3\6\53\12\37\0\4\6\2\12\1\0\143\12"+
"\1\6\1\12\10\0\1\6\6\0\2\12\2\0\1\6"+
"\4\0\2\12\12\0\3\12\2\6\1\12\17\6\1\0"+
"\1\12\1\0\36\12\33\0\2\6\131\12\13\0\1\12"+
"\16\6\12\0\41\12\11\0\2\12\4\6\1\12\5\6"+
"\26\12\4\0\1\12\11\0\1\12\3\0\1\12\5\0"+
"\22\6\31\12\3\0\4\6\13\12\65\6\25\12\1\6"+
"\10\12\26\6\60\0\66\12\3\0\1\12\22\0\1\12"+
"\7\0\12\12\2\0\2\6\12\0\1\6\20\12\3\0"+
"\1\6\10\12\2\6\2\12\2\6\26\12\1\6\7\12"+
"\1\6\1\12\3\6\4\12\2\6\1\0\1\12\7\0"+
"\2\6\2\0\2\6\3\0\1\12\10\6\1\0\4\6"+
"\2\12\1\6\3\12\2\0\2\6\12\0\4\12\7\6"+
"\2\12\4\6\3\0\1\6\6\12\4\6\2\12\2\6"+
"\26\12\1\6\7\12\1\6\2\12\1\6\2\12\1\6"+
"\2\12\2\6\1\0\1\6\5\0\4\6\2\0\2\6"+
"\3\0\3\6\1\0\7\6\4\12\1\6\1\12\7\6"+
"\14\0\3\12\1\0\13\6\3\0\1\6\11\12\1\6"+
"\3\12\1\6\26\12\1\6\7\12\1\6\2\12\1\6"+
"\5\12\2\6\1\0\1\12\10\0\1\6\3\0\1\6"+
"\3\0\2\6\1\12\17\6\2\12\2\0\2\6\12\0"+
"\1\6\1\12\7\6\1\12\6\0\1\6\3\0\1\6"+
"\10\12\2\6\2\12\2\6\26\12\1\6\7\12\1\6"+
"\2\12\1\6\5\12\2\6\1\0\1\12\7\0\2\6"+
"\2\0\2\6\3\0\10\6\2\0\4\6\2\12\1\6"+
"\3\12\2\0\2\6\12\0\1\6\1\12\20\6\1\0"+
"\1\12\1\6\6\12\3\6\3\12\1\6\4\12\3\6"+
"\2\12\1\6\1\12\1\6\2\12\3\6\2\12\3\6"+
"\3\12\3\6\14\12\4\6\5\0\3\6\3\0\1\6"+
"\4\0\2\6\1\12\6\6\1\0\16\6\12\0\11\6"+
"\1\12\6\6\4\0\1\6\10\12\1\6\3\12\1\6"+
"\27\12\1\6\20\12\3\6\1\12\7\0\1\6\3\0"+
"\1\6\4\0\7\6\2\0\1\6\3\12\5\6\2\12"+
"\2\0\2\6\12\0\20\6\1\12\3\0\1\6\10\12"+
"\1\6\3\12\1\6\27\12\1\6\12\12\1\6\5\12"+
"\2\6\1\0\1\12\7\0\1\6\3\0\1\6\4\0"+
"\7\6\2\0\7\6\1\12\1\6\2\12\2\0\2\6"+
"\12\0\1\6\2\12\15\6\4\0\1\6\10\12\1\6"+
"\3\12\1\6\51\12\2\0\1\12\7\0\1\6\3\0"+
"\1\6\4\0\1\12\5\6\3\12\1\0\7\6\3\12"+
"\2\0\2\6\12\0\12\6\6\12\2\6\2\0\1\6"+
"\22\12\3\6\30\12\1\6\11\12\1\6\1\12\2\6"+
"\7\12\3\6\1\0\4\6\6\0\1\6\1\0\1\6"+
"\10\0\6\6\12\0\2\6\2\0\15\6\60\12\1\0"+
"\2\12\7\0\4\6\10\12\10\0\1\6\12\0\47\6"+
"\2\12\1\6\1\12\2\6\2\12\1\6\1\12\2\6"+
"\1\12\6\6\4\12\1\6\7\12\1\6\3\12\1\6"+
"\1\12\1\6\1\12\2\6\2\12\1\6\4\12\1\0"+
"\2\12\6\0\1\6\2\0\1\12\2\6\5\12\1\6"+
"\1\12\1\6\6\0\2\6\12\0\2\6\4\12\40\6"+
"\1\12\27\6\2\0\6\6\12\0\13\6\1\0\1\6"+
"\1\0\1\6\1\0\4\6\2\0\10\12\1\6\44\12"+
"\4\6\24\0\1\6\2\0\5\12\13\0\1\6\44\0"+
"\11\6\1\0\71\6\53\12\24\0\1\12\12\0\6\6"+
"\6\12\4\0\4\12\3\0\1\12\3\0\2\12\7\0"+
"\3\12\4\0\15\12\14\0\1\12\17\0\2\6\46\12"+
"\1\6\1\12\5\6\1\12\2\6\53\12\1\6\115\12"+
"\1\6\4\12\2\6\7\12\1\6\1\12\1\6\4\12"+
"\2\6\51\12\1\6\4\12\2\6\41\12\1\6\4\12"+
"\2\6\7\12\1\6\1\12\1\6\4\12\2\6\17\12"+
"\1\6\71\12\1\6\4\12\2\6\103\12\2\6\3\0"+
"\40\6\20\12\20\6\126\12\2\6\6\12\3\6\u016c\12"+
"\2\6\21\12\1\6\32\12\5\6\113\12\3\6\13\12"+
"\7\6\15\12\1\6\4\12\3\0\13\6\22\12\3\0"+
"\13\6\22\12\2\0\14\6\15\12\1\6\3\12\1\6"+
"\2\0\14\6\64\12\40\0\3\6\1\12\3\6\2\12"+
"\1\0\2\6\12\0\41\6\4\0\1\6\12\0\6\6"+
"\130\12\10\6\5\12\2\0\42\12\1\0\1\12\5\6"+
"\106\12\12\6\37\12\1\6\14\0\4\6\14\0\12\6"+
"\12\0\36\12\2\6\5\12\13\6\54\12\4\6\32\12"+
"\6\6\12\0\46\6\27\12\5\0\4\6\65\12\12\0"+
"\1\6\35\0\2\6\13\0\6\6\12\0\15\6\1\12"+
"\10\6\16\0\102\6\5\0\57\12\21\0\7\12\4\6"+
"\12\0\21\6\11\0\14\6\3\0\36\12\15\0\2\12"+
"\12\0\54\12\16\0\14\6\44\12\24\0\10\6\12\0"+
"\3\6\3\12\12\0\44\12\2\6\11\12\107\6\3\0"+
"\1\6\25\0\4\12\1\0\4\12\3\0\2\12\3\0"+
"\6\6\300\12\72\0\1\6\5\0\26\12\2\6\6\12"+
"\2\6\46\12\2\6\6\12\2\6\10\12\1\6\1\12"+
"\1\6\1\12\1\6\1\12\1\6\37\12\2\6\65\12"+
"\1\6\7\12\1\6\1\12\3\6\3\12\1\6\7\12"+
"\3\6\4\12\2\6\6\12\4\6\15\12\5\6\3\12"+
"\1\6\7\12\16\6\5\0\30\6\2\3\5\0\20\6"+
"\2\12\23\6\1\12\13\6\5\0\1\6\12\0\1\6"+
"\1\12\15\6\1\12\20\6\15\12\3\6\40\12\20\6"+
"\15\0\4\6\1\0\3\6\14\0\21\6\1\12\4\6"+
"\1\12\2\6\12\12\1\6\1\12\3\6\5\12\6\6"+
"\1\12\1\6\1\12\1\6\1\12\1\6\4\12\1\6"+
"\13\12\2\6\4\12\5\6\5\12\4\6\1\12\21\6"+
"\51\12\u0177\6\57\12\1\6\57\12\1\6\205\12\6\6"+
"\4\12\3\0\2\12\14\6\46\12\1\6\1\12\5\6"+
"\1\12\2\6\70\12\7\6\1\12\17\6\1\0\27\12"+
"\11\6\7\12\1\6\7\12\1\6\7\12\1\6\7\12"+
"\1\6\7\12\1\6\7\12\1\6\7\12\1\6\7\12"+
"\1\6\40\0\57\6\1\12\325\6\3\12\31\6\11\12"+
"\6\0\1\6\5\12\2\6\5\12\4\6\126\12\2\6"+
"\2\0\2\6\3\12\1\6\132\12\1\6\4\12\5\6"+
"\52\12\2\6\136\12\21\6\33\12\65\6\306\12\112\6"+
"\353\12\25\6\215\12\103\6\56\12\2\6\15\12\3\6"+
"\20\12\12\0\2\12\24\6\57\12\1\0\4\6\12\0"+
"\1\6\37\12\2\0\120\12\2\0\45\6\11\12\2\6"+
"\147\12\2\6\44\12\1\6\10\12\77\6\13\12\1\0"+
"\3\12\1\0\4\12\1\0\27\12\5\0\20\6\1\12"+
"\7\6\64\12\14\6\2\0\62\12\22\0\12\6\12\0"+
"\6\6\22\0\6\12\3\6\1\12\1\6\1\12\2\6"+
"\12\0\34\12\10\0\2\6\27\12\15\0\14\6\35\12"+
"\3\6\4\0\57\12\16\0\16\6\1\12\12\0\6\6"+
"\5\12\1\0\12\12\12\0\5\12\1\6\51\12\16\0"+
"\11\6\3\12\1\0\10\12\2\0\2\6\12\0\6\6"+
"\27\12\3\6\1\12\3\0\62\12\1\0\1\12\3\0"+
"\2\12\2\0\5\12\2\0\1\12\1\0\1\12\30\6"+
"\3\12\2\6\13\12\5\0\2\6\3\12\2\0\12\6"+
"\6\12\2\6\6\12\2\6\6\12\11\6\7\12\1\6"+
"\7\12\1\6\53\12\1\6\12\12\12\6\163\12\10\0"+
"\1\6\2\0\2\6\12\0\6\6\244\12\14\6\27\12"+
"\4\6\61\12\4\6\u0100\3\156\12\2\6\152\12\46\6"+
"\7\12\14\6\5\12\5\6\1\12\1\0\12\12\1\6"+
"\15\12\1\6\5\12\1\6\1\12\1\6\2\12\1\6"+
"\2\12\1\6\154\12\41\6\153\12\22\6\100\12\2\6"+
"\66\12\50\6\15\12\3\6\20\0\20\6\20\0\3\6"+
"\2\12\30\6\3\12\31\6\1\12\6\6\5\12\1\6"+
"\207\12\2\6\1\0\4\6\1\12\13\6\12\0\7\6"+
"\32\12\4\6\1\12\1\6\32\12\13\6\131\12\3\6"+
"\6\12\2\6\6\12\2\6\6\12\2\6\3\12\3\6"+
"\2\12\3\6\2\12\22\6\3\0\4\6\14\12\1\6"+
"\32\12\1\6\23\12\1\6\2\12\1\6\17\12\2\6"+
"\16\12\42\6\173\12\105\6\65\12\210\6\1\0\202\6"+
"\35\12\3\6\61\12\17\6\1\0\37\6\40\12\15\6"+
"\36\12\5\6\46\12\5\0\5\6\36\12\2\6\44\12"+
"\4\6\10\12\1\6\5\12\52\6\236\12\2\6\12\0"+
"\6\6\44\12\4\6\44\12\4\6\50\12\10\6\64\12"+
"\234\6\67\12\11\6\26\12\12\6\10\12\230\6\6\12"+
"\2\6\1\12\1\6\54\12\1\6\2\12\3\6\1\12"+
"\2\6\27\12\12\6\27\12\11\6\37\12\101\6\23\12"+
"\1\6\2\12\12\6\26\12\12\6\32\12\106\6\70\12"+
"\6\6\2\12\100\6\1\12\3\0\1\6\2\0\5\6"+
"\4\0\4\12\1\6\3\12\1\6\33\12\4\6\3\0"+
"\4\6\1\0\40\6\35\12\3\6\35\12\43\6\10\12"+
"\1\6\34\12\2\0\31\6\66\12\12\6\26\12\12\6"+
"\23\12\15\6\22\12\156\6\111\12\67\6\63\12\15\6"+
"\63\12\15\6\3\0\65\12\17\0\37\6\12\0\17\6"+
"\4\0\55\12\13\0\2\6\1\0\22\6\31\12\7\6"+
"\12\0\6\6\3\0\44\12\16\0\1\6\12\0\20\6"+
"\43\12\1\0\2\6\1\12\11\6\3\0\60\12\16\0"+
"\4\12\5\6\3\0\3\6\12\0\1\12\1\6\1\12"+
"\43\6\22\12\1\6\31\12\14\0\6\6\1\0\101\6"+
"\7\12\1\6\1\12\1\6\4\12\1\6\17\12\1\6"+
"\12\12\7\6\57\12\14\0\5\6\12\0\6\6\4\0"+
"\1\6\10\12\2\6\2\12\2\6\26\12\1\6\7\12"+
"\1\6\2\12\1\6\5\12\2\6\1\0\1\12\7\0"+
"\2\6\2\0\2\6\3\0\2\6\1\12\6\6\1\0"+
"\5\6\5\12\2\0\2\6\7\0\3\6\5\0\213\6"+
"\65\12\22\0\4\12\5\6\12\0\46\6\60\12\24\0"+
"\2\12\1\6\1\12\10\6\12\0\246\6\57\12\7\0"+
"\2\6\11\0\27\6\4\12\2\0\42\6\60\12\21\0"+
"\3\6\1\12\13\6\12\0\46\6\53\12\15\0\10\6"+
"\12\0\66\6\32\12\3\6\17\0\4\6\12\0\u0166\6"+
"\100\12\12\0\25\6\2\12\12\0\50\12\7\0\1\12"+
"\4\0\10\6\1\0\10\6\1\12\13\0\50\12\2\6"+
"\4\12\20\0\46\6\71\12\7\6\11\12\1\6\45\12"+
"\10\0\1\6\10\0\1\12\17\6\12\0\30\6\36\12"+
"\2\6\26\0\1\6\16\0\111\6\7\12\1\6\2\12"+
"\1\6\46\12\6\0\3\6\1\0\1\6\2\0\1\6"+
"\7\0\1\12\1\0\10\6\12\0\246\6\232\12\146\6"+
"\157\12\21\6\304\12\274\6\57\12\321\6\107\12\271\6"+
"\71\12\7\6\37\12\1\6\12\0\146\6\36\12\2\6"+
"\5\0\13\6\60\12\7\0\11\6\4\12\14\6\12\0"+
"\11\6\25\12\5\6\23\12\160\6\105\12\13\6\1\12"+
"\56\0\20\6\4\0\15\12\100\6\2\12\36\6\355\12"+
"\23\6\363\12\15\6\37\12\121\6\u018c\12\4\6\153\12"+
"\5\6\15\12\3\6\11\12\7\6\12\12\3\6\2\0"+
"\1\6\4\0\301\6\5\0\3\6\26\0\2\6\7\0"+
"\36\6\4\0\224\6\3\0\273\6\125\12\1\6\107\12"+
"\1\6\2\12\2\6\1\12\2\6\2\12\2\6\4\12"+
"\1\6\14\12\1\6\1\12\1\6\7\12\1\6\101\12"+
"\1\6\4\12\2\6\10\12\1\6\7\12\1\6\34\12"+
"\1\6\4\12\1\6\5\12\1\6\1\12\3\6\7\12"+
"\1\6\u0154\12\2\6\31\12\1\6\31\12\1\6\37\12"+
"\1\6\31\12\1\6\37\12\1\6\31\12\1\6\37\12"+
"\1\6\31\12\1\6\37\12\1\6\31\12\1\6\10\12"+
"\2\6\151\0\4\6\62\0\10\6\1\0\16\6\1\0"+
"\26\6\5\0\1\6\17\0\120\6\7\0\1\6\21\0"+
"\2\6\7\0\1\6\2\0\1\6\5\0\325\6\305\12"+
"\13\6\7\0\51\6\104\12\7\0\5\6\12\0\246\6"+
"\4\12\1\6\33\12\1\6\2\12\1\6\1\12\2\6"+
"\1\12\1\6\12\12\1\6\4\12\1\6\1\12\1\6"+
"\1\12\6\6\1\12\4\6\1\12\1\6\1\12\1\6"+
"\1\12\1\6\3\12\1\6\2\12\1\6\1\12\2\6"+
"\1\12\1\6\1\12\1\6\1\12\1\6\1\12\1\6"+
"\1\12\1\6\2\12\1\6\1\12\2\6\4\12\1\6"+
"\7\12\1\6\4\12\1\6\4\12\1\6\1\12\1\6"+
"\12\12\1\6\21\12\5\6\3\12\1\6\5\12\1\6"+
"\21\12\104\6\327\12\51\6\65\12\13\6\336\12\2\6"+
"\u0182\12\16\6\u0131\12\37\6\36\12\343\6\1\0\36\6"+
"\140\0\200\6\360\0\20\6";
private static int [] zzUnpackcmap_blocks() {
int [] result = new int[27904];
int offset = 0;
offset = zzUnpackcmap_blocks(ZZ_CMAP_BLOCKS_PACKED_0, offset, result);
return result;
}
private static int zzUnpackcmap_blocks(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/**
* Translates DFA states to action switch labels.
*/
private static final int [] ZZ_ACTION = zzUnpackAction();
private static final String ZZ_ACTION_PACKED_0 =
"\1\0\1\1\2\2\1\3\1\4\1\5\1\6\1\7"+
"\1\10\1\11\1\1\1\12\2\13\1\14\1\15\1\16"+
"\1\17\1\20\1\5\1\21\24\5\1\22\1\23\1\3"+
"\1\0\1\6\1\0\1\24\1\25\1\26\12\0\1\13"+
"\2\26\1\27\1\30\1\13\71\5\2\3\2\6\21\0"+
"\1\26\1\0\1\13\1\0\1\31\31\5\1\0\15\5"+
"\1\0\1\32\2\5\1\0\27\5\27\0\1\5\1\0"+
"\1\33\7\5\1\0\5\5\1\34\7\5\1\35\1\5"+
"\3\0\1\36\3\5\1\0\2\5\1\36\2\5\1\37"+
"\1\5\5\0\1\40\2\0\7\5\1\41\1\0\3\5"+
"\1\0\5\5\1\42\4\5\5\0\1\43\3\0\1\44"+
"\13\0\1\5\3\0\4\5\1\45\2\5\2\0\1\46"+
"\1\0\3\5\1\47\1\5\1\0\3\5\1\0\1\5"+
"\1\50\1\0\3\5\1\0\2\5\2\0\1\5\14\0"+
"\5\5\1\0\1\5\2\0\3\5\1\0\3\5\1\51"+
"\4\5\2\0\1\52\1\53\7\0\1\54\1\0\1\55"+
"\2\0\1\56\6\0\1\57\1\5\7\0\1\5\1\0"+
"\1\5\7\0\1\5\1\0\3\5\2\0\1\5\1\0"+
"\1\5\2\0\1\5\1\60\7\0\3\5\1\0\2\5"+
"\4\0\1\5\16\0\1\33\1\0\2\5\1\51\1\0"+
"\1\5\7\0\1\34\1\5\1\0\2\5\1\0\4\5"+
"\15\0\1\61\1\62\4\0\1\63\2\0\1\5\10\0"+
"\1\5\1\0\1\5\12\0\1\64\1\0\1\5\1\0"+
"\1\5\1\0\1\5\4\0\1\5\1\65\1\66\1\5"+
"\10\0\1\5\1\0\2\5\1\0\1\5\6\0\1\5"+
"\4\0\1\67\3\0\1\37\6\0\1\33\1\0\1\5"+
"\11\0\1\33\1\0\2\5\1\0\1\5\33\0\1\5"+
"\1\0\1\5\2\0\1\33\6\0\1\70\1\0\1\5"+
"\1\0\1\5\7\0\1\71\12\0\2\5\30\0\1\72"+
"\5\0\1\5\3\0\1\73\12\0\1\5\4\0\1\74"+
"\11\0\1\75\2\0\1\76\10\0\1\5\1\0\1\71"+
"\10\0\1\5\4\0\1\77\5\0\1\36\4\0\1\36"+
"\5\0\1\5\15\0\1\36\5\0\1\37\2\0\1\100"+
"\14\0\1\41\3\0\1\41\2\0\1\5\6\0\1\101"+
"\1\102\2\0\1\103\4\0\1\104\5\0\1\105\4\0"+
"\1\106\1\0\1\5\11\0\1\107\5\0\1\110\1\0"+
"\1\5\43\0\1\111\1\0\1\112\1\113\1\114\2\0"+
"\1\115\3\0\1\116\26\0\1\5\16\0\1\51\1\117"+
"\6\0\1\120\2\0\1\32\1\51\17\0\1\121\2\0"+
"\1\122\12\0\1\5\10\0\1\123\7\0\1\124\1\125"+
"\17\0\1\126\7\0\1\127\2\0\1\130\1\131\4\0"+
"\1\131\4\0\1\132\2\0\1\133\6\0\1\134\1\135"+
"\1\136\1\137\6\0\1\140\2\0\1\71\15\0\1\141"+
"\1\142\17\0\1\143\22\0\1\144\16\0\1\145\1\0"+
"\1\146\15\0\1\147\1\150\1\151\1\0\1\152\6\0"+
"\1\153\1\154\1\155\2\0\1\156\3\0\1\157\1\160"+
"\3\0\1\161\2\0\1\162\5\0\1\34\5\0\1\163"+
"\10\0\1\164\1\165\1\166\1\167";
private static int [] zzUnpackAction() {
int [] result = new int[1227];
int offset = 0;
offset = zzUnpackAction(ZZ_ACTION_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAction(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/**
* Translates a state to a row index in the transition table
*/
private static final int [] ZZ_ROWMAP = zzUnpackRowMap();
private static final String ZZ_ROWMAP_PACKED_0 =
"\0\0\0\112\0\112\0\224\0\336\0\u0128\0\u0172\0\u01bc"+
"\0\112\0\112\0\112\0\u0206\0\u0250\0\u029a\0\u02e4\0\112"+
"\0\112\0\112\0\112\0\u0172\0\u032e\0\u0172\0\u0378\0\u03c2"+
"\0\u040c\0\u0456\0\u04a0\0\u04ea\0\u0534\0\u057e\0\u05c8\0\u0612"+
"\0\u065c\0\u06a6\0\u06f0\0\u073a\0\u0784\0\u07ce\0\u0818\0\u0862"+
"\0\u08ac\0\u08f6\0\112\0\112\0\112\0\u0940\0\112\0\u098a"+
"\0\112\0\112\0\u09d4\0\u0a1e\0\u0a68\0\u0ab2\0\u0afc\0\u0b46"+
"\0\u0b90\0\u0bda\0\u0c24\0\u0c6e\0\u0cb8\0\u0d02\0\112\0\u0d4c"+
"\0\112\0\112\0\u0d96\0\u0de0\0\u0e2a\0\u0e74\0\u0ebe\0\u0f08"+
"\0\u0f52\0\u0f9c\0\u0fe6\0\u1030\0\u107a\0\u10c4\0\u110e\0\u1158"+
"\0\u11a2\0\u11ec\0\u1236\0\u1280\0\u12ca\0\u1314\0\u135e\0\u13a8"+
"\0\u13f2\0\u143c\0\u1486\0\u14d0\0\u151a\0\u1564\0\u15ae\0\u15f8"+
"\0\u1642\0\u168c\0\u16d6\0\u1720\0\u176a\0\u17b4\0\u17fe\0\u1848"+
"\0\u1892\0\u18dc\0\u1926\0\u1970\0\u19ba\0\u1a04\0\u1a4e\0\u1a98"+
"\0\u1ae2\0\u1b2c\0\u1b76\0\u1bc0\0\u1c0a\0\u1c54\0\u1c9e\0\u1ce8"+
"\0\u1d32\0\u1d7c\0\u1dc6\0\u1e10\0\u1e5a\0\u1ea4\0\u1eee\0\u1f38"+
"\0\u1f82\0\u1fcc\0\u2016\0\u2060\0\u20aa\0\u20f4\0\u213e\0\u2188"+
"\0\u21d2\0\u221c\0\u2266\0\u22b0\0\u22fa\0\u2344\0\u238e\0\u23d8"+
"\0\u2422\0\u246c\0\u24b6\0\u2500\0\u254a\0\112\0\u2594\0\u25de"+
"\0\u2628\0\u2672\0\u26bc\0\u2706\0\u2750\0\u279a\0\u27e4\0\u282e"+
"\0\u2878\0\u28c2\0\u290c\0\u2956\0\u29a0\0\u29ea\0\u2a34\0\u2a7e"+
"\0\u2ac8\0\u2b12\0\u2b5c\0\u2ba6\0\u2bf0\0\u2c3a\0\u2c84\0\u2cce"+
"\0\u2d18\0\u2d62\0\u2dac\0\u2df6\0\u2e40\0\u2e8a\0\u2ed4\0\u2f1e"+
"\0\u2f68\0\u2fb2\0\u2ffc\0\u3046\0\u3090\0\u30da\0\u0172\0\u3124"+
"\0\u316e\0\u31b8\0\u3202\0\u324c\0\u3296\0\u32e0\0\u332a\0\u3374"+
"\0\u33be\0\u3408\0\u3452\0\u349c\0\u34e6\0\u3530\0\u357a\0\u35c4"+
"\0\u360e\0\u3658\0\u36a2\0\u36ec\0\u3736\0\u3780\0\u37ca\0\u3814"+
"\0\u385e\0\u38a8\0\u38f2\0\u393c\0\u3986\0\u39d0\0\u3a1a\0\u3a64"+
"\0\u3aae\0\u3af8\0\u3b42\0\u3b8c\0\u3bd6\0\u3c20\0\u3c6a\0\u3cb4"+
"\0\u3cfe\0\u3d48\0\u3d92\0\u3ddc\0\u3e26\0\u3e70\0\u3eba\0\u3f04"+
"\0\u3f4e\0\u3f98\0\u3fe2\0\u402c\0\u4076\0\u40c0\0\u410a\0\u4154"+
"\0\u419e\0\u41e8\0\u4232\0\u427c\0\u42c6\0\u4310\0\u435a\0\u43a4"+
"\0\u0172\0\u43ee\0\u4438\0\u4482\0\u44cc\0\u4516\0\u4560\0\u45aa"+
"\0\u45f4\0\u463e\0\u4688\0\u46d2\0\u471c\0\u4766\0\u47b0\0\u47fa"+
"\0\u4844\0\u488e\0\u48d8\0\u4922\0\u496c\0\u49b6\0\u4a00\0\u4a4a"+
"\0\u4a94\0\u4ade\0\u4b28\0\u4b72\0\u4bbc\0\u4c06\0\u0172\0\u4c50"+
"\0\u4c9a\0\u4ce4\0\u4d2e\0\u4d78\0\u4dc2\0\u4e0c\0\u4e56\0\u4ea0"+
"\0\u4eea\0\u4f34\0\u4f7e\0\u4fc8\0\u5012\0\u505c\0\u50a6\0\u50f0"+
"\0\u513a\0\u5184\0\u51ce\0\u0172\0\u5218\0\u5262\0\u52ac\0\u52f6"+
"\0\u5340\0\u538a\0\u53d4\0\u541e\0\u5468\0\112\0\u54b2\0\u54fc"+
"\0\u5546\0\112\0\u5590\0\u55da\0\u5624\0\u566e\0\u56b8\0\u5702"+
"\0\u574c\0\u5796\0\u57e0\0\u582a\0\u5874\0\u58be\0\u5908\0\u5952"+
"\0\u599c\0\u59e6\0\u5a30\0\u5a7a\0\u5ac4\0\u0172\0\u5b0e\0\u5b58"+
"\0\u5ba2\0\u5bec\0\u5c36\0\u5c80\0\u5cca\0\u5d14\0\u5d5e\0\u0172"+
"\0\u5da8\0\u5df2\0\u5e3c\0\u5e86\0\u5ed0\0\u5f1a\0\u5f64\0\u5fae"+
"\0\u5ff8\0\u6042\0\u608c\0\u60d6\0\u6120\0\u616a\0\u61b4\0\u61fe"+
"\0\u6248\0\u6292\0\u62dc\0\u6326\0\u6370\0\u63ba\0\u6404\0\u644e"+
"\0\u6498\0\u64e2\0\u652c\0\u6576\0\u65c0\0\u660a\0\u6654\0\u669e"+
"\0\u66e8\0\u6732\0\u677c\0\u67c6\0\u6810\0\u685a\0\u68a4\0\u68ee"+
"\0\u6938\0\u6982\0\u69cc\0\u6a16\0\u6a60\0\u6aaa\0\u6af4\0\u6b3e"+
"\0\u6b88\0\u6bd2\0\u6c1c\0\u6c66\0\u6cb0\0\u6cfa\0\112\0\u6d44"+
"\0\u6d8e\0\u6dd8\0\u6e22\0\u6e6c\0\u6eb6\0\u6f00\0\112\0\u6f4a"+
"\0\u6f94\0\u6fde\0\u7028\0\112\0\u7072\0\u70bc\0\u7106\0\u7150"+
"\0\u719a\0\u71e4\0\112\0\u722e\0\u7278\0\u72c2\0\u730c\0\u7356"+
"\0\u73a0\0\u73ea\0\u7434\0\u747e\0\u74c8\0\u7512\0\u755c\0\u75a6"+
"\0\u75f0\0\u763a\0\u7684\0\u76ce\0\u7718\0\u7762\0\u77ac\0\u77f6"+
"\0\u7840\0\u788a\0\u78d4\0\u791e\0\u7968\0\u79b2\0\u79fc\0\u7a46"+
"\0\u7a90\0\u7ada\0\112\0\u7b24\0\u7b6e\0\u7bb8\0\u7c02\0\u7c4c"+
"\0\u7c96\0\u7ce0\0\u7d2a\0\u7d74\0\u7dbe\0\u7e08\0\u7e52\0\u7e9c"+
"\0\u7ee6\0\u7f30\0\u7f7a\0\u7fc4\0\u800e\0\u8058\0\u80a2\0\u80ec"+
"\0\u8136\0\u8180\0\u81ca\0\u8214\0\u825e\0\u82a8\0\u82f2\0\u833c"+
"\0\u8386\0\u83d0\0\u841a\0\u8464\0\u84ae\0\u84f8\0\u8542\0\u858c"+
"\0\u85d6\0\u8620\0\u866a\0\u86b4\0\u86fe\0\u8748\0\u8792\0\u87dc"+
"\0\u8826\0\u8870\0\u88ba\0\u8904\0\u894e\0\u8998\0\u89e2\0\u8a2c"+
"\0\u8a76\0\u8ac0\0\u8b0a\0\u8b54\0\u8b9e\0\u8be8\0\u8c32\0\u8c7c"+
"\0\u8cc6\0\u8d10\0\u8d5a\0\u8da4\0\u8dee\0\u8e38\0\u8e82\0\u8ecc"+
"\0\112\0\112\0\u8f16\0\u8f60\0\u8faa\0\u8ff4\0\112\0\u903e"+
"\0\u9088\0\u90d2\0\u911c\0\u9166\0\u91b0\0\u91fa\0\u9244\0\u928e"+
"\0\u92d8\0\u9322\0\u936c\0\u93b6\0\u9400\0\u944a\0\u9494\0\u94de"+
"\0\u9528\0\u9572\0\u95bc\0\u9606\0\u9650\0\u969a\0\u96e4\0\112"+
"\0\u972e\0\u9778\0\u97c2\0\u980c\0\u9856\0\u98a0\0\u98ea\0\u9934"+
"\0\u997e\0\u99c8\0\u9a12\0\112\0\112\0\u9a5c\0\u9aa6\0\u9af0"+
"\0\u9b3a\0\u9b84\0\u9bce\0\u9c18\0\u9c62\0\u9cac\0\u9cf6\0\u9d40"+
"\0\u9d8a\0\u9dd4\0\u9e1e\0\u9e68\0\u9eb2\0\u9efc\0\u9f46\0\u9f90"+
"\0\u9fda\0\ua024\0\ua06e\0\ua0b8\0\ua102\0\ua14c\0\ua196\0\112"+
"\0\ua1e0\0\ua22a\0\ua274\0\112\0\ua2be\0\ua308\0\ua352\0\ua39c"+
"\0\ua3e6\0\ua430\0\ua47a\0\ua4c4\0\ua50e\0\ua558\0\ua5a2\0\ua5ec"+
"\0\ua636\0\ua680\0\ua6ca\0\ua714\0\ua75e\0\ua7a8\0\ua7f2\0\ua83c"+
"\0\ua886\0\ua8d0\0\ua91a\0\ua964\0\ua9ae\0\ua9f8\0\uaa42\0\uaa8c"+
"\0\uaad6\0\uab20\0\uab6a\0\uabb4\0\uabfe\0\uac48\0\uac92\0\uacdc"+
"\0\uad26\0\uad70\0\uadba\0\uae04\0\uae4e\0\uae98\0\uaee2\0\uaf2c"+
"\0\uaf76\0\uafc0\0\ub00a\0\ub054\0\ub09e\0\ub0e8\0\ub132\0\ub17c"+
"\0\ub1c6\0\ub210\0\ub25a\0\ub2a4\0\112\0\ub2ee\0\ub338\0\ub382"+
"\0\ub3cc\0\ub416\0\ub460\0\112\0\ub4aa\0\ub4f4\0\ub53e\0\ub588"+
"\0\ub5d2\0\ub61c\0\ub666\0\ub6b0\0\ub6fa\0\ub744\0\ub78e\0\ub7d8"+
"\0\ub822\0\ub86c\0\ub8b6\0\ub900\0\ub94a\0\ub994\0\ub9de\0\uba28"+
"\0\uba72\0\ubabc\0\ubb06\0\ubb50\0\ubb9a\0\ubbe4\0\ubc2e\0\ubc78"+
"\0\ubcc2\0\ubd0c\0\ubd56\0\ubda0\0\ubdea\0\ube34\0\ube7e\0\ubec8"+
"\0\ubf12\0\ubf5c\0\ubfa6\0\ubff0\0\uc03a\0\uc084\0\uc0ce\0\uc118"+
"\0\uc162\0\uc1ac\0\uc1f6\0\uc240\0\112\0\uc28a\0\uc2d4\0\uc31e"+
"\0\uc368\0\uc3b2\0\uc3fc\0\uc446\0\uc490\0\uc4da\0\uc524\0\uc56e"+
"\0\uc5b8\0\uc602\0\uc64c\0\uc696\0\uc6e0\0\uc72a\0\uc774\0\uc7be"+
"\0\uc808\0\uc852\0\uc89c\0\uc8e6\0\uc930\0\uc97a\0\112\0\uc9c4"+
"\0\uca0e\0\uca58\0\ucaa2\0\ucaec\0\ucb36\0\ucb80\0\ucbca\0\ucc14"+
"\0\112\0\ucc5e\0\ucca8\0\112\0\uccf2\0\ucd3c\0\ucd86\0\ucdd0"+
"\0\uce1a\0\uce64\0\uceae\0\ucef8\0\ucf42\0\ucf8c\0\u0172\0\ucfd6"+
"\0\ud020\0\ud06a\0\ud0b4\0\ud0fe\0\ud148\0\ud192\0\ud1dc\0\ud226"+
"\0\ud270\0\ud2ba\0\ud304\0\ud34e\0\ub666\0\ud398\0\ud3e2\0\ud42c"+
"\0\ud476\0\ud4c0\0\112\0\ud50a\0\ud554\0\ud59e\0\ud5e8\0\ud632"+
"\0\ud67c\0\ud6c6\0\ud710\0\ud75a\0\ud7a4\0\ud7ee\0\ud838\0\ud882"+
"\0\ud8cc\0\ud916\0\ud960\0\ud9aa\0\ud9f4\0\uda3e\0\uda88\0\udad2"+
"\0\udb1c\0\udb66\0\udbb0\0\udbfa\0\udc44\0\udc8e\0\udcd8\0\udd22"+
"\0\udd6c\0\uddb6\0\ude00\0\ude4a\0\112\0\ude94\0\udede\0\udf28"+
"\0\udf72\0\udfbc\0\ue006\0\ue050\0\ue09a\0\ue0e4\0\ue12e\0\ue178"+
"\0\ue1c2\0\112\0\ue20c\0\ue256\0\ue2a0\0\ue2ea\0\ue334\0\ue37e"+
"\0\ue3c8\0\ue412\0\ue45c\0\ue4a6\0\ue4f0\0\ue53a\0\ue584\0\112"+
"\0\112\0\ue5ce\0\ue618\0\112\0\ue662\0\ue6ac\0\ue6f6\0\ue740"+
"\0\112\0\ue78a\0\ue7d4\0\ue81e\0\ue868\0\ue8b2\0\112\0\ue8fc"+
"\0\ue946\0\ue990\0\ue9da\0\uea24\0\uea6e\0\ueab8\0\ueb02\0\ueb4c"+
"\0\ueb96\0\uebe0\0\uec2a\0\uec74\0\uecbe\0\ued08\0\ued52\0\112"+
"\0\ued9c\0\uede6\0\uee30\0\uee7a\0\ueec4\0\ud75a\0\uef0e\0\uef58"+
"\0\uefa2\0\uefec\0\uf036\0\uf080\0\uf0ca\0\uf114\0\uf15e\0\uf1a8"+
"\0\uf1f2\0\uf23c\0\uf286\0\uf2d0\0\uf31a\0\uf364\0\uf3ae\0\uf3f8"+
"\0\uf442\0\uf48c\0\uf4d6\0\uf520\0\uf56a\0\uf5b4\0\uf5fe\0\uf648"+
"\0\uf692\0\uf6dc\0\uf726\0\uf770\0\uf7ba\0\uf804\0\uf84e\0\uf898"+
"\0\uf8e2\0\uf92c\0\uf976\0\112\0\uf9c0\0\ue45c\0\112\0\112"+
"\0\ufa0a\0\ufa54\0\112\0\ufa9e\0\ufae8\0\ufb32\0\112\0\ufb7c"+
"\0\ufbc6\0\ufc10\0\ufc5a\0\ufca4\0\ufcee\0\ufd38\0\ufd82\0\ufdcc"+
"\0\ufe16\0\ufe60\0\ufeaa\0\ufef4\0\uff3e\0\uff88\0\uffd2\1\34"+
"\1\146\1\260\1\372\1\u0144\1\u018e\1\u01d8\1\u0222\1\u026c"+
"\1\u02b6\1\u0300\1\u034a\1\u0394\1\u03de\1\u0428\1\u0472\1\u04bc"+
"\1\u0506\1\u0550\1\u059a\1\u05e4\1\u062e\0\112\1\u0678\1\u06c2"+
"\1\u070c\1\u0756\1\u07a0\1\u07ea\0\112\1\u0834\1\u087e\1\u08c8"+
"\0\112\1\u0912\1\u095c\1\u09a6\1\u09f0\1\u0a3a\1\u0a84\1\u0ace"+
"\1\u0b18\1\u0b62\1\u0bac\1\u0bf6\1\u0c40\1\u0c8a\1\u0cd4\1\u0d1e"+
"\1\u0d68\1\u0db2\1\u0dfc\0\112\1\u0e46\1\u0e90\1\u0eda\1\u0f24"+
"\1\u0f6e\1\u0fb8\1\u1002\1\u104c\1\u1096\1\u10e0\1\u112a\1\u1174"+
"\1\u11be\1\u1208\1\u1252\1\u129c\1\u12e6\1\u1330\1\u137a\1\u13c4"+
"\1\u140e\1\u1458\1\u14a2\1\u14ec\1\u1536\1\u1580\1\u15ca\0\112"+
"\0\112\1\u1614\1\u165e\1\u16a8\1\u16f2\1\u173c\1\u1786\1\u17d0"+
"\1\u181a\1\u1864\1\u18ae\1\u18f8\1\u1942\1\u198c\1\u19d6\1\u1a20"+
"\0\112\1\u1a6a\1\u1ab4\1\u1afe\1\u1b48\1\u1b92\1\u1bdc\1\u1c26"+
"\0\112\1\u1c70\1\u1cba\1\u1d04\1\u1d4e\1\u1d98\1\u1de2\1\u1e2c"+
"\1\u1e76\1\u1ec0\1\u1f0a\1\u1f54\1\u1f9e\1\u1fe8\0\112\1\u2032"+
"\1\u207c\0\112\1\u20c6\1\u2110\1\u215a\1\u21a4\1\u21ee\1\u2238"+
"\0\112\0\112\0\112\0\112\1\u2282\1\u22cc\1\u2316\1\u2360"+
"\1\u23aa\1\u23f4\1\u243e\1\u2488\1\u24d2\0\112\1\u251c\1\u2566"+
"\1\u25b0\1\u25fa\1\u2644\1\u268e\1\u26d8\1\u2722\1\u276c\1\u27b6"+
"\1\u2800\1\u284a\1\u2894\0\112\0\112\1\u28de\1\u2928\1\u2972"+
"\1\u29bc\1\u2a06\1\u2a50\1\u2a9a\1\u2ae4\1\u2b2e\1\u2b78\1\u2bc2"+
"\1\u2c0c\1\u2c56\1\u2ca0\1\u2cea\0\112\1\u2d34\1\u2d7e\1\u2dc8"+
"\1\u2e12\1\u2e5c\1\u2ea6\1\u2ef0\1\u2f3a\1\u2f84\1\u2fce\1\u3018"+
"\1\u3062\1\u30ac\1\u30f6\1\u3140\1\u318a\1\u31d4\1\u321e\1\u3268"+
"\1\u32b2\1\u32fc\1\u3346\1\u3390\1\u33da\1\u3424\1\u346e\1\u34b8"+
"\1\u3502\1\u354c\1\u3596\1\u35e0\1\u362a\1\u3674\0\112\1\u36be"+
"\0\112\1\u3708\1\u3752\1\u379c\1\u37e6\1\u3830\1\u387a\1\u38c4"+
"\1\u390e\1\u3958\1\u39a2\1\u39ec\1\u3a36\1\u3a80\0\112\0\112"+
"\0\112\1\u3aca\0\112\1\u3b14\1\u3b5e\1\u3ba8\1\u3bf2\1\u3c3c"+
"\1\u3c86\1\u3cd0\1\u3d1a\0\112\1\u3d64\1\u3dae\0\112\1\u3df8"+
"\1\u3e42\1\u3e8c\0\112\0\112\1\u3ed6\1\u3f20\1\u3f6a\0\112"+
"\1\u3fb4\1\u3ffe\0\112\1\u4048\1\u4092\1\u40dc\1\u4126\1\u4170"+
"\0\112\1\u41ba\1\u4204\1\u424e\1\u4298\1\u42e2\0\112\1\u432c"+
"\1\u4376\1\u43c0\1\u440a\1\u4454\1\u449e\1\u44e8\1\u4532\0\112"+
"\0\112\0\112\0\112";
private static int [] zzUnpackRowMap() {
int [] result = new int[1227];
int offset = 0;
offset = zzUnpackRowMap(ZZ_ROWMAP_PACKED_0, offset, result);
return result;
}
private static int zzUnpackRowMap(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int high = packed.charAt(i++) << 16;
result[j++] = high | packed.charAt(i++);
}
return j;
}
/**
* The transition table of the DFA
*/
private static final int [] ZZ_TRANS = zzUnpackTrans();
private static final String ZZ_TRANS_PACKED_0 =
"\1\2\2\3\1\2\1\3\1\4\1\2\1\3\1\5"+
"\1\6\1\7\1\10\1\11\1\12\1\2\1\13\1\14"+
"\1\15\1\2\1\16\7\17\1\20\1\21\1\22\1\2"+
"\1\23\1\7\3\24\1\7\2\24\1\25\1\7\1\26"+
"\1\7\1\2\1\7\1\27\1\30\1\31\1\32\1\33"+
"\1\34\1\35\1\7\1\36\2\7\1\37\1\40\1\41"+
"\1\42\1\43\1\7\1\44\1\45\1\46\1\47\1\50"+
"\1\51\1\52\2\7\1\53\1\54\1\2\114\0\1\3"+
"\107\0\2\5\1\0\2\5\1\0\2\5\1\55\42\5"+
"\1\56\36\5\2\6\4\0\103\6\1\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\1\7\2\10\1\0\2\10\1\0\5\10\1\57\37\10"+
"\1\60\36\10\36\0\1\61\74\0\1\62\1\0\10\63"+
"\22\0\1\64\1\0\1\65\1\0\1\66\1\67\2\0"+
"\1\70\2\0\1\71\1\72\2\0\1\73\1\0\1\74"+
"\1\75\33\0\1\63\1\0\10\17\6\0\1\76\1\0"+
"\1\77\1\100\1\101\1\0\1\102\2\0\1\103\1\0"+
"\1\17\1\0\1\76\1\0\1\77\1\100\1\101\5\0"+
"\1\102\13\0\1\103\26\0\1\63\1\0\10\17\10\0"+
"\1\77\1\100\1\101\1\0\1\102\4\0\1\17\3\0"+
"\1\77\1\100\1\101\5\0\1\102\21\0\1\7\11\0"+
"\1\104\10\0\10\7\5\0\13\104\1\0\33\104\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\2\7\1\105\1\7\1\106\2\7\1\107\6\7\1\110"+
"\1\7\1\111\1\7\1\112\10\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\14\7\1\113"+
"\5\7\1\114\2\7\1\115\5\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\10\7\1\116"+
"\3\7\1\117\1\120\1\7\1\121\13\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\5\7"+
"\1\122\3\7\1\123\5\7\1\124\13\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\16\7"+
"\1\125\11\7\1\126\2\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\1\7\1\127\7\7"+
"\1\130\2\7\1\131\16\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\5\7\1\132\11\7"+
"\1\133\2\7\1\134\10\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\6\7\1\135\1\136"+
"\4\7\1\137\1\7\1\140\1\7\1\141\12\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\17\7\1\142\13\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\17\7\1\143\5\7\1\144"+
"\5\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\1\7\1\145\3\7\1\146\11\7\1\147"+
"\5\7\1\150\5\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\22\7\1\151\10\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\1\7\1\152\20\7\1\153\2\7\1\154\5\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\5\7\1\155\15\7\1\156\1\7\1\157\5\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\7\7\1\160\1\161\7\7\1\162\3\7\1\163\1\164"+
"\3\7\1\165\1\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\10\7\1\166\11\7\1\167"+
"\10\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\23\7\1\170\7\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\1\7\1\171"+
"\15\7\1\172\4\7\1\173\6\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\10\7\1\174"+
"\22\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\17\7\1\42\13\7\2\0\1\7\1\5"+
"\1\175\2\5\1\175\1\176\1\5\1\175\102\5\1\10"+
"\1\177\2\10\1\177\1\200\1\10\1\177\102\10\23\0"+
"\10\63\10\0\1\77\1\100\1\101\6\0\1\63\3\0"+
"\1\77\1\100\1\101\121\0\1\201\3\0\1\202\70\0"+
"\1\203\12\0\1\204\113\0\1\205\1\0\1\206\102\0"+
"\1\207\115\0\1\210\105\0\1\211\5\0\1\212\77\0"+
"\1\213\105\0\1\214\20\0\1\215\74\0\1\216\123\0"+
"\1\217\1\220\4\0\1\221\33\0\10\76\14\0\1\102"+
"\4\0\1\76\13\0\1\102\37\0\1\222\1\0\1\222"+
"\2\0\10\222\10\0\1\77\1\0\1\101\6\0\1\222"+
"\3\0\1\77\1\0\1\101\50\0\1\223\1\0\10\224"+
"\5\0\6\224\1\0\1\102\4\0\7\224\5\0\1\102"+
"\21\0\1\104\11\0\1\104\7\0\1\225\10\104\1\0"+
"\1\226\3\0\13\104\1\0\33\104\2\0\1\104\1\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\23\7"+
"\1\227\7\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\4\7\1\230\26\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\5\7"+
"\1\231\25\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\4\7\1\151\11\7\1\232\14\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\25\7\1\231\5\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\22\7\1\233\10\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\1\7\1\234\31\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\11\7\1\235\21\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\11\7\1\236\21\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\5\7\1\237\25\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\1\7\1\240\31\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\20\7\1\241\12\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\16\7\1\242\3\7\1\243\10\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\3\7"+
"\1\244\27\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\26\7\1\230\4\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\25\7"+
"\1\245\5\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\25\7\1\246\5\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\5\7"+
"\1\247\25\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\14\7\1\250\16\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\5\7"+
"\1\251\6\7\1\252\1\7\1\253\14\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\17\7"+
"\1\254\13\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\16\7\1\255\14\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\24\7"+
"\1\256\6\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\5\7\1\257\25\7\2\0\2\7"+
"\11\0\1\7\5\0\1\260\2\0\10\7\5\0\13\7"+
"\1\0\33\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\5\7\1\261\25\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\14\7"+
"\1\262\16\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\14\7\1\263\6\7\1\264\1\265"+
"\1\7\1\266\4\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\25\7\1\267\5\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\16\7\1\270\14\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\16\7\1\271\7\7\1\272"+
"\4\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\14\7\1\230\16\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\24\7\1\273"+
"\6\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\7\7\1\274\17\7\1\275\3\7\2\0"+
"\2\7\11\0\1\7\5\0\1\276\2\0\10\7\5\0"+
"\13\7\1\0\20\7\1\277\3\7\1\300\6\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\14\7\1\301\16\7\2\0\2\7\11\0\1\7\5\0"+
"\1\302\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\3\7\1\303\27\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\11\7\1\304\5\7\1\305"+
"\13\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\2\7\1\306\30\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\15\7\1\230"+
"\6\7\1\307\6\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\25\7\1\310\5\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\16\7\1\311\14\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\5\7\1\312\25\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\14\7\1\313\5\7\1\313\10\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\1\7\1\314"+
"\23\7\1\312\5\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\1\7\1\315\20\7\1\316"+
"\10\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\2\7\1\317\30\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\16\7\1\320"+
"\4\7\1\321\7\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\22\7\1\322\10\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\1\7\1\323\23\7\1\324\5\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\10\7\1\325"+
"\22\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\22\7\1\326\10\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\14\7\1\327"+
"\16\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\1\7\1\330\31\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\11\7\1\331"+
"\21\7\2\0\1\7\1\5\1\175\2\5\1\175\1\176"+
"\1\5\1\175\1\55\42\5\1\56\43\5\1\0\2\5"+
"\1\55\42\5\1\56\36\5\1\10\1\177\2\10\1\177"+
"\1\200\1\10\1\177\3\10\1\57\37\10\1\60\43\10"+
"\1\0\5\10\1\57\37\10\1\60\36\10\72\0\1\332"+
"\115\0\1\333\113\0\1\334\66\0\1\335\114\0\1\336"+
"\20\0\1\337\75\0\1\340\105\0\1\341\124\0\1\342"+
"\107\0\1\343\76\0\1\344\132\0\1\345\70\0\1\346"+
"\16\0\1\347\106\0\1\350\101\0\1\351\13\0\1\352"+
"\113\0\1\353\65\0\1\354\112\0\1\355\15\0\1\356"+
"\40\0\10\222\10\0\1\77\1\0\1\101\6\0\1\222"+
"\3\0\1\77\1\0\1\101\52\0\10\357\5\0\6\357"+
"\6\0\7\357\50\0\1\357\1\0\10\224\5\0\6\224"+
"\1\0\1\102\1\100\3\0\7\224\5\0\1\102\3\0"+
"\1\100\27\0\1\360\25\0\13\360\1\0\33\360\3\0"+
"\1\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\24\7\1\361\6\7\2\0\2\7\11\0\1\7\5\0"+
"\1\362\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\24\7\1\363\6\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\17\7\1\364\13\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\1\7\1\365\31\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\3\7\1\366\27\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\4\7\1\367\26\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\14\7\1\370\16\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\3\7\1\371\27\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\23\7\1\372\7\7\2\0"+
"\2\7\11\0\1\7\5\0\1\373\2\0\10\7\5\0"+
"\13\7\1\0\7\7\1\374\4\7\1\374\16\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\23\7\1\375\7\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\5\7\1\376\25\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\14\7\1\377\16\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\2\7\1\u0100\30\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\15\7\1\u0101\15\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\3\7\1\u0102\27\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\23\7\1\u0103\7\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\14\7\1\u0104\16\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\14\7\1\u0105\16\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\1\7\1\u0106\31\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\1\7\1\u0107\31\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\5\7\1\u0108\25\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\17\7\1\u0109\13\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\31\7\1\u010a\1\7\2\0"+
"\1\7\61\0\1\u010b\1\0\1\u010c\4\0\1\u010c\1\0"+
"\1\u010d\17\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\24\7\1\u010e\6\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\5\7\1\u010f"+
"\25\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\11\7\1\u0110\21\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\24\7\1\u0111"+
"\6\7\2\0\2\7\11\0\1\7\5\0\1\u0112\2\0"+
"\10\7\5\0\13\7\1\0\5\7\1\u0113\25\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\17\7\1\u0114\13\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\24\7\1\u0115\6\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\7\7\1\u0116\23\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\11\7\1\u0117\21\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\5\7\1\u0118\25\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\11\7\1\u0119\21\7\2\0"+
"\2\7\11\0\1\7\5\0\1\u011a\2\0\10\7\5\0"+
"\13\7\1\0\33\7\2\0\2\7\11\0\1\7\5\0"+
"\1\u011b\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\1\7\61\0\1\u011c\15\0\1\u011d\12\0\1\7\11\0"+
"\1\7\5\0\1\u011e\2\0\10\7\5\0\13\7\1\0"+
"\33\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\14\7\1\u011f\16\7\2\0\1\7\65\0"+
"\1\u0120\2\0\1\u0121\21\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\13\7\1\u0122\17\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\26\7\1\u0123\4\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\24\7\1\u0124\6\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\14\7\1\u0125\16\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\25\7\1\u0126\5\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\2\7\1\u0127\30\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\24\7\1\u0128\6\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\24\7\1\u0129\6\7\2\0\2\7\11\0\1\7\5\0"+
"\1\u012a\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\22\7\1\u012b\10\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\24\7\1\u012c\6\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\11\7\1\u012d\21\7\2\0\2\7\11\0\1\7\5\0"+
"\1\u012e\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\3\7\1\u012f\20\7\1\u0130\6\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\24\7\1\u0131"+
"\6\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\17\7\1\u0132\13\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\16\7\1\u0133"+
"\14\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u0134\25\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\22\7\1\313"+
"\10\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\1\7\1\u0135\31\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\1\7\1\u0136"+
"\31\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\2\7\1\u0137\30\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\24\7\1\u0138"+
"\6\7\2\0\1\7\73\0\1\u0139\73\0\1\u013a\113\0"+
"\1\u013b\131\0\1\u013c\21\0\1\u013d\173\0\1\u013e\110\0"+
"\1\u013f\111\0\1\u0140\111\0\1\u0141\102\0\1\u0142\105\0"+
"\1\u0143\120\0\1\u0144\114\0\1\u0145\77\0\1\u0146\124\0"+
"\1\u0147\106\0\1\u0148\124\0\1\u0149\107\0\1\u014a\111\0"+
"\1\u014b\70\0\1\u014c\115\0\1\u014d\53\0\10\357\5\0"+
"\6\357\2\0\1\100\3\0\7\357\11\0\1\100\15\0"+
"\1\360\11\0\1\360\7\0\1\225\10\360\1\0\1\226"+
"\3\0\13\360\1\0\33\360\2\0\1\360\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\22\7\1\u014e"+
"\10\7\2\0\1\7\60\0\1\u014f\1\0\1\u0150\2\0"+
"\1\u0120\2\0\1\u0121\21\0\1\7\11\0\1\7\5\0"+
"\1\u0151\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\24\7\1\u0152\6\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\31\7\1\u0153\1\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\13\7\1\u0154\17\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\7\7\1\u0155\23\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\4\7\1\u0156\26\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\13\7\1\u0157\17\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\23\7\1\u0158\7\7\2\0\1\7\70\0\1\u0159\21\0"+
"\1\7\11\0\1\7\5\0\1\u015a\2\0\10\7\5\0"+
"\13\7\1\0\33\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\24\7\1\u015b\6\7\2\0"+
"\2\7\11\0\1\7\5\0\1\u015c\2\0\10\7\5\0"+
"\13\7\1\0\33\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\1\7\1\u015d\31\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\14\7\1\u015e\16\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\25\7\1\u015f\5\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\5\7\1\u0160\25\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\4\7\1\u0161\26\7\2\0"+
"\2\7\11\0\1\7\5\0\1\u0162\2\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u0163\25\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\14\7\1\u0101"+
"\16\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\24\7\1\u0164\6\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\22\7\1\u0165"+
"\10\7\2\0\2\7\11\0\1\7\7\0\1\u0166\10\7"+
"\5\0\13\7\1\0\33\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\14\7\1\u0167\16\7"+
"\2\0\1\7\75\0\1\u0168\75\0\1\u0168\16\0\1\u0168"+
"\72\0\1\u0168\30\0\1\7\11\0\1\7\5\0\1\u0169"+
"\2\0\10\7\5\0\13\7\1\0\33\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\7\7"+
"\1\u016a\23\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\16\7\1\u016b\14\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\1\7"+
"\1\u016c\31\7\2\0\1\7\100\0\1\u016d\11\0\1\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\22\7"+
"\1\u016e\10\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\13\7\1\u016f\17\7\2\0\2\7"+
"\11\0\1\7\5\0\1\u0170\2\0\10\7\5\0\13\7"+
"\1\0\33\7\2\0\2\7\11\0\1\7\5\0\1\u0171"+
"\2\0\10\7\5\0\13\7\1\0\33\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\24\7"+
"\1\u0172\6\7\2\0\2\7\11\0\1\7\5\0\1\u0173"+
"\1\0\1\u0174\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\26\7\1\u0155\4\7\2\0\1\7\60\0\1\u0175\1\0"+
"\1\u0176\2\0\1\u0177\2\0\1\u0178\76\0\1\u0179\7\0"+
"\1\u017a\122\0\1\u017b\114\0\1\u017c\75\0\1\u0177\2\0"+
"\1\u0178\113\0\1\u017d\112\0\1\u017e\16\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\5\7\1\u017f"+
"\25\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\1\7\1\u0180\31\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\5\7\1\u0181"+
"\25\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\11\7\1\u0182\21\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\22\7\1\u0183"+
"\10\7\2\0\2\7\11\0\1\7\5\0\1\u0184\2\0"+
"\10\7\5\0\13\7\1\0\33\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\11\7\1\u0185"+
"\21\7\2\0\2\7\11\0\1\7\5\0\1\u0186\2\0"+
"\10\7\5\0\13\7\1\0\33\7\2\0\1\7\65\0"+
"\1\u0187\2\0\1\u0121\21\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\23\7\1\u0188\7\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\11\7\1\u0189\21\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\3\7\1\u018a\27\7\2\0"+
"\1\7\60\0\1\u014f\1\0\1\u0150\2\0\1\u018b\2\0"+
"\1\u0121\21\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\10\7\1\u018c\22\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\10\7\1\u018d"+
"\22\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u018e\25\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\27\7\1\u018f"+
"\3\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\23\7\1\u0190\7\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\22\7\1\u0191"+
"\10\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\24\7\1\u0192\6\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\14\7\1\u0193"+
"\16\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u0154\25\7\2\0\1\7\100\0"+
"\1\u0194\116\0\1\u0195\70\0\1\u0196\124\0\1\u0197\67\0"+
"\1\u0198\4\0\1\u0199\5\0\1\u019a\1\u019b\2\0\1\u019c"+
"\2\0\1\u019d\105\0\1\u019e\76\0\1\u019f\112\0\1\u01a0"+
"\120\0\1\u01a1\114\0\1\u01a2\77\0\1\u01a3\121\0\1\u01a4"+
"\113\0\1\u01a5\115\0\1\u01a6\67\0\1\u01a7\113\0\1\u01a8"+
"\131\0\1\u01a9\104\0\1\u01aa\115\0\1\u01ab\13\0\1\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\1\7"+
"\1\u01ac\31\7\2\0\1\7\73\0\1\u01ad\106\0\1\u01ae"+
"\77\0\1\u01af\1\u01b0\13\0\1\u01b1\3\0\1\u01b2\3\0"+
"\1\u01b3\6\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\1\7\1\u01b4\31\7\2\0\2\7\11\0"+
"\1\7\5\0\1\u01b5\2\0\10\7\5\0\13\7\1\0"+
"\33\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\14\7\1\u01b6\16\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\5\7\1\u0101"+
"\25\7\2\0\2\7\11\0\1\7\5\0\1\u01b7\2\0"+
"\10\7\5\0\13\7\1\0\33\7\2\0\2\7\11\0"+
"\1\7\5\0\1\u01b8\2\0\10\7\5\0\13\7\1\0"+
"\33\7\2\0\1\7\73\0\1\u01b9\76\0\1\u01ba\1\0"+
"\1\u01bb\27\0\1\7\11\0\1\7\5\0\1\u01bc\1\0"+
"\1\u01bd\10\7\5\0\13\7\1\0\22\7\1\u01be\10\7"+
"\2\0\1\7\74\0\1\u01bf\15\0\1\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\22\7\1\u01c0\10\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\5\7\1\u01c1\25\7\2\0\2\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\24\7\1\u01c2\6\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\4\0\1\u01c3"+
"\13\7\1\0\33\7\2\0\1\7\55\0\1\u01c4\34\0"+
"\1\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\4\7\1\u01c5\26\7\2\0\2\7\11\0\1\7\5\0"+
"\1\u01c6\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\11\7\1\u01c7\21\7\2\0\1\7\24\0\1\u01c8\1\0"+
"\1\u01c9\63\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\11\7\1\u01ca\21\7\2\0\1\7\106\0"+
"\1\u01cb\61\0\1\u01cc\1\u01cd\13\0\1\u01ce\1\0\1\u01cf"+
"\1\0\1\u01d0\2\0\1\u01d1\1\u01d2\6\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\1\7\1\u01d3"+
"\31\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u01d4\25\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\16\7\1\u01d5"+
"\14\7\2\0\1\7\73\0\1\u01d6\16\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\6\7\1\u01d7"+
"\24\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u01d8\25\7\2\0\1\7\56\0"+
"\1\u01d9\1\u01da\13\0\1\u01ce\1\0\1\u01cf\1\0\1\u01db"+
"\2\0\1\u01d1\1\u01d2\106\0\1\u01dc\11\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\17\7\1\u01dd"+
"\13\7\2\0\1\7\61\0\1\u01de\11\0\1\u01df\2\0"+
"\1\u01e0\4\0\1\u01e1\32\0\1\u01e2\35\0\1\u01e3\122\0"+
"\1\u01e4\106\0\1\u01e5\113\0\1\u01e6\112\0\1\u01e7\114\0"+
"\1\u01e8\105\0\1\u01e9\115\0\1\u01ea\72\0\1\u01eb\132\0"+
"\1\u01ec\103\0\1\u01ed\17\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\4\7\1\u01ee\26\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\24\7\1\u0155\6\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\3\7\1\u01ef\27\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\3\7\1\u0101\27\7\2\0\2\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\16\7\1\u01f0\14\7\2\0"+
"\1\7\65\0\1\u01f1\24\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\15\7\1\u01f2\15\7\2\0"+
"\1\7\56\0\1\u01f3\1\u01f4\13\0\1\u01f5\3\0\1\u01f6"+
"\2\0\1\u01f7\1\u01f8\100\0\1\u01f9\17\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\5\7\1\u01ee"+
"\25\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\3\7\1\u01fa\27\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\24\7\1\u01fb"+
"\6\7\2\0\1\7\72\0\1\u01fc\17\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\22\7\1\u01fd"+
"\10\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u01fe\25\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\15\7\1\u0156"+
"\15\7\2\0\2\7\11\0\1\7\5\0\1\u01ff\2\0"+
"\10\7\5\0\13\7\1\0\33\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\11\7\1\u0200"+
"\21\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\7\7\1\u0201\23\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\11\7\1\u0202"+
"\21\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\5\7\1\u0203\25\7\2\0\1\7\55\0"+
"\1\u0204\54\0\1\u0205\146\0\1\u0206\126\0\1\u0207\3\0"+
"\1\u0208\100\0\1\u0209\117\0\1\u020a\77\0\1\u020b\105\0"+
"\1\u020c\130\0\1\u020d\4\0\1\u020e\73\0\1\u020f\117\0"+
"\1\u0210\117\0\1\u0211\72\0\1\u0212\111\0\1\u0213\114\0"+
"\1\u0214\126\0\1\u0215\107\0\1\u0216\74\0\1\u0217\111\0"+
"\1\u0218\122\0\1\u0219\17\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\3\7\1\u021a\27\7\2\0"+
"\1\7\101\0\1\u021b\103\0\1\u021c\111\0\1\u021d\11\0"+
"\1\u021e\70\0\1\u021f\103\0\1\u0220\117\0\1\u0221\112\0"+
"\1\u0222\24\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\24\7\1\u0223\6\7\2\0\1\7\70\0"+
"\1\u0224\21\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\11\7\1\u0225\21\7\2\0\1\7\57\0"+
"\1\u0226\111\0\1\u0227\124\0\1\u0228\112\0\1\u0229\106\0"+
"\1\u022a\100\0\1\u022b\11\0\1\u022c\5\0\1\u022d\3\0"+
"\1\u022e\32\0\1\u022f\2\0\1\u0230\34\0\1\u0231\25\0"+
"\1\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\25\7\1\u0232\5\7\2\0\1\7\70\0\1\u0233\21\0"+
"\1\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\5\7\1\u0234\25\7\2\0\2\7\11\0\1\7\5\0"+
"\1\u0235\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\5\7\1\u0236\25\7\2\0\1\7\23\0\1\u0237\164\0"+
"\1\u0238\13\0\1\7\11\0\1\7\5\0\1\u0239\2\0"+
"\10\7\5\0\13\7\1\0\33\7\2\0\1\7\100\0"+
"\1\u023a\11\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\3\7\1\u023b\27\7\2\0\1\7\31\0"+
"\1\u023c\105\0\1\u023d\64\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\23\7\1\u023e\7\7\2\0"+
"\1\7\73\0\1\u023f\11\0\1\u0240\70\0\1\u0241\103\0"+
"\1\u0242\134\0\1\u0243\74\0\1\u0244\120\0\1\u0245\103\0"+
"\1\u0246\24\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\14\7\1\u0247\16\7\2\0\2\7\11\0"+
"\1\7\10\0\10\7\4\0\1\u0248\13\7\1\0\33\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\3\7\1\u0249\20\7\1\u024a\6\7\2\0\1\7"+
"\20\0\1\u024b\71\0\1\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\1\7\1\u024c\31\7\2\0\2\7"+
"\11\0\1\7\5\0\1\u024d\2\0\10\7\5\0\13\7"+
"\1\0\33\7\2\0\1\7\73\0\1\u024e\11\0\1\u024f"+
"\70\0\1\u0250\111\0\1\u0251\120\0\1\u0252\16\0\1\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\22\7"+
"\1\u0253\10\7\2\0\1\7\104\0\1\u0254\63\0\1\u0255"+
"\114\0\1\u0256\115\0\1\u0257\55\0\1\u0258\156\0\1\u0259"+
"\114\0\1\u025a\103\0\1\u025b\116\0\1\u025c\103\0\1\u025d"+
"\115\0\1\u025e\112\0\1\u025f\105\0\1\u0260\102\0\1\u0261"+
"\47\0\1\u0262\152\0\1\u0263\26\0\1\7\11\0\1\7"+
"\5\0\1\u0264\2\0\10\7\5\0\13\7\1\0\33\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\24\7\1\u0265\6\7\2\0\2\7\11\0\1\7"+
"\5\0\1\u0266\2\0\10\7\5\0\13\7\1\0\33\7"+
"\2\0\1\7\72\0\1\u0267\17\0\1\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\5\7\1\u0156\25\7"+
"\2\0\1\7\73\0\1\u0268\11\0\1\u0269\70\0\1\u026a"+
"\103\0\1\u026b\117\0\1\u026c\120\0\1\u026d\103\0\1\u026e"+
"\124\0\1\u026f\11\0\1\7\11\0\1\7\5\0\1\u0270"+
"\2\0\10\7\5\0\13\7\1\0\33\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\6\7"+
"\1\u0271\24\7\2\0\1\7\100\0\1\u0263\11\0\1\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\17\7"+
"\1\u0272\13\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\24\7\1\u0125\6\7\2\0\1\7"+
"\102\0\1\u0273\7\0\1\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\5\7\1\u0274\25\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\23\7"+
"\1\u0101\7\7\2\0\2\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\14\7\1\u0155\16\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\4\0\1\u0275\13\7\1\0"+
"\33\7\2\0\1\7\100\0\1\u0276\71\0\1\u0277\121\0"+
"\1\u0278\113\0\1\u0279\115\0\1\u027a\74\0\1\u027b\107\0"+
"\1\u027c\132\0\1\u027d\70\0\1\u027e\16\0\1\u027f\70\0"+
"\1\u0280\112\0\1\u0281\134\0\1\u0282\71\0\1\u0283\50\0"+
"\1\u0284\172\0\1\u0285\71\0\1\u0286\130\0\1\u0287\31\0"+
"\1\u0288\164\0\1\u0289\16\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\24\7\1\u0101\6\7\2\0"+
"\1\7\56\0\1\u028a\110\0\1\u01fc\127\0\1\u028b\116\0"+
"\1\u028c\66\0\1\u028d\122\0\1\u028e\116\0\1\u028f\76\0"+
"\1\u028c\31\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\11\7\1\u0290\21\7\2\0\1\7\61\0"+
"\1\u0291\30\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\23\7\1\u0292\7\7\2\0\1\7\55\0"+
"\1\u0293\120\0\1\u0294\110\0\1\u0295\127\0\1\u0296\103\0"+
"\1\u0297\106\0\1\u0298\102\0\1\u0299\130\0\1\u029a\76\0"+
"\1\u029b\55\0\1\u029c\145\0\1\u029d\24\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\3\7\1\u029e"+
"\27\7\2\0\1\7\55\0\1\u029f\34\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\4\7\1\u02a0"+
"\26\7\2\0\1\7\100\0\1\u02a1\11\0\1\7\11\0"+
"\1\7\5\0\1\u02a2\2\0\10\7\5\0\13\7\1\0"+
"\33\7\2\0\1\7\104\0\1\u02a3\103\0\1\u02a4\105\0"+
"\1\u02a5\112\0\1\u02a6\16\0\1\7\11\0\1\7\5\0"+
"\1\u02a7\2\0\10\7\5\0\13\7\1\0\33\7\2\0"+
"\2\7\11\0\1\7\10\0\10\7\5\0\13\7\1\0"+
"\24\7\1\u02a8\6\7\2\0\1\7\73\0\1\u02a9\116\0"+
"\1\u02aa\66\0\1\u02ab\122\0\1\u02ac\110\0\1\u02ad\117\0"+
"\1\u02ae\106\0\1\u02af\101\0\1\u02b0\31\0\1\7\11\0"+
"\1\7\5\0\1\u02b1\2\0\10\7\5\0\13\7\1\0"+
"\33\7\2\0\1\7\23\0\1\u02b2\66\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\5\7\1\u02b3"+
"\25\7\2\0\2\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\11\7\1\u02b4\21\7\2\0\1\7\56\0"+
"\1\u02b5\1\u02b6\1\u0175\1\0\1\u0176\5\0\1\u0178\6\0"+
"\1\u02b7\12\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\3\7\1\u0155\27\7\2\0\1\7\57\0"+
"\1\u02b8\1\u02b9\4\0\1\u02ba\5\0\1\u02bb\1\u02bc\2\0"+
"\1\u02bd\2\0\1\u02be\102\0\1\u02bf\116\0\1\u02c0\66\0"+
"\1\u02c1\127\0\1\u02c2\36\0\1\u02c3\71\0\1\7\11\0"+
"\1\7\5\0\1\u02c4\2\0\10\7\5\0\13\7\1\0"+
"\33\7\2\0\1\7\57\0\1\u02c5\120\0\1\u02c6\122\0"+
"\1\u02c7\72\0\1\u02c8\124\0\1\u02c9\74\0\1\u02ca\110\0"+
"\1\u01e6\117\0\1\u025c\103\0\1\u02cb\134\0\1\u02cc\107\0"+
"\1\u02cd\33\0\1\u02ce\116\0\1\u02cf\42\0\1\u02d0\43\0"+
"\1\u02d1\166\0\1\u02d2\12\0\1\7\11\0\1\7\10\0"+
"\10\7\5\0\13\7\1\0\5\7\1\u02d3\25\7\2\0"+
"\1\7\73\0\1\u02d4\6\0\1\u02d5\1\u02d6\106\0\1\u02d7"+
"\104\0\1\u02d8\116\0\1\u02d9\66\0\1\u02da\122\0\1\u02db"+
"\116\0\1\u02dc\106\0\1\u02dd\101\0\1\u02de\53\0\1\u02df"+
"\152\0\1\u02e0\10\0\1\u02e1\15\0\1\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\20\7\1\u0101\12\7"+
"\2\0\2\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\16\7\1\u02e2\14\7\2\0\1\7\61\0\1\u02e3"+
"\30\0\1\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\16\7\1\u021a\14\7\2\0\1\7\23\0\1\u02e4"+
"\153\0\1\u02e5\101\0\1\u02e6\124\0\1\u02e7\114\0\1\u02e8"+
"\73\0\1\u02e9\124\0\1\u02ea\76\0\1\u02eb\120\0\1\u02ec"+
"\114\0\1\u02ed\77\0\1\u02ee\132\0\1\u02ef\70\0\1\u02f0"+
"\115\0\1\u02f1\122\0\1\u02f2\116\0\1\u02f3\73\0\1\u02f4"+
"\126\0\1\u02f5\22\0\1\u02f6\201\0\1\u02f7\112\0\1\u02f8"+
"\101\0\1\u02f9\111\0\1\u02fa\102\0\1\u0295\126\0\1\u0295"+
"\74\0\1\u02fb\126\0\1\u02fc\13\0\1\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\17\7\1\u02fd\13\7"+
"\2\0\1\7\72\0\1\u02fe\17\0\1\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\24\7\1\u02ff\6\7"+
"\2\0\1\7\77\0\1\u0300\67\0\1\u0301\112\0\1\u0302"+
"\110\0\1\u02fc\111\0\1\u0303\134\0\1\u0304\107\0\1\u0305"+
"\73\0\1\u0306\114\0\1\u0307\26\0\1\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\24\7\1\u0308\6\7"+
"\2\0\1\7\100\0\1\u0309\11\0\1\7\11\0\1\7"+
"\5\0\1\u030a\2\0\10\7\5\0\13\7\1\0\33\7"+
"\2\0\1\7\73\0\1\u030b\103\0\1\u030c\47\0\10\u030d"+
"\5\0\6\u030d\7\0\6\u030d\104\0\1\u030e\115\0\1\u030f"+
"\50\0\1\u0310\152\0\1\u011c\30\0\1\7\11\0\1\7"+
"\5\0\1\u0311\2\0\10\7\5\0\13\7\1\0\33\7"+
"\2\0\1\7\70\0\1\u0312\102\0\1\u0313\126\0\1\u0313"+
"\74\0\1\u0314\107\0\1\u0315\130\0\1\u0316\70\0\1\u0317"+
"\115\0\1\u0318\107\0\1\u0319\2\0\1\u031a\6\0\1\u031b"+
"\124\0\1\u031c\5\0\1\7\11\0\1\7\5\0\1\u031d"+
"\2\0\10\7\5\0\13\7\1\0\33\7\2\0\2\7"+
"\11\0\1\7\10\0\10\7\5\0\13\7\1\0\1\7"+
"\1\u031e\31\7\2\0\1\7\105\0\1\u031f\70\0\1\u0320"+
"\111\0\1\u0321\120\0\1\u0322\5\0\1\u0323\75\0\1\u0324"+
"\116\0\1\u0325\75\0\1\u0326\126\0\1\u0327\116\0\1\u0328"+
"\1\u0329\75\0\1\u032a\114\0\1\u032b\102\0\1\u032c\126\0"+
"\1\u032c\111\0\1\u032d\73\0\1\u0175\1\0\1\u0176\2\0"+
"\1\u0177\105\0\1\u032e\111\0\1\u032f\111\0\1\u0330\131\0"+
"\1\u0331\71\0\1\u0332\121\0\1\u0333\110\0\1\u0334\126\0"+
"\1\u0335\61\0\1\u0336\113\0\1\u0337\2\0\1\u0338\6\0"+
"\1\u0339\75\0\1\u033a\121\0\1\u033b\51\0\1\u02cf\167\0"+
"\1\u033c\6\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\4\7\1\u0101\26\7\2\0\1\7\56\0"+
"\1\u033d\126\0\1\u033e\103\0\1\u033f\46\0\1\u0340\157\0"+
"\1\u0341\102\0\1\u0342\126\0\1\u0342\74\0\1\u0343\126\0"+
"\1\u0344\70\0\1\u0345\115\0\1\u0346\55\0\1\u02cf\42\0"+
"\1\u0347\102\0\1\u0348\131\0\1\u0348\10\0\1\7\11\0"+
"\1\7\10\0\10\7\5\0\13\7\1\0\11\7\1\u0349"+
"\21\7\2\0\1\7\76\0\1\u034a\117\0\1\u034b\100\0"+
"\1\u034c\116\0\1\u034d\111\0\1\u034e\116\0\1\u034f\64\0"+
"\1\u0350\121\0\1\u0351\114\0\1\u0352\77\0\1\u0353\121\0"+
"\1\u0354\117\0\1\u0355\104\0\1\u0356\117\0\1\u0357\114\0"+
"\1\u0358\105\0\1\u0359\102\0\1\u035a\124\0\1\u035b\63\0"+
"\1\u035c\115\0\1\u0263\111\0\1\u035d\107\0\1\u02fc\132\0"+
"\1\u0295\11\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\16\7\1\u0101\14\7\2\0\1\7\63\0"+
"\1\u035e\126\0\1\u035f\103\0\1\u0360\107\0\1\u028c\120\0"+
"\1\u0361\76\0\1\u0362\112\0\1\u0363\105\0\1\u0364\114\0"+
"\1\u0365\25\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\17\7\1\u0366\13\7\2\0\1\7\62\0"+
"\1\u0367\126\0\1\u0368\32\0\1\u0369\163\0\1\u036a\124\0"+
"\1\u036b\107\0\1\u036c\66\0\1\u0175\4\0\1\u0177\2\0"+
"\1\u0178\112\0\1\u036d\101\0\1\u036e\107\0\1\u036f\121\0"+
"\1\u0370\122\0\1\u0313\111\0\1\u0371\31\0\1\u0372\161\0"+
"\1\u0373\106\0\1\u0374\105\0\1\u0375\53\0\10\u0376\5\0"+
"\6\u0376\7\0\6\u0376\112\0\1\u02e0\7\0\1\u0377\1\u02e1"+
"\15\0\1\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\24\7\1\u0378\6\7\2\0\1\7\100\0\1\u0334"+
"\66\0\1\u0379\127\0\1\u037a\110\0\1\u037b\116\0\1\u037c"+
"\110\0\1\u037d\112\0\1\u037e\1\u037f\77\0\1\u0380\113\0"+
"\1\u0381\76\0\1\u0382\130\0\1\u0383\113\0\1\u0384\74\0"+
"\1\u0385\50\0\1\u0386\171\0\1\u032c\103\0\1\u0387\11\0"+
"\1\u0388\101\0\1\u0389\74\0\1\u038a\122\0\1\u038b\43\0"+
"\1\u0174\113\0\1\u038c\146\0\1\u025c\122\0\1\u038d\107\0"+
"\1\u038e\106\0\1\u038f\105\0\1\u0390\110\0\1\u0391\131\0"+
"\1\u0392\76\0\1\u0393\112\0\1\u0394\110\0\1\u0395\104\0"+
"\1\u0396\121\0\1\u0347\102\0\1\u0397\107\0\1\u0398\132\0"+
"\1\u0342\111\0\1\u0399\31\0\1\u039a\156\0\1\u039b\124\0"+
"\1\u039c\11\0\1\7\11\0\1\7\10\0\10\7\5\0"+
"\13\7\1\0\32\7\1\u0265\2\0\1\7\65\0\1\u039d"+
"\47\0\10\u039e\5\0\6\u039e\7\0\6\u039e\121\0\1\u039f"+
"\74\0\1\u03a0\111\0\1\u03a1\54\0\1\u03a2\151\0\1\u03a3"+
"\111\0\1\u03a4\112\0\1\u03a5\122\0\1\u03a6\116\0\1\u03a7"+
"\77\0\1\u03a8\117\0\1\u03a9\103\0\1\u03aa\124\0\1\u03ab"+
"\66\0\1\u03ac\134\0\1\u03ad\74\0\1\u03ae\125\0\1\u035f"+
"\105\0\1\u03af\110\0\1\u03b0\41\0\1\u03b1\113\0\1\u03b2"+
"\65\0\1\7\11\0\1\7\10\0\10\7\5\0\13\7"+
"\1\0\22\7\1\u0101\10\7\2\0\1\7\73\0\1\u03b3"+
"\123\0\1\u03b4\66\0\1\u0176\2\0\1\u0177\2\0\1\u0178"+
"\111\0\1\u03b5\41\0\1\u03b6\111\0\1\u03b7\146\0\1\u03b8"+
"\111\0\1\u03b9\134\0\1\u0318\76\0\1\u03ba\121\0\1\u01cf"+
"\4\0\1\u01d1\64\0\1\u03bb\115\0\1\u03bc\130\0\1\u03bd"+
"\73\0\1\u0335\27\0\1\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\11\7\1\u03be\21\7\2\0\1\7"+
"\76\0\1\u025c\111\0\1\u01e6\112\0\1\u03bf\112\0\1\u03c0"+
"\72\0\1\u03c1\130\0\1\u03c2\72\0\1\u03c3\111\0\1\u03c4"+
"\135\0\1\u03c5\104\0\1\u03c6\72\0\1\u03c7\130\0\1\u03c8"+
"\66\0\1\u03c9\131\0\1\u01cf\114\0\1\u03ca\76\0\1\u03cb"+
"\124\0\1\u03cc\111\0\1\u0332\111\0\1\u03cd\42\0\1\u03ce"+
"\137\0\1\u03cf\107\0\1\u03d0\115\0\1\u03d1\130\0\1\u03d2"+
"\71\0\1\u03d3\55\0\1\u03d4\5\0\1\u03d5\157\0\1\u03d6"+
"\72\0\1\u03d7\110\0\1\u03d8\112\0\1\u03d9\105\0\1\u03da"+
"\134\0\1\u0346\76\0\1\u03db\126\0\1\u01f7\107\0\1\u03dc"+
"\73\0\1\u03dd\127\0\1\u03de\71\0\1\u03df\51\0\1\u03e0"+
"\111\0\1\u03e1\164\0\1\u03e2\116\0\1\u03e3\70\0\1\u03e4"+
"\132\0\1\u03e5\76\0\1\u03e6\116\0\1\u0295\103\0\1\u025c"+
"\106\0\1\u03e7\110\0\1\u03e8\114\0\1\u03e9\52\0\1\u022f"+
"\1\0\1\u03ea\35\0\1\u03eb\56\0\1\u03ec\156\0\1\u03ed"+
"\105\0\1\u03ee\104\0\1\u03ef\104\0\1\u03f0\106\0\1\u03f1"+
"\140\0\1\u03f2\77\0\1\u0313\107\0\1\u03f3\120\0\1\u03f4"+
"\102\0\1\u03f5\105\0\1\u03f6\25\0\1\7\11\0\1\7"+
"\10\0\10\7\5\0\13\7\1\0\17\7\1\u03f7\13\7"+
"\2\0\1\7\100\0\1\u03f8\104\0\1\u03f9\75\0\1\u03fa"+
"\107\0\1\u03fb\132\0\1\u03fc\72\0\1\u03fd\123\0\1\u03fe"+
"\105\0\1\u03ff\122\0\1\u0400\114\0\1\u0401\102\0\1\u032c"+
"\100\0\1\u0402\130\0\1\u03d9\76\0\1\u0403\44\0\1\u0404"+
"\152\0\1\u035f\127\0\1\u0405\102\0\1\u0406\105\0\1\u0407"+
"\123\0\1\u0408\44\0\1\u0409\137\0\1\u040a\111\0\1\u03cb"+
"\52\0\1\u040b\163\0\1\u0342\107\0\1\u040c\53\0\1\u03d5"+
"\144\0\1\u040d\111\0\1\u040e\101\0\1\u040f\133\0\1\u0410"+
"\111\0\1\u0411\112\0\1\u0412\70\0\1\u0413\107\0\1\u0414"+
"\113\0\1\u0415\125\0\1\u0416\36\0\1\u02a7\111\0\1\u0417"+
"\113\0\1\u0418\114\0\1\u0419\151\0\1\u041a\115\0\1\u041b"+
"\77\0\1\u041c\124\0\1\u041d\74\0\1\u041e\132\0\1\u041f"+
"\33\0\1\u0420\152\0\1\u0421\127\0\1\u0422\72\0\1\u0422"+
"\124\0\1\u03f5\16\0\1\7\11\0\1\7\10\0\10\7"+
"\5\0\13\7\1\0\16\7\1\u023b\14\7\2\0\1\7"+
"\76\0\1\u0423\104\0\1\u0424\120\0\1\u0425\103\0\1\u0426"+
"\101\0\1\u0427\127\0\1\u0428\104\0\1\u0429\75\0\1\u042a"+
"\52\0\1\u042b\1\0\1\u042c\144\0\1\u042d\132\0\1\u03d9"+
"\106\0\1\u042e\111\0\1\u02d4\7\0\1\u02d6\105\0\1\u02cd"+
"\72\0\1\u02cd\124\0\1\u0406\102\0\1\u042f\103\0\1\u0430"+
"\13\0\1\u0431\100\0\1\u0432\107\0\1\u0433\125\0\1\u0434"+
"\116\0\1\u0435\114\0\1\u0436\111\0\1\u0437\63\0\1\u0438"+
"\120\0\1\u0439\115\0\1\u043a\105\0\1\u043b\117\0\1\u043c"+
"\103\0\1\u043d\13\0\1\u043e\77\0\1\u043f\106\0\1\u0440"+
"\46\0\1\u0441\155\0\1\u0442\106\0\1\u0443\130\0\1\u0444"+
"\107\0\1\u0445\106\0\3\u0446\34\0\1\u0447\172\0\1\u0448"+
"\32\0\1\u0449\107\0\1\u044a\1\0\1\u042c\146\0\1\u044b"+
"\107\0\1\u044c\54\0\1\u044d\167\0\1\u044e\35\0\1\u042c"+
"\164\0\1\u044f\112\0\1\u0450\103\0\1\u0400\113\0\1\u03d9"+
"\74\0\1\u0451\127\0\1\u0452\73\0\1\u0453\126\0\1\u0454"+
"\74\0\1\u0455\121\0\1\u0456\111\0\1\u0457\124\0\1\u0458"+
"\66\0\1\u0459\141\0\1\u045a\105\0\1\u045b\74\0\1\u045c"+
"\102\0\1\u045d\132\0\1\u045e\35\0\1\u045f\144\0\1\u042f"+
"\111\0\1\u0460\111\0\1\u0461\113\0\1\u0462\130\0\1\u0463"+
"\74\0\1\u0464\111\0\1\u0465\107\0\1\u0466\117\0\1\u0467"+
"\120\0\1\u0468\116\0\1\u0469\65\0\1\u046a\132\0\1\u046b"+
"\33\0\1\u046c\171\0\1\u046d\111\0\1\u046e\111\0\1\u046f"+
"\76\0\1\u0470\116\0\1\u0471\113\0\1\u0472\106\0\1\u0473"+
"\44\0\1\u0474\161\0\1\u0475\110\0\1\u0476\114\0\1\u0477"+
"\120\0\1\u0478\63\0\1\u0479\132\0\1\u047a\66\0\1\u047b"+
"\125\0\1\u047c\101\0\1\u042a\122\0\1\u047d\103\0\1\u047e"+
"\112\0\1\u047f\116\0\1\u0480\115\0\1\u0481\71\0\1\u0430"+
"\120\0\1\u0482\103\0\1\u0483\111\0\1\u0484\125\0\1\u0485"+
"\76\0\1\u0486\112\0\1\u0487\106\0\1\u0488\64\0\1\u0489"+
"\145\0\1\u0446\116\0\1\u048a\74\0\1\u048b\56\0\1\u048c"+
"\146\0\1\u048d\125\0\1\u048e\110\0\1\u048f\113\0\1\u0490"+
"\102\0\1\u0491\111\0\1\u0492\103\0\1\u0493\115\0\1\u0494"+
"\113\0\1\u0495\117\0\1\u0496\102\0\1\u0497\111\0\1\u0498"+
"\117\0\1\u0499\107\0\1\u049a\114\0\1\u049b\103\0\1\u049c"+
"\116\0\1\u049d\115\0\1\u049e\74\0\1\u03d0\126\0\1\u0465"+
"\76\0\1\u049f\126\0\1\u04a0\111\0\1\u04a1\70\0\1\u04a2"+
"\121\0\1\u04a3\103\0\1\u04a4\111\0\1\u04a5\122\0\1\u04a6"+
"\100\0\1\u04a7\136\0\1\u04a8\66\0\1\u04a9\103\0\1\u04aa"+
"\115\0\1\u04ab\135\0\1\u04ac\26\0\1\u04ad\111\0\1\u04ae"+
"\111\0\1\u04af\165\0\1\u04b0\33\0\1\u04b1\152\0\1\u04b2"+
"\111\0\1\u04b3\122\0\1\u04b4\115\0\1\u04b5\111\0\1\u04b6"+
"\111\0\1\u04b7\74\0\1\u04b8\110\0\1\u04b9\114\0\1\u04ba"+
"\103\0\1\u04bb\111\0\1\u04bc\111\0\1\u04bd\132\0\1\u04be"+
"\74\0\1\u04bf\122\0\1\u04c0\111\0\1\u04c1\111\0\1\u04c2"+
"\115\0\1\u04c3\76\0\1\u04c4\111\0\1\u04c5\111\0\1\u04c6"+
"\121\0\1\u04c7\77\0\1\u04c8\111\0\1\u04c9\111\0\1\u04ca"+
"\126\0\1\u04cb\13\0";
private static int [] zzUnpackTrans() {
int [] result = new int[83324];
int offset = 0;
offset = zzUnpackTrans(ZZ_TRANS_PACKED_0, offset, result);
return result;
}
private static int zzUnpackTrans(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
value--;
do result[j++] = value; while (--count > 0);
}
return j;
}
/** Error code for "Unknown internal scanner error". */
private static final int ZZ_UNKNOWN_ERROR = 0;
/** Error code for "could not match input". */
private static final int ZZ_NO_MATCH = 1;
/** Error code for "pushback value was too large". */
private static final int ZZ_PUSHBACK_2BIG = 2;
/**
* Error messages for {@link #ZZ_UNKNOWN_ERROR}, {@link #ZZ_NO_MATCH}, and
* {@link #ZZ_PUSHBACK_2BIG} respectively.
*/
private static final String ZZ_ERROR_MSG[] = {
"Unknown internal scanner error",
"Error: could not match input",
"Error: pushback value was too large"
};
/**
* ZZ_ATTRIBUTE[aState] contains the attributes of state {@code aState}
*/
private static final int [] ZZ_ATTRIBUTE = zzUnpackAttribute();
private static final String ZZ_ATTRIBUTE_PACKED_0 =
"\1\0\2\11\5\1\3\11\4\1\4\11\27\1\3\11"+
"\1\0\1\11\1\0\2\11\1\1\12\0\1\1\1\11"+
"\1\1\2\11\76\1\21\0\1\1\1\0\1\1\1\0"+
"\1\11\31\1\1\0\15\1\1\0\3\1\1\0\27\1"+
"\27\0\1\1\1\0\10\1\1\0\17\1\3\0\4\1"+
"\1\0\7\1\5\0\1\1\2\0\10\1\1\0\3\1"+
"\1\0\12\1\5\0\1\11\3\0\1\11\13\0\1\1"+
"\3\0\7\1\2\0\1\1\1\0\5\1\1\0\3\1"+
"\1\0\2\1\1\0\3\1\1\0\2\1\2\0\1\1"+
"\14\0\5\1\1\0\1\1\2\0\3\1\1\0\10\1"+
"\2\0\1\1\1\11\7\0\1\11\1\0\1\1\2\0"+
"\1\11\6\0\1\11\1\1\7\0\1\1\1\0\1\1"+
"\7\0\1\1\1\0\3\1\2\0\1\1\1\0\1\1"+
"\2\0\1\1\1\11\7\0\3\1\1\0\2\1\4\0"+
"\1\1\16\0\1\1\1\0\3\1\1\0\1\1\7\0"+
"\2\1\1\0\2\1\1\0\4\1\15\0\2\11\4\0"+
"\1\11\2\0\1\1\10\0\1\1\1\0\1\1\12\0"+
"\1\11\1\0\1\1\1\0\1\1\1\0\1\1\4\0"+
"\1\1\2\11\1\1\10\0\1\1\1\0\2\1\1\0"+
"\1\1\6\0\1\1\4\0\1\11\3\0\1\11\6\0"+
"\1\1\1\0\1\1\11\0\1\1\1\0\2\1\1\0"+
"\1\1\33\0\1\1\1\0\1\1\2\0\1\11\6\0"+
"\1\11\1\0\1\1\1\0\1\1\7\0\1\1\12\0"+
"\2\1\30\0\1\11\5\0\1\1\3\0\1\1\12\0"+
"\1\1\4\0\1\11\11\0\1\11\2\0\1\11\10\0"+
"\1\1\1\0\1\1\10\0\1\1\4\0\1\1\5\0"+
"\1\11\4\0\1\1\5\0\1\1\15\0\1\1\5\0"+
"\1\1\2\0\1\11\14\0\1\11\3\0\1\1\2\0"+
"\1\1\6\0\2\11\2\0\1\11\4\0\1\11\5\0"+
"\1\11\4\0\1\1\1\0\1\1\11\0\1\11\5\0"+
"\1\1\1\0\1\1\43\0\1\11\1\0\1\1\2\11"+
"\2\0\1\11\3\0\1\11\26\0\1\1\16\0\1\1"+
"\1\11\6\0\1\11\2\0\1\1\1\11\17\0\1\1"+
"\2\0\1\11\12\0\1\1\10\0\1\1\7\0\2\11"+
"\17\0\1\11\7\0\1\11\2\0\2\1\4\0\1\1"+
"\4\0\1\11\2\0\1\11\6\0\4\11\6\0\1\1"+
"\2\0\1\11\15\0\2\11\17\0\1\11\22\0\1\1"+
"\16\0\1\11\1\0\1\11\15\0\3\11\1\0\1\11"+
"\6\0\2\1\1\11\2\0\1\11\3\0\2\11\3\0"+
"\1\11\2\0\1\11\5\0\1\11\5\0\1\11\10\0"+
"\4\11";
private static int [] zzUnpackAttribute() {
int [] result = new int[1227];
int offset = 0;
offset = zzUnpackAttribute(ZZ_ATTRIBUTE_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAttribute(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/** Input device. */
private java.io.Reader zzReader;
/** Current state of the DFA. */
private int zzState;
/** Current lexical state. */
private int zzLexicalState = YYINITIAL;
/**
* This buffer contains the current text to be matched and is the source of the {@link #yytext()}
* string.
*/
private char zzBuffer[] = new char[ZZ_BUFFERSIZE];
/** Text position at the last accepting state. */
private int zzMarkedPos;
/** Current text position in the buffer. */
private int zzCurrentPos;
/** Marks the beginning of the {@link #yytext()} string in the buffer. */
private int zzStartRead;
/** Marks the last character in the buffer, that has been read from input. */
private int zzEndRead;
/**
* Whether the scanner is at the end of file.
* @see #yyatEOF
*/
private boolean zzAtEOF;
/**
* The number of occupied positions in {@link #zzBuffer} beyond {@link #zzEndRead}.
*
* When a lead/high surrogate has been read from the input stream into the final
* {@link #zzBuffer} position, this will have a value of 1; otherwise, it will have a value of 0.
*/
private int zzFinalHighSurrogate = 0;
/** Number of newlines encountered up to the start of the matched text. */
private int yyline;
/** Number of characters from the last newline up to the start of the matched text. */
private int yycolumn;
/** Number of characters up to the start of the matched text. */
private long yychar;
/** Whether the scanner is currently at the beginning of a line. */
@SuppressWarnings("unused")
private boolean zzAtBOL = true;
/** Whether the user-EOF-code has already been executed. */
@SuppressWarnings("unused")
private boolean zzEOFDone;
/* user code: */
public final int getTokenStart() {
return (int) yychar;
}
public final int getTokenEnd() {
return getTokenStart() + yylength();
}
/**
* Creates a new scanner
*
* @param in the java.io.Reader to read input from.
*/
public SmaliLexer(java.io.Reader in) {
this.zzReader = in;
}
/**
* Translates raw input code points to DFA table row
*/
private static int zzCMap(int input) {
int offset = input & 255;
return offset == input ? ZZ_CMAP_BLOCKS[offset] : ZZ_CMAP_BLOCKS[ZZ_CMAP_TOP[input >> 8] | offset];
}
/**
* Refills the input buffer.
*
* @return {@code false} iff there was new input.
* @exception java.io.IOException if any I/O-Error occurs
*/
private boolean zzRefill() throws java.io.IOException {
/* first: make room (if you can) */
if (zzStartRead > 0) {
zzEndRead += zzFinalHighSurrogate;
zzFinalHighSurrogate = 0;
System.arraycopy(zzBuffer, zzStartRead,
zzBuffer, 0,
zzEndRead - zzStartRead);
/* translate stored positions */
zzEndRead -= zzStartRead;
zzCurrentPos -= zzStartRead;
zzMarkedPos -= zzStartRead;
zzStartRead = 0;
}
/* is the buffer big enough? */
if (zzCurrentPos >= zzBuffer.length - zzFinalHighSurrogate) {
/* if not: blow it up */
char newBuffer[] = new char[zzBuffer.length * 2];
System.arraycopy(zzBuffer, 0, newBuffer, 0, zzBuffer.length);
zzBuffer = newBuffer;
zzEndRead += zzFinalHighSurrogate;
zzFinalHighSurrogate = 0;
}
/* fill the buffer with new input */
int requested = zzBuffer.length - zzEndRead;
int numRead = zzReader.read(zzBuffer, zzEndRead, requested);
/* not supposed to occur according to specification of java.io.Reader */
if (numRead == 0) {
throw new java.io.IOException(
"Reader returned 0 characters. See JFlex examples/zero-reader for a workaround.");
}
if (numRead > 0) {
zzEndRead += numRead;
if (Character.isHighSurrogate(zzBuffer[zzEndRead - 1])) {
if (numRead == requested) { // We requested too few chars to encode a full Unicode character
--zzEndRead;
zzFinalHighSurrogate = 1;
} else { // There is room in the buffer for at least one more char
int c = zzReader.read(); // Expecting to read a paired low surrogate char
if (c == -1) {
return true;
} else {
zzBuffer[zzEndRead++] = (char)c;
}
}
}
/* potentially more input available */
return false;
}
/* numRead < 0 ==> end of stream */
return true;
}
/**
* Closes the input reader.
*
* @throws java.io.IOException if the reader could not be closed.
*/
public final void yyclose() throws java.io.IOException {
zzAtEOF = true; // indicate end of file
zzEndRead = zzStartRead; // invalidate buffer
if (zzReader != null) {
zzReader.close();
}
}
/**
* Resets the scanner to read from a new input stream.
*
*
Does not close the old reader.
*
*
All internal variables are reset, the old input stream cannot be reused (internal
* buffer is discarded and lost). Lexical state is set to {@code ZZ_INITIAL}.
*
*
Internal scan buffer is resized down to its initial length, if it has grown.
*
* @param reader The new input stream.
*/
public final void yyreset(java.io.Reader reader) {
zzReader = reader;
zzEOFDone = false;
yyResetPosition();
zzLexicalState = YYINITIAL;
if (zzBuffer.length > ZZ_BUFFERSIZE) {
zzBuffer = new char[ZZ_BUFFERSIZE];
}
}
/**
* Resets the input position.
*/
private final void yyResetPosition() {
zzAtBOL = true;
zzAtEOF = false;
zzCurrentPos = 0;
zzMarkedPos = 0;
zzStartRead = 0;
zzEndRead = 0;
zzFinalHighSurrogate = 0;
yyline = 0;
yycolumn = 0;
yychar = 0L;
}
/**
* Returns whether the scanner has reached the end of the reader it reads from.
*
* @return whether the scanner has reached EOF.
*/
public final boolean yyatEOF() {
return zzAtEOF;
}
/**
* Returns the current lexical state.
*
* @return the current lexical state.
*/
public final int yystate() {
return zzLexicalState;
}
/**
* Enters a new lexical state.
*
* @param newState the new lexical state
*/
public final void yybegin(int newState) {
zzLexicalState = newState;
}
/**
* Returns the text matched by the current regular expression.
*
* @return the matched text.
*/
public final String yytext() {
return new String(zzBuffer, zzStartRead, zzMarkedPos-zzStartRead);
}
/**
* Returns the character at the given position from the matched text.
*
*
It is equivalent to {@code yytext().charAt(pos)}, but faster.
*
* @param position the position of the character to fetch. A value from 0 to {@code yylength()-1}.
*
* @return the character at {@code position}.
*/
public final char yycharat(int position) {
return zzBuffer[zzStartRead + position];
}
/**
* How many characters were matched.
*
* @return the length of the matched text region.
*/
public final int yylength() {
return zzMarkedPos-zzStartRead;
}
/**
* Reports an error that occurred while scanning.
*
*
In a well-formed scanner (no or only correct usage of {@code yypushback(int)} and a
* match-all fallback rule) this method will only be called with things that
* "Can't Possibly Happen".
*
*
If this method is called, something is seriously wrong (e.g. a JFlex bug producing a faulty
* scanner etc.).
*
*
Usual syntax/scanner level error handling should be done in error fallback rules.
*
* @param errorCode the code of the error message to display.
*/
private static void zzScanError(int errorCode) {
String message;
try {
message = ZZ_ERROR_MSG[errorCode];
} catch (ArrayIndexOutOfBoundsException e) {
message = ZZ_ERROR_MSG[ZZ_UNKNOWN_ERROR];
}
throw new Error(message);
}
/**
* Pushes the specified amount of characters back into the input stream.
*
*
They will be read again by then next call of the scanning method.
*
* @param number the number of characters to be read again. This number must not be greater than
* {@link #yylength()}.
*/
public void yypushback(int number) {
if ( number > yylength() )
zzScanError(ZZ_PUSHBACK_2BIG);
zzMarkedPos -= number;
}
/**
* Resumes scanning until the next regular expression is matched, the end of input is encountered
* or an I/O-Error occurs.
*
* @return the next token.
* @exception java.io.IOException if any I/O-Error occurs.
*/
@NotNull
public SmaliToken advance() throws java.io.IOException {
int zzInput;
int zzAction;
// cached fields:
int zzCurrentPosL;
int zzMarkedPosL;
int zzEndReadL = zzEndRead;
char[] zzBufferL = zzBuffer;
int [] zzTransL = ZZ_TRANS;
int [] zzRowMapL = ZZ_ROWMAP;
int [] zzAttrL = ZZ_ATTRIBUTE;
while (true) {
zzMarkedPosL = zzMarkedPos;
yychar+= zzMarkedPosL-zzStartRead;
boolean zzR = false;
int zzCh;
int zzCharCount;
for (zzCurrentPosL = zzStartRead ;
zzCurrentPosL < zzMarkedPosL ;
zzCurrentPosL += zzCharCount ) {
zzCh = Character.codePointAt(zzBufferL, zzCurrentPosL, zzMarkedPosL);
zzCharCount = Character.charCount(zzCh);
switch (zzCh) {
case '\u000B': // fall through
case '\u000C': // fall through
case '\u0085': // fall through
case '\u2028': // fall through
case '\u2029':
yyline++;
yycolumn = 0;
zzR = false;
break;
case '\r':
yyline++;
yycolumn = 0;
zzR = true;
break;
case '\n':
if (zzR)
zzR = false;
else {
yyline++;
yycolumn = 0;
}
break;
default:
zzR = false;
yycolumn += zzCharCount;
}
}
if (zzR) {
// peek one character ahead if it is
// (if we have counted one line too much)
boolean zzPeek;
if (zzMarkedPosL < zzEndReadL)
zzPeek = zzBufferL[zzMarkedPosL] == '\n';
else if (zzAtEOF)
zzPeek = false;
else {
boolean eof = zzRefill();
zzEndReadL = zzEndRead;
zzMarkedPosL = zzMarkedPos;
zzBufferL = zzBuffer;
if (eof)
zzPeek = false;
else
zzPeek = zzBufferL[zzMarkedPosL] == '\n';
}
if (zzPeek) yyline--;
}
zzAction = -1;
zzCurrentPosL = zzCurrentPos = zzStartRead = zzMarkedPosL;
zzState = ZZ_LEXSTATE[zzLexicalState];
// set up zzAction for empty match case:
int zzAttributes = zzAttrL[zzState];
if ( (zzAttributes & 1) == 1 ) {
zzAction = zzState;
}
zzForAction: {
while (true) {
if (zzCurrentPosL < zzEndReadL) {
zzInput = Character.codePointAt(zzBufferL, zzCurrentPosL, zzEndReadL);
zzCurrentPosL += Character.charCount(zzInput);
}
else if (zzAtEOF) {
zzInput = YYEOF;
break zzForAction;
}
else {
// store back cached positions
zzCurrentPos = zzCurrentPosL;
zzMarkedPos = zzMarkedPosL;
boolean eof = zzRefill();
// get translated positions and possibly new buffer
zzCurrentPosL = zzCurrentPos;
zzMarkedPosL = zzMarkedPos;
zzBufferL = zzBuffer;
zzEndReadL = zzEndRead;
if (eof) {
zzInput = YYEOF;
break zzForAction;
}
else {
zzInput = Character.codePointAt(zzBufferL, zzCurrentPosL, zzEndReadL);
zzCurrentPosL += Character.charCount(zzInput);
}
}
int zzNext = zzTransL[ zzRowMapL[zzState] + zzCMap(zzInput) ];
if (zzNext == -1) break zzForAction;
zzState = zzNext;
zzAttributes = zzAttrL[zzState];
if ( (zzAttributes & 1) == 1 ) {
zzAction = zzState;
zzMarkedPosL = zzCurrentPosL;
if ( (zzAttributes & 8) == 8 ) break zzForAction;
}
}
}
// store back cached position
zzMarkedPos = zzMarkedPosL;
if (zzInput == YYEOF && zzStartRead == zzCurrentPos) {
zzAtEOF = true;
{
return SmaliToken.EOF;
}
}
else {
switch (zzAction < 0 ? zzAction : ZZ_ACTION[zzAction]) {
case 1:
{ return SmaliToken.BAD_CHARACTER;
}
// fall through
case 120: break;
case 2:
{ return SmaliToken.WHITESPACE;
}
// fall through
case 121: break;
case 3:
{ return SmaliToken.DOUBLE_QUOTED_STRING;
}
// fall through
case 122: break;
case 4:
{ return SmaliToken.LINE_COMMENT;
}
// fall through
case 123: break;
case 5:
{ return SmaliToken.IDENTIFIER;
}
// fall through
case 124: break;
case 6:
{ return SmaliToken.SINGLE_QUOTED_STRING;
}
// fall through
case 125: break;
case 7:
{ return SmaliToken.OPEN_PAREN;
}
// fall through
case 126: break;
case 8:
{ return SmaliToken.CLOSE_PAREN;
}
// fall through
case 127: break;
case 9:
{ return SmaliToken.COMMA;
}
// fall through
case 128: break;
case 10:
{ return SmaliToken.DOT;
}
// fall through
case 129: break;
case 11:
{ return SmaliToken.INTEGER_LITERAL;
}
// fall through
case 130: break;
case 12:
{ return SmaliToken.COLON;
}
// fall through
case 131: break;
case 13:
{ return SmaliToken.SEMICOLON;
}
// fall through
case 132: break;
case 14:
{ return SmaliToken.EQUAL;
}
// fall through
case 133: break;
case 15:
{ return SmaliToken.AT;
}
// fall through
case 134: break;
case 16:
{ return SmaliToken.PRIMITIVE_TYPE;
}
// fall through
case 135: break;
case 17:
{ return SmaliToken.VOID_TYPE;
}
// fall through
case 136: break;
case 18:
{ return SmaliToken.OPEN_BRACE;
}
// fall through
case 137: break;
case 19:
{ return SmaliToken.CLOSE_BRACE;
}
// fall through
case 138: break;
case 20:
{ return SmaliToken.ARROW;
}
// fall through
case 139: break;
case 21:
{ return SmaliToken.DOTDOT;
}
// fall through
case 140: break;
case 22:
{ return SmaliToken.DOUBLE_LITERAL;
}
// fall through
case 141: break;
case 23:
{ return SmaliToken.FLOAT_LITERAL;
}
// fall through
case 142: break;
case 24:
{ return SmaliToken.LONG_LITERAL;
}
// fall through
case 143: break;
case 25:
{ return SmaliToken.CLASS_TYPE;
}
// fall through
case 144: break;
case 26:
{ return SmaliToken.INSTRUCTION_FORMAT10x;
}
// fall through
case 145: break;
case 27:
{ return SmaliToken.INSTRUCTION_FORMAT23x;
}
// fall through
case 146: break;
case 28:
{ return SmaliToken.ACCESS_SPEC;
}
// fall through
case 147: break;
case 29:
{ return SmaliToken.INSTRUCTION_FORMAT10t;
}
// fall through
case 148: break;
case 30:
{ return SmaliToken.INSTRUCTION_FORMAT22c_FIELD;
}
// fall through
case 149: break;
case 31:
{ return SmaliToken.INSTRUCTION_FORMAT12x_OR_ID;
}
// fall through
case 150: break;
case 32:
{ return SmaliToken.NULL;
}
// fall through
case 151: break;
case 33:
{ return SmaliToken.INSTRUCTION_FORMAT21c_FIELD;
}
// fall through
case 152: break;
case 34:
{ return SmaliToken.TRUE;
}
// fall through
case 153: break;
case 35:
{ return SmaliToken.ENUM_DIRECTIVE;
}
// fall through
case 154: break;
case 36:
{ return SmaliToken.LINE_DIRECTIVE;
}
// fall through
case 155: break;
case 37:
{ return SmaliToken.ANNOTATION_VISIBILITY;
}
// fall through
case 156: break;
case 38:
{ return SmaliToken.INSTRUCTION_FORMAT31i_OR_ID;
}
// fall through
case 157: break;
case 39:
{ return SmaliToken.FALSE;
}
// fall through
case 158: break;
case 40:
{ return SmaliToken.INSTRUCTION_FORMAT22t;
}
// fall through
case 159: break;
case 41:
{ return SmaliToken.INSTRUCTION_FORMAT11x;
}
// fall through
case 160: break;
case 42:
{ return SmaliToken.CATCH_DIRECTIVE;
}
// fall through
case 161: break;
case 43:
{ return SmaliToken.CLASS_DIRECTIVE;
}
// fall through
case 162: break;
case 44:
{ return SmaliToken.FIELD_DIRECTIVE;
}
// fall through
case 163: break;
case 45:
{ return SmaliToken.LOCAL_DIRECTIVE;
}
// fall through
case 164: break;
case 46:
{ return SmaliToken.PARAMETER_DIRECTIVE;
}
// fall through
case 165: break;
case 47:
{ return SmaliToken.SUPER_DIRECTIVE;
}
// fall through
case 166: break;
case 48:
{ return SmaliToken.INSTRUCTION_FORMAT21t;
}
// fall through
case 167: break;
case 49:
{ return SmaliToken.LOCALS_DIRECTIVE;
}
// fall through
case 168: break;
case 50:
{ return SmaliToken.METHOD_DIRECTIVE;
}
// fall through
case 169: break;
case 51:
{ return SmaliToken.SOURCE_DIRECTIVE;
}
// fall through
case 170: break;
case 52:
{ return SmaliToken.INSTRUCTION_FORMAT11n;
}
// fall through
case 171: break;
case 53:
{ return SmaliToken.INSTRUCTION_FORMAT20t;
}
// fall through
case 172: break;
case 54:
{ return SmaliToken.INSTRUCTION_FORMAT30t;
}
// fall through
case 173: break;
case 55:
{ return SmaliToken.INSTRUCTION_FORMAT32x;
}
// fall through
case 174: break;
case 56:
{ return SmaliToken.INSTRUCTION_FORMAT21s;
}
// fall through
case 175: break;
case 57:
{ return SmaliToken.HIDDENAPI_RESTRICTION;
}
// fall through
case 176: break;
case 58:
{ return SmaliToken.VERIFICATION_ERROR_TYPE;
}
// fall through
case 177: break;
case 59:
{ return SmaliToken.INSTRUCTION_FORMAT22s_OR_ID;
}
// fall through
case 178: break;
case 60:
{ return SmaliToken.CATCHALL_DIRECTIVE;
}
// fall through
case 179: break;
case 61:
{ return SmaliToken.EPILOGUE_DIRECTIVE;
}
// fall through
case 180: break;
case 62:
{ return SmaliToken.PROLOGUE_DIRECTIVE;
}
// fall through
case 181: break;
case 63:
{ return SmaliToken.FIELD_OFFSET;
}
// fall through
case 182: break;
case 64:
{ return SmaliToken.INSTRUCTION_FORMAT22c_TYPE;
}
// fall through
case 183: break;
case 65:
{ return SmaliToken.END_FIELD_DIRECTIVE;
}
// fall through
case 184: break;
case 66:
{ return SmaliToken.END_LOCAL_DIRECTIVE;
}
// fall through
case 185: break;
case 67:
{ return SmaliToken.END_PARAMETER_DIRECTIVE;
}
// fall through
case 186: break;
case 68:
{ return SmaliToken.REGISTERS_DIRECTIVE;
}
// fall through
case 187: break;
case 69:
{ return SmaliToken.INSTRUCTION_FORMAT21c_TYPE;
}
// fall through
case 188: break;
case 70:
{ return SmaliToken.INSTRUCTION_FORMAT51l;
}
// fall through
case 189: break;
case 71:
{ return SmaliToken.INSTRUCTION_FORMAT22cs_FIELD;
}
// fall through
case 190: break;
case 72:
{ return SmaliToken.INLINE_INDEX;
}
// fall through
case 191: break;
case 73:
{ return SmaliToken.METHOD_HANDLE_TYPE_FIELD;
}
// fall through
case 192: break;
case 74:
{ return SmaliToken.VTABLE_INDEX;
}
// fall through
case 193: break;
case 75:
{ return SmaliToken.ANNOTATION_DIRECTIVE;
}
// fall through
case 194: break;
case 76:
{ return SmaliToken.ARRAY_DATA_DIRECTIVE;
}
// fall through
case 195: break;
case 77:
{ return SmaliToken.END_METHOD_DIRECTIVE;
}
// fall through
case 196: break;
case 78:
{ return SmaliToken.IMPLEMENTS_DIRECTIVE;
}
// fall through
case 197: break;
case 79:
{ return SmaliToken.INSTRUCTION_FORMAT22x;
}
// fall through
case 198: break;
case 80:
{ return SmaliToken.INSTRUCTION_FORMAT22b;
}
// fall through
case 199: break;
case 81:
{ return SmaliToken.INSTRUCTION_FORMAT21c_STRING;
}
// fall through
case 200: break;
case 82:
{ return SmaliToken.INSTRUCTION_FORMAT21ih;
}
// fall through
case 201: break;
case 83:
{ return SmaliToken.INSTRUCTION_FORMAT35c_METHOD;
}
// fall through
case 202: break;
case 84:
{ return SmaliToken.INSTRUCTION_FORMAT12x;
}
// fall through
case 203: break;
case 85:
{ return SmaliToken.INSTRUCTION_FORMAT22s;
}
// fall through
case 204: break;
case 86:
{ return SmaliToken.INSTRUCTION_FORMAT31i;
}
// fall through
case 205: break;
case 87:
{ return SmaliToken.INSTRUCTION_FORMAT22c_FIELD_ODEX;
}
// fall through
case 206: break;
case 88:
{ return SmaliToken.INSTRUCTION_FORMAT35c_CALL_SITE;
}
// fall through
case 207: break;
case 89:
{ return SmaliToken.INSTRUCTION_FORMAT35c_METHOD_OR_METHOD_HANDLE_TYPE;
}
// fall through
case 208: break;
case 90:
{ return SmaliToken.INSTRUCTION_FORMAT31t;
}
// fall through
case 209: break;
case 91:
{ return SmaliToken.INSTRUCTION_FORMAT21c_FIELD_ODEX;
}
// fall through
case 210: break;
case 92:
{ return SmaliToken.PACKED_SWITCH_DIRECTIVE;
}
// fall through
case 211: break;
case 93:
{ return SmaliToken.RESTART_LOCAL_DIRECTIVE;
}
// fall through
case 212: break;
case 94:
{ return SmaliToken.SPARSE_SWITCH_DIRECTIVE;
}
// fall through
case 213: break;
case 95:
{ return SmaliToken.SUBANNOTATION_DIRECTIVE;
}
// fall through
case 214: break;
case 96:
{ return SmaliToken.INSTRUCTION_FORMAT35mi_METHOD;
}
// fall through
case 215: break;
case 97:
{ return SmaliToken.END_ANNOTATION_DIRECTIVE;
}
// fall through
case 216: break;
case 98:
{ return SmaliToken.END_ARRAY_DATA_DIRECTIVE;
}
// fall through
case 217: break;
case 99:
{ return SmaliToken.METHOD_HANDLE_TYPE_METHOD;
}
// fall through
case 218: break;
case 100:
{ return SmaliToken.INSTRUCTION_FORMAT35c_TYPE;
}
// fall through
case 219: break;
case 101:
{ return SmaliToken.INSTRUCTION_FORMAT21c_METHOD_TYPE;
}
// fall through
case 220: break;
case 102:
{ return SmaliToken.INSTRUCTION_FORMAT21lh;
}
// fall through
case 221: break;
case 103:
{ return SmaliToken.END_PACKED_SWITCH_DIRECTIVE;
}
// fall through
case 222: break;
case 104:
{ return SmaliToken.END_SPARSE_SWITCH_DIRECTIVE;
}
// fall through
case 223: break;
case 105:
{ return SmaliToken.END_SUBANNOTATION_DIRECTIVE;
}
// fall through
case 224: break;
case 106:
{ return SmaliToken.INSTRUCTION_FORMAT31c;
}
// fall through
case 225: break;
case 107:
{ return SmaliToken.INSTRUCTION_FORMAT45cc_METHOD;
}
// fall through
case 226: break;
case 108:
{ return SmaliToken.INSTRUCTION_FORMAT35ms_METHOD;
}
// fall through
case 227: break;
case 109:
{ return SmaliToken.INSTRUCTION_FORMAT3rc_METHOD;
}
// fall through
case 228: break;
case 110:
{ return SmaliToken.INSTRUCTION_FORMAT21c_METHOD_HANDLE;
}
// fall through
case 229: break;
case 111:
{ return SmaliToken.INSTRUCTION_FORMAT3rc_CALL_SITE;
}
// fall through
case 230: break;
case 112:
{ return SmaliToken.INSTRUCTION_FORMAT35c_METHOD_ODEX;
}
// fall through
case 231: break;
case 113:
{ return SmaliToken.INSTRUCTION_FORMAT10x_ODEX;
}
// fall through
case 232: break;
case 114:
{ return SmaliToken.INSTRUCTION_FORMAT3rmi_METHOD;
}
// fall through
case 233: break;
case 115:
{ return SmaliToken.INSTRUCTION_FORMAT3rc_TYPE;
}
// fall through
case 234: break;
case 116:
{ return SmaliToken.INSTRUCTION_FORMAT3rc_METHOD_ODEX;
}
// fall through
case 235: break;
case 117:
{ return SmaliToken.INSTRUCTION_FORMAT4rcc_METHOD;
}
// fall through
case 236: break;
case 118:
{ return SmaliToken.INSTRUCTION_FORMAT3rms_METHOD;
}
// fall through
case 237: break;
case 119:
{ return SmaliToken.INSTRUCTION_FORMAT20bc;
}
// fall through
case 238: break;
default:
zzScanError(ZZ_NO_MATCH);
}
}
}
}
}