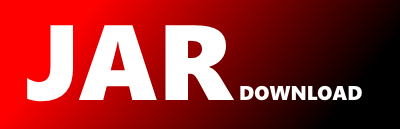
com.blade.kit.Assert Maven / Gradle / Ivy
package com.blade.kit;
import com.blade.exception.BladeException;
import java.util.concurrent.Callable;
/**
* Assert Kit
*
* @author biezhi
* 2017/5/31
*/
public class Assert {
public static void greaterThan(double num, double exp, String msg) {
if (num < exp) {
throw new IllegalArgumentException(msg);
}
}
public static void notNull(Object object, String msg) {
if (null == object) {
throw new IllegalArgumentException(msg);
}
}
public static void notEmpty(String str, String msg) {
if (null == str || "".equals(str)) {
throw new IllegalArgumentException(msg);
}
}
public static void notEmpty(T[] arr, String msg) {
if (null == arr || arr.length == 0) {
throw new IllegalArgumentException(msg);
}
}
public static T wrap(Callable callable) {
try {
return callable.call();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static void throwException(String msg) throws BladeException {
throw new BladeException(msg);
}
public static void throwException(Throwable t) throws BladeException {
throw new BladeException(t);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy