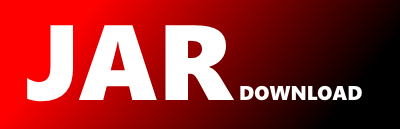
com.blazebit.expression.azure.subscription.api.TenantsApi Maven / Gradle / Ivy
The newest version!
package com.blazebit.expression.azure.subscription.api;
import com.blazebit.expression.azure.invoker.ApiException;
import com.blazebit.expression.azure.invoker.ApiClient;
import com.blazebit.expression.azure.invoker.ApiResponse;
import com.blazebit.expression.azure.invoker.Configuration;
import com.blazebit.expression.azure.invoker.Pair;
import jakarta.ws.rs.core.GenericType;
import com.blazebit.expression.azure.subscription.model.CheckResourceNameResult;
import com.blazebit.expression.azure.subscription.model.CloudError;
import com.blazebit.expression.azure.subscription.model.ResourceName;
import com.blazebit.expression.azure.subscription.model.TenantListResult;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-27T17:30:38.203241700+01:00[Europe/Berlin]", comments = "Generator version: 7.5.0")
public class TenantsApi {
private ApiClient apiClient;
public TenantsApi() {
this(Configuration.getDefaultApiClient());
}
public TenantsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Checks resource name validity
* A resource name is valid if it is not a reserved word, does not contains a reserved word and does not start with a reserved word
* @param apiVersion The API version to use for this operation. (required)
* @param resourceNameDefinition Resource object with values for resource name and resource type (optional)
* @return CheckResourceNameResult
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 OK - Returns status as allowed or not. -
0 Error response describing why the operation failed. -
*/
public CheckResourceNameResult checkResourceName(String apiVersion, ResourceName resourceNameDefinition) throws ApiException {
return checkResourceNameWithHttpInfo(apiVersion, resourceNameDefinition).getData();
}
/**
* Checks resource name validity
* A resource name is valid if it is not a reserved word, does not contains a reserved word and does not start with a reserved word
* @param apiVersion The API version to use for this operation. (required)
* @param resourceNameDefinition Resource object with values for resource name and resource type (optional)
* @return ApiResponse<CheckResourceNameResult>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 OK - Returns status as allowed or not. -
0 Error response describing why the operation failed. -
*/
public ApiResponse checkResourceNameWithHttpInfo(String apiVersion, ResourceName resourceNameDefinition) throws ApiException {
// Check required parameters
if (apiVersion == null) {
throw new ApiException(400, "Missing the required parameter 'apiVersion' when calling checkResourceName");
}
// Query parameters
List localVarQueryParams = new ArrayList<>(
apiClient.parameterToPairs("", "api-version", apiVersion)
);
String localVarAccept = apiClient.selectHeaderAccept("application/json");
String localVarContentType = apiClient.selectHeaderContentType("application/json");
String[] localVarAuthNames = new String[] {"azure_auth"};
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("TenantsApi.checkResourceName", "/providers/Microsoft.Resources/checkResourceName", "POST", localVarQueryParams, resourceNameDefinition,
new LinkedHashMap<>(), new LinkedHashMap<>(), new LinkedHashMap<>(), localVarAccept, localVarContentType,
localVarAuthNames, localVarReturnType, false);
}
/**
*
* Gets the tenants for your account.
* @param apiVersion The API version to use for this operation. (required)
* @return TenantListResult
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 OK - Returns an array of tenants. -
0 Error response describing why the operation failed. -
*/
public TenantListResult tenantsList(String apiVersion) throws ApiException {
return tenantsListWithHttpInfo(apiVersion).getData();
}
/**
*
* Gets the tenants for your account.
* @param apiVersion The API version to use for this operation. (required)
* @return ApiResponse<TenantListResult>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 OK - Returns an array of tenants. -
0 Error response describing why the operation failed. -
*/
public ApiResponse tenantsListWithHttpInfo(String apiVersion) throws ApiException {
// Check required parameters
if (apiVersion == null) {
throw new ApiException(400, "Missing the required parameter 'apiVersion' when calling tenantsList");
}
// Query parameters
List localVarQueryParams = new ArrayList<>(
apiClient.parameterToPairs("", "api-version", apiVersion)
);
String localVarAccept = apiClient.selectHeaderAccept("application/json");
String localVarContentType = apiClient.selectHeaderContentType();
String[] localVarAuthNames = new String[] {"azure_auth"};
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("TenantsApi.tenantsList", "/tenants", "GET", localVarQueryParams, null,
new LinkedHashMap<>(), new LinkedHashMap<>(), new LinkedHashMap<>(), localVarAccept, localVarContentType,
localVarAuthNames, localVarReturnType, false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy