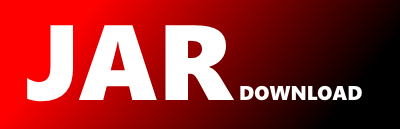
com.blazebit.persistence.view.change.MapChangeModel Maven / Gradle / Ivy
/*
* Copyright 2014 - 2019 Blazebit.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.blazebit.persistence.view.change;
import com.blazebit.persistence.view.metamodel.MapAttribute;
import com.blazebit.persistence.view.metamodel.PluralAttribute;
import com.blazebit.persistence.view.metamodel.SingularAttribute;
import java.util.List;
import java.util.Map;
/**
* An interface for accessing the dirty state of an object.
*
* @param The key type of the map represented by the change model
* @param The element type of the map represented by the change model
* @author Christian Beikov
* @since 1.2.0
*/
public interface MapChangeModel extends PluralChangeModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy