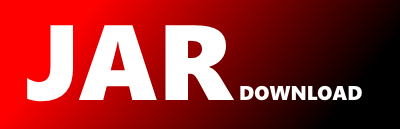
com.blazebit.persistence.view.change.PluralChangeModel Maven / Gradle / Ivy
/*
* Copyright 2014 - 2019 Blazebit.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.blazebit.persistence.view.change;
import com.blazebit.persistence.view.metamodel.MapAttribute;
import com.blazebit.persistence.view.metamodel.PluralAttribute;
import com.blazebit.persistence.view.metamodel.SingularAttribute;
import java.util.List;
/**
* An interface for accessing the dirty state of an object.
*
* @param The plural container type of represented by the change model
* @param The element type of the container represented by the change model
* @author Christian Beikov
* @since 1.2.0
*/
public interface PluralChangeModel extends ChangeModel {
/**
* Returns the change models of all added, removed or mutated elements.
*
* @return The dirty change models
*/
public List> getElementChanges();
/**
* Returns the change models of all added elements.
*
* @return The dirty change models
*/
public List> getAddedElements();
/**
* Returns the change models of all removed elements.
*
* @return The dirty change models
*/
public List> getRemovedElements();
/**
* Returns the change models of all mutated elements.
*
* @return The dirty change models
*/
public List> getMutatedElements();
/**
* Returns the change models for the elements attribute.
*
* @param attributePath The name of the attribute or path to sub-attribute
* @param The element type of the singular attribute
* @return The change model for the attribute
* @throws IllegalStateException if invoked on a change model that corresponds to a basic attribute
* @throws IllegalArgumentException if attribute of the given name does not otherwise exist
*/
public List extends ChangeModel> get(String attributePath);
/**
* Returns the change models for the elements attribute.
*
* @param attribute The singular attribute
* @param The element type of the singular attribute
* @return The change model for the attribute
*/
public List> get(SingularAttribute attribute);
/**
* Returns the change models for the elements attribute.
*
* @param attribute The collection attribute
* @param The container type of the plural attribute
* @param The value type of the plural attribute
* @return The change model for the attribute
*/
public > List> get(PluralAttribute attribute);
/**
* Returns the change models for the elements attribute.
*
* @param attribute The map attribute
* @param The key type of the map attribute
* @param The value type of the map attribute
* @return The change model for the attribute
*/
public List> get(MapAttribute attribute);
/**
* Returns whether the change model for the attribute is dirty.
* Essentially, this is a more efficient short-hand for get(attributePath).isDirty()
.
*
* @param attributePath The name of the attribute or path to sub-attribute
* @return True if the change model for the attribute path is dirty, false otherwise
* @throws IllegalStateException if invoked on a change model that corresponds to a basic attribute
* @throws IllegalArgumentException if attribute of the given name does not otherwise exist
*/
public boolean isDirty(String attributePath);
/**
* Returns whether the target attribute path was changed by updating or mutating it,
* but still has the same identity regarding parent objects it is contained in.
* If any of the parent objects as denoted by the attribute path are updated i.e. the identity was changed,
* this returns true
. Mutations or updates to the target object also cause true
to be returned.
* This method always returns true
when the collection was altered i.e. objects removed or added.
* In all other cases, this method returns false
.
*
* @param attributePath The name of the attribute or path to sub-attribute
* @return True if the attribute was changed, false otherwise
* @throws IllegalStateException if invoked on a change model that corresponds to a basic attribute
* @throws IllegalArgumentException if attribute of the given name does not otherwise exist
*/
public boolean isChanged(String attributePath);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy