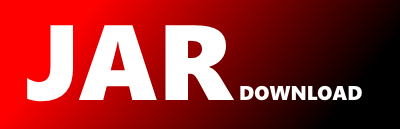
com.blazebit.persistence.testsuite.base.AbstractJpaPersistenceTest Maven / Gradle / Ivy
/*
* Copyright 2014 Blazebit.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.blazebit.persistence.testsuite.base;
import com.blazebit.persistence.Criteria;
import com.blazebit.persistence.CriteriaBuilderFactory;
import com.blazebit.persistence.spi.CriteriaBuilderConfiguration;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.net.URL;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.logging.LogManager;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.PersistenceException;
import javax.persistence.spi.PersistenceProvider;
import javax.persistence.spi.PersistenceProviderResolver;
import javax.persistence.spi.PersistenceProviderResolverHolder;
import javax.persistence.spi.PersistenceUnitInfo;
import javax.persistence.spi.PersistenceUnitTransactionType;
import org.junit.After;
import org.junit.Before;
import org.junit.BeforeClass;
/**
*
* @author Christian Beikov
* @since 1.0
*/
public abstract class AbstractJpaPersistenceTest {
protected EntityManager em;
protected CriteriaBuilderFactory cbf;
@BeforeClass
public static void initLogging() {
try {
LogManager.getLogManager().readConfiguration(AbstractJpaPersistenceTest.class.getResourceAsStream(
"/logging.properties"));
} catch (Exception e) {
e.printStackTrace(System.err);
}
}
@Before
public void init() {
EntityManagerFactory factory = createEntityManagerFactory("TestsuiteBase", createProperties("drop-and-create"));
em = factory.createEntityManager();
CriteriaBuilderConfiguration config = Criteria.getDefault();
config = configure(config);
cbf = config.createCriteriaBuilderFactory(factory);
}
@After
public void destruct() {
EntityManagerFactory factory = em.getEntityManagerFactory();
// NOTE: We need to close the entity manager or else we could run into a deadlock on some dbms platforms
// I am looking at you MySQL..
em.close();
factory.close();
// Drop the tables again with the same persistence unit
factory = createEntityManagerFactory("TestsuiteBase", createProperties("drop"));
factory.close();
}
private Properties createProperties(String dbAction) {
Properties properties = new Properties();
properties.put("javax.persistence.jdbc.url", System.getProperty("jdbc.url"));
properties.put("javax.persistence.jdbc.user", System.getProperty("jdbc.user", ""));
properties.put("javax.persistence.jdbc.password", System.getProperty("jdbc.password", ""));
properties.put("javax.persistence.jdbc.driver", System.getProperty("jdbc.driver"));
properties.put("javax.persistence.sharedCache.mode", "NONE");
properties.put("javax.persistence.schema-generation.database.action", dbAction);
properties = applyProperties(properties);
return properties;
}
protected abstract Class>[] getEntityClasses();
protected CriteriaBuilderConfiguration configure(CriteriaBuilderConfiguration config) {
return config;
}
protected Properties applyProperties(Properties properties) {
return properties;
}
private EntityManagerFactory createEntityManagerFactory(String persistenceUnitName, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy