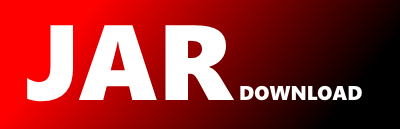
com.bigdata.bfs.BlockIdentifierIterator Maven / Gradle / Ivy
package com.bigdata.bfs;
import java.util.Iterator;
import com.bigdata.btree.ITuple;
import com.bigdata.btree.ITupleIterator;
import com.bigdata.btree.keys.KeyBuilder;
import com.bigdata.util.Bytes;
/**
* Extracts the block identifier from the key.
*
* @author Bryan Thompson
* @version $Id$
*/
public class BlockIdentifierIterator implements Iterator {
final private String id;
final private int version;
final private ITupleIterator src;
public String getId() {
return id;
}
public int getVersion() {
return version;
}
public BlockIdentifierIterator(String id, int version, ITupleIterator src) {
if (id == null)
throw new IllegalArgumentException();
if (src == null)
throw new IllegalArgumentException();
this.id = id;
this.version = version;
this.src = src;
}
public boolean hasNext() {
return src.hasNext();
}
public Long next() {
ITuple tuple = src.next();
byte[] key = tuple.getKey();
long block = KeyBuilder.decodeLong(key, key.length
- Bytes.SIZEOF_LONG);
return block;
}
/**
* Removes the last visited block for the file version.
*/
public void remove() {
src.remove();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy