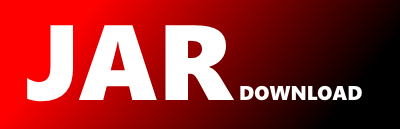
com.bigdata.btree.ILeafCursor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bigdata-core Show documentation
Show all versions of bigdata-core Show documentation
Blazegraph(TM) DB Core Platform. It contains all Blazegraph DB dependencies other than Blueprints.
package com.bigdata.btree;
/**
* Leaf cursor interface.
*
* @author Bryan Thompson
* @version $Id$
*/
public interface ILeafCursor extends Cloneable {
/**
* The backing B+Tree.
*/
AbstractBTree getBTree();
/**
* The current leaf (always defined).
*/
L leaf();
/**
* Return the first leaf.
*/
L first();
/**
* Return the last leaf.
*/
L last();
/**
* Find the leaf that would span the key.
*
* @param key
* The key
*
* @return The leaf which would span that key and never null
.
*
* @throws IllegalArgumentException
* if the key is null
.
*/
L seek(byte[] key);
/**
* Position this cursor on the same leaf as the given cursor.
*
* @param src
* A cursor.
*
* @return The leaf on which the given cursor was positioned.
*
* @throws IllegalArgumentException
* if the argument is null
* @throws IllegalArgumentException
* if the argument is a cursor for a different
* {@link AbstractBTree}.
*/
L seek(ILeafCursor src);
/**
* Clone the cursor.
*/
ILeafCursor clone();
/**
* Return the previous leaf in the natural order of the B+Tree. The
* cursor position is unchanged if there is no prececessor.
*
* @return The previous leaf -or- null
iff there is no
* previous leaf.
*/
L prior();
/**
* Return the next leaf in the natural order of the B+Tree. The cursor
* position is unchanged if there is no successor.
*
* @return The next leaf -or- null
iff there is no next
* leaf.
*/
L next();
}