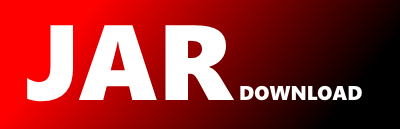
com.bigdata.btree.data.IAbstractNodeDataCoder Maven / Gradle / Ivy
/*
Copyright (C) SYSTAP, LLC DBA Blazegraph 2006-2016. All rights reserved.
Contact:
SYSTAP, LLC DBA Blazegraph
2501 Calvert ST NW #106
Washington, DC 20008
[email protected]
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; version 2 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
/*
* Created on Aug 28, 2009
*/
package com.bigdata.btree.data;
import java.io.Serializable;
import com.bigdata.io.AbstractFixedByteArrayBuffer;
import com.bigdata.io.DataOutputBuffer;
/**
* Interface for coding (compressing) an {@link INodeData} or {@link ILeafData}
* onto a byte[].
*
* @author Bryan Thompson
* @version $Id$
*/
public interface IAbstractNodeDataCoder extends
Serializable {
/**
* Return true
if this implementation can code data records for
* B+Tree nodes.
*
* @see INodeData
*/
boolean isNodeDataCoder();
/**
* Return true
if this implementation can code data records
* for B+Tree leaves.
*
* @see ILeafData
*/
boolean isLeafDataCoder();
/**
* Encode the data, returning a slice containing the coded data.
*
* Note: Implementations of this method are typically heavy. While it is
* always valid to {@link #encode(IAbstractNodeData, DataOutputBuffer)} an
* {@link IAbstractNodeData}, DO NOT invoke this arbitrarily on
* data which may already be coded. The {@link IAbstractNodeCodedData}
* interface will always be implemented for coded data.
*
* @param node
* The node or leaf data.
* @param buf
* A buffer on which the coded data will be written.
*
* @return A slice onto the post-condition state of the caller's buffer
* whose view corresponds to the coded record. This may be written
* directly onto an output stream or the slice may be converted to
* an exact fit byte[].
*
* @throws UnsupportedOperationException
* if {@link IAbstractNodeData#isLeaf()} is true
* and this {@link IAbstractNodeDataCoder} can not code B+Tree
* {@link ILeafData} records.
*
* @throws UnsupportedOperationException
* if {@link IAbstractNodeData#isLeaf()} is false
* and this {@link IAbstractNodeDataCoder} can not code B+Tree
* {@link INodeData} records.
*/
AbstractFixedByteArrayBuffer encode(T node, DataOutputBuffer buf);
/**
* Encode the data, returning a reference to a coded instance of the data.
*
* Note: Implementations of this method are typically heavy. While it is
* always valid to {@link #encode(IAbstractNodeData, DataOutputBuffer)} an
* {@link IAbstractNodeData}, DO NOT invoke this arbitrarily on
* data which may already be coded. The {@link IAbstractNodeCodedData}
* interface will always be implemented for coded data.
*
* @param node
* The node or leaf data.
* @param buf
* A buffer on which the coded data will be written.
*
* @return A reference to a coded instance of the data.
* {@link IAbstractNodeData#data()} is a slice onto the
* post-condition state of the caller's buffer whose view
* corresponds to the coded record. This may be written directly
* onto an output stream or the slice may be converted to an exact
* fit byte[].
*
* @throws UnsupportedOperationException
* if {@link IAbstractNodeData#isLeaf()} is true
* and this {@link IAbstractNodeDataCoder} can not code B+Tree
* {@link ILeafData} records.
*
* @throws UnsupportedOperationException
* if {@link IAbstractNodeData#isLeaf()} is false
* and this {@link IAbstractNodeDataCoder} can not code B+Tree
* {@link INodeData} records.
*/
T encodeLive(T node, DataOutputBuffer buf);
/**
* Return an {@link IAbstractNodeData} instance which can access the coded
* data.
*
* @param data
* The record containing the coded data.
*
* @return A view of the coded data.
*/
T decode(AbstractFixedByteArrayBuffer data);
}