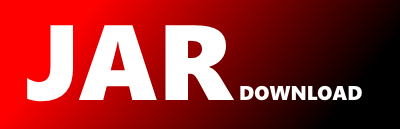
com.bigdata.counters.render.XHTMLRenderer Maven / Gradle / Ivy
package com.bigdata.counters.render;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.StringWriter;
import java.io.Writer;
import java.lang.reflect.Field;
import java.text.DateFormat;
import java.text.Format;
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.regex.Pattern;
import org.apache.log4j.Logger;
import com.bigdata.counters.CounterSet;
import com.bigdata.counters.HistoryInstrument;
import com.bigdata.counters.ICounter;
import com.bigdata.counters.ICounterNode;
import com.bigdata.counters.ICounterSet;
import com.bigdata.counters.IHistoryEntry;
import com.bigdata.counters.IServiceCounters;
import com.bigdata.counters.PeriodEnum;
import com.bigdata.counters.query.CSet;
import com.bigdata.counters.query.CounterSetSelector;
import com.bigdata.counters.query.HistoryTable;
import com.bigdata.counters.query.ICounterSelector;
import com.bigdata.counters.query.PivotTable;
import com.bigdata.counters.query.ReportEnum;
import com.bigdata.counters.query.TimestampFormatEnum;
import com.bigdata.counters.query.URLQueryModel;
import com.bigdata.counters.query.URLQueryParam;
import com.bigdata.service.Event;
import com.bigdata.service.IEventReportingService;
import com.bigdata.util.HTMLUtility;
/**
* (X)HTML rendering of a {@link CounterSet}.
*
* @todo UI widgets for regex filters, depth, correlated.
*
* @todo make documentation available on the counters via click through on their
* name.
*
* @author Bryan Thompson
*/
public class XHTMLRenderer implements IRenderer {
final static private Logger log = Logger.getLogger(XHTMLRenderer.class);
final public static String ps = ICounterSet.pathSeparator;
static final private String encoding = "UTF-8";
/*
* Note: the page is valid for any of these doctypes.
*/
// final private DoctypeEnum doctype = DoctypeEnum.html_4_01_strict
// final private DoctypeEnum doctype = DoctypeEnum.html_4_01_transitional
static final private DoctypeEnum doctype = DoctypeEnum.xhtml_1_0_strict;
/**
* Describes the state of the controller.
*/
private final URLQueryModel model;
/**
* Selects the counters to be rendered.
*/
private final ICounterSelector counterSelector;
/**
* @param model
* Describes the state of the controller (e.g., as parsed from
* the URL query parameters).
* @param counterSelector
* Selects the counters to be rendered.
*/
public XHTMLRenderer(final URLQueryModel model,
final ICounterSelector counterSelector) {
if (model == null)
throw new IllegalArgumentException();
if (counterSelector == null)
throw new IllegalArgumentException();
this.model = model;
this.counterSelector = counterSelector;
}
/**
* @param w
* @throws IOException
*/
@Override
public void render(final Writer w) throws IOException {
writeXmlDecl(w);
writeDocType(w);
writeHtml(w);
writeHead(w);
writeBody(w);
w.write("