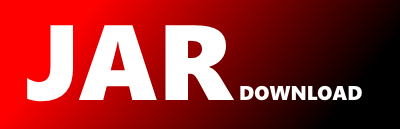
com.bigdata.relation.accesspath.AbstractElementBuffer Maven / Gradle / Ivy
Show all versions of bigdata-core Show documentation
package com.bigdata.relation.accesspath;
import com.bigdata.relation.IMutableRelation;
import com.bigdata.relation.IRelation;
import com.bigdata.striterator.ChunkedArrayIterator;
import com.bigdata.striterator.IChunkedOrderedIterator;
import com.bigdata.striterator.IKeyOrder;
/**
* Base class for {@link IBuffer} of {@link IRelation} elements whose target is
* a mutation (insert, delete, or update) of some {@link IMutableRelation}.
*
* @author Bryan Thompson
* @version $Id$
*
* @param
* The generic type of the [R]elation elements.
*
* @see IMutableRelation
*/
abstract public class AbstractElementBuffer extends AbstractArrayBuffer {
private final IMutableRelation relation;
private final IKeyOrder keyOrder;
protected IMutableRelation getRelation() {
return relation;
}
/**
* The natural order in which the elements will appear in the buffer -or-
* null
if you do not have a strong guarentee for
* that order (from the ctor).
*/
protected IKeyOrder getKeyOrder() {
return keyOrder;
}
/**
* @param capacity
* The buffer capacity.
* @param relation
* The target relation.
* @param keyOrder
* The natural order in which the elements will appear in the
* buffer -or- null
if you do not have a
* strong guarantee for that order.
* @param filter
* An optional filter for keeping elements out of the buffer.
*/
protected AbstractElementBuffer(final int capacity,
final IMutableRelation relation, final IKeyOrder keyOrder,
final IElementFilter filter) {
super(capacity, relation.getElementClass(), filter);
// if (relation == null)
// throw new IllegalArgumentException();
this.relation = relation;
this.keyOrder = keyOrder; // MAY be null.
}
/**
* Delegates to {@link #flush(IChunkedOrderedIterator)}
*/
@Override
final protected long flush(final int n, final R[] a) {
final IChunkedOrderedIterator itr = new ChunkedArrayIterator(n,
a, null/* keyOrder(unknown) */);
return flush(itr);
}
/**
* Concrete implementations must process the elements, causing the
* appropriate mutation on the target {@link IRelation}.
*
* Note: The elements generally appear in an arbitrary order and need to be
* sorted into ordered chunks using {@link IKeyOrder#getComparator()} for
* the desired natural order(s).
*
* @param itr
*
* @return The #of elements that were modified in the backing relation when
* the buffer was flushed
*/
abstract protected long flush(IChunkedOrderedIterator itr);
/**
* Buffer writes on {@link IMutableRelation#insert(IChunkedOrderedIterator)}
* when it is {@link #flush() flushed}.
*
* @author Bryan Thompson
* @version $Id$
* @param
*/
public static class InsertBuffer extends AbstractElementBuffer {
// /**
// * Ctor variant when the element will be written into the buffer in an
// * unknown order.
// *
// * @param capacity
// * @param relation
// */
// public InsertBuffer(int capacity, IMutableRelation relation) {
//
// this(capacity, relation,null/*keyOrder*/);
//
// }
/**
* Ctor variant used when you have a strong guarantee of the
* order in which the elements will be written into the buffer.
*
* @param capacity
* @param relation
* @param keyOrder
* @param filter
*/
public InsertBuffer(int capacity, IMutableRelation relation,
IKeyOrder keyOrder, IElementFilter filter) {
super(capacity, relation, keyOrder, filter);
}
@Override
protected long flush(IChunkedOrderedIterator itr) {
return getRelation().insert(itr);
}
}
/**
* Buffer writes on {@link IMutableRelation#delete(IChunkedOrderedIterator)}
* when it is {@link #flush() flushed}.
*
* @author Bryan Thompson
* @version $Id$
* @param
*/
public static class DeleteBuffer extends AbstractElementBuffer {
// /**
// * Ctor variant when the element will be written into the buffer in an
// * unknown order.
// *
// * @param capacity
// * @param relation
// */
// protected DeleteBuffer(int capacity, IMutableRelation relation) {
//
// this(capacity,relation,null/*keyOrder*/);
//
// }
/**
* Ctor variant used when you have a strong guarantee of the
* order in which the elements will be written into the buffer.
*
* @param capacity
* @param relation
* @param keyOrder
*/
protected DeleteBuffer(int capacity, IMutableRelation relation,
IKeyOrder keyOrder, IElementFilter filter) {
super(capacity, relation, keyOrder, filter);
}
@Override
protected long flush(IChunkedOrderedIterator itr) {
return getRelation().delete(itr);
}
}
// /**
// * Buffer writes on
// * {@link IMutableRelation#update(IChunkedIterator, ITransform)} when it is
// * {@link #flush() flushed}.
// *
// * @author Bryan Thompson
// * @version $Id$
// * @param
// */
// public static class UpdateBuffer extends AbstractElementBuffer {
//
// private final ITransform transform;
//
// /**
// * Ctor variant when the element will be written into the buffer in an
// * unknown order.
// *
// * @param capacity
// * @param relation
// * @param transform
// */
// protected UpdateBuffer(int capacity, IMutableRelation relation, ITransform transform) {
//
// this(capacity, relation, transform, null/*keyOrder*/);
//
// }
//
// /**
// * Ctor variant used when you have a strong guarantee of the
// * order in which the elements will be written into the buffer.
// *
// * @param capacity
// * @param relation
// * @param transform
// * @parma keyOrder
// */
// protected UpdateBuffer(int capacity, IMutableRelation relation,
// ITransform transform, IKeyOrder keyOrder) {
//
// super(capacity, relation, keyOrder);
//
// if (transform == null)
// throw new IllegalArgumentException();
//
// this.transform = transform;
//
// }
//
// @Override
// protected long flush(IChunkedOrderedIterator itr) {
//
// return getRelation().update(itr, transform);
//
// }
//
// }
}