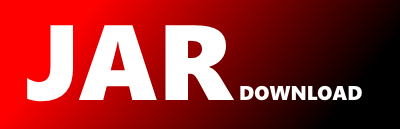
com.bigdata.relation.accesspath.EmptyAccessPath Maven / Gradle / Ivy
Show all versions of bigdata-core Show documentation
package com.bigdata.relation.accesspath;
import java.util.Collections;
import com.bigdata.bop.IPredicate;
import com.bigdata.btree.IIndex;
import com.bigdata.striterator.ChunkedWrappedIterator;
import com.bigdata.striterator.IChunkedOrderedIterator;
import com.bigdata.striterator.IKeyOrder;
/**
* An access path that is known to be empty.
*
* @author Bryan Thompson
* @version $Id$
*/
public class EmptyAccessPath implements IAccessPath {
private final IPredicate predicate;
private final IKeyOrder keyOrder;
/**
* Ctor variant does not specify the {@link #getPredicate()} or the
* {@link #getKeyOrder()} and those methods will throw an
* {@link UnsupportedOperationException} if invoked.
*/
public EmptyAccessPath() {
this(null/* predicate */, null/* keyOrder */);
}
/**
* Note: the {@link #getPredicate()} and {@link #getKeyOrder()} and methods
* will throw an {@link UnsupportedOperationException} if the corresponding
* argument is null.
*
* @param predicate
* @param keyOrder
*/
public EmptyAccessPath(IPredicate predicate, IKeyOrder keyOrder) {
// if (predicate == null)
// throw new IllegalArgumentException();
//
// if (keyOrder == null)
// throw new IllegalArgumentException();
this.predicate = predicate;
this.keyOrder = keyOrder;
}
/**
* {@inheritDoc}
* @throws UnsupportedOperationException
* unless the caller specified an {@link IPredicate} to the
* ctor.
*/
@Override
public IPredicate getPredicate() {
if (predicate == null)
throw new UnsupportedOperationException();
return predicate;
}
/**
* {@inheritDoc}
* @throws UnsupportedOperationException
* unless the caller specified an {@link IKeyOrder} to the ctor.
*/
@Override
public IKeyOrder getKeyOrder() {
if (keyOrder == null)
throw new UnsupportedOperationException();
return keyOrder;
}
/**
* {@inheritDoc}
* @throws UnsupportedOperationException
* since no index was selected.
*/
@Override
public IIndex getIndex() {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
* Always returns true
.
*/
@Override
public boolean isEmpty() {
return true;
}
/**
* {@inheritDoc}
Always returns ZERO(0).
*/
@Override
public long rangeCount(boolean exact) {
return 0;
}
// /**
// * Visits nothing.
// */
// @SuppressWarnings("unchecked")
// @Override
// public ITupleIterator rangeIterator() {
//
// return EmptyTupleIterator.INSTANCE;
//
// }
/**
* Visits nothing.
*/
@Override
public IChunkedOrderedIterator iterator() {
return iterator(0L/* offset */, 0L/* limit */, 0/* capacity */);
}
// /**
// * Visits nothing.
// */
// public IChunkedOrderedIterator iterator(int limit, int capacity) {
//
// return iterator(0L/* offset */, limit, capacity);
//
// }
/**
* Visits nothing.
*/
@Override
@SuppressWarnings("unchecked")
public IChunkedOrderedIterator iterator(final long offset,
final long limit, final int capacity) {
return new ChunkedWrappedIterator(Collections.EMPTY_LIST.iterator());
}
/**
* Does nothing and always returns ZERO(0).
*/
@Override
public long removeAll() {
return 0L;
}
}