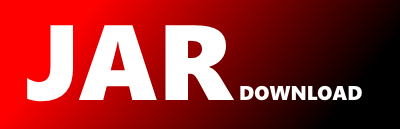
com.bigdata.relation.accesspath.IBlockingBuffer Maven / Gradle / Ivy
/*
Copyright (C) SYSTAP, LLC DBA Blazegraph 2006-2016. All rights reserved.
Contact:
SYSTAP, LLC DBA Blazegraph
2501 Calvert ST NW #106
Washington, DC 20008
[email protected]
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; version 2 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
/*
* Created on Jun 26, 2008
*/
package com.bigdata.relation.accesspath;
import java.util.concurrent.Future;
import java.util.concurrent.FutureTask;
import com.bigdata.relation.IMutableRelation;
import com.bigdata.relation.IRelation;
import com.bigdata.striterator.IChunkedIterator;
/**
*
* Interface provides an iterator to drain chunks from an {@link IBuffer}.
*
* CONOPS
*
* This interface is useful where one (or more) processes will write
* asynchronously on the {@link IBuffer} while another drains it via the
* {@link #iterator()}. For better performance in a multi-threaded environment,
* each thread is given an {@link UnsynchronizedArrayBuffer} of some capacity.
* The threads populate their {@link UnsynchronizedArrayBuffer}s in parallel
* using non-blocking operations. The {@link UnsynchronizedArrayBuffer}s in
* turn are configured to flush chunks of elements onto an either an
* {@link IBuffer} whose generic type is E[]
. Each element in
* the target {@link IBuffer} is therefore a chunk of elements from one of the
* source {@link UnsynchronizedArrayBuffer}s.
*
*
* There are two families of synchronized {@link IBuffer}s
*
*
* - An {@link IBuffer} that targets a mutable {@link IRelation};
* and
* - An {@link IBlockingBuffer} that exposes an {@link IAsynchronousIterator}
* for reading chunks of elements.
*
*
* This design means that blocking operations are restricted to chunk-at-a-time
* operations, primarily when an {@link UnsynchronizedArrayBuffer}<E>
* overflows onto an {@link IBuffer}<<E[]>
and when the
* {@link IBuffer}<<E[]>
either is flushed onto an
* {@link IMutableRelation} or drained by an {@link IChunkedIterator}.
*
*
* @author Bryan Thompson
* @version $Id$
* @param
* The generic type of the elements in the chunks.
*/
public interface IBlockingBuffer extends IRunnableBuffer {
/**
* Return an iterator reading from the buffer. It is NOT safe for concurrent
* processes to consume the iterator. The iterator will visit elements in
* the order in which they were written on the buffer, but note that the
* elements may be written onto the {@link IBlockingBuffer} by concurrent
* processes in which case the order is not predictable without additional
* synchronization.
*
* @return The iterator.
*/
public IAsynchronousIterator iterator();
/**
* This is a NOP since the {@link #iterator()} is the only way to consume
* data written on the buffer.
*
* @return ZERO (0L)
*/
public long flush();
/**
* Set the {@link Future} for the source processing writing on the
* {@link IBlockingBuffer} (the producer).
*
* Note: You should always wrap the task as a {@link FutureTask} and set the
* {@link Future} on the {@link IBlockingBuffer} before you start the
* consumer. This ensures that the producer will be cancelled if the
* consumer is interrupted.
*
* @param future
* The {@link Future}.
*
* @throws IllegalArgumentException
* if the argument is null
.
* @throws IllegalStateException
* if the future has already been set.
*
* @see
* BlockingBuffer.close() does not unblock threads
*
* @todo There should be a generic type for this.
*/
public void setFuture(Future future);
}