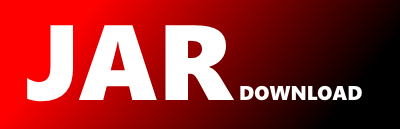
com.bigdata.relation.rule.eval.Solution Maven / Gradle / Ivy
/*
Copyright (C) SYSTAP, LLC DBA Blazegraph 2006-2016. All rights reserved.
Contact:
SYSTAP, LLC DBA Blazegraph
2501 Calvert ST NW #106
Washington, DC 20008
[email protected]
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; version 2 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
/*
* Created on Jun 24, 2008
*/
package com.bigdata.relation.rule.eval;
import java.io.Serializable;
import com.bigdata.bop.IBindingSet;
import com.bigdata.bop.IPredicate;
import com.bigdata.relation.IRelation;
import com.bigdata.relation.rule.IRule;
/**
* Flyweight implementation.
*
* @author Bryan Thompson
* @version $Id$
*/
public class Solution implements ISolution, Serializable {
/**
*
*/
private static final long serialVersionUID = 5477431033714540993L;
private final E e;
private final IRule rule;
private final IBindingSet bindingSet;
/**
* Constructs the element iff requested, saves the rule reference iff
* requested, and clones and saves the bindingSet iff requested. The
* requested behavior depends on {@link IJoinNexus#solutionFlags()}. When
* requested, the element is created using
* {@link IRelation#newElement(IPredicate, IBindingSet)} for the
* {@link IRelation} that is named by the head of the rule.
*
* @param e
* The element.
* @param rule
* The rule.
* @param bindingSet
* The binding set for this solution. If
* {@link IJoinNexus#BINDINGS} was specified, then the binding
* set will be cloned by this ctor.
*
* @throws IllegalArgumentException
* if any parameter is null
.
*/
@SuppressWarnings("unchecked")
public Solution(final IJoinNexus joinNexus, final IRule rule,
final IBindingSet bindingSet) {
if (joinNexus == null)
throw new IllegalArgumentException();
if (rule == null)
throw new IllegalArgumentException();
if (bindingSet == null)
throw new IllegalArgumentException();
final int flags = joinNexus.solutionFlags();
if ((flags & IJoinNexus.ELEMENT) != 0) {
/*
* The relation is responsible for how the elements are materialized
* from the bindings.
*
* Note: Caching for this relation is very important!
*/
// the head of the rule.
final IPredicate head = rule.getHead();
// the relation for the head of the rule.
final IRelation relation = joinNexus.getHeadRelationView(head);
// use the relation's element factory.
this.e = (E) relation.newElement(head.args(), bindingSet); // TODO BOp.args() is not efficient!
// // use the relation's element factory.
// this.e = (E) relation.newElement(head, bindingSet);
} else {
this.e = null;
}
if ((flags & IJoinNexus.BINDINGS) != 0) {
this.bindingSet = bindingSet.clone();
} else {
this.bindingSet = null;
}
if ((flags & IJoinNexus.RULE) != 0) {
this.rule = rule;
} else {
this.rule = null;
}
}
public E get() {
return e;
}
public IRule getRule() {
return rule;
}
public IBindingSet getBindingSet() {
return bindingSet;
}
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append("Solution");
sb.append("{ element=" + e);
sb.append(", rule=" + rule);
sb.append(", bindingSet=" + bindingSet);
sb.append("}");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy