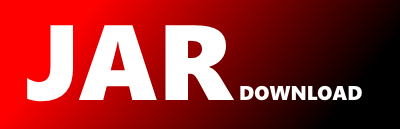
com.bigdata.resources.Priority Maven / Gradle / Ivy
package com.bigdata.resources;
/**
* Class used to place {@link ViewMetadata} objects into a total order based on
* a formula used to prioritize them for some kind of operation such as a
* compacting merge.
*
* The natural order of [Priority] is DESCENDING (largest numerical
* value to smallest numerical value)
*
* @author Bryan Thompson
* @version $Id$
* @param
*/
class Priority implements Comparable> {
public final double d;
public final T v;
public Priority(final double d, final T v) {
this.d = d;
this.v = v;
}
/**
* Places {@link Priority}s into descending order.
*/
public int compareTo(final Priority o) {
return d < o.d ? 1 : d > o.d ? -1 : 0;
}
// /**
// * Comparator places {@link Priority}s into ascending order (smallest
// * numerical value to largest numerical value).
// */
// public Comparator> asc() {
//
// return new Comparator>() {
//
// public int compare(Priority t, Priority o) {
//
// return t.d < o.d ? -1 : t.d > o.d ? 1 : 0;
//
// }
//
// };
//
// }
//
// /**
// * Comparator places {@link Priority}s into descending order (largest
// * numerical value to smallest numerical value).
// */
// public Comparator> dsc() {
//
// return new Comparator>() {
//
// public int compare(Priority t, Priority o) {
//
// return t.d < o.d ? 1 : t.d > o.d ? -1 : 0;
//
// }
//
// };
//
// }
}