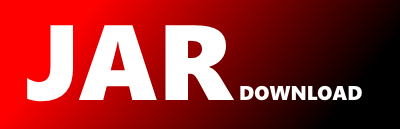
com.bigdata.search.MultiTokenHitCollector Maven / Gradle / Ivy
package com.bigdata.search;
import java.util.Collection;
import java.util.concurrent.ConcurrentHashMap;
import org.apache.log4j.Logger;
/**
* Multi-token implementation of {@link IHitCollector} backed by a
* {@link ConcurrentHashMap}.
*
* @author mikepersonick
*
* @param
* The generic type of the document identifier.
*/
public class MultiTokenHitCollector> implements IHitCollector {
protected static final transient Logger log = Logger.getLogger(MultiTokenHitCollector.class);
final ConcurrentHashMap> hits;
public MultiTokenHitCollector(final Collection> tasks) {
int initialCapacity = 0;
for (CountIndexTask task : tasks) {
final long rangeCount = task.getRangeCount();
if (rangeCount > Integer.MAX_VALUE) {
throw new RuntimeException("too many hits");
}
final int i = (int) rangeCount;
/*
* find the max
*/
if (i > initialCapacity) {
initialCapacity = i;
}
}
/*
* Note: The actual concurrency will be the #of distinct query
* tokens.
*/
final int concurrencyLevel = tasks.size();
if (log.isInfoEnabled()) {
log.info("initial capacity: " + initialCapacity);
}
hits = new ConcurrentHashMap>(initialCapacity,
.75f/* loadFactor */, concurrencyLevel);
}
@Override
public Hit putIfAbsent(V v, Hit hit) {
return hits.putIfAbsent(v, hit);
}
@Override
public Hit[] getHits() {
return hits.values().toArray(new Hit[hits.size()]);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy